Introduction
As a designer and developer, I’ve always been obsessed with typography. There’s something special about the way certain fonts look on a page or screen that can make or break the overall success of a design. As Material UI continues to evolve and improve, the latest version, Material UI V5, offers some exciting changes and new features. In this article, we’ll dive deep into typography in Material UI V5 and explore how it can be used to create beautiful and effective designs.
What Is Material UI?
Material UI is a user interface design library that is built on top of the popular React JavaScript library. It provides a set of pre-built components and styles, making it easy to create consistent and visually appealing interfaces for web applications. Material UI was inspired by Google’s Material Design, which is a design language that emphasizes clean and minimal design, with bold typography and a focus on user interaction.
What’s New In Material UI V5?
Material UI V5 is the latest version of the framework and brings a host of new and improved features. Some of the most significant changes in this version include:
- Removal of the CSS baseline – Material UI V5 no longer includes a CSS baseline, which means that you have complete control over the styling of your application.
- Modular architecture – Material UI V5 is built on a more modular architecture, which makes it easier to customize and extend the framework.
- Improved TypeScript support – Material UI V5 now has improved TypeScript support, making it easier to type-check your code and catch potential errors.
- Improved accessibility – Material UI V5 includes improved accessibility support, making it easier to build applications that are usable for all users.
Importance of Typography in Material UI
Typography plays a crucial role in design, and it is no different in Material UI. The right typography can make or break the effectiveness of a design. Good typography can set the tone and mood, while also enhancing the readability and comprehension of the content. In Material UI, typography is used to communicate information to the user, and it is crucial to ensure that it is easy to read, accessible, and visually appealing.
Understanding Typography
Before we dive into Material UI V5’s typography, let’s take a step back and explore some typography basics. Typography refers to the style, arrangement, and appearance of text, including the choice of font, font size, line spacing, color, and more. Here are some basic typography principles:
- Legibility – The main goal of typography is to make text easy to read.
- Hierarchy – Typography helps to create a visual hierarchy that guides the user through the content.
- Alignment – Using consistent alignment throughout a design creates balance and harmony.
- Contrast – Contrast is used to create emphasis and draw attention to important elements.
Typography in Material UI V5
Now that we have a basic understanding of typography let’s dive into how it is used in Material UI V5. Material UI V5 provides a set of components that are designed to make typography management easier for developers. Let’s explore some of these components in more detail:
- Typography Component – This is the main component for managing typography in Material UI V5. It includes a set of predefined styles for typography, such as headers, body text, and subheadings. With the Typography component, developers can easily style their text with a few lines of code.
import React from 'react';
import { Typography } from '@mui/material';
const App = () => {
return (
<Typography variant="h1">
Welcome to my website
</Typography>
);
};
export default App;
- Text Component – The Text component is used to render any kind of text in Material UI V5. It is highly customizable and provides a wide range of options for font size, line spacing, and more.
import React from 'react';
import { Text } from '@mui/material';
const App = () => {
return (
<Text variant="body1">
Hello, world!
</Text>
);
};
export default App;
- Link Component – The Link component is used to create clickable links in Material UI V5. It can be customized with various colors and styles, making it easy to match the design of the rest of your application.
import React from 'react';
import { Link } from '@mui/material';
const App = () => {
return (
<Link href="https://www.google.com">
Go to Google
</Link>
);
};
export default App;
- Button Component – The Button component is used to create interactive buttons in Material UI V5. It includes a wide range of customization options, including size, shape, and color.
import React from 'react';
import { Button } from '@mui/material';
const App = () => {
return (
<Button variant="contained" color="primary">
Click me!
</Button>
);
};
export default App;
Styling Typography In Material UI V5
Material UI V5 provides several options for styling typography. The first approach is to use the predefined styles provided by the Typography component. This approach is quick and easy and works well for most applications. However, if you need more advanced customizations, Material UI V5 also allows you to customize typography in several different ways.
