Hey there, fellow developers! Are you excited about exploring the latest version of Material UI and all the new features it brings? If so, you’ve landed in the right place because today we’re going to dive deep into the Switch Component in Material UI v5.
Introduction
Before we get into the nitty-gritty of the Switch Component, let’s first introduce what Material UI is. In a nutshell, Material UI is a React-based UI library that provides ready-made components to help developers quickly build beautiful and responsive web applications. One of the essential components in Material UI is the Switch Component.
In UI design, the Switch Component is a critical element that enables users to toggle between two states. For instance, it’s commonly used to turn on and off settings, activate or deactivate notifications, or select between two options. With Material UI v5, the Switch Component has undergone some significant changes, so let’s explore what’s new.
What’s new in Material UI v5?
Material UI v5 brings several improvements, including enhanced performance, reduced bundle sizes, improved customization options, and better accessibility. One significant change in v5 is the component naming convention; all components have been renamed using the styled
property of Material UI. This change may impact your current project’s migration to Material UI v5, so be sure to check your codebase.
The Switch Component has also received several updates to its API. Previously, the component had several issues with accessibility, which have all been resolved in v5. There are also improvements to the component’s animation and styling.
Understanding Switch Component in Material UI v5
Now let’s explore the Switch Component in more detail. The Switch Component is available in both controlled and uncontrolled forms. Here is an example of the basic syntax for the Switch Component:
import Switch from '@mui/material/Switch';
<Switch checked={checked} onChange={handleChange} />;
In this example, we import the Switch component and provide two props: checked
and onChange
. The checked
prop defines whether the switch is on or off, and the onChange
prop provides a function to be called when the switch toggles states.
Types of Switch Component
The Switch component is available in many variations, including the following:
Basic Switch Component
The Basic Switch Component is the most common type of switch and has a classic toggle button style. Here is the code for the Basic Switch Component:
import Switch from '@mui/material/Switch';
<Switch />;
Disabled Switch Component
The Disabled Switch Component is a switch that is non-interactive, meaning the user can’t switch between on and off states. Here is the code for the Disabled Switch Component:
import Switch from '@mui/material/Switch';
<Switch disabled />;
Switch with Label
The Switch Component with a label displays a text title next to the switch. The label conveys the purpose of the switch. Here is the code for the Switch Component with a Label:
import Switch from '@mui/material/Switch';
import FormControlLabel from '@mui/material/FormControlLabel';
import FormGroup from '@mui/material/FormGroup';
import Typography from '@mui/material/Typography';
const [state, setState] = useState({
checkedA: true,
checkedB: true,
});
const handleChange = (event) => {
setState({ ...state, [event.target.name]: event.target.checked });
};
<FormGroup>
<Typography variant="body1">Do you allow in-app purchases?</Typography>
<FormControlLabel control={<Switch checked={state.checkedA} onChange={handleChange} name="checkedA" />} label="Allow In-app Purchases" />
</FormGroup>
In this example, we import FormControlLabel
and FormGroup
from Material UI along with Typography
. We define a useState
hook that initializes two parameters, then we pass them to the FormGroup
.
Switch Component in Controlled Form
The Controlled Form Switch Component is a switch that performs logic based on the value of the checked
prop. Here is the code for the Switch Component in controlled form:
import Switch from '@mui/material/Switch';
const [state, setState] = useState({
checkedA: true,
checkedB: true,
});
const handleChange = (event) => {
setState({ ...state, [event.target.name]: event.target.checked });
};
<Switch checked={state.checkedB} onChange={handleChange} name="checkedB" />;
Usage of Switch Component with Examples
Let’s look at some use cases and examples to see the Switch Component in action.
Use Case 1 – Register for Newsletters
Suppose you have a newsletter on your website that visitors can subscribe to receive notifications about your services or products. The Switch Component in this case allows users to decide whether to receive newsletters or not. Here is the code for this example:
import Switch from '@mui/material/Switch';
import FormControlLabel from '@mui/material/FormControlLabel';
const [state, setState] = useState({
checked: false,
});
const handleChange = (event) => {
setState({ ...state, [event.target.name]: event.target.checked });
};
<FormControlLabel control={<Switch checked={state.checked} onChange={handleChange} name="checked" />} label="Register for newsletters" />
In this example, we define a useState
hook that initializes the checked
parameter to false
. We pass this parameter to the Switch Component
, which then toggles the checkbox based on the state of the parameter.
