Introduction
As a web developer, I know that one of the most important things to consider when building a website or web application is how the user interface (UI) will interact with the user. A good UI design should be both intuitive and dynamic – allowing the user to perform actions with ease. And one of the best UI elements to enhance interactivity are tooltips.
In this article, I will be discussing how to use tooltips in Material UI V5. Material UI is a popular open-source UI library for React. It provides reusable UI components that can help speed up your development process. With the latest update to Material UI V5, there have been some changes to the way tooltips are implemented. So, let’s dive in!
What Are Tooltips And Why Are They Important?
Tooltips are small, often hidden, elements that appear when a user hovers over a specific UI element. They typically contain a brief description or information related to the element. Tooltips can be used to provide helpful hints, explain functionality, or give context to an element that may not be immediately clear.
Tooltips are an important part of a good UI design because they enhance the user experience by providing useful information and improving navigability. They also save on space by only displaying information when needed, rather than cluttering up the UI with text.
Overview Of Material UI V5
Before diving into tooltips specifically, let’s take a quick look at Material UI V5. Material UI is a React component library that provides pre-built UI components that can be used to quickly create UI elements. With the latest update to Material UI V5, there have been some changes to the structure of the library and the way UI components are implemented.
The most significant change in Material UI V5 is that the team has split the library into two parts: Core and Lab. The Core part is deemed stable and is recommended for production use. Lab, on the other hand, is considered experimental and may change in the future.
Another change in Material UI V5 is the introduction of a new styling solution. The Styled Components API has been removed in favor of the Material UI Styles API. This new API provides a more structured and intuitive approach to styling components, with better support for server-side rendering and global styles.
ToolTips in Material UI V5
Now that we have a better understanding of Material UI V5 and its recent changes, let’s get into tooltips. Material UI V5 provides a simple and intuitive way to include tooltips in your UI design.
To include a tooltip in your Material UI V5 project, you first need to import the Tooltip component from the ‘@mui/material’ package.
import { Tooltip } from '@mui/material';
After importing the Tooltip component, you can add it to any UI element that you wish to provide a tooltip for. Let’s say we have a button that we want to provide a tooltip for. We would wrap the button element in the Tooltip component, and include a ‘title’ prop that contains the text we want to appear in the tooltip.
<Tooltip title="Click me!" arrow>
<Button variant="contained" color="primary">
My Button
</Button>
</Tooltip>
In this example, we’re passing in a string “Click me!” as the title prop for our Tooltip component. This string will be displayed in a tooltip box when the user hovers over the button. We’ve also set the ‘arrow’ prop to ‘true’, which adds an arrow to the tooltip box that points to the UI element that the tooltip is describing.
Props Used in Material UI V5 for Tooltips
Material UI V5 provides several different props that can be used to customize the appearance and behavior of tooltips. Let’s go over some of the most important ones.
Title:
This prop is required and allows you to set the text content of the tooltip.
Arrow:
This prop determines whether or not to show an arrow pointing towards the UI element. If true, an arrow will be displayed.
Placement:
This prop sets the positioning of the tooltip container. By default, it is positioned below and centered to the target element. This can be overridden by setting this prop to one of the following options: top-end, top-start, top, right-end, right-start, right, bottom-end, bottom-start, bottom, left-end, left-start, left.
EnterDelay:
This prop sets the number of milliseconds to wait before showing the tooltip. The default value is 0.
LeaveDelay:
This prop sets the number of milliseconds to wait before hiding the tooltip. The default value is 0.
Interactive:
This prop is a boolean that determines whether or not the tooltip can be interacted with. If true, the tooltip will not disappear on mouse leave and can be interacted with.
DisableFocusListener:
This prop is a boolean that determines whether or not to disable the focus listener on the target element. If true, the tooltip will not appear when the target element is focused.
DisableHoverListener:
This prop is a boolean that determines whether or not to disable the hover listener on the target element. If true, the tooltip will not appear when the target element is hovered over.
Example Code for Using Tooltips in Material UI V5
Let’s look at an example to see how we can use a few of these props to customize the appearance and behavior of our tooltip.
import { Tooltip } from '@mui/material';
<Tooltip title="I'm an interactive tooltip!" enterDelay={500} leaveDelay={200} interactive>
<Button variant="contained" color="primary">
My Interactive Button >:D
</Button>
</Tooltip>
In this example, we’ve set the title to “I’m an interactive tooltip!”. We’ve also set the enterDelay to 500 milliseconds and the leaveDelay to 200 milliseconds, so the tooltip takes half a second to appear and disappears after 200 milliseconds. Finally, we set the ‘interactive’ prop to ‘true’ so that the tooltip won’t disappear when the user moves their mouse away, and can be interacted with.
