Introduction
Hello everyone, today I am going to talk about one of the most useful components in Material UI V5 – Autocomplete. Material UI is a popular React UI framework that provides a wide range of pre-designed components to make the development of complex user interfaces simpler.
Autocomplete is an intuitive component that provides suggestions while the user types into a text field. The suggestions are usually based on previous search history or predefined options. This allows users to quickly find the options they want, and reduces the need for long lists and dropdown menus.
In this article, I will be discussing the benefits of using Autocomplete in Material UI V5, how to set it up, advanced features, best practices, and future prospects.
Benefits of using Autocomplete in Material UI V5
Improved User Experience
As mentioned earlier, Autocomplete provides suggestions while the user types, making the search process more efficient, dynamic, and user-friendly. By minimizing the need to manually select options from long lists or dropdown menus, Autocomplete makes the user experience more seamless.
Efficient and Dynamic
With Autocomplete, the user can easily search through options based on what they type in, rather than scrolling through long lists or opening dropdowns. Furthermore, if the user makes a mistake, Autocomplete will automatically correct
the input, providing a better search experience. This dynamic and efficient search approach can save users a lot of time and effort.
Built-in Validation and Error Handling
Another key advantage of Autocomplete in Material UI V5 is its built-in validation and error handling. It ensures that users can only choose valid options and that the selected value is accurate. This makes the form submission more reliable and prevents errors or invalid input.
Setting up Autocomplete in Material UI V5
Now let’s dive into how to set up Autocomplete in Material UI V5. The setup process is simple and straightforward, even for beginners.
Basic Implementation
First, we need to install Material UI V5 by running the following command in the terminal:
npm install @mui/material @emotion/react @emotion/styled
Next, we can import the Autocomplete component from @mui/material/Autocomplete
. Here’s a simple example that shows how to use Autocomplete:
import * as React from 'react';
import Autocomplete from '@mui/material/Autocomplete';
import TextField from '@mui/material/TextField';
const options = ['Option 1', 'Option 2', 'Option 3'];
export default function BasicAutocomplete() {
return (
<Autocomplete
options={options}
renderInput={(params) => (
<TextField
{...params}
label="Choose an option"
/>
)}
/>
);
}
In this example, we’re using the basic Autocomplete component to display a dropdown list of options. options
is an array of predefined options that we want to display. The renderInput
prop is used to render the text input field.
Customization Options
Material UI Autocomplete provides a variety of customization options that allow you to tailor the component to your specific needs. Some of the customization options include:
getOptionLabel
This prop is used to define how the options in the Autocomplete list will be labeled. The default value is the option object’s label property, but you can customize it to suit your needs.renderOption
This prop is used to define how the options in the Autocomplete list will be rendered. By default, the options are displayed in a simple list, but withrenderOption
, you can customize how they look and feel.filterOptions
This prop is used to define a custom filtering function that will be applied to the available options. This is useful when you want to customize how filtering is done for specific use cases.
Here’s an example of how to use some of these customization options:
import * as React from 'react';
import TextField from '@mui/material/TextField';
import Autocomplete, { createFilterOptions } from '@mui/material/Autocomplete';
const filter = createFilterOptions();
function CustomAutocomplete() {
const [value, setValue] = React.useState(null);
return (
<Autocomplete
value={value}
onChange={(event, newValue) => {
if (typeof newValue === 'string') {
setValue({
label: newValue,
});
} else if (newValue && newValue.inputValue) {
// Create a new value from the user's input
setValue({
label: newValue.inputValue,
});
} else {
setValue(newValue);
}
}}
filterOptions={(options, params) => {
const filtered = filter(options, params);
if (params.inputValue !== '') {
filtered.push({
inputValue: params.inputValue,
label: `Add "${params.inputValue}"`,
});
}
return filtered;
}}
selectOnFocus
clearOnBlur
handleHomeEndKeys
id="free-solo-with-text-demo"
options={options}
getOptionLabel={(option) => {
// Value selected with enter, right from the input
if (typeof option === 'string') {
return option;
}
// Add "xxx" option created dynamically
if (option.inputValue) {
return option.inputValue;
}
// Regular option
return option.label;
}}
renderOption={(props, option) => <li {...props}>{option.label}</li>}
freeSolo
renderInput={(params) => (
<TextField {...params} label="Custom Search" />
)}
/>
);
}
In this example, we’re using createFilterOptions
to customize the filtering options for the Autocomplete component. We’re also using getOptionLabel
, renderOption
, and renderInput
to customize the appearance and functionality of the Autocomplete component.
