As a Node.js developer, I have always been fascinated by the vast array of modules and packages that can be used to simplify the development process. One such package that has long intrigued me is the Net package. In this article, I’ll delve deep into what the Net package is, how to set it up, and how to use it to build powerful servers and clients.
What is the Net Package in Node.js?
The Net package is a built-in module in Node.js that provides a way to create TCP and HTTP servers and clients. It is essentially a wrapper around the core net
module that simplifies the process of creating and managing network connections.
What I find most fascinating about the Net package is how it can be used to create both TCP and HTTP servers and clients. This makes it incredibly versatile and useful when building web applications.
Setting up Net Package in Node.js
Before we dive into the specifics of the Net package, let’s first ensure that we have Node.js installed on our system. If you’re not sure whether Node.js is installed on your system, go to the command line and type node -v
. If you see a version number printed out, then you’re good to go!
Assuming you have Node.js installed, the first step in setting up the Net package is to initialize a new Node.js project. You can do this by navigating to an empty directory on your system and typing npm init
into the command line. This will start an interactive prompt that will ask you a few questions about your project, such as its name, version, and dependencies.
Once your Node.js project is set up, you can install the Net package by typing npm install net
into the command line. This will install the Net package and its dependencies into your project directory.
Now that the Net package is installed, we can start using it in our Node.js project.
Understanding the Net Package in Node.js
The Net package in Node.js consists of two main modules: the Server module and the Socket module.
The Server module is used to create TCP and HTTP servers. When you create a TCP server using the Server module, it listens on a specific port for incoming connections. When a connection is established, the server emits a connection
event that you can subscribe to in order to handle incoming data. Similarly, when you create an HTTP server using the Server module, it listens on a specific port for incoming HTTP requests. When a request is received, the server emits a request
event that you can subscribe to in order to handle the request.
The Socket module is used to create TCP and HTTP clients. When you create a TCP client using the Socket module, you can connect to a specific IP address and port on a remote server. Once the connection is established, you can send data to the remote server and receive data back. When you create an HTTP client using the Socket module, you can send HTTP requests to a remote server and receive HTTP responses back.
Building a Server with Net Package in Node.js
Now that we understand the basics of the Net package, let’s dive into some code examples of how to use it to build servers and clients.
Building a basic TCP server
The first example we’ll look at is how to build a basic TCP server using the Server module in the Net package. This server will simply listen for incoming connections and send a simple message back to the client.
const net = require('net');
const server = net.createServer(socket => {
console.log('Client connected');
socket.on('data', data => {
console.log(`Received data: ${data}`);
// send a response back to the client
socket.write(`You said: ${data}`);
});
socket.on('end', () => {
console.log('Client disconnected');
});
});
server.listen(3000, () => {
console.log('Server listening on port 3000');
});
In this example, we create a new TCP server using net.createServer()
. We pass a callback function to this method that is executed whenever a new client connects to the server.
Inside the callback function, we listen for incoming data using the socket.on('data', ...)
method. This method allows us to subscribe to incoming data and respond to it in whatever way we choose.
When we receive incoming data, we simply log it to the console and send a response back to the client using the socket.write()
method.
Finally, we listen for the end
event to be emitted by the client, indicating that they have disconnected from the server. When this happens, we log a message to the console indicating that the client has disconnected.
Building a basic HTTP server
The next example we’ll look at is how to build a basic HTTP server using the Server module in the Net package. This server will listen for incoming HTTP requests and send back a simple response.
const net = require('net');
const server = net.createServer(socket => {
console.log('Client connected');
socket.on('data', data => {
console.log(`Received data: ${data}`);
const response = `HTTP/1.1 200 OK
Content-Type: text/plain
Hello, world!`;
// send the HTTP response back to the client
socket.write(response);
socket.end();
});
socket.on('end', () => {
console.log('Client disconnected');
});
});
server.listen(3000, () => {
console.log('Server listening on port 3000');
});
In this example, we again create a new TCP server using net.createServer()
. We pass a callback function to this method that is executed whenever a new client connects to the server.
Inside the callback function, we listen for incoming data using the socket.on('data', ...)
method. This time, however, we are expecting an HTTP request to be sent to the server. When we receive this request, we construct an HTTP response and send it back to the client using the socket.write()
method. We then call socket.end()
to close the connection.