Styles for Tooltips in Material UI V5
While tooltips in Material UI V5 are generally customizable via props, there may be times when you want to customize the look of your tooltips further. For example, you may want to change the
background color, text color, or arrow size. Luckily, Material UI V5 provides an easy way to override the default styles of the Tooltip component.
To override the default styles, we can create a new makeStyles() hook and pass in the styles we want to override. Here’s an example:
import { Tooltip, makeStyles } from '@material-ui/core';
const useStyles = makeStyles({
tooltip: {
backgroundColor: '#00bfff',
fontSize: '16px',
fontWeight: '600',
},
arrow: {
color: '#00bfff',
fontSize: '20px',
},
});
function App() {
const classes = useStyles();
return (
<Tooltip title="My Custom Tooltip" enterDelay={500} leaveDelay={200} classes={{ tooltip: classes.tooltip, arrow: classes.arrow }}>
<Button variant="contained" color="primary">
My Custom Button
</Button>
</Tooltip>
);
}
In this example, we’ve created a new makeStyles() hook and passed in an object with two properties: tooltip and arrow. The tooltip property is responsible for modifying the tooltip box itself. We’ve changed its background color to ‘#00bfff’, and adjusted the font size and weight to make the text more visible.
The arrow property modifies the arrow pointing to the UI element. We’ve changed its color to match the background color of the tooltip, and increased its size to make it stand out.
We then pass these classes into our Tooltip component via the ‘classes’ prop. This will override the default styles and apply our custom styles instead.
Examples of Tooltips in Material UI V5
Now that we have a good understanding of how to use and style tooltips in Material UI V5, let’s look at a few examples to see them in action.
Basic Tooltip Example:
In this example, we have a simple button that displays a tooltip when hovered over. The tooltip contains a short message, letting the user know what clicking the button will do.
import { Tooltip } from '@mui/material';
function App() {
return (
<Tooltip title="Click me!" arrow>
<Button variant="contained" color="primary">
My Button
</Button>
</Tooltip>
);
}
Tooltip with Arrow Example:
In this example, we’ve added an arrow to the tooltip to help the user identify which element the tooltip is describing. We’ve also made the font size larger to make the text easier to read.
import { Tooltip } from '@mui/material';
function App() {
return (
<Tooltip title="I'm a tooltip with an arrow!" arrow>
<Button variant="contained" color="primary">
My Button
</Button>
</Tooltip>
);
}
Tooltip with Custom Styles Example:
In this example, we’ve customized the background color and font styles of the tooltip using the makeStyles() hook.
import { Tooltip, makeStyles } from '@mui/material';
const useStyles = makeStyles({
tooltip: {
backgroundColor: '#00bfff',
fontSize: '16px',
fontWeight: '600',
},
arrow: {
color: '#00bfff',
fontSize: '20px',
},
});
function App() {
const classes = useStyles();
return (
<Tooltip title="My Custom Tooltip" enterDelay={500} leaveDelay={200} classes={{ tooltip: classes.tooltip, arrow: classes.arrow }}>
<Button variant="contained" color="primary">
My Custom Button
</Button>
</Tooltip>
);
}
Interactive Tooltip Example:
In this example, we’ve made the tooltip interactive by setting the ‘interactive’ prop to true. This allows the user to interact with the tooltip itself, rather than having it disappear when the mouse leaves the target element.
import { Tooltip } from '@mui/material';
function App() {
return (
<Tooltip title="I'm an interactive tooltip!" enterDelay={500} leaveDelay={200} interactive>
<Button variant="contained" color="primary">
My Interactive Button >:D
</Button>
</Tooltip>
);
}
Conclusion
Tooltips are an important part of a good UI design. They provide useful information and context without cluttering up the UI with text. Material UI V5 provides an easy and intuitive way to include tooltips in your UI design, with several customizable props and the ability to override default styles.
Whether you’re new to web development or a seasoned pro, I highly recommend checking out Material UI V5 and considering tooltips for your UI design. With the help of these dynamic UI elements, you can create a more intuitive and interactive user experience.
