Introduction
Hey there fellow developers! Have you ever wanted to create a floating action button that seamlessly integrates with your project’s design? Well, look no further than Material UI V5 floating action buttons!
Floating action buttons, or FABs, have become a popular design element in web design due to their ease of use and functionality. They allow users to take a primary action in a simplified manner without navigating through multiple pages or menus. In this article, we’ll be exploring the benefits of using Material UI V5 floating action buttons, how to implement them into your project, and best practices for using them in your design.
But first, let’s dive into what makes Material UI V5 so special and how it enhances the floating action button experience.
Material UI V5 is a UI framework that provides pre-built components and styles for web applications. It follows the Material Design guidelines set forth by Google, which makes for a consistent, modern, and user-friendly design. Material UI V5 not only takes the hassle out of designing components from scratch but also provides customization options to fit your project’s specific needs.
Now, let’s look at the benefits of using Material UI V5 floating action buttons.
Benefit 1: Ease of use with pre-built components
One of the biggest benefits of using Material UI V5 floating action buttons is the pre-built components it provides. With just a few lines of code, you can easily implement a FAB that follows the Material Design guidelines and fits seamlessly into your project’s design. Plus, Material UI V5 has a wide variety of pre-built components that are styled to work together, making the overall design process much smoother.
Benefit 2: Customizable options for design and functionality
Material UI V5 floating action buttons also provide extensive customization options. You can customize the color, size, icon, and even the animation of the button. This makes it possible to integrate the floating action button into your project’s design while maintaining consistency. Plus, the ability to add functionality to the button allows for a more user-friendly and intuitive experience.
Benefit 3: Consistency in design with Material Design guidelines
Material UI V5 floating action buttons follow the Material Design guidelines, which provide a consistent and modern design for web applications. By using these pre-built components, you can ensure your project’s design is up-to-date and user-friendly.
Now that we know the benefits of using Material UI V5 floating action buttons, let’s dive into how to implement them into your project.
Implementation of Material UI V5 floating action buttons
To get started with Material UI V5 floating action buttons, you will need to install Material UI V5. You can do this by adding the following script to your HTML document:
<!-- Material-UI CDNs -->
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700&display=swap" />
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons" />
<link rel="stylesheet" href="https://unpkg.com/@mui/material@next/dist/mui-next.min.css" />
<!-- React and ReactDOM -->
<script src="https://unpkg.com/[email protected]/umd/react.production.min.js"></script>
<script src="https://unpkg.com/[email protected]/umd/react-dom.production.min.js"></script>
<!-- Material-UI -->
<script src="https://unpkg.com/@mui/material@next/dist/mui-next.min.js"></script>
Once you’ve installed Material UI V5, you can create a basic floating action button with the following code:
import * as React from 'react';
import Fab from '@mui/material/Fab';
import AddIcon from '@mui/icons-material/Add';
export default function BasicFAB() {
return (
<Fab color="primary" aria-label="add">
<AddIcon />
</Fab>
);
}
This code will create a basic floating action button with a primary color and an add icon in the center.
Adding iconography to the floating action button
Iconography can make a floating action button much more recognizable and intuitive. Material UI V5 provides a wide variety of icons to choose from. To add an icon to the floating action button, you will need to import the icon and add it to the button as shown below:
import * as React from 'react';
import Fab from '@mui/material/Fab';
import AddIcon from '@mui/icons-material/Add';
export default function IconFAB() {
return (
<Fab color="primary" aria-label="add">
<AddIcon />
</Fab>
);
}
Implementing animation with floating action buttons
Adding animation can make a floating action button much more interactive and appealing to the user. Material UI V5 provides animation options for its floating action buttons. To add animation to the button, you can add the animate={{}}
attribute with the desired animation options as shown below:
import * as React from 'react';
import Fab from '@mui/material/Fab';
import AddIcon from '@mui/icons-material/Add';
export default function AnimatedFAB() {
return (
<Fab
color="primary"
aria-label="add"
animate={{ scale: [1, 1.1, 0.9, 1] }}
>
<AddIcon />
</Fab>
);
}
And there you have it! You’ve implemented a basic, icon-based, and animated floating action button with Material UI V5.