Building a HTTPS server
The final example we’ll look at is how to build a basic HTTPS server using the Server module in the Net package. This server will listen for incoming HTTPS requests and send back a simple response.
const fs = require('fs');
const https = require('https');
const net = require('net');
const options = {key: fs.readFileSync('server.key'),
cert: fs.readFileSync('server.cert')
};
const server = https.createServer(options, (req, res) => {
console.log('Received HTTPS request');
res.writeHead(200);
res.end('Hello, world!');
});
server.listen(3000, () => {
console.log('Server listening on port 3000');
});
In this example, we create an HTTPS server using the https.createServer()
method. We pass in an options object that specifies the path to the SSL key and certificate files.
Inside the callback function, we handle incoming HTTPS requests using the req
and res
objects, just like we would with a regular Node.js HTTP server.
This example is a bit simpler than the previous two, since HTTPS requests are essentially the same as HTTP requests, with the added security of SSL/TLS.
Building a Client with Net Package in Node.js
Now that we’ve looked at how to build servers using the Net package in Node.js, let’s turn our attention to clients.
Connecting to a TCP server from a client
The first example we’ll look at is how to connect to a TCP server from a client using the Socket module in the Net package.
const net = require('net');
const client = net.createConnection({ port: 3000 }, () => {
console.log('Connected to server');
// send some data to the server
client.write('Hello, server!');
});
client.on('data', data => {
console.log(`Received data from server: ${data}`);
});
client.on('end', () => {
console.log('Disconnected from server');
});
In this example, we create a new TCP client using net.createConnection()
. We pass in an options object that specifies the port number of the server we want to connect to.
Inside the callback function, we log a message to the console indicating that we’ve connected to the server. We then send some data to the server using the client.write()
method.
We subscribe to the data
event to handle incoming data from the server. When data is received, we log it to the console.
Finally, we subscribe to the end
event to handle the case where the server closes the connection.
Sending HTTP requests from a client
The next example we’ll look at is how to send HTTP requests from a client using the Socket module in the Net package.
const net = require('net');
const request = `GET / HTTP/1.1
Host: localhost:3000
`;
const client = net.createConnection({ port: 3000 }, () => {
console.log('Connected to server');
// send the HTTP request to the server
client.write(request);
});
client.on('data', data => {
console.log(`Received data from server: ${data}`);
});
client.on('end', () => {
console.log('Disconnected from server');
});
In this example, we create a new TCP client using net.createConnection()
, just like before. We again pass in an options object that specifies the port number of the server we want to connect to.
This time, however, we send an HTTP request to the server using the client.write()
method. We construct the HTTP request as a string and pass it in as the argument to client.write()
.
When we receive data back from the server, we log it to the console just like before.
Sending HTTPS requests from a client
The final example we’ll look at is how to send HTTPS requests from a client using the Socket module in the Net package.
const https = require('https');
const net = require('net');
const request = `GET / HTTP/1.1
Host: localhost:3000
`;
const options = {
hostname: 'localhost',
port: 3000,
path: '/',
method: 'GET'
};
const req = https.request(options, res => {
console.log(`Status code: ${res.statusCode}`);
res.on('data', d => {
process.stdout.write(d);
});
});
req.on('error', error => {
console.error(error);
});
req.write(request);
req.end();
In this example, we use the https
module to send an HTTPS request to the server. We construct a options
object that specifies the hostname, port, path, and method for the request.
We then use the https.request()
method to send the request and receive the response. We bind a callback function to the data
event of the response object to handle incoming data from the server.
Conclusion
In this article, we’ve explored the Net package in Node.js, including how to set it up and use it to build powerful servers and clients. We’ve seen how the Server and Socket modules can be used to create TCP and HTTP servers and clients, and we’ve looked at several code examples demonstrating how to implement these features.
While the Net package may seem daunting at first, especially for developers new to networking concepts, I encourage you to dive right in and experiment with it. By leveraging the power of the Net package, you can build powerful, high-performance web applications with ease. Good luck, and happy coding!