Use Case 2 – Activate Dark Mode
Suppose you have a feature on your website that allows visitors to switch between light mode and dark mode. The Switch Component can be used to turn on and off this feature. Here is the code for this example:
import Switch from '@mui/material/Switch';
import FormControlLabel from '@mui/material/FormControlLabel';
const [state, setState] = useState({
checked: false,
});
const handleChange = (event) => {
setState({ ...state, [event.target.name]: event.target.checked });
};
<FormControlLabel control={<Switch checked={state.checked} onChange={handleChange} name="checked" />} label="Activate Dark Mode" />
Again, we define a useState
hook that initializes the checked
parameter to false
. We pass this parameter to the Switch Component
, which then toggles the checkbox based on the state of the parameter.
Styling Switch Component in Material UI v5
One of the significant benefits of Material UI is customization. You can customize the Switch Component’s color and shape using themes and style overrides. You can use theme
to configure the Switch Component’s color and to override its CSS properties.
Here is an example of how to override the Switch Component’s color using the ThemeProvider
component:
import { createTheme, ThemeProvider } from '@mui/material/styles';
import Switch from '@mui/material/Switch';
const theme = createTheme({
components: {
MuiSwitch: {
styleOverrides: {
root: {
color: "#2196f3",
'& .MuiSwitch-thumb': {
backgroundColor: "#fff",
},
'& .MuiSwitch-track': {
backgroundColor: "#2196f3",
},
'& .MuiSwitch-switchBase.Mui-checked + .MuiSwitch-track': {
opacity: 0.5,
},
},
},
},
},
});
<ThemeProvider theme={theme}>
<Switch />
</ThemeProvider>
In the example above, we created a custom theme with customized style overrides for the Switch Component. We used color
to change the component’s default color and added backgroundColor
to Thumb
and Track
to customize the component’s appearance. You can also use the opacity
property to adjust the opacity of the Switch Component.
Theming Switch Component
Similarly, Material UI provides ThemeProvider
component which enables us to override the default theme of an application with custom color schemes. Here is an example of how to customize the Switch Component using ThemeProvider
:
import { createTheme, ThemeProvider } from '@mui/material/styles';
import Switch from '@mui/material/Switch';
const theme = createTheme({
palette: {
common: {
black: "#000",
white: "#fff",
},
primary: {
main: "#2196f3",
light: "#64b5f6",
dark: "#1976d2",
contrastText: "#fff",
},
},
});
<ThemeProvider theme={theme}>
<Switch />
</ThemeProvider>
In this example, we defined a palette with a custom color scheme using createTheme
and passed it to <ThemeProvider>
. Within the palette
object, we have primary
and common
properties that define the color scheme for the Switch Component.
Customization of colors, shapes and styles
If you want to customize additional properties of the Switch Component, Material UI provides several classes for its different parts. The main classes for the Switch Component are:
MuiSwitch-root
MuiSwitch-thumb
MuiSwitch-switchBase
MuiSwitch-track
MuiSwitch-colorPrimary
MuiSwitch-colorSecondary
MuiSwitch-sizeSmall
MuiSwitch-sizeMedium
MuiSwitch-sizeLarge
Suppose you want to customize the Switch Component’s shape by changing the size. In that case, you can use the size
property or size
classes to adjust the size of the component. Here is an example of how to customize the Switch Component’s size:
import Switch from '@mui/material/Switch';
<Switch size="small" />
<Switch size="medium" />
<Switch size="large" />
This example shows how to customize the size of the Switch Component using the size
property.
Best Practices for implementing Switch Component in Material UI v5
As with most UI components, there are some best practices to follow when implementing the Switch Component in Material UI v5. Let’s take a look at some of these best practices:
Accessibility considerations
Accessibility is critical when designing interfaces. Ensure that the Switch Component has accessible labels that provide users with information about its function. All components in the Material UI v5 library are W3C-compliant, which means they’re designed with accessibility in mind.
Use of correct input elements with Switch Component
The Switch Component should be used with the correct input elements. For instance, the Switch Component is used with the FormControlLabel
or FormGroup
components that offer more control over the Checkbox’s label and layout to build a better user interface.
Consistency in UI design and placement of Switch Component
Consistency is key in UI design, and the same applies to the Switch Component. Maintain consistency in the placement of the Switch Component and other UI components across your application. Make sure that the Switch Component is used only for what it’s intended to do.
Conclusion
In conclusion, Material UI v5 provides more significant improvements to the Switch Component, such as enhanced performance, customization, and accessibility. In this article, we covered the basics of the Switch Component, its types, and how to use it with some real-world examples. We also examined how to customize and style the Switch Component and implement best practices to achieve a better UI design.