Custom Filtering Options
One of the most powerful features of Autocomplete in Material UI V5 is the ability to customize filtering options. This allows you to define how the component should filter the available options based on the user’s input.
There are two ways to customize filtering options: by passing in a custom filtering function to the filterOptions
prop or by defining a custom options
array with the desired filtering options.
Here’s an example of how to use a custom filtering function to filter the options based on the user’s input:
import * as React from 'react';
import TextField from '@mui/material/TextField';
import Autocomplete from '@mui/material/Autocomplete';
const options = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
{ label: 'Other Option', value: 'other' },
];
const filterOptions = (options, { inputValue }) =>
options.filter((option) =>
option.label.toLowerCase().includes(inputValue.toLowerCase())
);
export default function CustomFiltering() {
const [value, setValue] = React.useState(null);
return (
<Autocomplete
value={value}
onChange={(event, newValue) => {
setValue(newValue);
}}
filterOptions={filterOptions}
options={options}
getOptionLabel={(option) => option.label}
renderInput={(params) => (
<TextField {...params} label="Custom Filtering" />
)}
/>
);
}
In this example, we’re defining a custom filtering function called filterOptions
that filters the available options based on the user’s input. This function takes the available options and the user’s input as parameters and returns a filtered array of options based
on the filtering logic. We’re also passing this function to the filterOptions
prop to make use of it in the Autocomplete component.
Handling Selected Values
Handling selected values in Autocomplete is easy. When a user selects an option from the dropdown list, the Autocomplete component returns an event object that contains the selected value.
Here’s an example of how to handle selected values in Autocomplete:
import * as React from 'react';
import TextField from '@mui/material/TextField';
import Autocomplete from '@mui/material/Autocomplete';
const options = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
{ label: 'Other Option', value: 'other' },
];
export default function HandleSelection() {
const [value, setValue] = React.useState(null);
return (
<Autocomplete
value={value}
onChange={(event, newValue) => {
setValue(newValue);
}}
options={options}
getOptionLabel={(option) => option.label}
renderInput={(params) => (
<TextField {...params} label="Handle Selection" />
)}
/>
);
}
In this example, we’re using the onChange
event to handle selected values. As soon as the user selects an option from the dropdown list, the onChange
event fires, and the selected value is stored in the newValue
argument. We’re using this argument to update the component state and display the selected value in the input field.
Advanced Features of Autocomplete in Material UI V5
Grouped Options
Autocomplete in Material UI V5 also supports grouping options, which allows you to categorize the available options and present them in a more organized way.
Here’s an example of how to use grouped options in Autocomplete:
import * as React from 'react';
import TextField from '@mui/material/TextField';
import Autocomplete, { groupedOptions } from '@mui/material/Autocomplete';
const options = [
{ label: 'Group 1', options: [{ label: 'Option 1', value: 'option1' }] },
{ label: 'Group 2', options: [{ label: 'Option 2', value: 'option2' }] },
];
export default function GroupedOptions() {
const [value, setValue] = React.useState(null);
return (
<Autocomplete
value={value}
onChange={(event, newValue) => {
setValue(newValue);
}}
options={groupedOptions(options, (option) => option.options)}
getOptionLabel={(option) => option.label}
renderInput={(params) => (
<TextField {...params} label="Grouped Options" />
)}
/>
);
}
In this example, we’re using the groupedOptions
helper function from Material UI to group the available options based on their categories. We’re then using this grouped options object in the Autocomplete component to display the options in a categorized list.
Free Solo Mode
Free solo mode is another useful feature of Autocomplete in Material UI V5. It allows users to enter values that are not in the predefined options list.
