Introduction
Let’s take a look at Alerts In Material UI V5. With its sleek design and easy-to-use features Material UI V5 makes it easy to show your users alerts.
Alerts are an essential element of any website or application, allowing us to provide users with important information and updates. In this article, we’re going to take a deep dive into Material UI V5 alerts, exploring the different types, customization options, and common use cases.
Material UI V5 Alerts
Material UI V5 offers four different types of alerts: success, warning, error, and info. Each alert type has its own unique style, making it easy to differentiate between different types of alerts.
One of the great things about Material UI V5 alerts is that they can be easily customized to fit the style of your website or application. For example, you can change the background color, text color, border radius, and more. To customize an alert, you simply need to pass a set of properties to the alert component.
import React from 'react';
import Alert from '@mui/material/Alert';
import Stack from '@mui/material/Stack';
export default function CustomizedAlerts() {
return (
<Stack sx={{ width: '100%' }} spacing={2}>
<Alert variant="filled" severity="success">
This is a success alert — check it out!
</Alert>
<Alert variant="filled" severity="warning">
This is a warning alert — check it out!
</Alert>
<Alert variant="filled" severity="error">
This is an error alert — check it out!
</Alert>
<Alert variant="filled" severity="info">
This is an info alert — check it out!
</Alert>
</Stack>
);
}
In this code example, we’re importing the Alert and Stack components from Material UI. We’re then rendering four different alerts with different severity levels (success, warning, error, and info). The variant
property is set to “filled”, which gives the alerts a solid filled background. We can also set variant
to “outlined” which gives the alerts an outlined border.
We can further customize an alert by passing additional properties. For example, we can add an icon to the alert by setting the icon
property:
<Alert
icon={<CheckCircleIcon sx={{ color: green[600] }} />}
severity="success"
>
This is a success alert — check it out!
</Alert>
In this example, we’re adding the CheckCircleIcon
component from Material UI as the icon for the success alert. We’re also setting the icon color to green.
Handling Alert State
When working with alerts, it’s important to handle the alert state. This involves managing when alerts are shown or hidden based on different events or user interactions.
In Material UI V5, we can use React hooks to manage the alert state. We can set up a variable to track the alert open state, and then use a useState
hook to update the state when necessary.
import React, { useState } from 'react';
import Alert from '@mui/material/Alert';
export default function AlertExample() {
const [open, setOpen] = useState(true);
const handleClose = (event, reason) => {
if (reason === 'clickaway') {
return;
}
setOpen(false);
};
return (
<div>
{open && (
<Alert onClose={handleClose} severity="error">
This is an error alert — check it out!
</Alert>
)}
</div>
);
}
In this code example, we’re using the useState
hook to set the open
variable to true
by default. We’re also defining a handleClose
function that updates the open
state to false
when the user clicks the close button on the alert.
Advanced Alert Customization
Material UI V5 alerts can be customized even further with more advanced techniques. For example, we can add icons to alerts using the Avatar
component:
import React from 'react';
import Alert from '@mui/material/Alert';
import Avatar from '@mui/material/Avatar';
import Stack from '@mui/material/Stack';
import GradeIcon from '@mui/icons-material/Grade';
export default function AvatarAlerts() {
return (
<Stack sx={{ width: '100%' }} spacing={2}>
<Alert
action={
<Avatar
sx={{ bgcolor: '#3f51b5' }}
>
<GradeIcon />
</Avatar>
}
severity="success"
>
This is a success alert with an icon — check it out!
</Alert>
<Alert
action={
<Avatar
sx={{ bgcolor: '#f44336' }}
>
<ErrorIcon />
</Avatar>
}
severity="error"
>
This is an error alert with an icon — check it out!
</Alert>
</Stack>
);
}
In this example, we’re importing the Avatar
component from Material UI. We’re then rendering two different alerts with different severity levels (success, error) and a custom avatar icon for each.
We can also customize the alert theme to match the style of our website or application using the ThemeProvider
component:
import React from 'react';
import Alert from '@mui/material/Alert';
import { createTheme, ThemeProvider } from '@mui/material/styles';
const theme = createTheme({
components: {
MuiAlert: {
styleOverrides: {
root: {
backgroundColor: '#f7dfc5'
},
message: {
color: '#6e2f00'
},
action: {
color: '#6e2f00'
}
}
}
}
});
export default function CustomizedAlerts() {
return (
<ThemeProvider theme={theme}>
<Alert severity="warning">
This is a warning alert with a custom theme — check it out!