With that, we hope this article helped you get started with the Switch Component within Material UI v5. Do you have any questions or feedback on the Switch Component? Let us know in the comments!
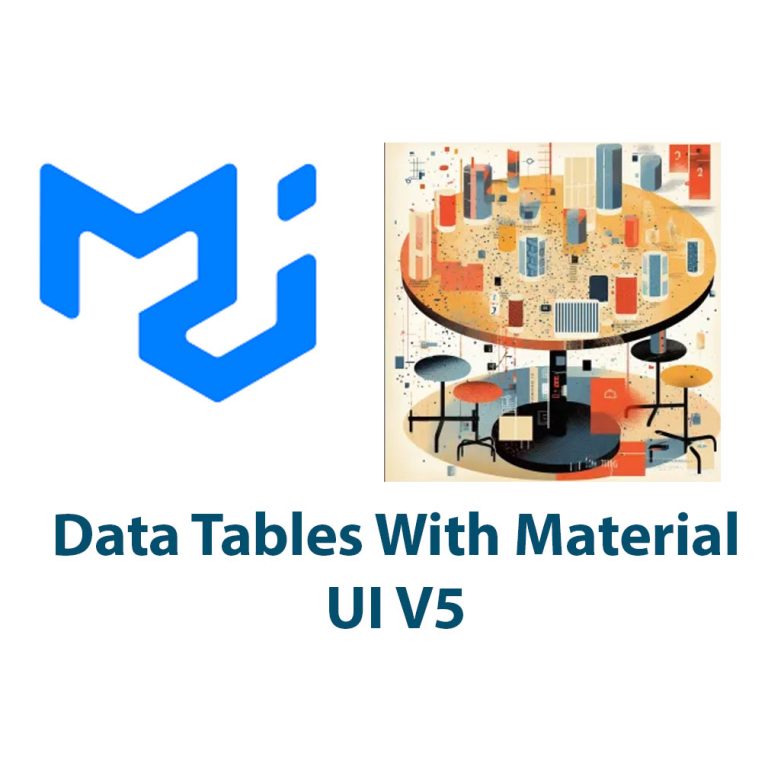
Datatables With Material V5
Introduction Material UI V5 is a popular, open-source library that provides pre-built UI components, themes, and styles for building user interfaces with React. Data tables are a common component used in web development to display data in a tabular format. In this article, I’ll show you how to use Material UI V5 to create stylish […]
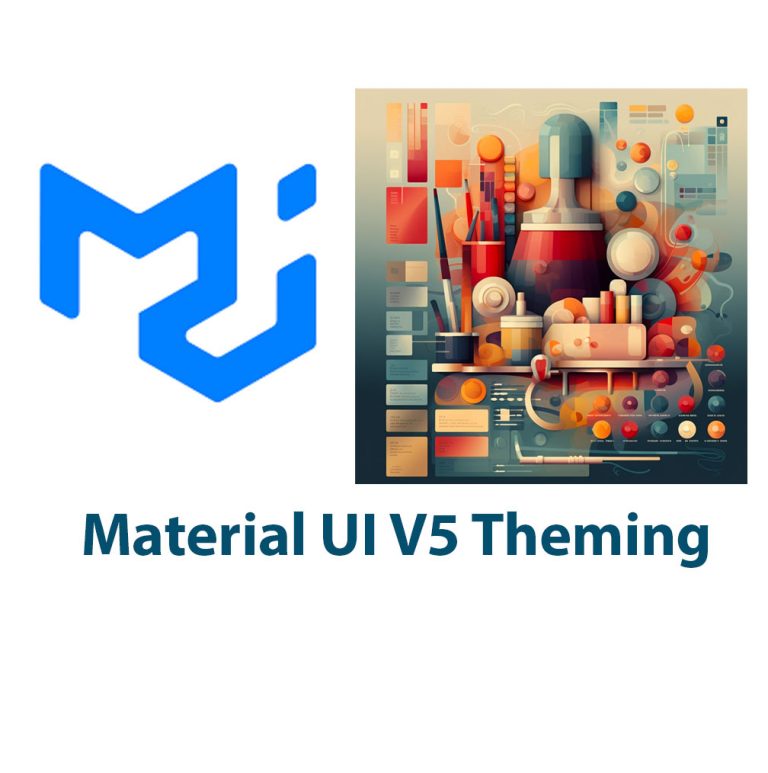
Material UI V5 Theming
Introduction: Popular React UI framework Material UI provides a large selection of pre-built components to easily build responsive user interfaces. The library’s design approach, which is based on Google’s