Here’s an example of how to use free solo mode in Autocomplete:
import * as React from 'react';
import TextField from '@mui/material/TextField';
import Autocomplete from '@mui/material/Autocomplete';
const options = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
];
export default function FreeSolo() {
const [value, setValue] = React.useState(null);
return (
<Autocomplete
value={value}
freeSolo
onChange={(event, newValue) => {
setValue(newValue);
}}
options={options}
getOptionLabel={(option) => option.label}
renderInput={(params) => (
<TextField {...params} label="Free Solo" />
)}
/>
);
}
In this example, we’re using the freeSolo
prop to enable free solo mode in the Autocomplete component. This allows users to enter any input value, even if it’s not in the predefined options list.
Multiple Autocomplete
Another advanced feature of Autocomplete in Material UI V5 is the ability to use multiple autocomplete components simultaneously.
Here’s an example of how to use multiple autocomplete in Material UI V5:
import * as React from 'react';
import TextField from '@mui/material/TextField';
import Autocomplete from '@mui/material/Autocomplete';
const options1 = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
];
const options2 = [
{ label: 'Option 3', value: 'option3' },
{ label: 'Option 4', value: 'option4' },
];
export default function MultipleAutocomplete() {
const [value1, setValue1] = React.useState(null);
const [value2, setValue2] = React.useState(null);
return (
<div>
<Autocomplete
value={value1}
onChange={(event, newValue) => {
setValue1(newValue);
}}
options={options1}
getOptionLabel={(option) => option.label}
renderInput={(params) => (
<TextField {...params} label="Autocomplete 1" />
)}
/>
<Autocomplete
value={value2}
onChange={(event, newValue) => {
setValue2(newValue);
}}
options={options2}
getOptionLabel={(option) => option.label}
renderInput={(params) => (
<TextField {...params} label="Autocomplete 2" />
)}
/>
</div>
);
}
In this example, we’re using two Autocomplete components simultaneously, each with their options and labels.
Auto-Highlighting Results
Another useful feature of Autocomplete in Material UI V5 is auto-highlighting results. This makes it easier for users to quickly see which part of their search query matches the available options.
Here’s an example of how to use auto-highlighting results in Autocomplete:
Autocomplete from ‘@mui/material/Autocomplete’;
const options = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
{ label: 'Other Option', value: 'other' },
];
export default function AutoHighlight() {
const [value, setValue] = React.useState(null);
return (
{
setValue(newValue);
}}
options={options}
getOptionLabel={(option) => option.label}
renderInput={(params) => (
)}
autoHighlight
/>
);
}
In this example, we’re using the autoHighlight
prop to enable auto-highlighting for the Autocomplete component. This makes it easier for users to see the available options that match their search query.
Conclusion
In conclusion, Autocomplete in Material UI V5 is a powerful and versatile component that provides a range of features for building dynamic and user-friendly search experiences. By making use of the customization options and advanced features available in Material UI, developers can create intuitive and efficient search interfaces that enhance the user experience and improve overall website functionality.
So, go ahead and try out Autocomplete in Material UI V5 for your next project and explore the full range of features and capabilities it has to offer. With the right expertise and creativity, you can build search experiences that are truly exceptional.