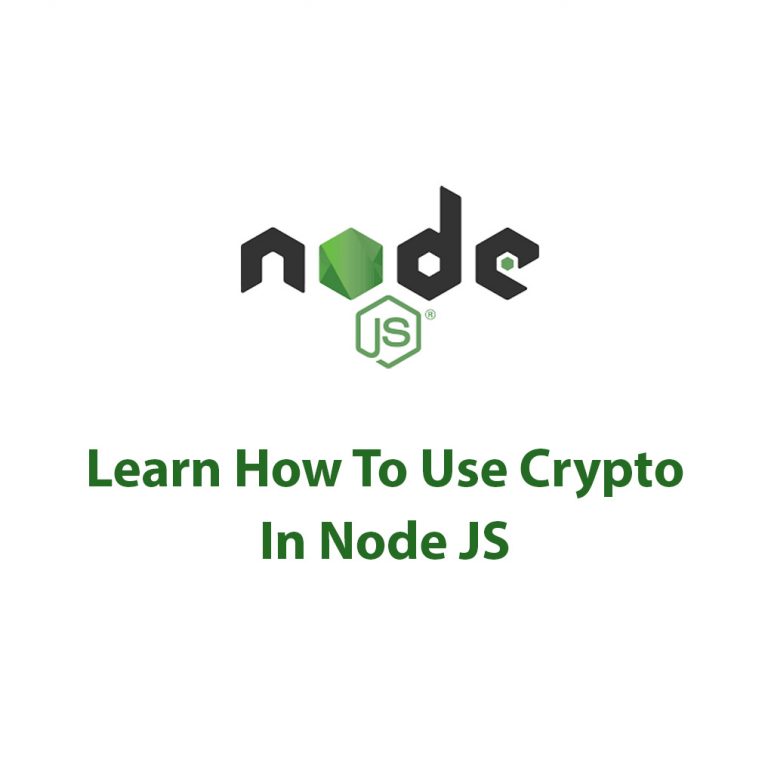
Using Crypto In Node JS
Have you ever wondered how some of the most secure websites and applications keep your data safe from malicious attacks? Well, one of the answers lies in the use of cryptography! Cryptography is the art of writing or solving codes and ciphers, and it has been around for centuries. In the world of computer science, […]
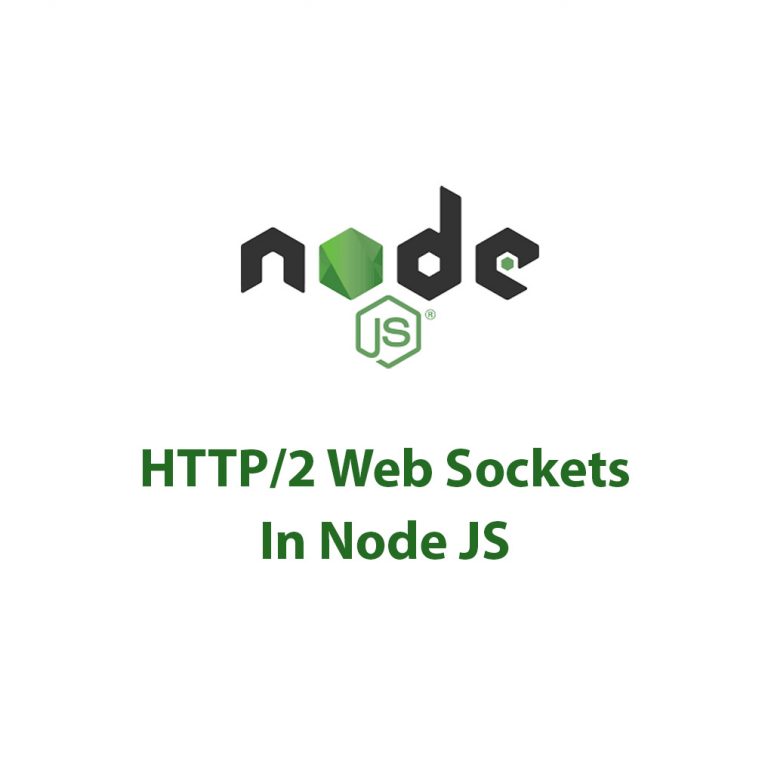
Working With HTTP/2 (Web Sockets) In Node JS
Introduction As a web developer, I’m always on the lookout for improvements in the technology that drives our web applications. Lately, HTTP/2 and WebSockets are getting a lot of attention for their potential to enhance web browsing experiences and make web applications even faster and more dynamic. Both of these specifications are a departure from […]
