Introduction
Hello everyone! In this article, we are going to talk about Material UI V5 with Typescript. If you are a React developer, you must have heard of Material UI. It is one of the most popular React UI component libraries out there. On the other hand, Typescript is a superset of JavaScript that provides static typing and improves the developer experience.
Material UI V5 is the latest version of Material UI, where the whole library is rewritten from scratch to support new features and improvements. It is faster, more customizable, and provides a more natural way of writing CSS styles.
So, what happens if we integrate Typescript with Material UI V5? In this article, we are going to explore the benefits, how to integrate Typescript, and how to use Material UI V5 with Typescript.
What’s new in Material UI V5?
Before diving into using Material UI with Typescript, let’s talk about some of the new features of Material UI V5. Material UI V5 is a major release, and it comes with many new features and improvements. Let’s have a look at some of them.
- Faster and Lightweight
In Material UI V5, the library is rewritten from scratch, which means it has better performance and is lighter than its previous versions. Also, the new version comes with a better packaging system, which means you can import only the components that you need and use.
- Improved Customization
Customization is one of the essential parts of any UI library, and Material UI is known for providing extreme customization capabilities. Material UI V5 takes it one step further by introducing a new CSS engine called “Emotion.” It gives you better control over CSS styles and improves the customization experience.
- New Components
Material UI V5 comes with many new components, such as Autocomplete, Rating, and ToggleButton. These components are highly customizable and provide a great user experience.
- Improved Accessibility
Accessibility is critical for any UI library, and Material UI V5 makes it easier to make your application accessible. It provides an accessibility module that helps you make your components more accessible.
Integrating Typescript with Material UI V5
Now that we have explored the new features of Material UI V5 let’s discuss the benefits of integrating Typescript with Material UI V5.
Benefits of using Typescript in Material UI
Typescript brings many benefits to any JavaScript project, and Material UI is no exception. Here are some benefits of using Typescript in Material UI.
- Static Typing
One of the major benefits of using Typescript with Material UI is static typing. Typescript provides type checking, which helps you catch bugs and improve your code quality. When you use Material UI components in your project, you can pass props and state to these components. In the absence of proper type checking, it is easy to misspell, mistype, or misuse these props and state. Typescript ensures that you use these components correctly and improves the robustness of your code.
- Better IDE Support
Typescript provides better IDE support than JavaScript. Modern IDEs such as VS Code, Webstorm, or Atom have built-in support for Typescript. They provide better code completion, type checking, and debugging capabilities. When you use Material UI with Typescript, you get better IDE support, which improves your productivity and reduces development time.
- Improved Code Readability
Typescript provides better code readability than JavaScript. When you use types and interfaces in Typescript, your code becomes easier to read and understand. Also, when you work with Material UI components, the types and interfaces act as documentation for these components and improve your code’s readability.
Steps to integrate Typescript with Material UI V5
Now that we have discussed the benefits let’s dive into the steps to integrate Typescript with Material UI V5.
Step 1: Create a new React project with Typescript
The first step is to create a new React project with Typescript. You can use create-react-app to create a new project.
npx create-react-app my-app --template typescript
Step 2: Install Material UI
The second step is to install Material UI. You can install Material UI using npm or yarn.
npm install @material-ui/core
or
yarn add @material-ui/core
Step 3: Install Typings
The third step is to install typings for Material UI to get type definitions. You can install typings using npm or yarn.
npm install @types/material-ui
or
yarn add @types/material-ui
Step 4: Import Material UI Components
The last step is to import Material UI components and use them in your project.
import { Button } from '@material-ui/core';
function MyButton() {
return (
<Button variant="contained" color="primary">
Click Me
</Button>
);
}
Best practices for using Typescript in Material UI
Let’s discuss some best practices for using Typescript with Material UI.