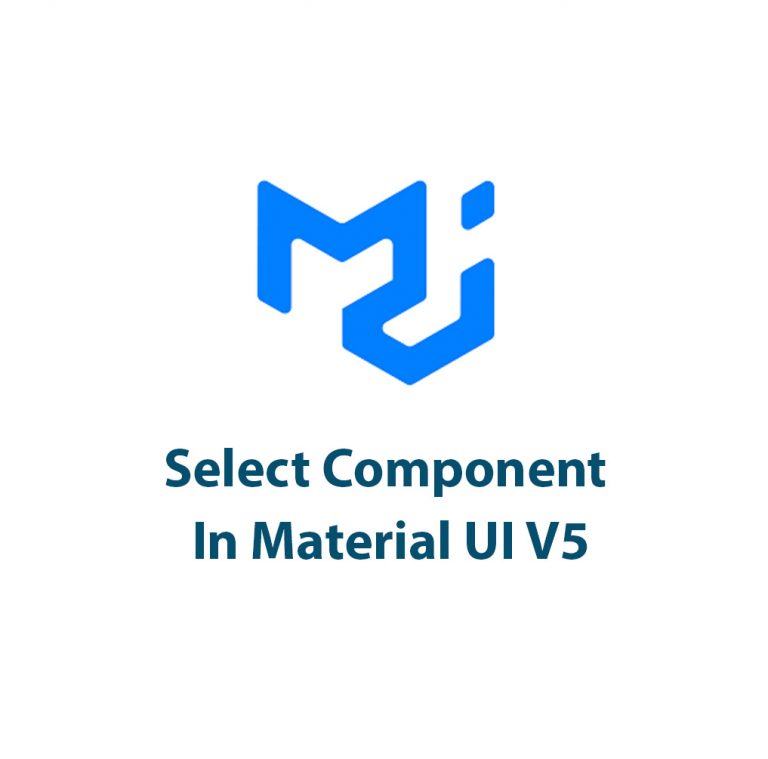
Select Component In Material UI V5
Hey there, fellow developers! In this article, we’re going to dive into the world of Material UI and take a closer look at one of its most essential components – the Select Component. I’m excited to share with you how to implement and customize this component in Material UI V5. So, let’s get started! Introduction […]
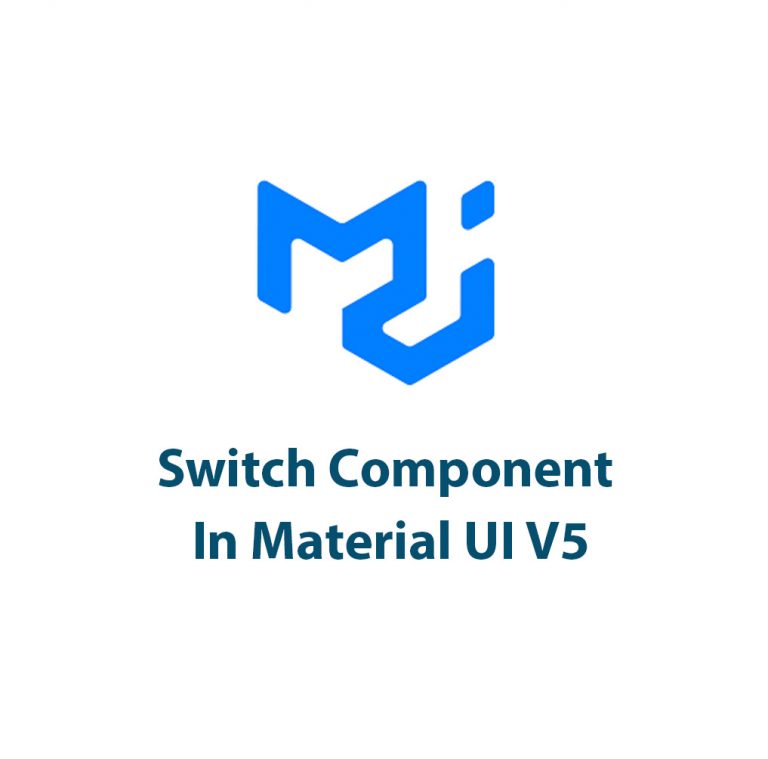
Switch Component In Material UI V5
Hey there, fellow developers! Are you excited about exploring the latest version of Material UI and all the new features it brings? If so, you’ve landed in the right place because today