Material Design principles, has helped it become more well-liked among developers. The design framework of Material UI is crucial to the creation of user interfaces since […]
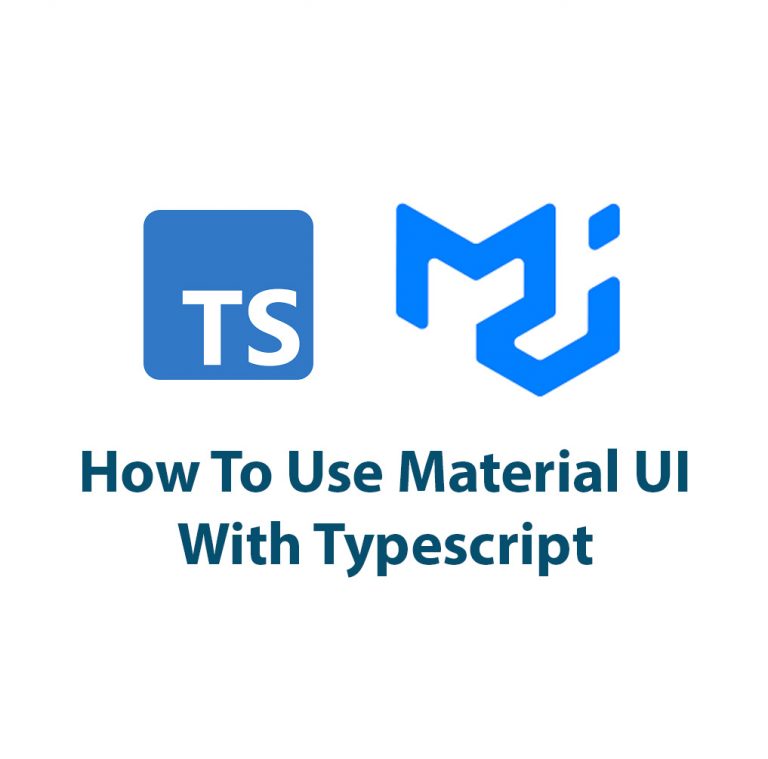
Use Material UI V5 With Typescript
Introduction Hello everyone! In this article, we are going to talk about Material UI V5 with Typescript. If you are a React developer, you must have heard of Material UI. It is one of the most popular React UI component libraries out there. On the other hand, Typescript is a superset of JavaScript that provides […]
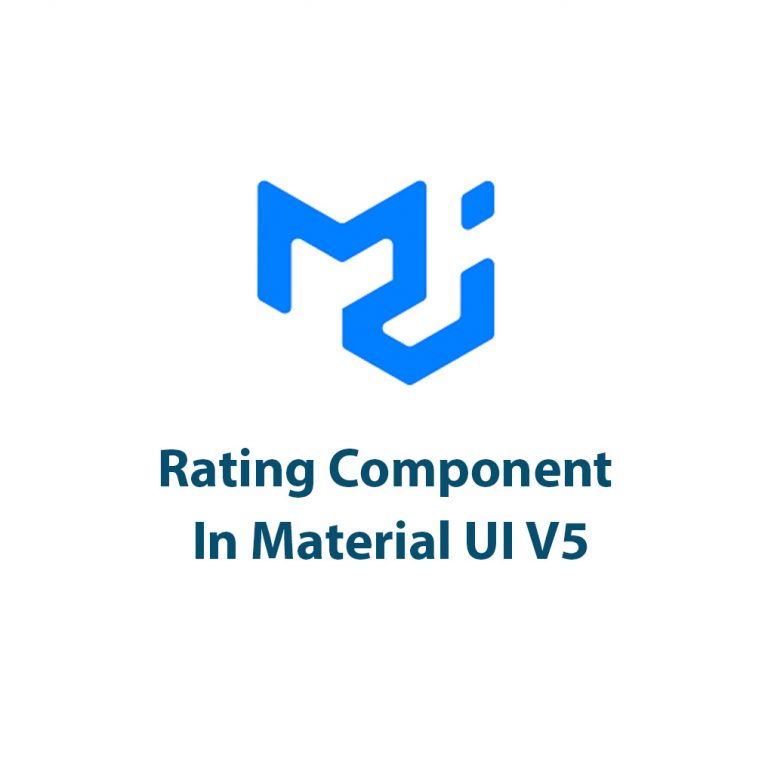
Rating Component In Material UI V5
Hello and welcome to this exciting article about the Rating Component in Material UI V5! As a web developer, I have come to really appreciate the simplicity and flexibility that this UI library provides, especially when it comes to components that add interactivity to user interfaces. In this article, I’m going to walk you through […]