- Theme Customization – Material UI V5 includes a theme system that allows developers to customize the style of their application across all components, including typography. This approach is useful when you want to maintain consistency across your application.
import React from 'react';
import { ThemeProvider, createTheme } from '@mui/material/styles';
import { Typography } from '@mui/material';
const theme = createTheme({
typography: {
h1: {
fontSize: '3rem',
},
},
});
const App = () => {
return (
<ThemeProvider theme={theme}>
<Typography variant="h1">
Large Heading
</Typography>
</ThemeProvider>
);
};
export default App;
- Custom CSS – Material UI V5 allows you to add custom CSS to your application, which can be used to customize the typography. This approach is useful when you need more precise control over the styling of your typography.
import React from 'react';
import { Typography } from '@mui/material';
const App = () => {
return (
<Typography variant="h1" style={{ fontFamily: 'Roboto', fontSize: 48, fontWeight: 'bold' }}>
Custom Typography
</Typography>
);
};
export default App;
Best Practices for Typography in Material UI V5
As with any design element, there are some best practices to consider when working with typography in Material UI V5. Here are some of the most important things to keep in mind:
- Font Choices – Material UI V5 includes a set of pre-defined fonts that work well with the Material Design language. When choosing a font for your design, keep legibility, readability, and brand identity in mind.
- Font Sizes – Font size is a critical element of typography, and it’s crucial to consider the font size when designing for different devices and screen sizes. Material UI V5 provides several predefined font size values that can be used to create a consistent layout across different devices.
- Line Height – Line height is the vertical space between lines of text and plays a crucial role in enhancing the legibility and readability of the content. Material UI V5 provides several predefined line height values that can be used to adjust the spacing between lines of text.
- Spacing – Spacing is another essential aspect of typography and is used to create a visual hierarchy in the design. Material UI V5 includes several predefined spacing values that can be used to create consistent spacing throughout your application.
- Color – Color is used to create emphasis and draw attention to important elements in the design. Material UI V5 includes a range of predefined color options that can be used to create a harmonious color palette for your application.
- Accessibility Considerations – When working with typography, it’s important to consider accessibility. Material UI V5 includes improved accessibility support, but it’s still crucial to keep in mind color contrast ratios, font size, and other accessibility guidelines.
Examples of Typography in Material UI V5
Now that we have covered the basics of typography in Material UI, let’s take a look at some real-world examples of typography in Material UI V5.
- Demo of Typography Components – The following example demonstrates some of the Typography components in Material UI V5 and how they can be used to create different types of text.
import React from 'react';
import { Typography } from '@mui/material';
const App = () => {
return (
<div>
<Typography variant="h1">Large Heading</Typography>
<Typography variant="h2">Medium Heading</Typography>
<Typography variant="h3">Small Heading</Typography>
<Typography variant="body1">Body Text</Typography>
<Typography variant="caption">Caption Text</Typography>
</div>
);
};
export default App;
- Custom Typography Example – The following example demonstrates how to use custom CSS to create a unique typography style for your application.
import React from 'react';
import { Typography } from '@mui/material';
const App = () => {
return (
<Typography variant="h1" style={{ fontFamily: 'Montserrat', fontSize: 48, fontWeight: 'bold', color: '#FF5722' }}>
Custom Typography
</Typography>
);
};
export default App;
- Real-World Example – The following example is a screenshot of the typography used in Google’s Material Design Guidelines.
Conclusion
Typography is an essential element of design, and it plays a crucial role in Material UI V5. Material UI V5 provides a range of components and options for managing typography in your application. Whether you are using predefined styles or customizing your typography, keep in mind the best practices we’ve covered to ensure that your typography enhances the legibility, readability, and overall effectiveness of your design.