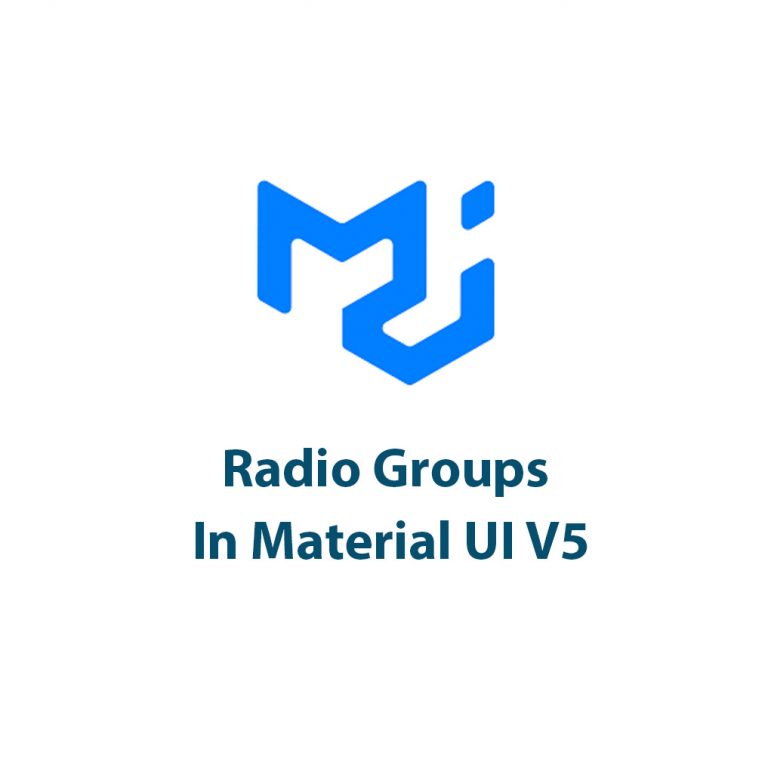
Radio Groups In Material UI V5
Introduction Hello everyone! Material UI has long been one of my favorite UI libraries, and with the recent release of version 5, I thought it would be a great time to explore one of its fundamental components: Radio Groups. In this article, we’ll dive into what Radio Groups are, how they can be used in […]
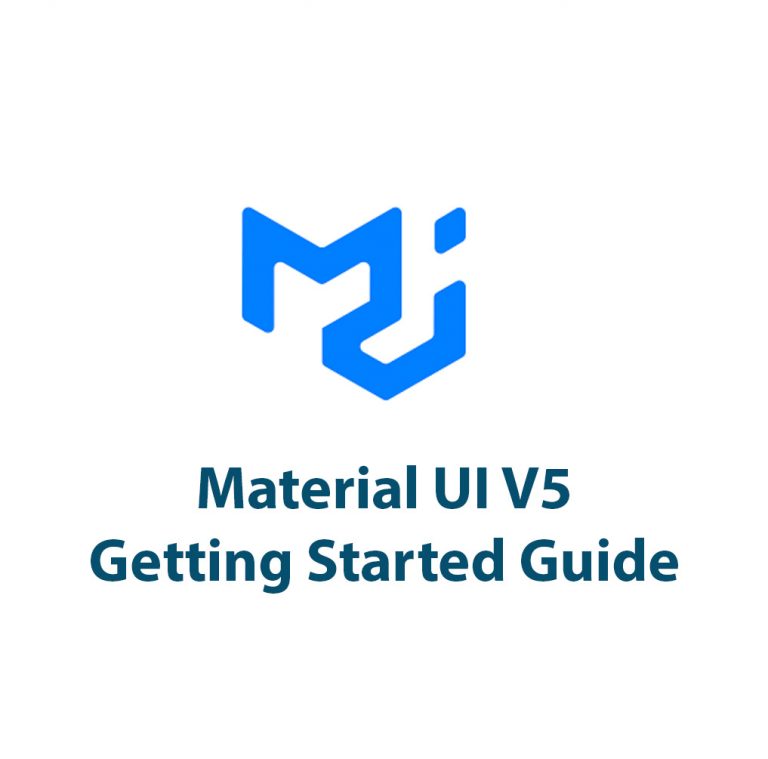
Material UI V5: Getting Started Guide
Introduction Hello, fellow online professionals! Are you prepared to explore the fascinating Material UI V5 world? You’ve come to the correct place if you’re trying to improve the appearance and feel of your online applications. The powerful framework that implements Google’s Material Design principles, Material UI V5, will be demonstrated to you step-by-step in this […]