But what about customizing the button design to fit your project’s specific needs? Let’s dive into that next.
Customizing Floating Action Buttons in Material UI V5
Material UI V5 provides customization options for its floating action buttons. Let’s explore a few of those options.
Changing color and size of the floating action button
Changing the color and size of the floating action button can be easily done with Material UI V5. You can add the color
attribute and choose from a wide variety of defined colors, or add a custom color using the style
attribute as shown below:
import * as React from 'react';
import Fab from '@mui/material/Fab';
import AddIcon from '@mui/icons-material/Add';
export default function ColoredFAB() {
return (
<Fab
color="secondary" // Defined color
style={{ backgroundColor: '#AB47BC',
color: '#fff' }} // Custom color
aria-label="add"
>
<AddIcon />
</Fab>
);
}
You can also change the size of the floating action button using the size
attribute. The size
attribute can have various values such as small
, medium
, and large
, or can be set to a custom value by passing a number to it.
Adding text and labels to the button
Adding text and labels to the button can make it more understandable and user-friendly. Material UI V5 provides a Typography
component that you can use to add a label to the button. You can also add an icon and text to the button by using the Button
component as shown below:
import * as React from 'react';
import Fab from '@mui/material/Fab';
import AddIcon from '@mui/icons-material/Add';
import Typography from '@mui/material/Typography';
import Button from '@mui/material/Button';
export default function LabeledFAB() {
return (
<>
<Fab color="primary" aria-label="add">
<AddIcon />
</Fab>
<Typography variant="body1">Add New Item</Typography>
<Button variant="contained" color="primary" startIcon={<AddIcon />}>
Add New Item
</Button>
</>
);
}
Implementing additional functionality with the button
Floating action buttons not only provide the ability to take a primary action, but also the potential to add additional functionality to the button. You can add a tooltip to the button to describe its functionality, or even use it to trigger a modal or a form. By using Material UI V5 components, you can quickly add functionality to your floating action button and enhance the user experience.
Best Practices for Using Floating Action Buttons in Material UI V5
While Material UI V5 floating action buttons are a great design element, it’s important to consider best practices when adding them to your project.
Placement of the button
Placement is key when it comes to floating action buttons. Consider where the button needs to be placed to make it easily accessible and noticeable to the user. It’s important to test the placement and make sure it doesn’t interfere with any other important elements in your design.
Using only necessary buttons
It’s important to consider the number of floating action buttons you need in your design. While they can be useful, having too many can cause confusion and overwhelm the user. By limiting the number of floating action buttons, you can create a more focused and straightforward user experience.
Accessibility considerations
Material UI V5 provides accessibility options for its floating action buttons. It’s important to make sure your floating action button is accessible to all users, including those who rely on assistive technology. You can ensure accessibility by adding aria-*
attributes to your button and making sure it can be navigated with a keyboard.
Testing the button for user experience
Finally, it’s essential to test the floating action button for user experience. Consider how the button fits into your overall design and how it interacts with different screen sizes and devices. By testing the button, you can ensure a seamless and user-friendly experience for all users.
Conclusion
In conclusion, Material UI V5 floating action buttons provide an easy-to-use and customizable design element that can enhance the user experience of your web application. By following the Material Design guidelines and customizing the button to fit your project’s specific needs, you can create a more modern and user-friendly design. Finally, by considering best practices and testing the button for user experience, you can ensure a seamless and straightforward user experience for all users. So, give Material UI V5 floating action buttons a try in your next project and see the difference they can make!