we’re going to dive deep into the Switch Component in Material UI v5. Introduction Before we get into the nitty-gritty of the Switch […]
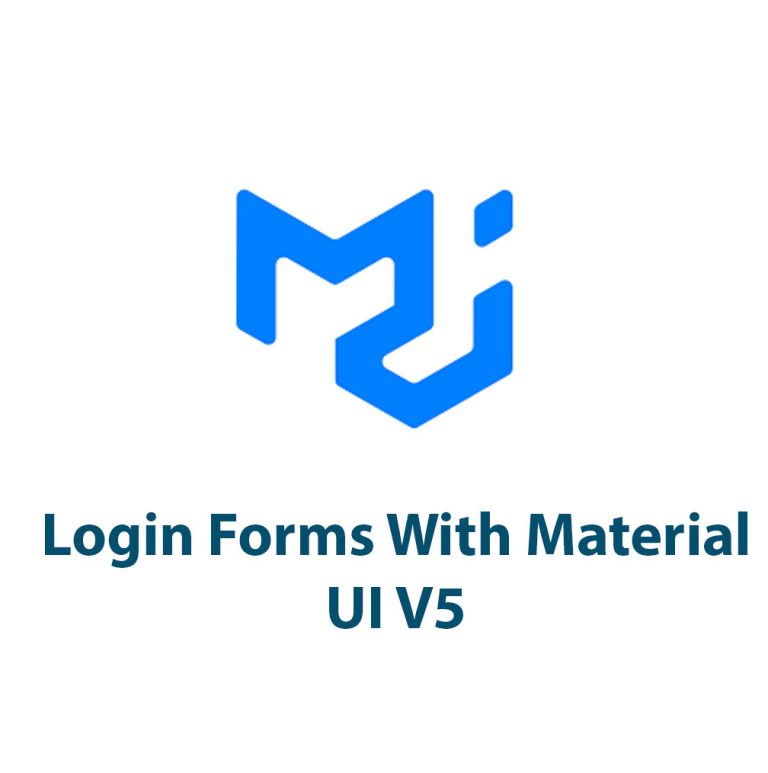
Create Login Forms With Material UI v5
Introduction As a web developer, I’m always on the lookout for efficient ways to create stunning user interfaces. That’s why I’m excited to explore Material UI V5, a library of React components that make it easy to build beautiful web apps. And in this article, I’m going to focus on one essential element of any […]
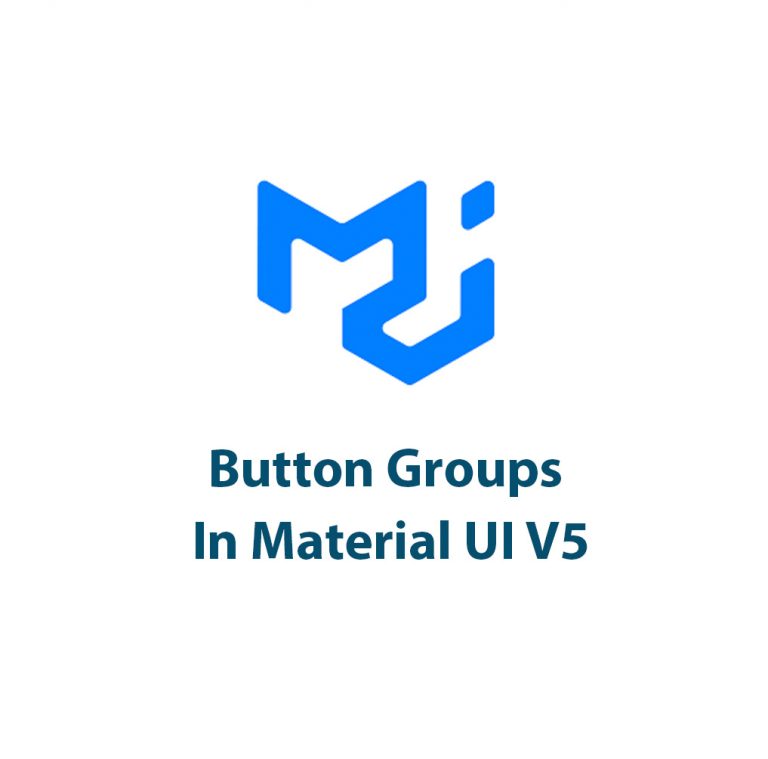
Button Groups In Material UI V5
