As a developer, I’m always on the lookout for tools and frameworks that can make my job easier. That’s why I love Material UI. This popular React UI component library provides a wealth of customizable and responsive components that can be easily integrated into any project. One useful component of Material UI is the backdrop component. In this article, I’ll explore everything there is to know about backdrops in Material UI V5.
What Are Backdrops?
If you’re not familiar with backdrops, they are simply a way to create a translucent screen that displays some sort of content on top. In Material UI, backdrops are often used as a way to visually enhance modal windows, sidebars, and loading indicators. They provide a smooth visual transition by covering the rest of the screen with a slightly translucent dark or gray screen.
Types of Backdrops in Material UI V5
Material UI V5 provides three types of backdrops: simple backdrops, translucent backdrops, and custom backdrops.
Simple Backdrop
The Simple Backdrop is the standard backdrop that covers the entire screen area. It has an opacity of 0.5 (or configurable with “opacity” prop) and a dark-gray color. This backdrop provides a simple and clean solution for modal windows, sidebars, and loading indicators.
To use a simple backdrop in Material UI V5, use the Backdrop
component and wrap it around the content you want to display. Here’s an example:
import { Backdrop } from '@mui/material';
function Example() {
const [openBackdrop, setOpenBackdrop] = useState(false);
return (
<>
<Button onClick={() => setOpenBackdrop(true)}>Open Backdrop</Button>
<Backdrop open={openBackdrop} onClick={() => setOpenBackdrop(false)} />
</>
);
}
In this example, we use a state hook to manage the openBackdrop
state. When the user clicks the “Open Backdrop” button, the setOpenBackdrop()
function is called with the value true
, which opens the backdrop. We also add an onClick
event handler to the backdrop to close it when the user clicks on it. The backdrop will fade in and fade out smoothly when opened or closed.
Translucent Backdrop
The Translucent Backdrop is similar to the Simple Backdrop, but it has a configurable opacity value. It’s useful when you want to draw attention to the content on top of the backdrop while still making the content below it slightly visible.
To use a translucent backdrop in Material UI V5, use the Backdrop
component and add the invisible
prop to the content you want to display on top of it. Here’s an example:
import { Backdrop } from '@mui/material';
function Example() {
const [openBackdrop, setOpenBackdrop] = useState(false);
return (
<>
<Button onClick={() => setOpenBackdrop(true)}>Open Backdrop</Button>
<Backdrop open={openBackdrop} onClick={() => setOpenBackdrop(false)} style={{ backgroundColor: 'rgba(255, 255, 255, 0.5)' }} />
<div style={{ position: 'absolute', top: '50%', left: '50%', transform: 'translate(-50%, -50%)', background: 'white', padding: 16 }}>
<h2 style={{ margin: '0px' }}>This is some content on top of the backdrop</h2>
<p>This content is styled with a white background</p>
</div>
</>
);
}
In this example, we use a state hook to manage the openBackdrop
state. When the user clicks the “Open Backdrop” button, the setOpenBackdrop()
function is called with the value true
, which opens the backdrop. We also add an onClick
event handler to the backdrop to close it when the user clicks on it. The backdrop has a white color and an opacity of 0.5.
The content on top of the backdrop is styled with a white background color and positioned in the center of the screen using the position
and transform
CSS properties. The invisible
prop is added to the content to make it appear on top of the translucent backdrop.
Custom Backdrop
The Custom Backdrop allows you to create a completely customizable backdrop with any combination of colors and styles. With this backdrop, you can create something that perfectly matches the design of your website or application.