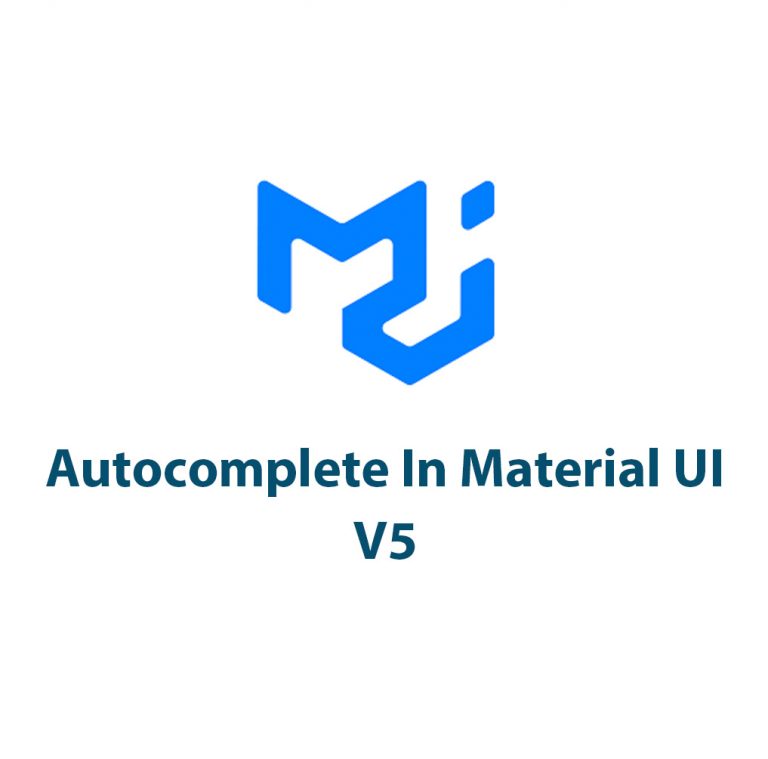
Autocomplete In Material UI V5
Introduction Hello everyone, today I am going to talk about one of the most useful components in Material UI V5 – Autocomplete. Material UI is a popular React UI framework that provides a wide range of pre-designed components to make the development of complex user interfaces simpler. Autocomplete is an intuitive component that provides suggestions […]
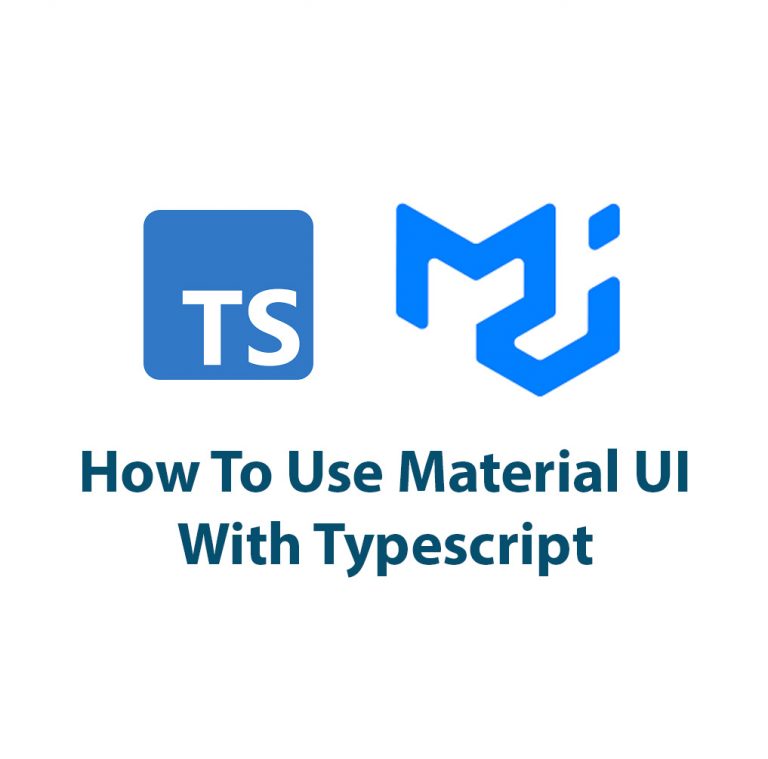
Use Material UI V5 With Typescript
Introduction Hello everyone! In this article, we are going to talk about Material UI V5 with Typescript. If you are a React developer, you must have heard of Material UI. It is one of the most popular React UI component libraries out there. On the other hand, Typescript is a superset of JavaScript that provides […]
