As a web developer, I’m always on the lookout for new and improved tools and frameworks to make my life easier. And one of the tools that has been a staple in my toolkit is Material UI.
If you’re not familiar with Material UI, it is a library that provides pre-built UI components for web developers to use in their projects. It’s based on Google’s Material Design guidelines and offers a clean and modern look that’s easy to implement.
One of the components that I’ve found particularly useful in Material UI is the Chip component. This component is used to represent small pieces of information, such as tags or categories, in a clean and visually appealing way.
In previous versions of Material UI, there have been some limitations and drawbacks to the Chip component. But with Material UI V5, there have been some major updates and improvements to this component that have really impressed me.
So in this article, I want to explore the Chip component in Material UI V5 in detail. We’ll look at the changes and updates that have been made, as well as the benefits of using the new version of the Chip component in your web development projects.
Chip Component in Material UI V4
First, let’s take a quick look at the previous version of the Chip component in Material UI, which was in version 4.
The Chip component in V4 was functional, but it had some limitations. For example, there was limited customization available for the component. You could only change the color and size of the Chip. Additionally, it was difficult to visually distinguish between different types of Chips, such as active and inactive Chips.
Here’s an example of what the Chip component looked like in Material UI V4:
import React from 'react';
import Chip from '@material-ui/core/Chip';
function MyChipComponent() {
return (
<div>
<Chip label="Default" />
<Chip label="Inactive" />
<Chip label="Active" />
</div>
);
}
export default MyChipComponent;
As you can see, the Chip component in V4 is straightforward to use, but it lacks some of the visual customization options that developers would prefer.
Introduction to Material UI V5
Now, let’s dive into what’s new with Material UI V5 and how it relates to the Chip component.
Material UI V5 has been a much-anticipated release, and there are several new features and updates in this version that make it a worthwhile upgrade.
Some of the highlights of Material UI V5 include:
- Improved performance: The new version of Material UI has focused heavily on improving performance and reducing the size of the library. This means faster load times and better performance overall.
- More customization: With Material UI V5, there are more CSS customization options available for the components. This allows for greater flexibility and easier customization to match your branding or design needs.
- More accessible: Material UI V5 has made efforts to improve accessibility for users with disabilities. This includes better keyboard navigation and focus management.
But what about the Chip component specifically? Let’s take a closer look at the changes and updates for this component in V5.
Changes in Chip Component in Material UI V5
The changes to the Chip component in Material UI V5 are significant, and they address many of the limitations and drawbacks of the previous version.
Here are a few of the key updates that have been made to the Chip component in V5:
- New Variants
In Material UI V5, there are now four different variants of the Chip component: default, outlined, filled, and text. Each variant offers a different visual style, making it easier to distinguish between different Chips and match them to your design needs.
Here’s an example of what the Chip component looks like with each variant:
import React from 'react';
import Chip from '@mui/material/Chip';
function MyChipComponent() {
return (
<div>
<Chip label="Default" variant="default" />
<Chip label="Outlined" variant="outlined" />
<Chip label="Filled" variant="filled" />
<Chip label="Text" variant="text" />
</div>
);
}
export default MyChipComponent;
As you can see, the new variants offer a lot more flexibility in terms of the visual style of the Chip component. This makes it easier to use in different types of projects and match the look and feel of your website or application.
- More Customization Options
Material UI V5 also offers more customization options for the Chip component, beyond just changing the color and size. You can now customize the typography, border radius, and spacing of the Chips.
Here’s an example of how you can customize the typography of the Chip component in Material UI V5:
import React from 'react';
import Chip from '@mui/material/Chip';
import { makeStyles } from '@mui/material/styles';
const useStyles = makeStyles((theme) => ({
customFont: {
fontFamily: 'Roboto',
fontStyle: 'italic',
fontSize: '16px',
},
}));
function MyChipComponent() {
const classes = useStyles();
return (
<div>
<Chip label="Default" />
<Chip label="Custom Font" classes={{ label: classes.customFont }} />
</div>
);
}
export default MyChipComponent;
In this example, we’re using the makeStyles
function to define a custom style for the typography of the Chip component. Then, we apply this custom style to our second Chip component by adding classes={{ label: classes.customFont }}
as a prop.
- More Accessibility Features
Finally, Material UI V5 has made a number of improvements to the accessibility of the Chip component. This includes better focus management, improved keyboard navigation, and more accessible markup.