</Alert>
</ThemeProvider>
);
}
In this example, we’re creating a custom theme
using the createTheme
function from Material UI. We’re then defining a custom theme for the MuiAlert
component, which overrides the default style properties. In this case, we’re setting the background color to a light yellow, the message color to a dark brown, and the action color to the same dark brown.
Common Alert Use Cases
Now that we’ve explored the different types, customization options, and advanced techniques for Material UI V5 alerts, let’s take a look at some common use cases for alerts in web development.
Form Validation
Form validation is a common use case for alerts, as it allows users to see if they’ve made any mistakes or missed any required fields before submitting the form. Here’s an example of how we can use Material UI V5 alerts for form validation:
import React, { useState } from 'react';
import Alert from '@mui/material/Alert';
import Button from '@mui/material/Button';
import TextField from '@mui/material/TextField';
import Stack from '@mui/material/Stack';
export default function FormValidation() {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const [alert, setAlert] = useState(null);
const handleSubmit = (e) => {
e.preventDefault();
if (!name || !email) {
setAlert(
<Alert onClose={() => setAlert(null)} severity="error">
Please fill out all required fields.
</Alert>
);
return;
}
// Form submission logic here
};
return (
<>
{alert}
<form onSubmit={handleSubmit}>
<Stack direction="column" spacing={2}>
<TextField
label="Name"
variant="outlined"
required
value={name}
onChange={(e) => setName(e.target.value)}
/>
<TextField
label="Email"
variant="outlined"
required
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
<Button type="submit" variant="contained">Submit</Button>
</Stack>
</form>
</>
);
}
In this example, we’re using the useState
hook to set up variables for the name and email form fields, as well as the alert state. We’re then defining a handleSubmit
function that checks if the name and email fields are filled out. If not, we’re displaying an error alert using the Material UI V5 Alert
component. If the fields are valid, we can perform the form submission logic.
User Feedback
Alerts can also be used to provide users with feedback on their actions, such as successfully creating a new account or logging in. Here’s an example of how we can use Material UI V5 alerts for user feedback:
import React, { useState } from 'react';
import Alert from '@mui/material/Alert';
import Button from '@mui/material/Button';
export default function UserFeedback() {
const [alert, setAlert] = useState(null);
const handleCreateAccount = () => {
// Logic to create new account
setAlert(
<Alert onClose={() => setAlert(null)} severity="success">
Your account has been successfully created!
</Alert>
);
};
return (
<>
{alert}
<Button variant="contained" onClick={handleCreateAccount}>Create Account</Button>
</>
);
}
In this example, we’re using the useState
hook to set up the alert state. We’re then defining a handleCreateAccount
function that performs the logic to create a new account. Once the account is created, we’re displaying a success alert using the Material UI V5 Alert
component.
Conclusion
Material UI V5 alerts offer a sleek, customizable, and easy-to-use solution for alerts in web development. With their different types, customization options, and advanced techniques, Material UI V5 alerts can handle a wide range of use cases, from form validation to user feedback. Whether you’re a seasoned developer or just starting out, Material UI V5 alerts are definitely worth exploring. With a burst of excitement, I urge you to try out Material UI V5 alerts in your own projects and incorporate them into your web development toolbox.