To use a custom backdrop in Material UI V5, use the Backdrop
component and add your own styles. Here’s an example:
import { Backdrop } from '@mui/material';
function Example() {
const [openBackdrop, setOpenBackdrop] = useState(false);
return (
<>
<Button onClick={() => setOpenBackdrop(true)}>Open Backdrop</Button>
<Backdrop open={openBackdrop} onClick={() => setOpenBackdrop(false)} style={{ backgroundColor: '#E1E1E1', opacity: '0.9', zIndex: 9999 }} />
<div style={{ position: 'fixed', top: '50%', left: '50%', transform: 'translate(-50%, -50%)', background: 'white', padding: 16, zIndex: 10000 }}>
<h2 style={{ margin: '0px' }}>This is some custom content on top of the backdrop</h2>
<p>This content is styled with a white background</p>
</div>
</>
);
}
In this example, we use a state hook to manage the openBackdrop
state. When the user clicks the “Open Backdrop” button, the setOpenBackdrop()
function is called with the value true
, which opens the backdrop. We also add an onClick
event handler to the backdrop to close it when the user clicks on it.
The backdrop is styled with a light gray color and an opacity of 0.9 using the backgroundColor
and opacity
CSS properties. We also added a zIndex
value to ensure that the backdrop is displayed above other elements on the page.
The content on top of the backdrop is styled with a white background color and positioned in the center of the screen using the position
and transform
CSS properties. The zIndex
property is also added to ensure that the content is displayed above the backdrop.
How to Implement Backdrops in
Material UI V5
To use backdrops in your Material UI V5 project, you’ll first need to make sure that you’ve installed the Material UI package by running npm install @mui/material
in your project directory.
Once you’ve installed the package, you can import the Backdrop component from the @mui/material
package and start using it in your app.
Here’s a code snippet that demonstrates how to use the backdrop component:
import React, { useState } from 'react';
import { Button, Backdrop } from '@mui/material';
const App = () => {
const [openBackdrop, setOpenBackdrop] = useState(false);
return (
<>
<Button onClick={() => setOpenBackdrop(true)}>Open backdrop</Button>
<Backdrop
open={openBackdrop}
onClick={() => setOpenBackdrop(false)}
/>
</>
);
};
export default App;
In this example, we first import the Button
and Backdrop
components from Material UI. We then define our functional component, App
, that contains a state hook called openBackdrop
. This hook controls whether the backdrop is open or closed.
When we click the Open backdrop
button, we call the setOpenBackdrop
function with the value true
, which opens the backdrop using the open
prop. When we click on the backdrop itself, we close it by calling the setOpenBackdrop
function with the value false
.
Customization of Backdrops
Material UI V5 provides a lot of flexibility in terms of customizing your backdrops. Here are a few ways you can customize your backdrops:
CSS Styling
One way to customize your backdrops is to add CSS styles to your backdrop. This can be done by passing a style object to the style
prop on the Backdrop component. Here’s an example:
<Backdrop
open={openBackdrop}
onClick={() => setOpenBackdrop(false)}
style={{ backgroundColor: '#000', opacity: 0.5 }}
>
In this example, we’re setting the background color of the backdrop to black (#000
) and the opacity to 0.5, which will make it slightly translucent.
Props
Another way to customize your backdrops is to pass in props to the Backdrop component. Here are a few useful props you can use:
invisible
The invisible
prop can be used to make the content on top of the backdrop appear unrestricted. This can be useful when you want to display content such as dropdown menus or tooltips over the backdrop. When this prop is used, the backdrop will become invisible, but still be clickable. Here’s an example:
<Backdrop
open={openBackdrop}
onClick={() => setOpenBackdrop(false)}
invisible
>
TransitionComponent
The TransitionComponent
prop can be used to create custom transitions for your backdrops. This prop expects a component that accepts children
, in
, and appear
props as input. Here’s an example:
import { Backdrop, Fade } from '@mui/material';
<Backdrop
open={openBackdrop}
onClick={() => setOpenBackdrop(false)}
TransitionComponent={Fade}
TransitionProps={{ timeout: 600 }}
>
In this example, we’re using the Fade
component from Material UI for the transition. We’re also setting the timeout
prop on the TransitionProps
object to 600ms, which will make the transition last for 0.6 seconds.