For example, the Chip component in V5 uses semantic markup that allows for better screen reader compatibility. The role
attribute on the Chip element is set to button
by default, making it easier for users of assistive technologies to understand the purpose of the component.
Customization of Chip Component in Material UI V5
One of the benefits of the updates to the Chip component in Material UI V5 is the ability to customize it more easily.
Here are a few examples of how you can customize the Chip component in V5 using CSS and the makeStyles
function:
- Changing the Background Color
import React from 'react';
import Chip from '@mui/material/Chip';
import { makeStyles } from '@mui/material/styles';
const useStyles = makeStyles((theme) => ({
customChip: {
backgroundColor: '#f44336',
},
}));
function MyChipComponent() {
const classes = useStyles();
return (
<div>
<Chip label="Default" />
<Chip label="Custom Color" className={classes.customChip} />
</div>
);
}
export default MyChipComponent;
In this example, we’re using the makeStyles
function to define a custom style for the background color of the Chip component. Then, we apply this custom style to our second Chip component by adding className={classes.customChip}
as a prop.
- Changing the Border Radius
import React from 'react';
import Chip from '@material-ui/core/Chip';
import { makeStyles } from '@material-ui/core/styles';
const useStyles = makeStyles((theme) => ({
customChip: {
borderRadius: '50px',
},
}));
function MyChipComponent() {
const classes = useStyles();
return (
<div>
<Chip label="Default" />
<Chip label="Custom Border Radius" className={classes.customChip} />
</div>
);
}
export default MyChipComponent;
This example shows how to change the border radius of the Chip component. By setting the borderRadius
property in the makeStyles
function, we can give the Chip component more rounded edges.
- Changing the Spacing
import React from 'react';
import Chip from '@material-ui/core/Chip';
import { makeStyles } from '@material-ui/core/styles';
const useStyles = makeStyles((theme) => ({
customChip: {
margin: theme.spacing(1),
},
}));
function MyChipComponent() {
const classes = useStyles();
return (
<div>
<Chip label="Default" />
<Chip label="Custom Spacing" className={classes.customChip} />
</div>
);
}
export default MyChipComponent;
In this example, we’re using the theme.spacing
property in the makeStyles
function to add some spacing around the Chip component. This makes it stand out more on the page and gives it a cleaner look.
Optimal Use of Chip Component in Material UI V5
Now that we’ve explored the updates and customization options for the Chip component in Material UI V5, let’s talk about how to use it effectively in your web development projects.
Here are a few best practices to keep in mind when using the Chip component:
- Use Clear Labels: The labels on your Chips should be informative and easy to understand. Consider using concise labels that accurately describe the information being represented.
- Provide Visual Feedback: Make sure to provide clear visual feedback when a Chip is clicked or selected. A change in color or border can help indicate to the user that the Chip is active or has been clicked.
- Group Related Chips: If you have multiple Chips that are related to each other (such as tags or categories), consider grouping them together visually. This can make it easier for the user to understand the relationship between the Chips.
- Style for Accessibility: When customizing the Chip component, make sure to keep accessibility in mind. The component should be easily navigable using a keyboard, and the visual styles should be clear and easy to read for users with different levels of vision.
Final Thoughts
The updates and improvements made to the Chip component in Material UI V5 have made it an even more powerful tool for web developers. With more customization options and accessibility features, it’s easier than ever to use the Chip component in a wide range of projects and applications.
When using the Chip component, it’s important to keep in mind best practices for usability and accessibility. With these considerations in mind, the Chip component can be a valuable addition to your toolkit for web development projects of all shapes and sizes.