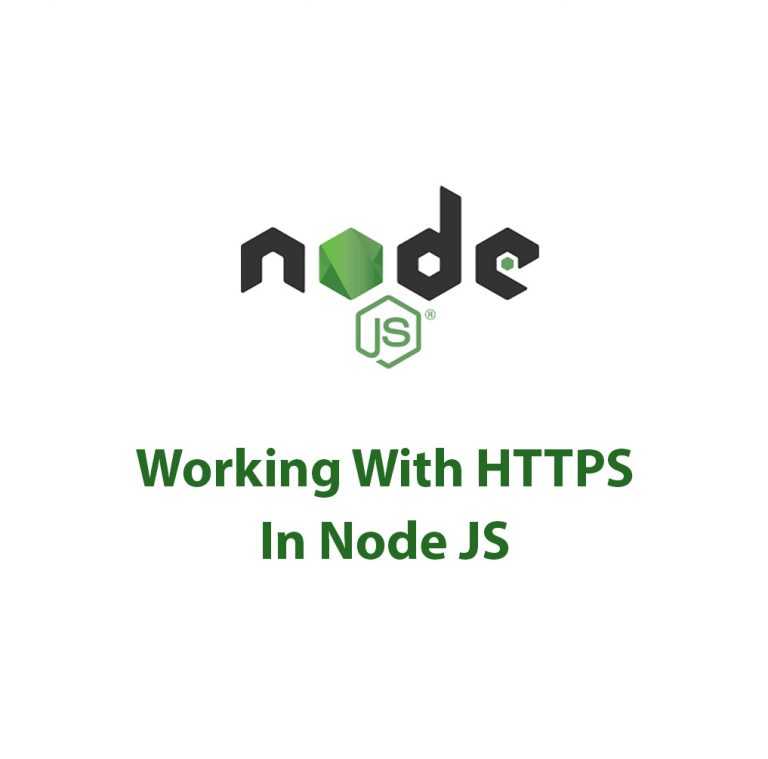
Working With HTTPS In Node JS
As a developer, I’m always on the lookout for security protocols that can help me protect users’ sensitive information. HTTP, while functional, lacks the encryption necessary to truly secure data transmission over the web. This is where HTTPS comes in. But working with HTTPS can be a bit daunting, especially if you’re new to it. […]
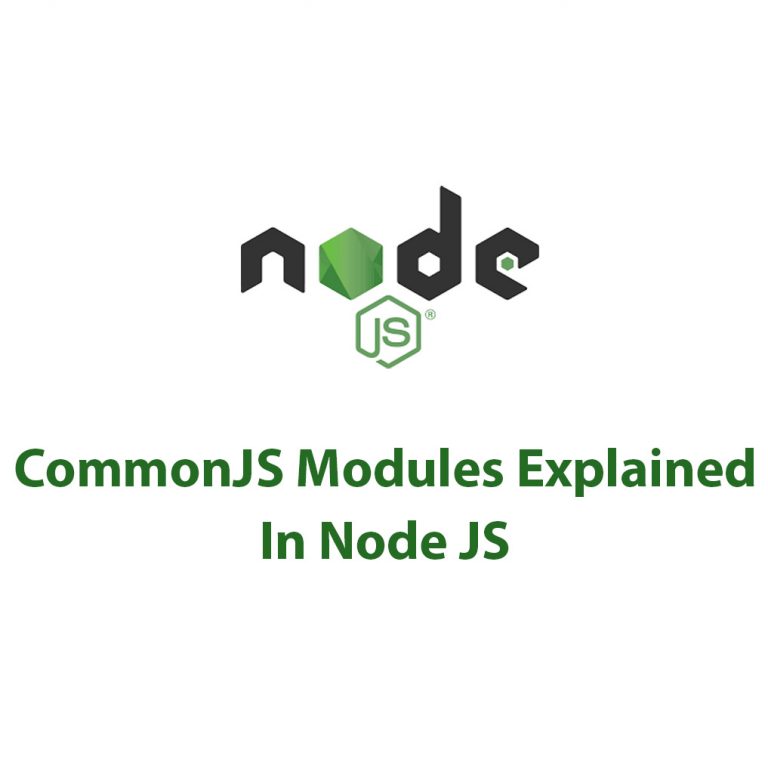
CommonJS Modules In Node JS
Introduction As a developer, I’ve always been interested in the ways that different technologies and tools can work together to create truly powerful and flexible applications. And one of the cornerstones of modern development is the use of modular code – breaking up large, complex programs into smaller, more manageable pieces. One technology that has […]