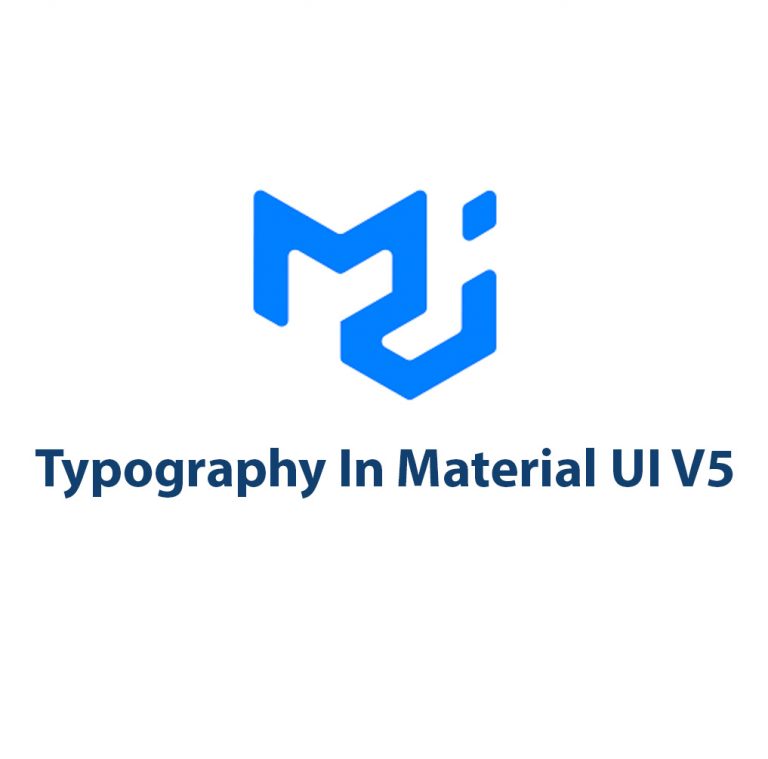
Typography In Material UI V5: A Closer Look
Introduction As a designer and developer, I’ve always been obsessed with typography. There’s something special about the way certain fonts look on a page or screen that can make or break the overall success of a design. As Material UI continues to evolve and improve, the latest version, Material UI V5, offers some exciting changes […]
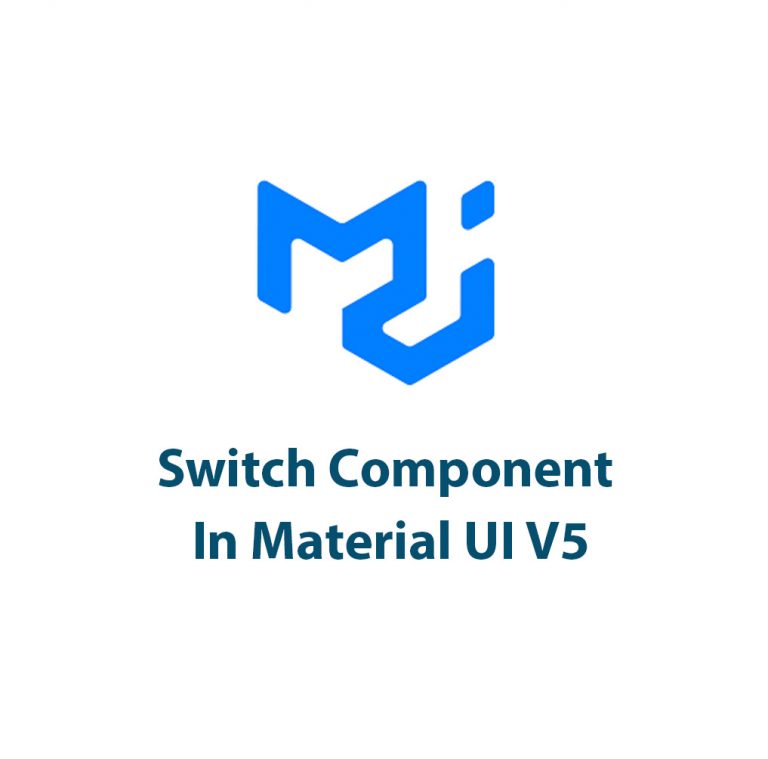
Switch Component In Material UI V5
Hey there, fellow developers! Are you excited about exploring the latest version of Material UI and all the new features it brings? If so, you’ve landed in the right place because today we’re going to dive deep into the Switch Component in Material UI v5. Introduction Before we get into the nitty-gritty of the Switch […]
