Hey there folks! If you’re reading this, chances are you are already familiar with Material UI, an immensely popular UI library for creating beautiful React components. One of the most common elements in user interfaces is a checkbox. In this article, we’ll explore how you can use checkboxes in Material UI to create user-friendly and customizable experiences for your users.
Before we dive into the nitty-gritty details of Material UI’s Checkbox component, let’s quickly cover the basics of getting started with Material UI.
To use Material UI, you first need to install it in your project. You can install it by running the following command in your terminal:
npm install @mui/material
Once Material UI is installed, you can import the necessary modules for using checkboxes:
import Checkbox from '@mui/material/Checkbox';
import FormControlLabel from '@mui/material/FormControlLabel';
Great! Now let’s move onto the fun part: Material UI’s Checkbox API.
Understanding Material UI’s Checkbox API
Material UI’s Checkbox component is incredibly flexible and comes with a large set of props, making it easy to customize checkboxes to suit your needs.
To use a basic Material UI Checkbox, all you need to do is simply render it in your JSX code:
<Checkbox />
By default, the Checkbox is unchecked. To check it, you can use the checked
prop:
<Checkbox checked />
You can also customize the Checkbox by changing its color, size and style using the various props. For example, you can change its color by passing the color
prop:
<Checkbox color="primary" />
You can also disable the Checkbox by using the disabled
prop:
<Checkbox disabled />
Types of Material UI Checkboxes
Material UI provides three common types of checkboxes: default, checked, and disabled checkboxes.
The default checkbox is the most basic one. It is unchecked by default, and can be checked or unchecked by the user.
<Checkbox />
The checked checkbox is pre-checked. It is often used to indicate that a certain option is selected by default. You can create a checked checkbox by passing the checked
prop:
<Checkbox checked />
The disabled checkbox is a checkbox that users can’t interact with. It’s often used to indicate that a certain option cannot be selected. You can create a disabled checkbox by passing the disabled
prop:
<Checkbox disabled />
Customizing Material UI Checkboxes
One of the best features of Material UI’s Checkbox component is its flexibility. You can easily customize a Checkbox to fit your design needs.
Adding custom label text and styles
You can add custom label text to your Checkbox using the FormLabelControl
component:
<FormControlLabel control={<Checkbox />} label="Label Text" />
You can also style the label using the label
prop:
<FormControlLabel control={<Checkbox />} label="Label Text" labelPlacement="end" />
Changing Checkbox color and ripple effect
You can customize the color of your Checkbox by using the color
prop:
<Checkbox color="primary" />
You can also change the ripple effect using the disableRipple
prop:
<Checkbox disableRipple />
Customizing Material UI Checkbox Styles
You can customize the style of your Checkbox by using the style
prop:
<Checkbox style={{ color: "#f44336" }} />
Handling state in Material UI Checkboxes
When using a Checkbox in your UI, you may want to track its state in order to perform certain actions based on whether or not the Checkbox is checked. That’s where the value
and onChange
prop come into play.
Understanding value and onChange attributes
The value
prop lets you define the current value of your Checkbox. You can set it to either true or false depending on your use case.
<Checkbox checked={myCheckboxValue} onChange={() => setMyCheckboxValue(!myCheckboxValue)} />
The onChange
prop lets you define a function to be called every time the Checkbox is clicked. This function should update the state of your UI.
Handling state in controlled and uncontrolled checkboxes
In React, you can manage Checkbox state in two ways: controlled and uncontrolled.
In controlled component, the state of your Checkbox is managed by React. You need to pass the current state of your Checkbox and an onChange function.
const [isChecked, setIsChecked] = useState(false);
<Checkbox checked={isChecked} onChange={(e) => setIsChecked(e.target.checked)} />;
In uncontrolled component, the state of your Checkbox is managed by the browser. You don’t need to pass the current value of your Checkbox or an onChange function.
<Checkbox defaultChecked />;
Best practices when using Material UI Checkboxes
While Checkboxes can be a useful tool in UI design, it’s important to follow best practices to ensure you maintain a good user experience.
Keep labels concise
When adding a label to your Checkbox, it’s important to keep it concise. Longer labels can make your UI cluttered and difficult to navigate.
Consider accessibility
Accessibility is important when designing any UI component, and Checkboxes are no exception. Make sure your Checkbox is accessible to all users with disabilities by adding appropriate aria-labels
.
Avoid using checkboxes for non-binary choices
Checkboxes are best suited for binary choices (i.e., true or false). If you have more than two options, consider using a different UI element, such as a radio button or a dropdown menu.