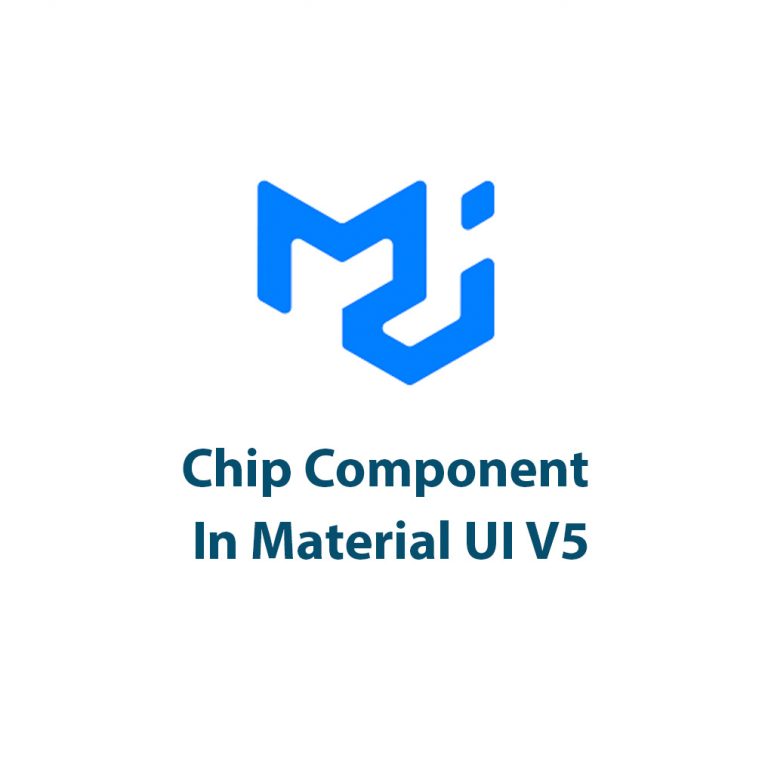
Chip Component In Material UI V5
As a web developer, I’m always on the lookout for new and improved tools and frameworks to make my life easier. And one of the tools that has been a staple in my toolkit is Material UI. If you’re not familiar with Material UI, it is a library that provides pre-built UI components for web […]
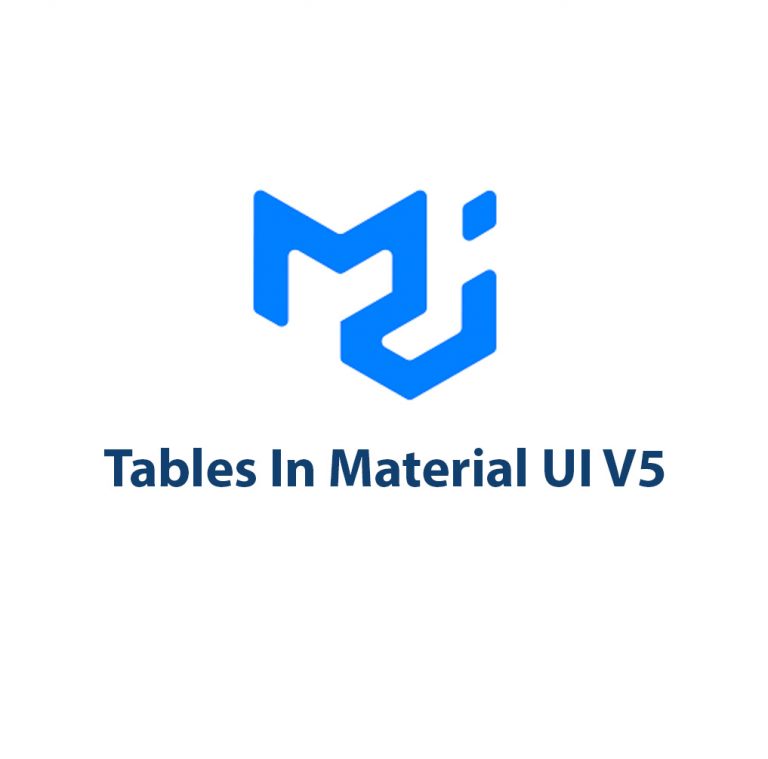
Tables In Material UI V5
Introduction As a web developer, I often find myself struggling to create functional and visually appealing tables for my web applications. When I discovered Material UI V5, I was intrigued by the promise of a streamlined and customizable table component. In this article, I’ll delve into the world of Material UI V5 Tables and share […]