Use Cases of Backdrops in Material UI V5
Backdrops can be used in a variety of ways in your Material UI V5 projects. Here are a few common use cases:
Modal Windows
One of the most common use cases for backdrops is in modal windows. Modal windows are windows that appear on top of another screen and require the user’s attention. By using a backdrop behind the modal window, you can give the illusion of the modal window being a separate window while still covering the rest of the screen.
Sidebars
Backdrops can also be used to enhance sidebars. When you open a sidebar, you can use a backdrop to dim the background and draw attention to the sidebar. This can be especially useful in mobile applications, where the screen size is limited.
Loading Indicators
Backdrops can also be used to display loading indicators. By covering the screen with a backdrop and displaying a loading indicator on top, you can give the user feedback that their action has been recognized and the app is currently doing something.
Best Practices for Backdrops in Material UI V5
To ensure that your backdrops are accessible and performant, here are a few best practices to follow:
Accessibility
When using a backdrop, it’s important to make sure that the backdrop is accessible to all users. This means that users who rely on screen readers or other assistive technologies should still be able to use your app with the backdrop on.
To make your backdrop accessible, make sure it has a visible and meaningful alternative text when it’s hovered over by the keyboard. You can add this text using the aria-label
attribute.
Performance
Backdrops can be performance-intensive, especially when they cover the entire screen. To improve performance, you can use the invisible
prop to make the backdrop invisible, but still clickable.
Another way to improve performance is to use the TransitionComponent
prop to customize the transition duration of the backdrop.
Customization
When customizing your backdrop, make sure that it still fits with the overall design of your app. A backdrop that is too different from the rest of your app can be distracting and confusing for users.
Also, keep accessibility in mind when customizing your backdrop. Make sure that any changes you make don’t affect the ability of users to interact with the backdrop.
Conclusion
Backdrops are a powerful tool to enhance the visual appeal and functionality of your Material UI V5 projects. With a variety of options for customization and implementation, backdrops can be used in a variety of use cases and offer a great way to visually communicate important information to your users. Remember to always follow best practices in terms of accessibility and performance and have fun experimenting with your backdrops!