Conclusion
Material UI’s Checkbox component is a powerful tool that can help you create beautiful and user-friendly UIs. By following best practices and customizing your Checkboxes to suit your needs, you can ensure that your users have a great experience. So go forth, create beautiful interfaces with Material UI Checkboxes, and happy coding!
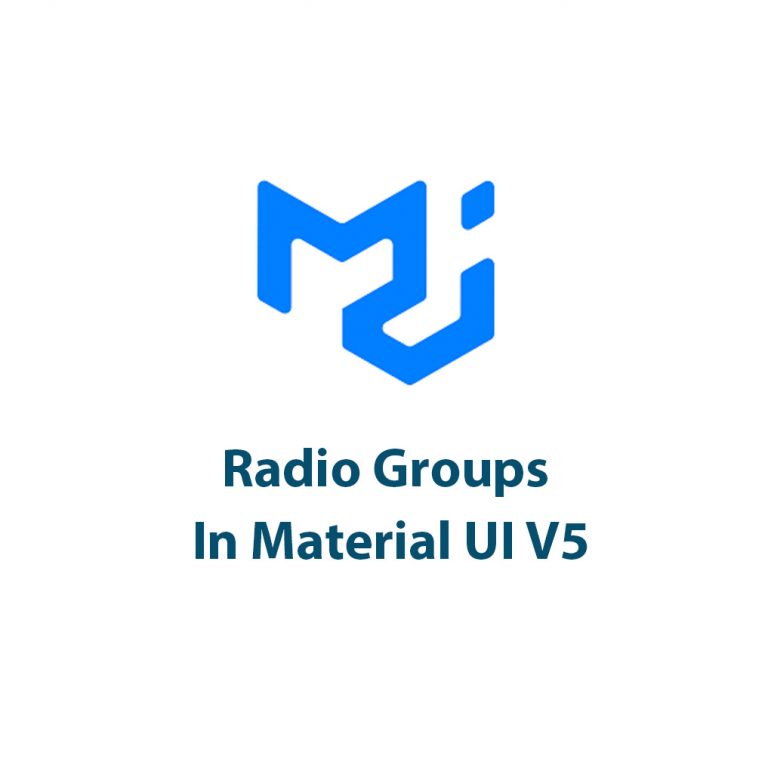
Radio Groups In Material UI V5
Introduction Hello everyone! Material UI has long been one of my favorite UI libraries, and with the recent release of version 5, I thought it would be a great time to explore one of its fundamental components: Radio Groups. In this article, we’ll dive into what Radio Groups are, how they can be used in […]
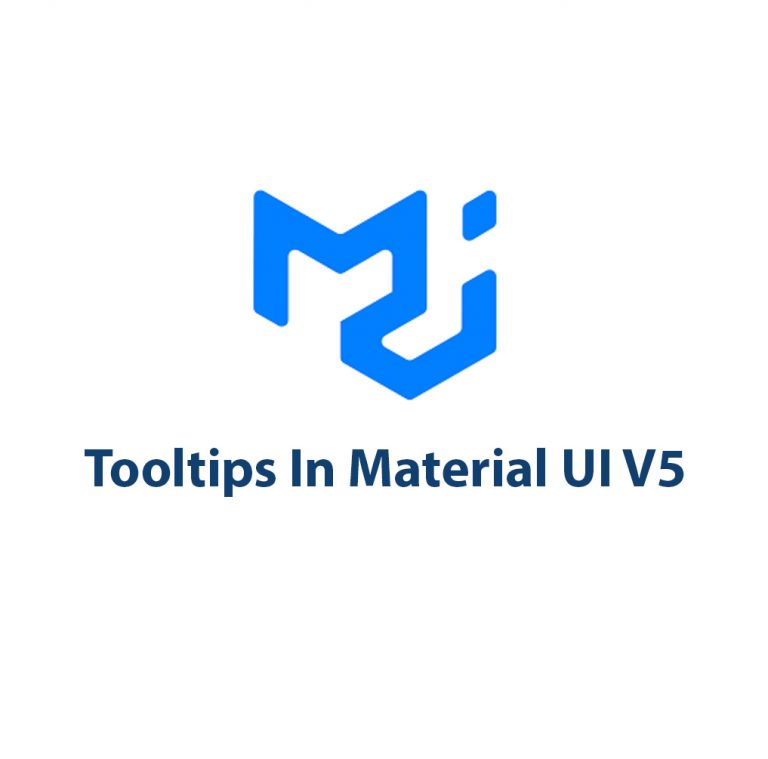
Tooltips in Material UI V5: A Guide To Dynamic UI Elements