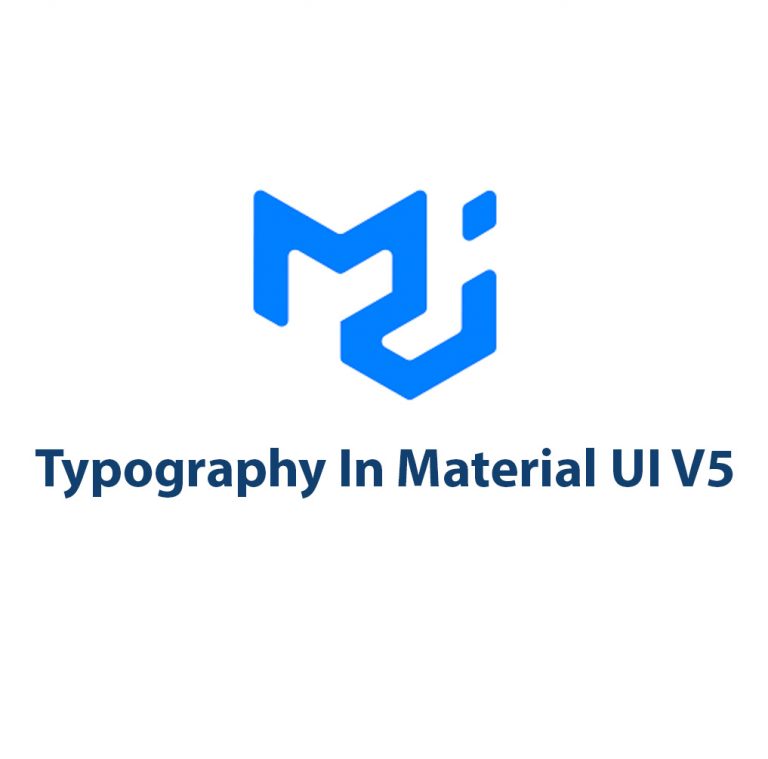
Typography In Material UI V5: A Closer Look
Introduction As a designer and developer, I’ve always been obsessed with typography. There’s something special about the way certain fonts look on a page or screen that can make or break the overall success of a design. As Material UI continues to evolve and improve, the latest version, Material UI V5, offers some exciting changes […]
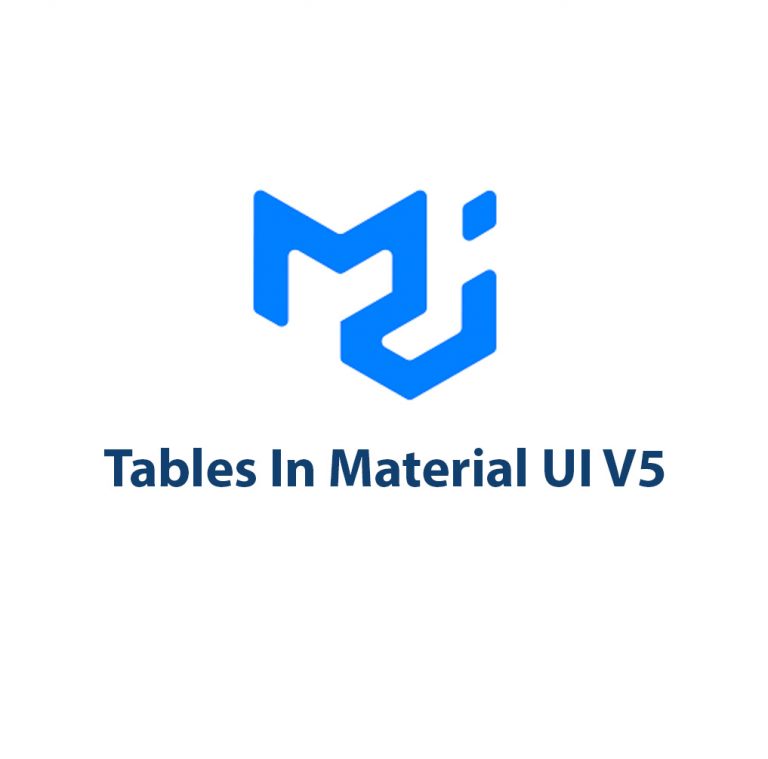
Tables In Material UI V5
Introduction As a web developer, I often find myself struggling to create functional and visually appealing tables for my web applications. When I discovered Material UI V5, I was intrigued by the promise of a streamlined and customizable table component. In this article, I’ll delve into the world of Material UI V5 Tables and share […]