- Define Interfaces and Types
When you use Material UI components in your project, it is recommended to define interfaces and types for props and state. It improves the code’s readability and ensures correct usage of these components.
interface MyButtonProps {
text: string;
onClick: () => void;
}
function MyButton(props: MyButtonProps) {
return (
<Button variant="contained" color="primary" onClick={props.onClick}>
{props.text}
</Button>
);
}
- Use Theme Provider
Material UI provides Theme Provider, which allows you to customize the look and feel of Material UI components. When you use Theme Provider with Typescript, it is recommended to define the theme interface to get better type checking.
declare module '@material-ui/core/styles/createMuiTheme' {
interface Theme {
myCustomThemeValue: string;
}
interface ThemeOptions {
myCustomThemeValue?: string;
}
}
const theme = createMuiTheme({
myCustomThemeValue: '#000',
});
function MyApp() {
return (
<ThemeProvider theme={theme}>
<MyButton text="Click Me" onClick={() => alert('Button Clicked!')} />
</ThemeProvider>
);
}
- Use JSS for Styling
Material UI provides JSS (JavaScript Style Sheets) for styling. JSS is a CSS in JS solution that allows you to write CSS styles in JavaScript. When you use JSS with Typescript, it is recommended to define the styles interface to get better type checking.
```
const useStyles =
makeStyles((theme: Theme) => createStyles({
myButton: {
color: theme.palette.primary.contrastText,
backgroundColor: theme.palette.primary.main,
'&:hover': {
backgroundColor: theme.palette.primary.dark,
},
},
}));
interface MyButtonProps {
text: string;
onClick: () => void;
}
function MyButton(props: MyButtonProps) {
const classes = useStyles();
return (
<Button className={classes.myButton} onClick={props.onClick}>
{props.text}
</Button>
);
}
```
Examples of using Material UI V5 with Typescript
Now that we have seen how to integrate Typescript with Material UI V5 and some best practices, let’s dive into some examples of using Material UI V5 with Typescript.
- Using Autocomplete Component
The Autocomplete component is one of the new components in Material UI V5. It provides a great user experience and is highly customizable. Let’s see how we can use the Autocomplete component with Typescript.
interface Option {
value: string;
label: string;
}
const options: Option[] = [
{ value: 'apple', label: 'Apple' },
{ value: 'banana', label: 'Banana' },
{ value: 'cherry', label: 'Cherry' },
{ value: 'orange', label: 'Orange' },
];
interface AutocompleteProps {
label: string;
options: Option[];
}
function MyAutocomplete(props: AutocompleteProps) {
const { label, options } = props;
return (
<Autocomplete
options={options}
getOptionLabel={(option: Option) => option.label}
renderInput={(params) => <TextField {...params} label={label} />}
/>
);
}
- Using Rating Component
The Rating component is another new component in Material UI V5. It provides a star rating feature and is highly customizable. Let’s see how we can use the Rating component with Typescript.
interface RatingProps {
name: string;
}
function MyRating(props: RatingProps) {
const { name } = props;
const [value, setValue] = useState<number | null>(2);
return (
<Rating
name={name}
value={value}
onChange={(event: React.ChangeEvent<{}>, newValue: number | null) => {
setValue(newValue);
}}
/>
);
}
Conclusion
So, that’s all about Material UI V5 with Typescript. We explored some of the new features in Material UI V5, discussed the benefits of integrating Typescript with Material UI, and saw how to use Material UI V5 with Typescript. We also saw some best practices and examples of using Material UI V5 with Typescript.
Material UI V5 with Typescript is a great combination, and it can bring many benefits to your project. With better type checking, improved code readability, and better IDE support, your development experience can improve significantly.
I hope this article was helpful for you. Happy coding!