Hey there! Welcome to my article all about button groups in Material UI V5. In this article, I’ll be giving you an in-depth insight into button groups, how they are used in UI designing, and how you can create them in Material UI V5. Introduction Before we dive into button groups, let’s start with some […]
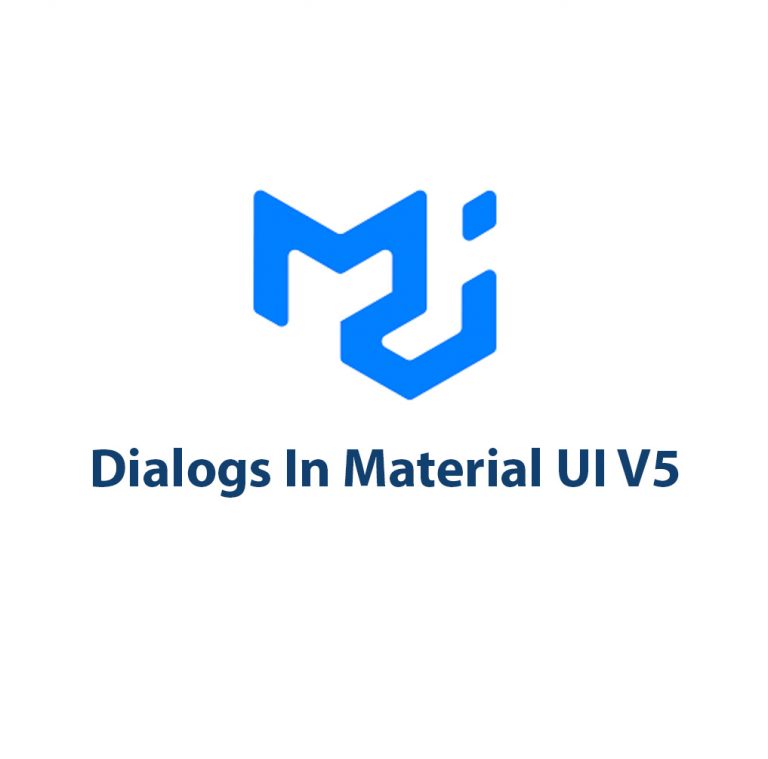
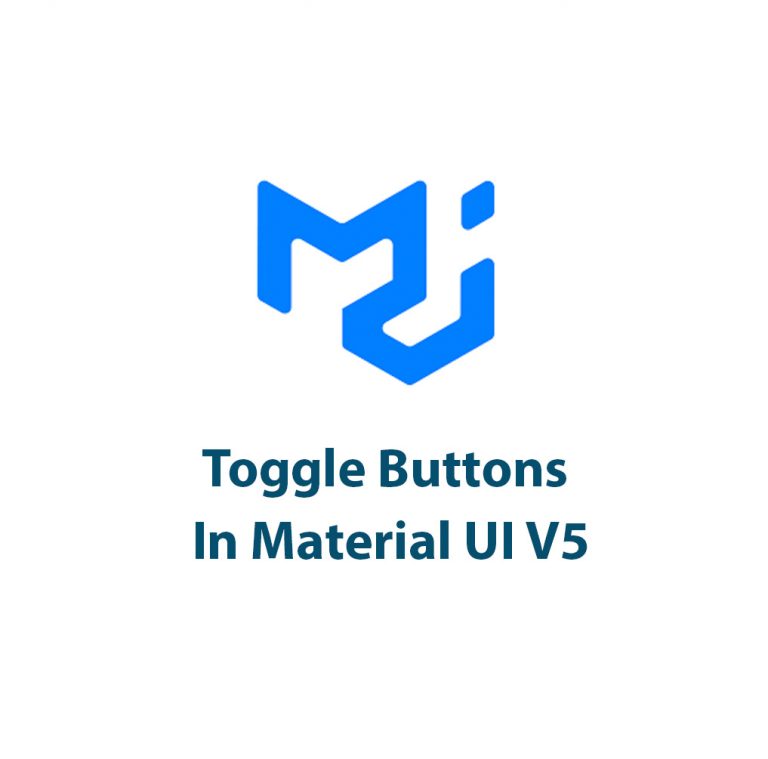
Toggle Buttons In Material UI V5
As a developer, I’ll be the first to admit that sometimes I find myself perplexed by certain UI design choices. As someone who spends a lot of time within the realm of code, I focus more on functionality and less on design. But the more I learn about UI design, the more I realize the […]