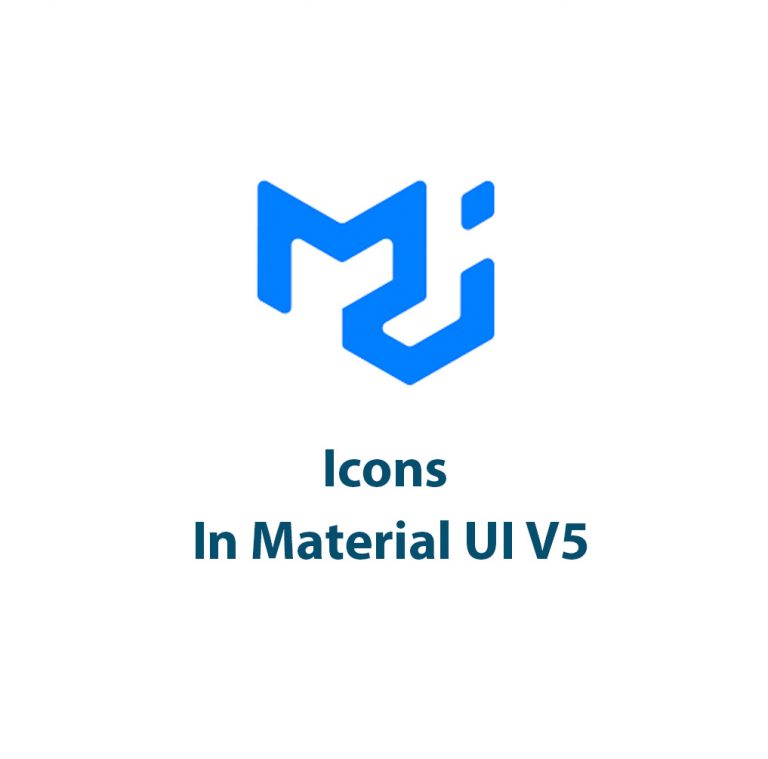
Icons In Material UI V5
Introduction: Material UI is a popular open-source framework for building web applications. Built on top of React, it provides a set of pre-built and customizable components, including typography, forms, and icons. In Material UI, icons play a crucial role in designing visually appealing interfaces and enabling smooth user experience. With the recent release of Material […]
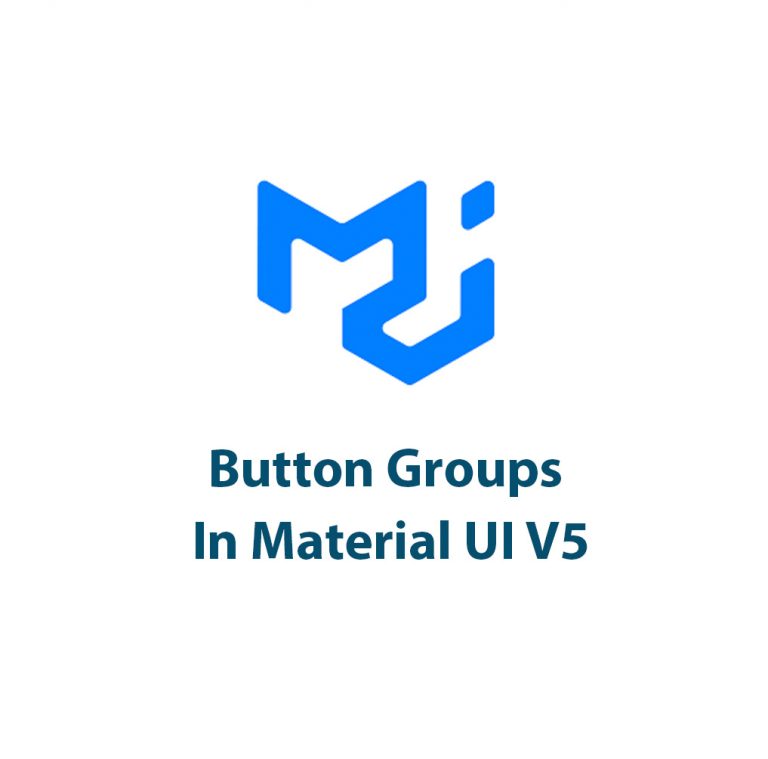
Button Groups In Material UI V5
Hey there! Welcome to my article all about button groups in Material UI V5. In this article, I’ll be giving you an in-depth insight into button groups, how they are used in UI designing, and how you can create them in Material UI V5. Introduction Before we dive into button groups, let’s start with some […]