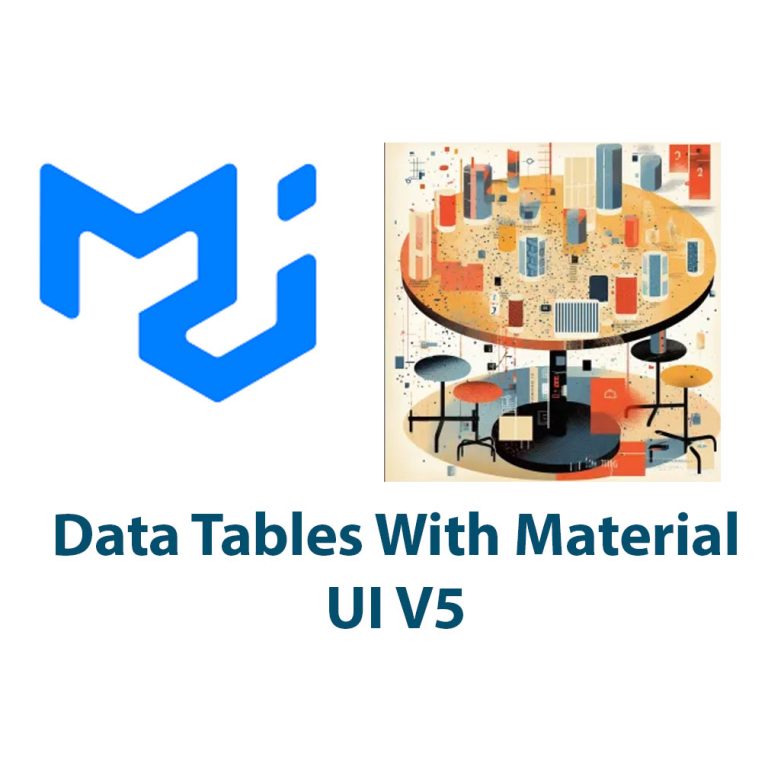
Datatables With Material V5
Introduction Material UI V5 is a popular, open-source library that provides pre-built UI components, themes, and styles for building user interfaces with React. Data tables are a common component used in web development to display data in a tabular format. In this article, I’ll show you how to use Material UI V5 to create stylish […]
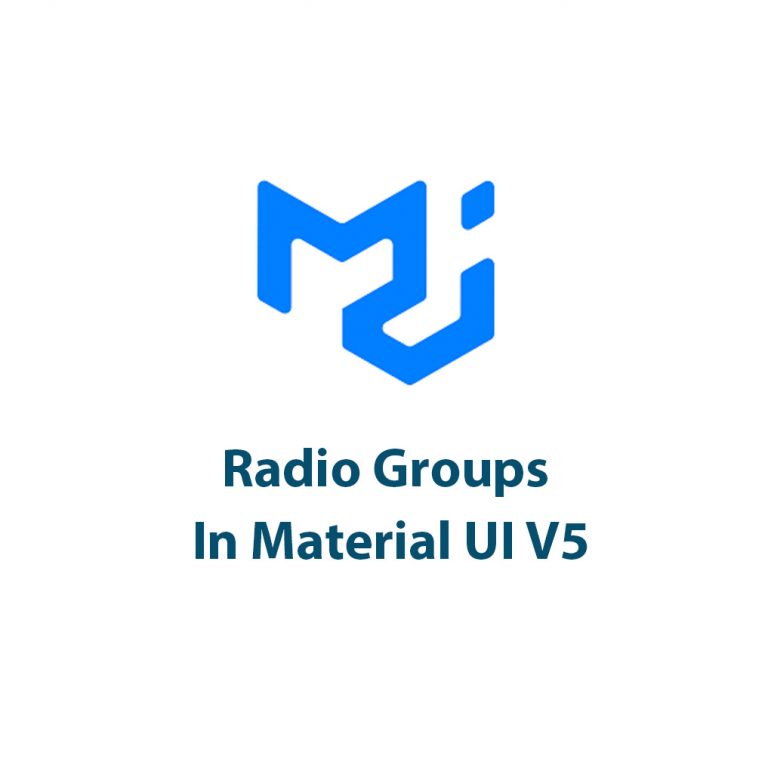
Radio Groups In Material UI V5
Introduction Hello everyone! Material UI has long been one of my favorite UI libraries, and with the recent release of version 5, I thought it would be a great time to explore one of its fundamental components: Radio Groups. In this article, we’ll dive into what Radio Groups are, how they can be used in […]