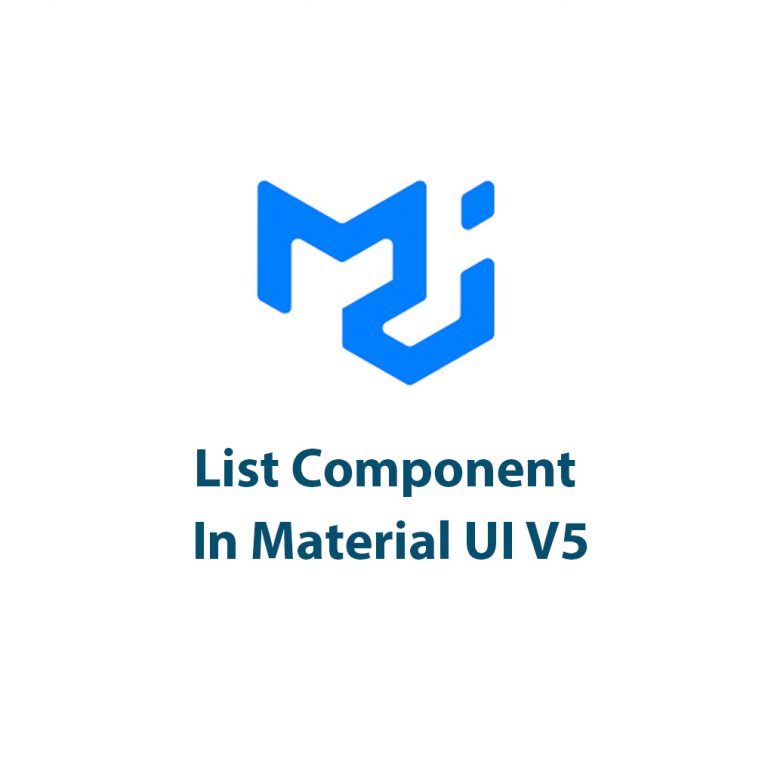
Lists In Material UI V5
Introduction As a UX designer and front-end developer, I’m always on the lookout for tools and libraries that make my job easier. When I first discovered Material UI, I was impressed by how it simplified UI development and improved the consistency of my designs. In this article, I want to focus specifically on lists in […]
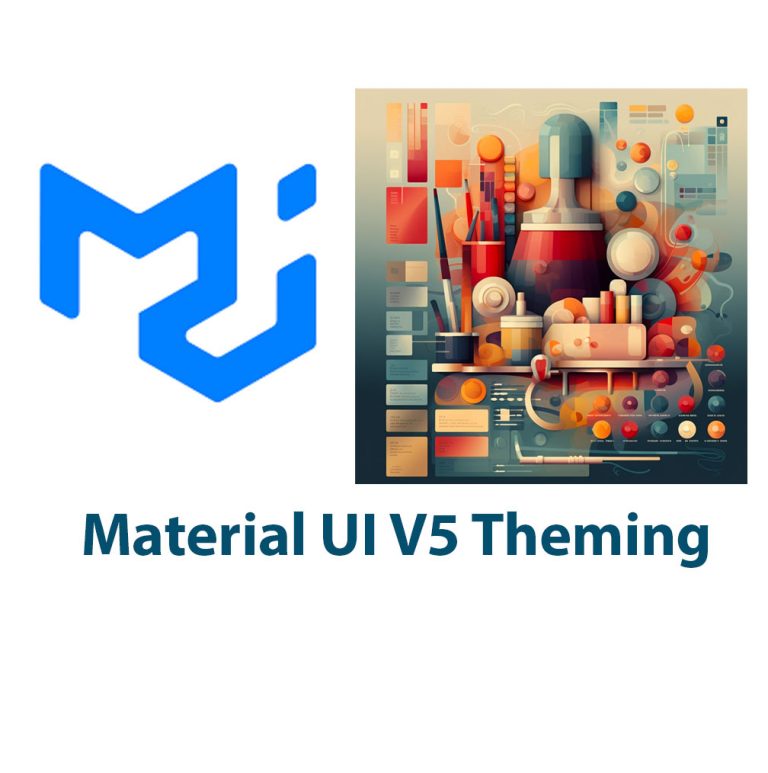
Material UI V5 Theming
Introduction: Popular React UI framework Material UI provides a large selection of pre-built components to easily build responsive user interfaces. The library’s design approach, which is based on Google’s Material Design principles, has helped it become more well-liked among developers. The design framework of Material UI is crucial to the creation of user interfaces since […]