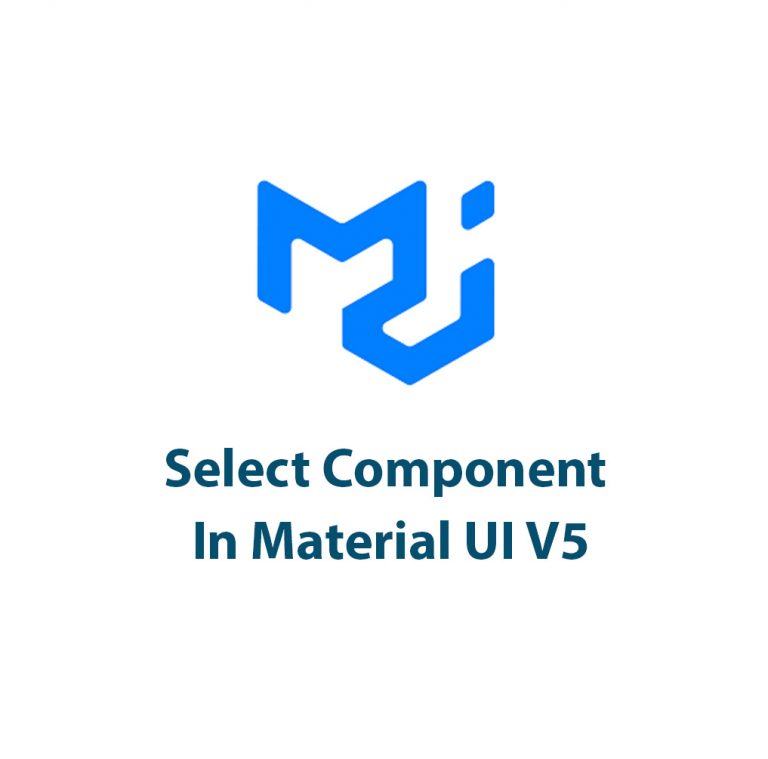
Select Component In Material UI V5
Hey there, fellow developers! In this article, we’re going to dive into the world of Material UI and take a closer look at one of its most essential components – the Select Component. I’m excited to share with you how to implement and customize this component in Material UI V5. So, let’s get started! Introduction […]
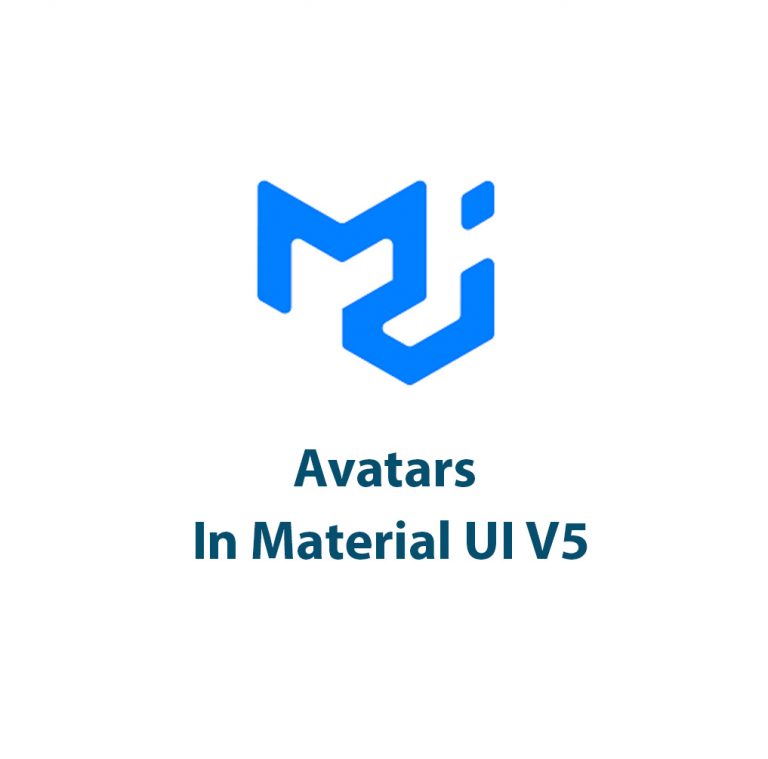
Avatars In Material UI V5
As a developer, I have always been fascinated by the way avatars enhance the user experience in web applications. They provide a personalized touch and make users feel more connected to the platform. That’s why I was excited to learn that Material UI V5 had revamped their avatar component. In this article, I’ll share my […]