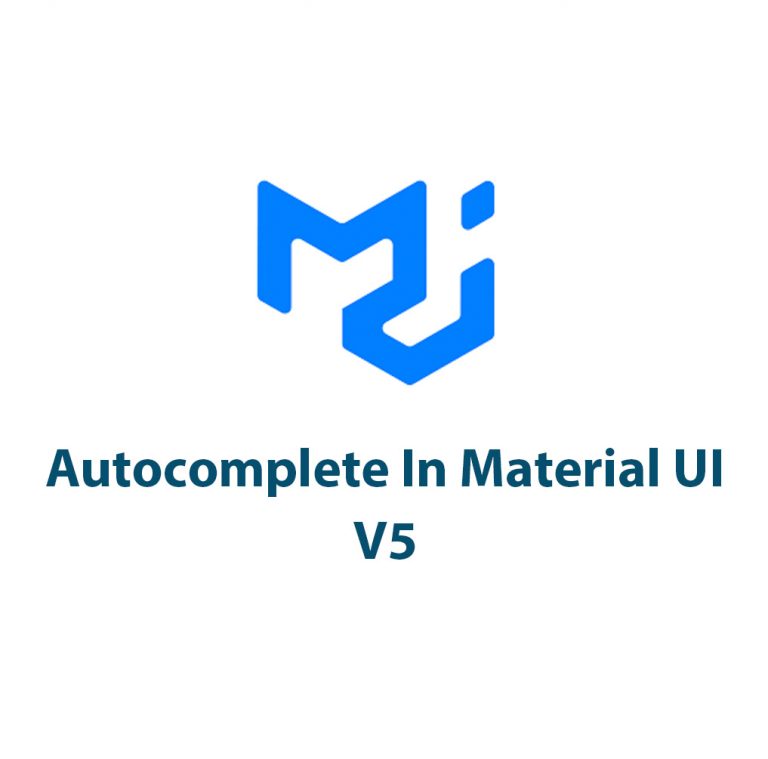
Autocomplete In Material UI V5
Introduction Hello everyone, today I am going to talk about one of the most useful components in Material UI V5 – Autocomplete. Material UI is a popular React UI framework that provides a wide range of pre-designed components to make the development of complex user interfaces simpler. Autocomplete is an intuitive component that provides suggestions […]
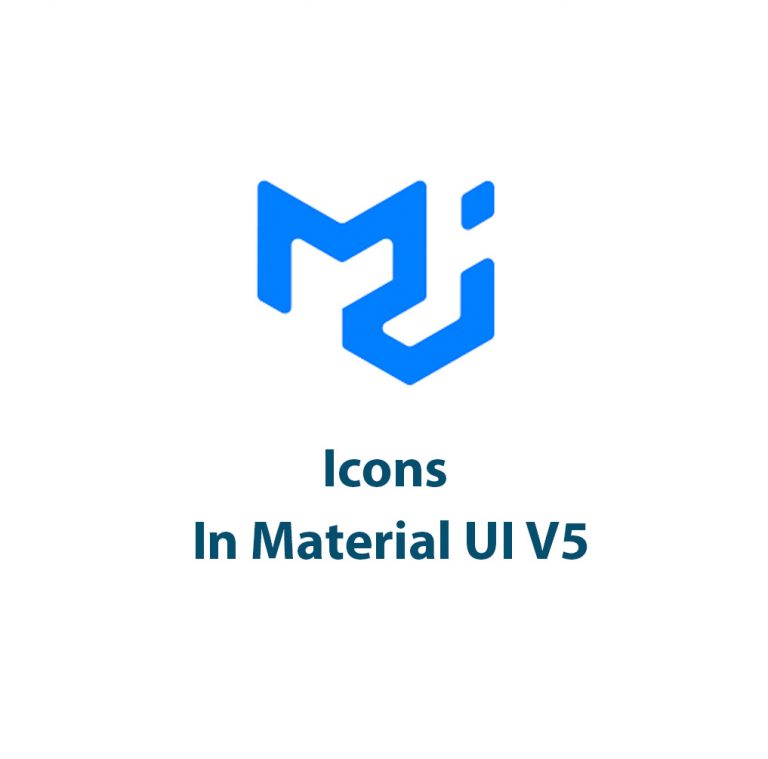
Icons In Material UI V5
Introduction: Material UI is a popular open-source framework for building web applications. Built on top of React, it provides a set of pre-built and customizable components, including typography, forms, and icons. In Material UI, icons play a crucial role in designing visually appealing interfaces and enabling smooth user experience. With the recent release of Material […]
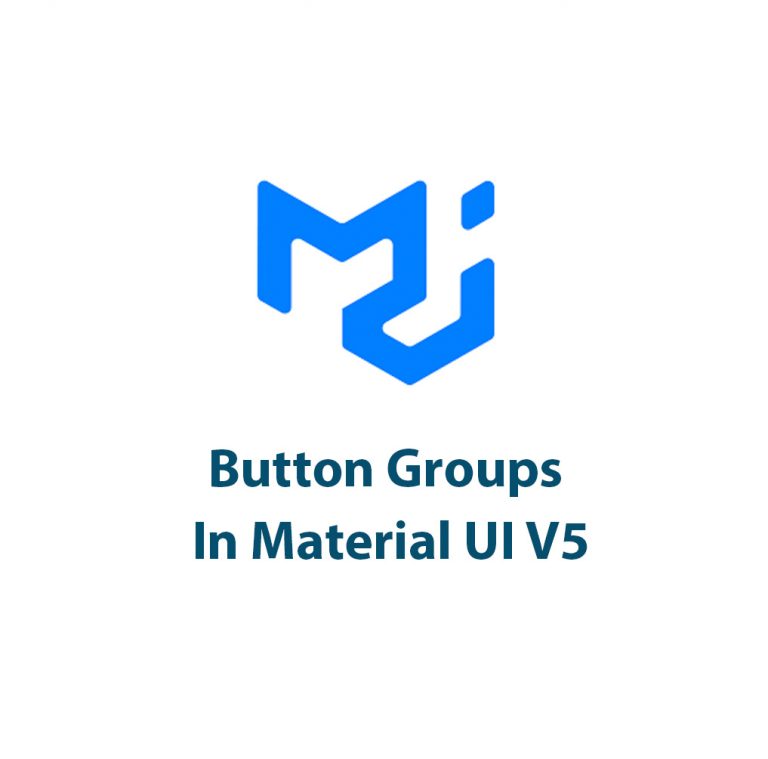
Button Groups In Material UI V5
Hey there! Welcome to my article all about button groups in Material UI V5. In this article, I’ll be giving you an in-depth insight into button groups, how they are used in UI designing, and how you can create them in Material UI V5. Introduction Before we dive into button groups, let’s start with some […]