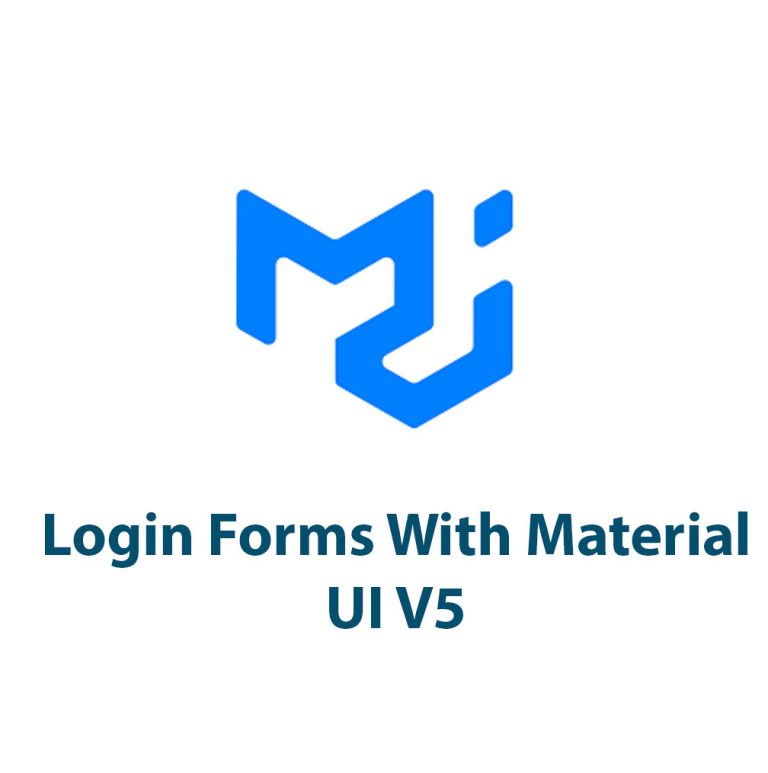
Create Login Forms With Material UI v5
Introduction As a web developer, I’m always on the lookout for efficient ways to create stunning user interfaces. That’s why I’m excited to explore Material UI V5, a library of React components that make it easy to build beautiful web apps. And in this article, I’m going to focus on one essential element of any […]
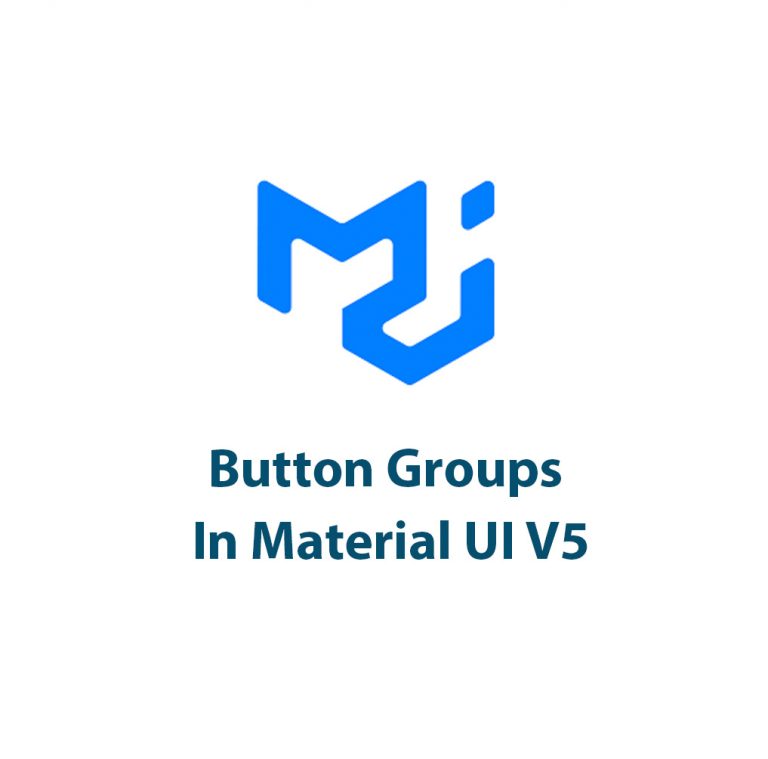
Button Groups In Material UI V5
Hey there! Welcome to my article all about button groups in Material UI V5. In this article, I’ll be giving you an in-depth insight into button groups, how they are used in UI designing, and how you can create them in Material UI V5. Introduction Before we dive into button groups, let’s start with some […]