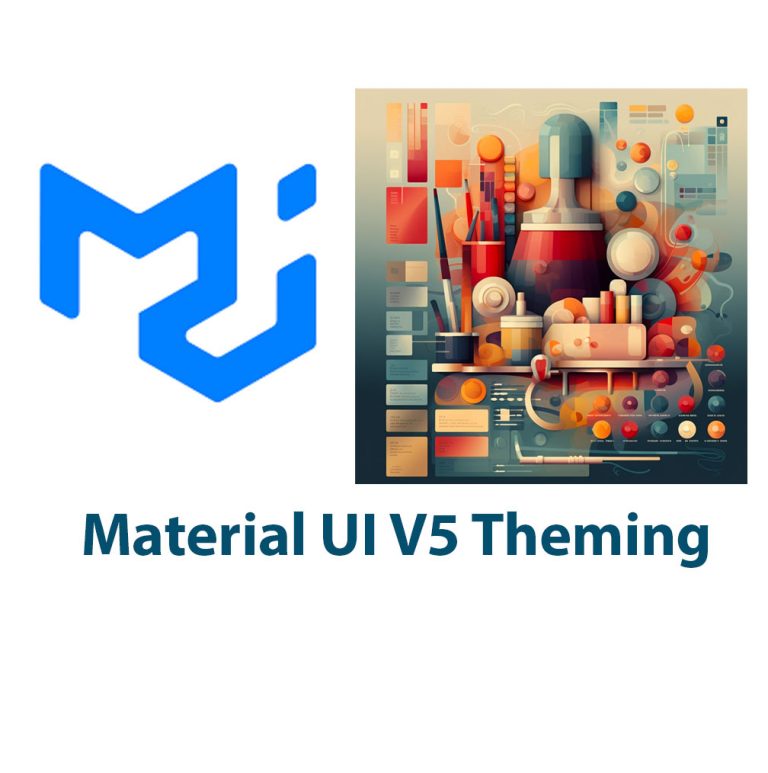
Material UI V5 Theming
Introduction: Popular React UI framework Material UI provides a large selection of pre-built components to easily build responsive user interfaces. The library’s design approach, which is based on Google’s Material Design principles, has helped it become more well-liked among developers. The design framework of Material UI is crucial to the creation of user interfaces since […]
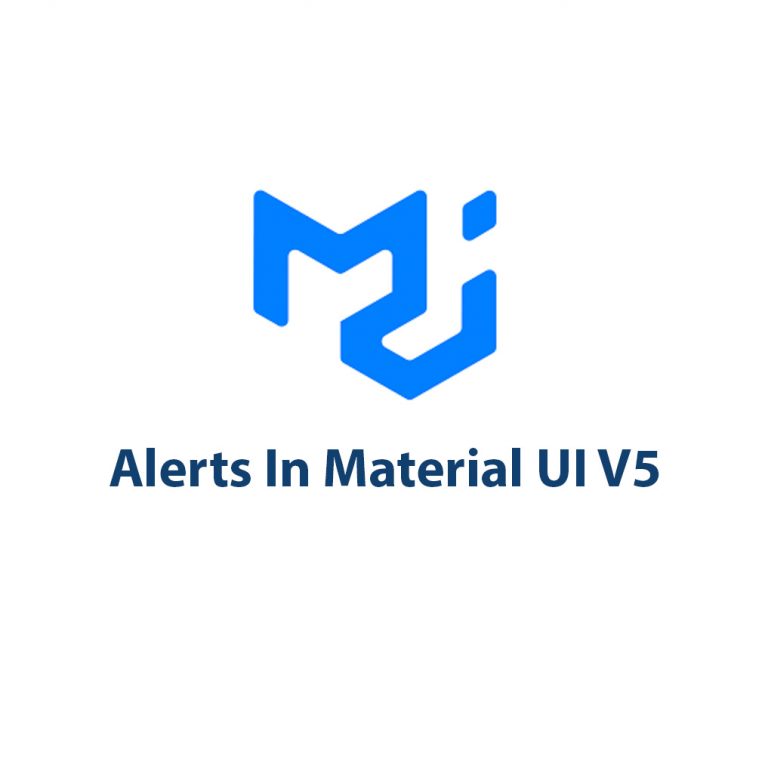
Alerts in Material UI V5
Introduction Let’s take a look at Alerts In Material UI V5. With its sleek design and easy-to-use features Material UI V5 makes it easy to show your users alerts. Alerts