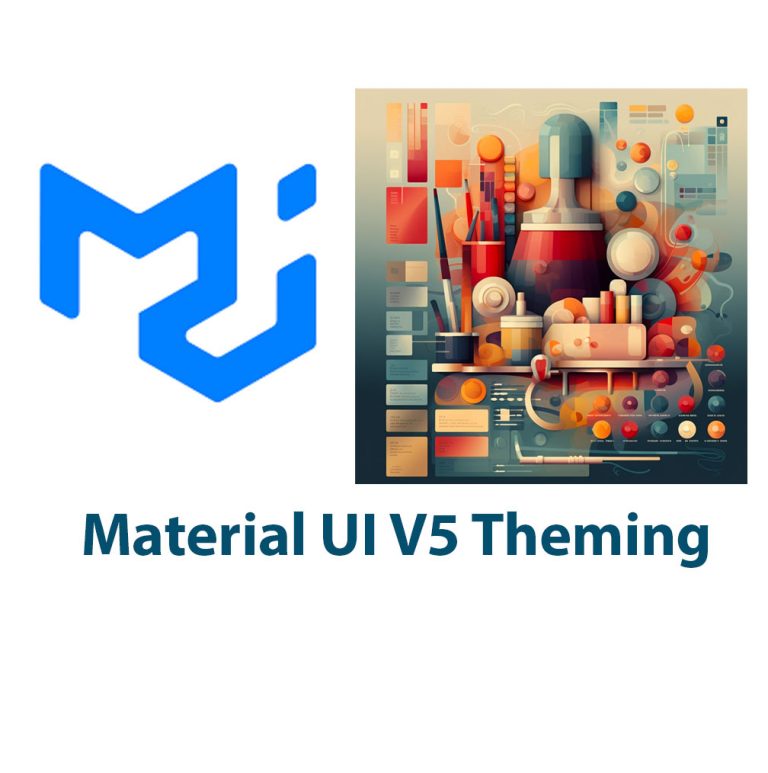
Material UI V5 Theming
Introduction: Popular React UI framework Material UI provides a large selection of pre-built components to easily build responsive user interfaces. The library’s design approach, which is based on Google’s Material Design principles, has helped it become more well-liked among developers. The design framework of Material UI is crucial to the creation of user interfaces since […]
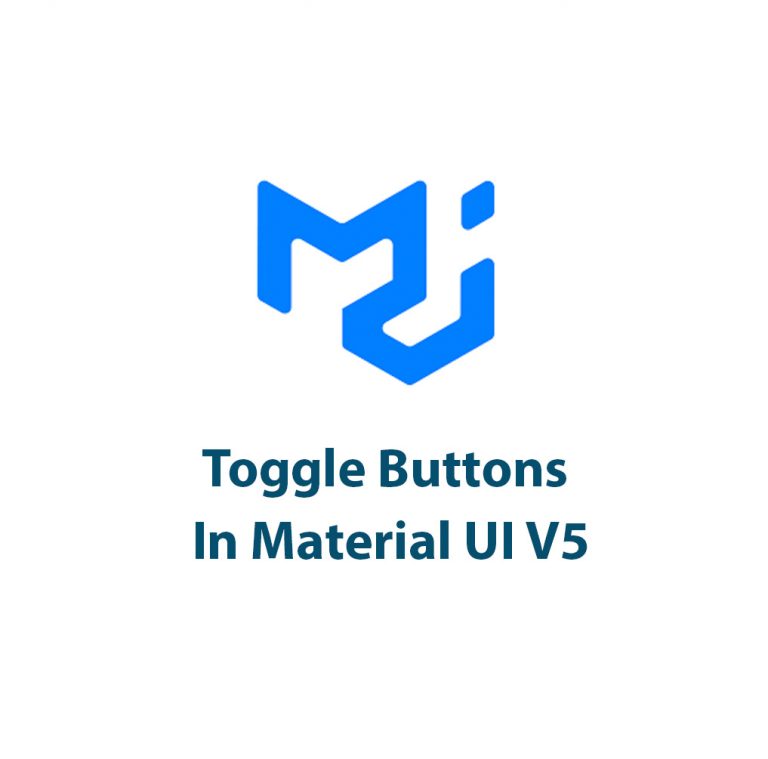
Toggle Buttons In Material UI V5
As a developer, I’ll be the first to admit that sometimes I find myself perplexed by certain UI design choices. As someone who spends a lot of time within the realm of code, I focus more on functionality and less on design. But the more I learn about UI design, the more I realize the […]
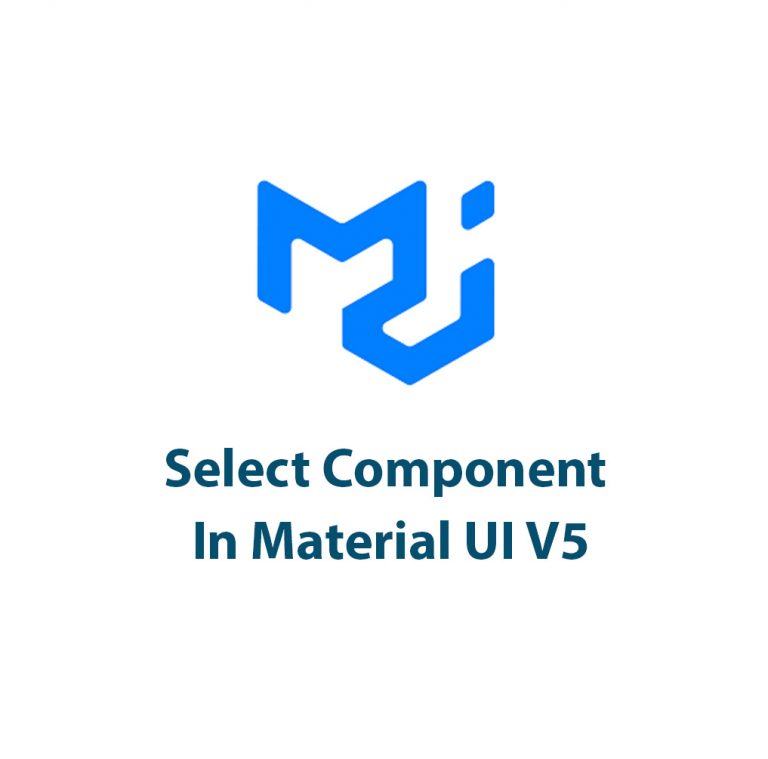
Select Component In Material UI V5
Hey there, fellow developers! In this article, we’re going to dive into the world of Material UI and take a closer look at one of its most essential components – the Select Component. I’m excited to share with you how to implement and customize this component in Material UI V5. So, let’s get started! Introduction […]