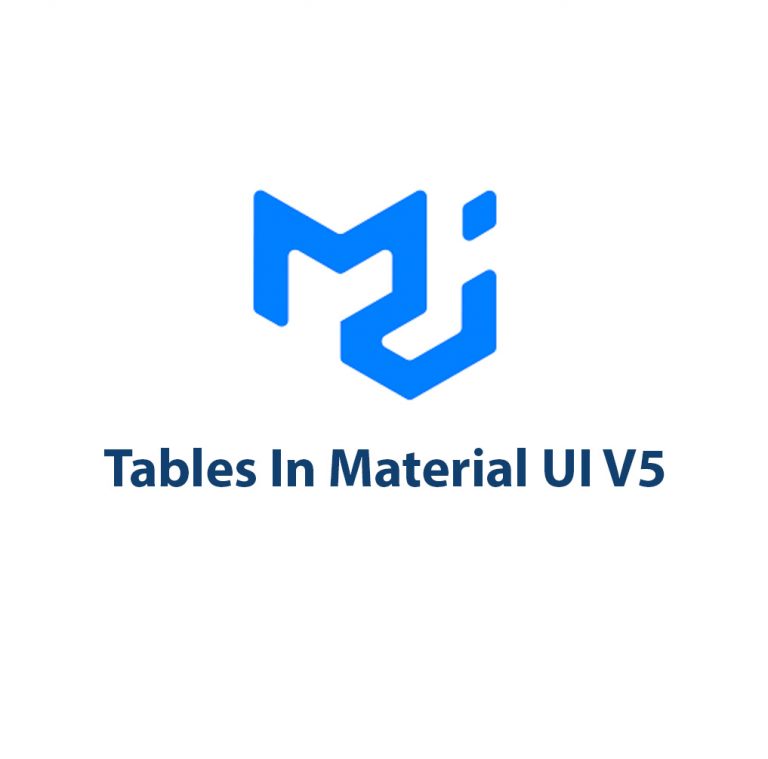
Tables In Material UI V5
Introduction As a web developer, I often find myself struggling to create functional and visually appealing tables for my web applications. When I discovered Material UI V5, I was intrigued by the promise of a streamlined and customizable table component. In this article, I’ll delve into the world of Material UI V5 Tables and share […]
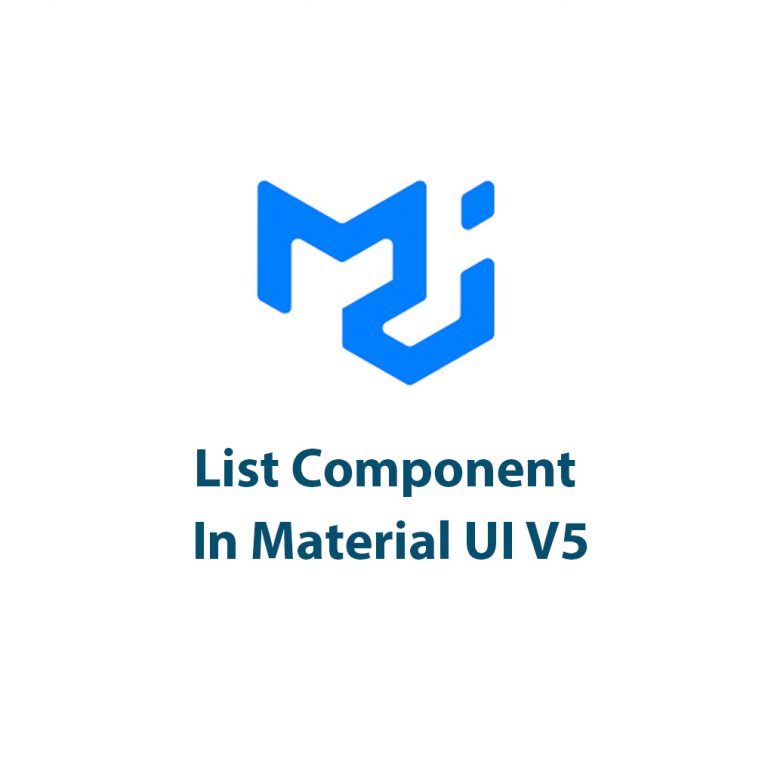
Lists In Material UI V5
Introduction As a UX designer and front-end developer, I’m always on the lookout for tools and libraries that make my job easier. When I first discovered Material UI, I was impressed by how it simplified UI development and improved the consistency of my designs. In this article, I want to focus specifically on lists in […]