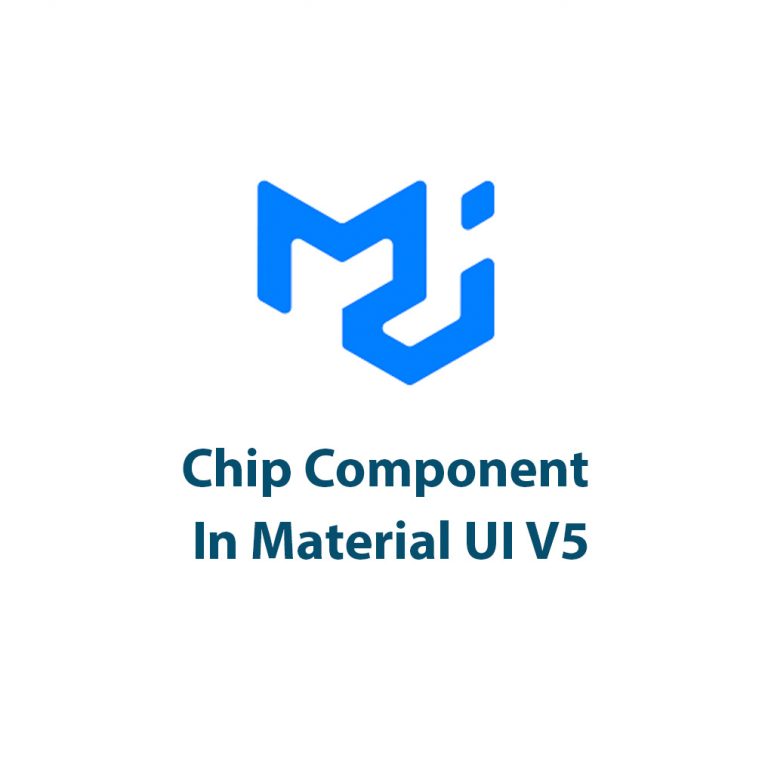
Chip Component In Material UI V5
As a web developer, I’m always on the lookout for new and improved tools and frameworks to make my life easier. And one of the tools that has been a staple in my toolkit is Material UI. If you’re not familiar with Material UI, it is a library that provides pre-built UI components for web […]
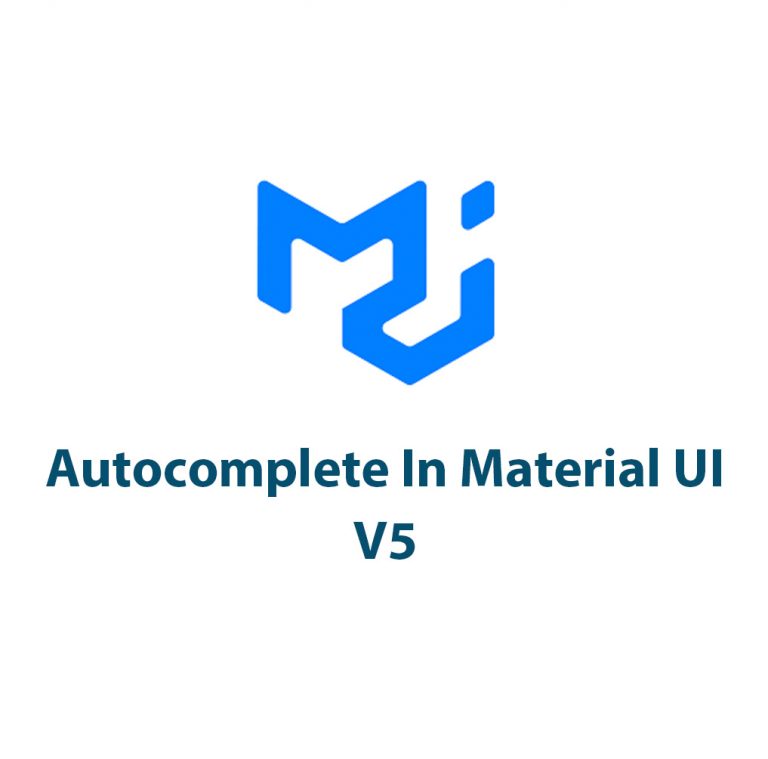
Autocomplete In Material UI V5
Introduction Hello everyone, today I am going to talk about one of the most useful components in Material UI V5 – Autocomplete. Material UI is a popular React UI framework that provides a wide range of pre-designed components to make the development of complex user interfaces simpler. Autocomplete is an intuitive component that provides suggestions […]