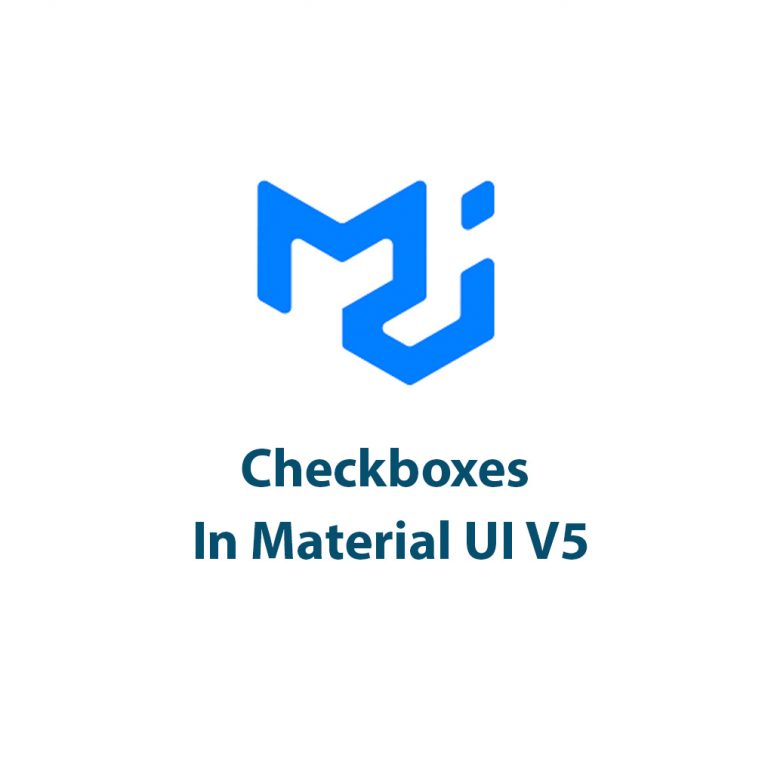
Checkboxes In Material UI V5
Hey there folks! If you’re reading this, chances are you are already familiar with Material UI, an immensely popular UI library for creating beautiful React components. One of the most common elements in user interfaces is a checkbox. In this article, we’ll explore how you can use checkboxes in Material UI to create user-friendly and […]
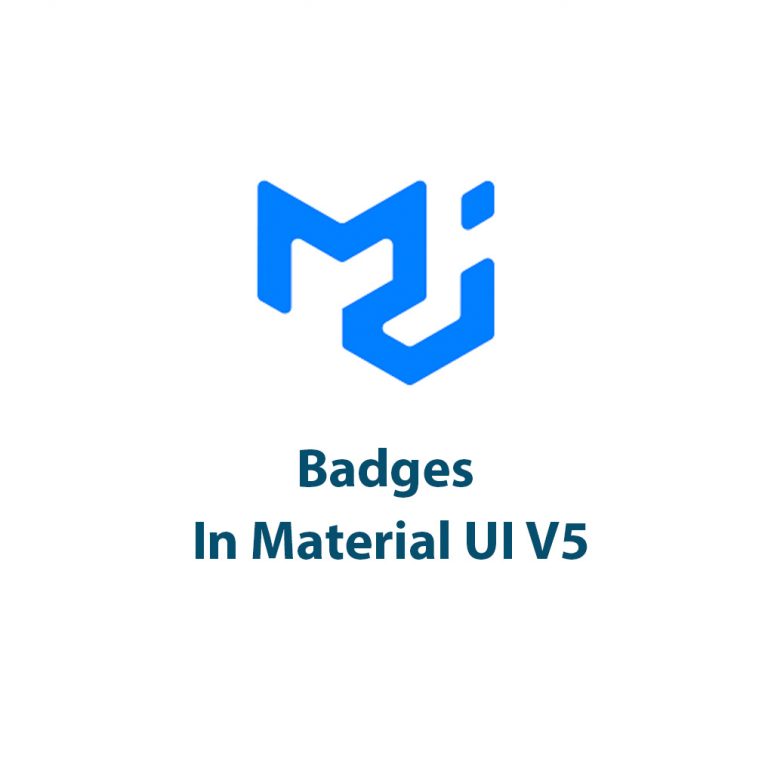
Badges In Material UI V5
As a web developer, I’ve always been fascinated by the small design elements that can make a big impact on the overall user experience of a website. One such element is the badge, often used to highlight new or important information to users. In the latest release of Material UI, version 5, badges have seen […]