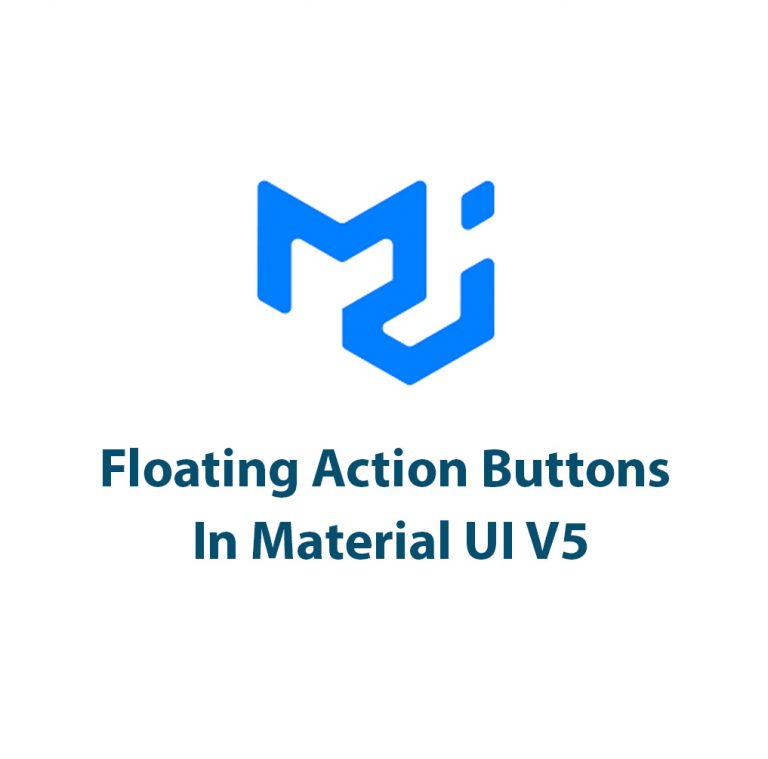
Floating Action Buttons In Material UI V5
Introduction Hey there fellow developers! Have you ever wanted to create a floating action button that seamlessly integrates with your project’s design? Well, look no further than Material UI V5 floating action buttons! Floating action buttons, or FABs, have become a popular design element in web design due to their ease of use and functionality. […]
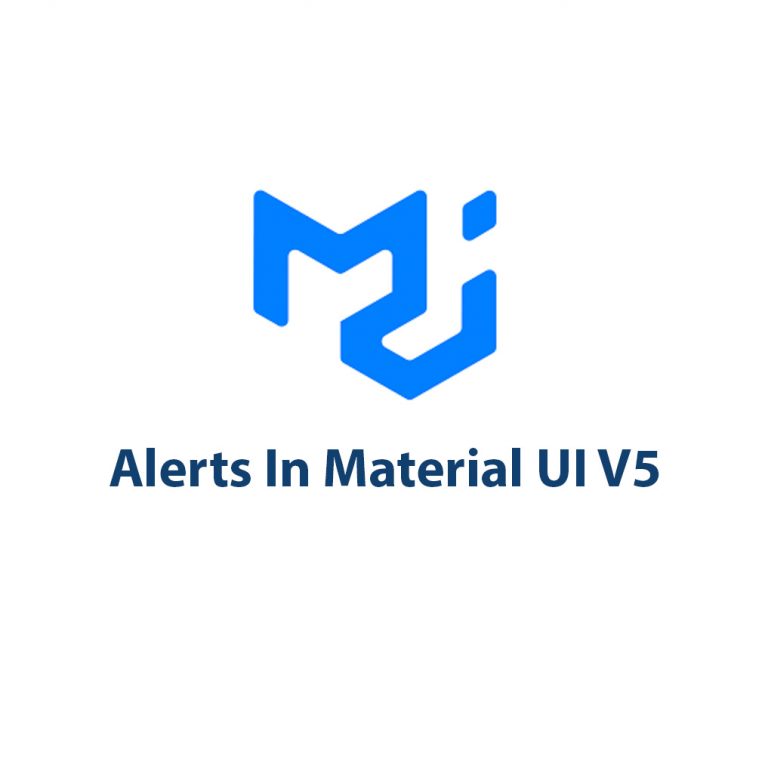
Alerts in Material UI V5
Introduction Let’s take a look at Alerts In Material UI V5. With its sleek design and easy-to-use features Material UI V5 makes it easy to show your users alerts. Alerts are an essential element of any website or application, allowing us to provide users with important information and updates. In this article, we’re going to […]