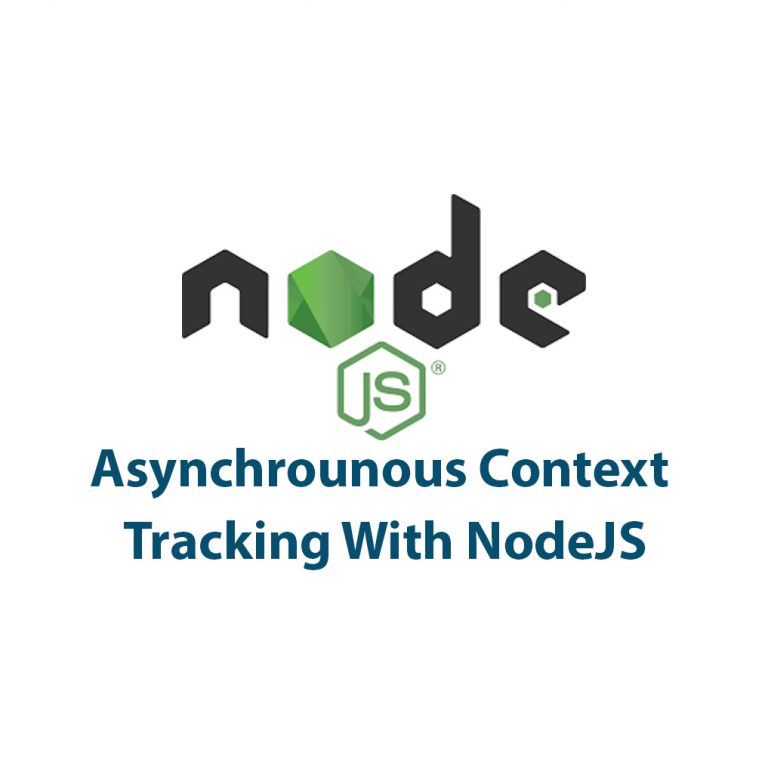
Asynchronous Context Tracking With Node JS
As someone who has spent a lot of time working with Node JS, I have come to understand the importance of Asynchronous Context Tracking in the development of high-performance applications. In this article, I will explore the concept of Asynchronous Context Tracking with Node JS, its advantages, techniques, challenges and best practices. Before we dive […]
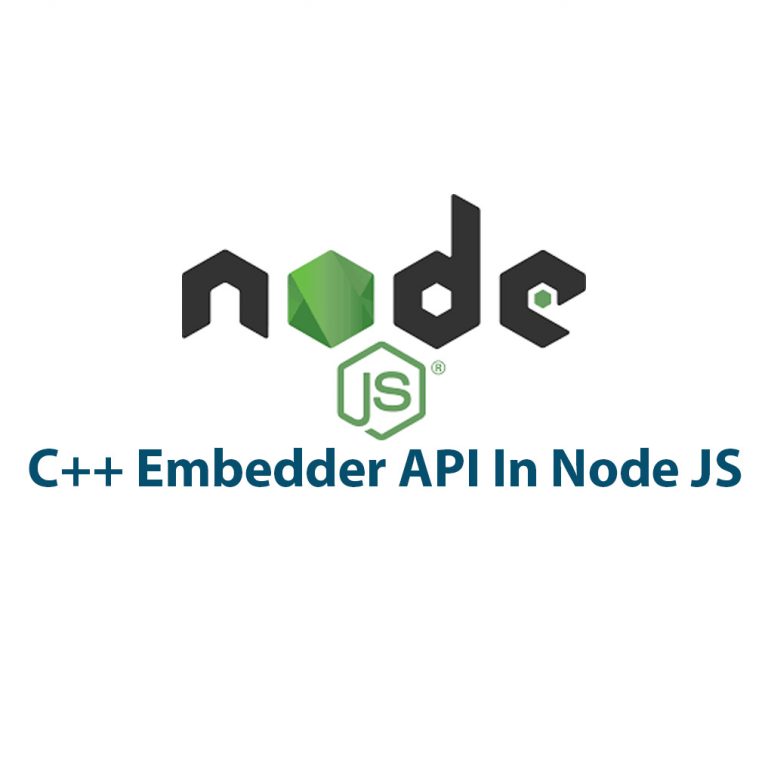
C++ Embedder API With Node JS
Introduction: As a programmer, I have always been fascinated with the power of NodeJS. It is a popular JavaScript runtime that can be used for server-side scripting. The beauty of NodeJS is that it allows for easy handling of I/O operations. However, sometimes the complexities of a project may go beyond just JavaScript coding, and […]