are an essential element of any website or application, allowing us to provide users with important information and updates. In this article, we’re going to […]
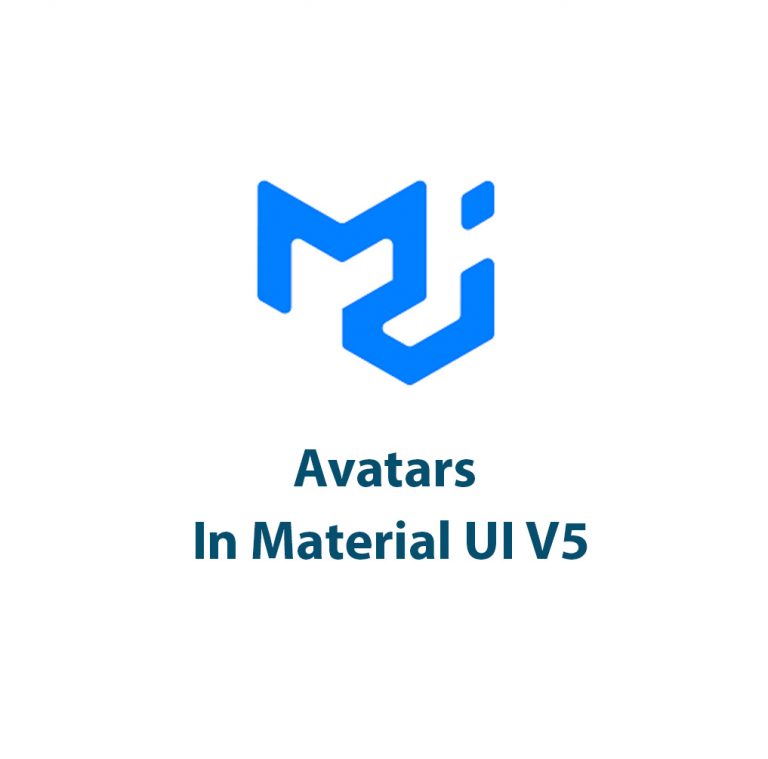
Avatars In Material UI V5
As a developer, I have always been fascinated by the way avatars enhance the user experience in web applications. They provide a personalized touch and make users feel more connected to the platform. That’s why I was excited to learn that Material UI V5 had revamped their avatar component. In this article, I’ll share my […]
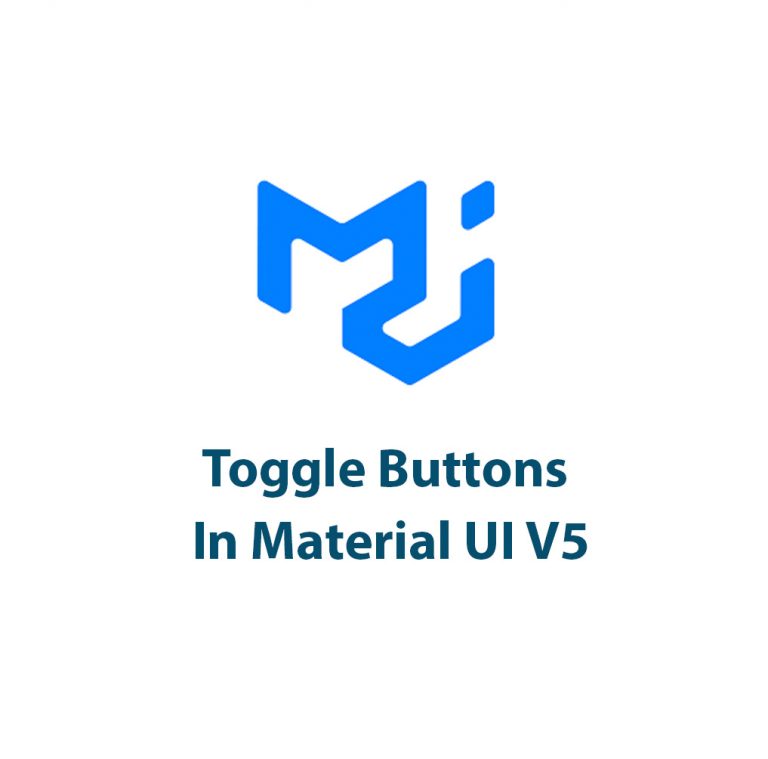
Toggle Buttons In Material UI V5