Introduction As a web developer, I know that one of the most important things to consider when building a website or web application is how the user interface (UI) will interact with the user. A good UI design should be both intuitive and dynamic – allowing the user to perform actions with ease. And one […]
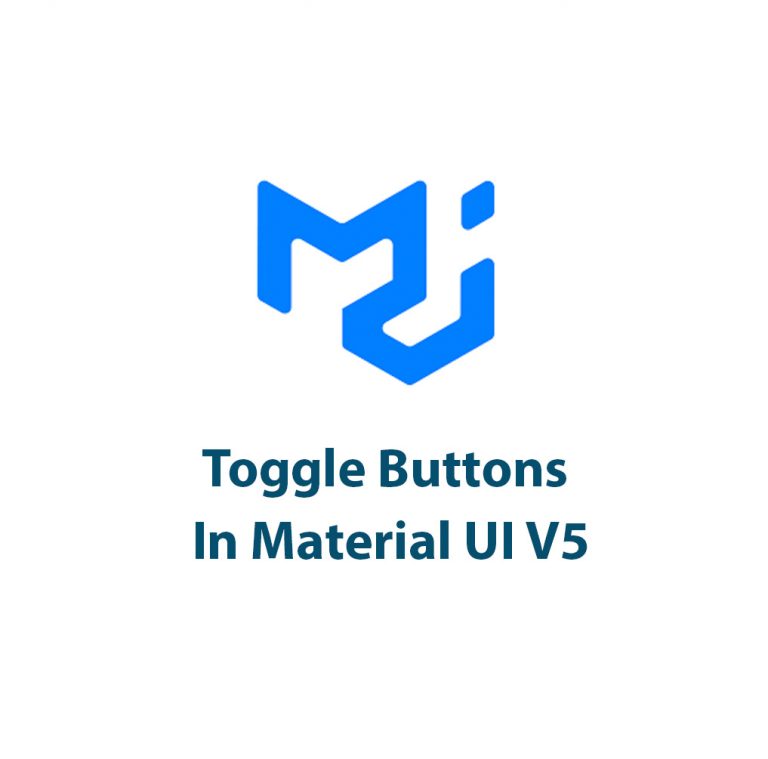
Toggle Buttons In Material UI V5
As a developer, I’ll be the first to admit that sometimes I find myself perplexed by certain UI design choices. As someone who spends a lot of time within the realm of code, I focus more on functionality and less on design. But the more I learn about UI design, the more I realize the […]
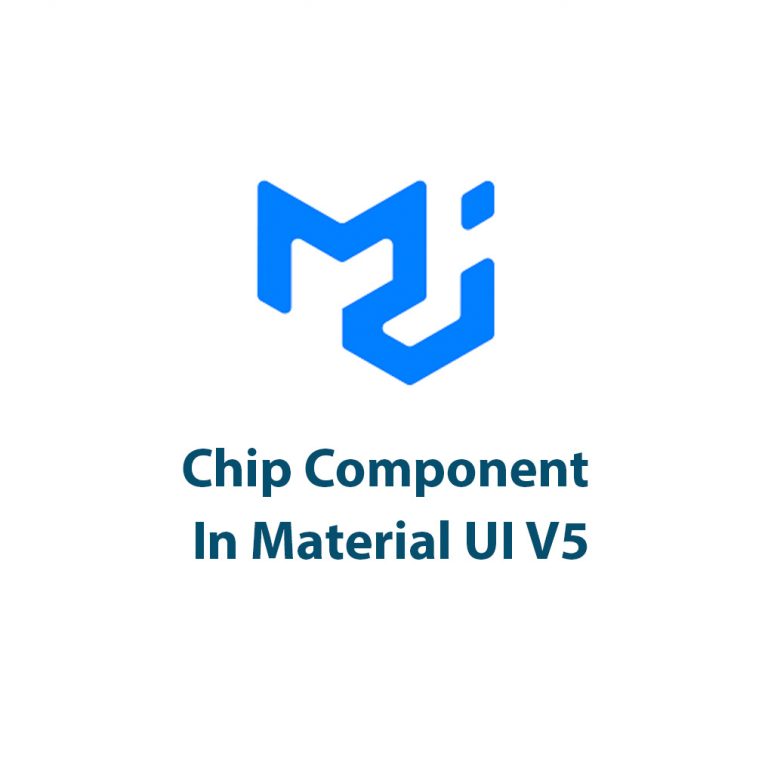
Chip Component In Material UI V5
As a web developer, I’m always on the lookout for new and improved tools and frameworks to make my life easier. And one of the tools that has been a staple in my toolkit is Material UI. If you’re not familiar with Material UI, it is a library that provides pre-built UI components for web […]