As a developer, I’ll be the first to admit that sometimes I find myself perplexed by certain UI design choices. As someone who spends a lot of time within the realm of code, I focus more on functionality and less on design. But the more I learn about UI design, the more I realize the […]
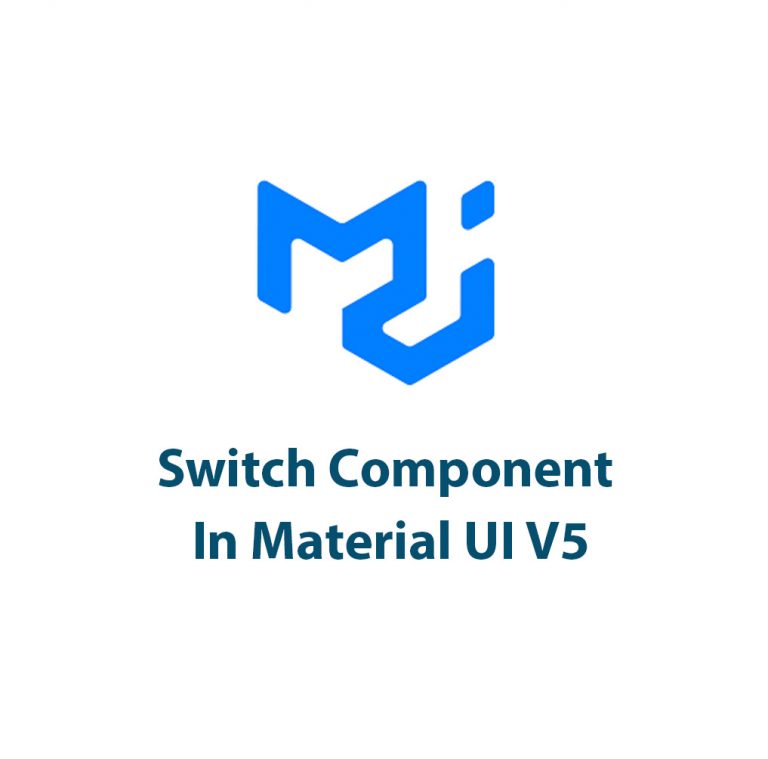
Switch Component In Material UI V5
Hey there, fellow developers! Are you excited about exploring the latest version of Material UI and all the new features it brings? If so, you’ve landed in the right place because today we’re going to dive deep into the Switch Component in Material UI v5. Introduction Before we get into the nitty-gritty of the Switch […]
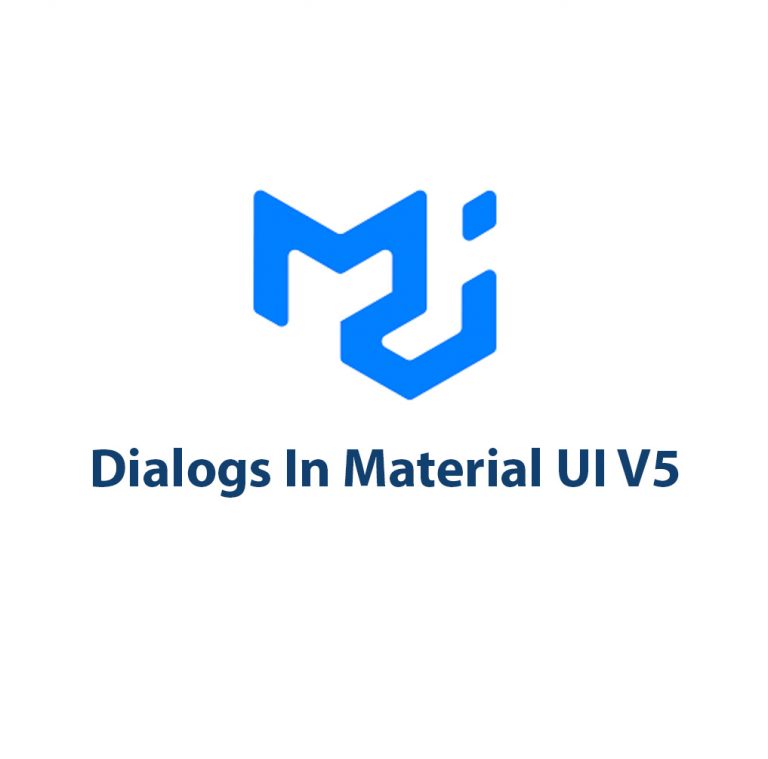