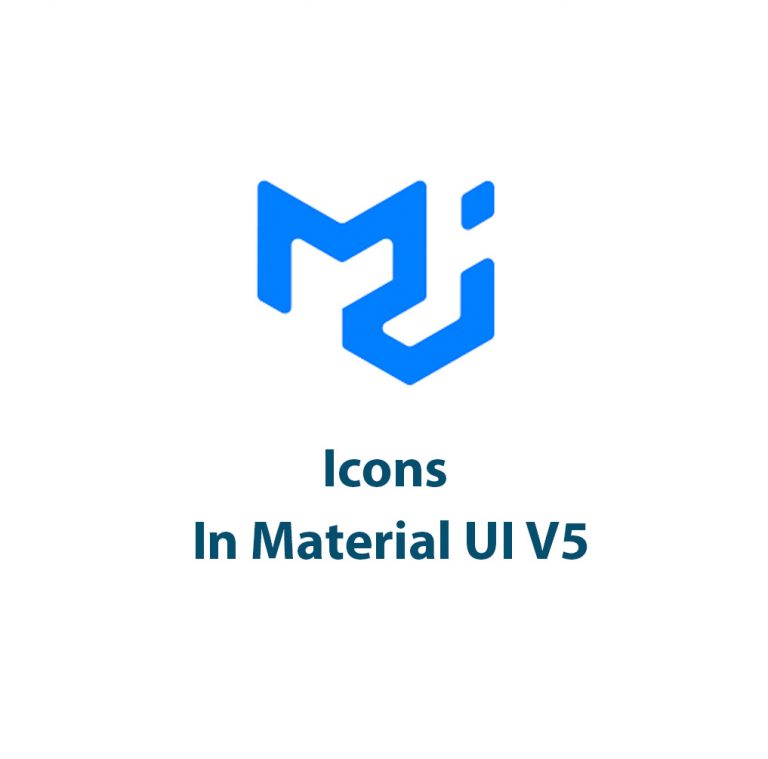
Icons In Material UI V5
Introduction: Material UI is a popular open-source framework for building web applications. Built on top of React, it provides a set of pre-built and customizable components, including typography, forms, and icons. In Material UI, icons play a crucial role in designing visually appealing interfaces and enabling smooth user experience. With the recent release of Material […]
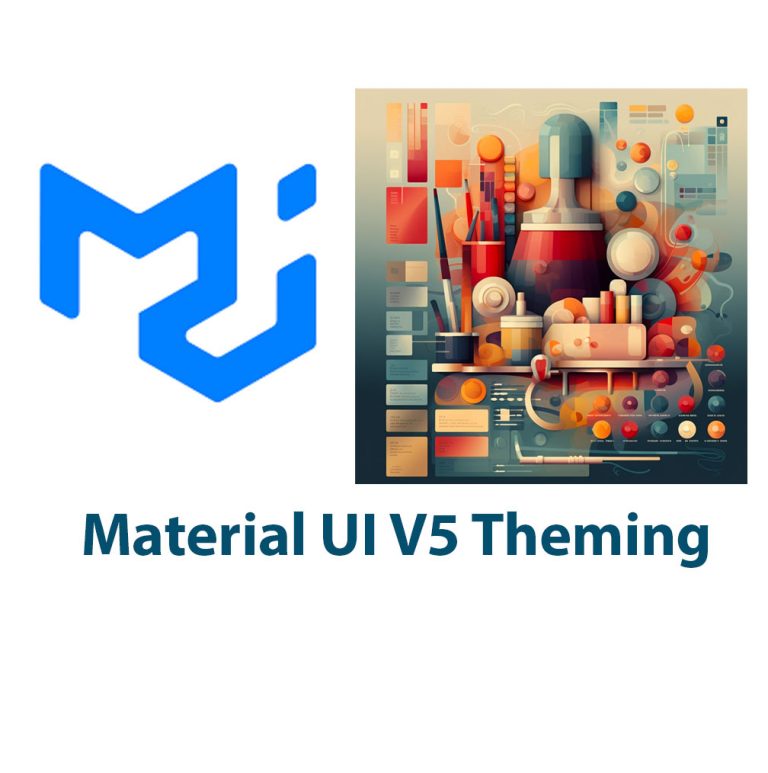
Material UI V5 Theming
Introduction: Popular React UI framework Material UI provides a large selection of pre-built components to easily build responsive user interfaces. The library’s design approach, which is based on Google’s Material Design principles, has helped it become more well-liked among developers. The design framework of Material UI is crucial to the creation of user interfaces since […]