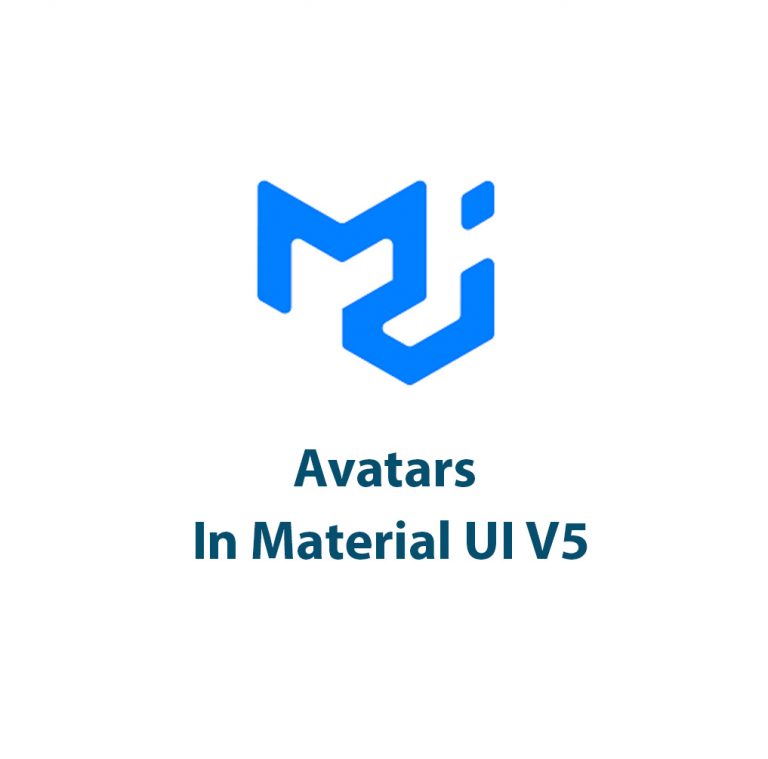
Avatars In Material UI V5
As a developer, I have always been fascinated by the way avatars enhance the user experience in web applications. They provide a personalized touch and make users feel more connected to the platform. That’s why I was excited to learn that Material UI V5 had revamped their avatar component. In this article, I’ll share my […]
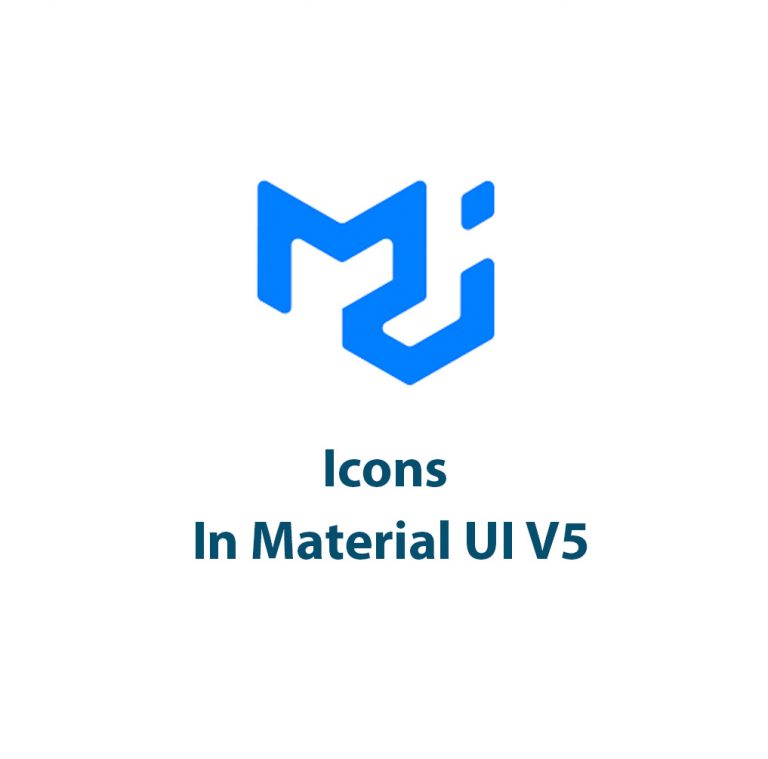
Icons In Material UI V5
Introduction: Material UI is a popular open-source framework for building web applications. Built on top of React, it provides a set of pre-built and customizable components, including typography, forms, and icons. In Material UI, icons play a crucial role in designing visually appealing interfaces and enabling smooth user experience. With the recent release of Material […]
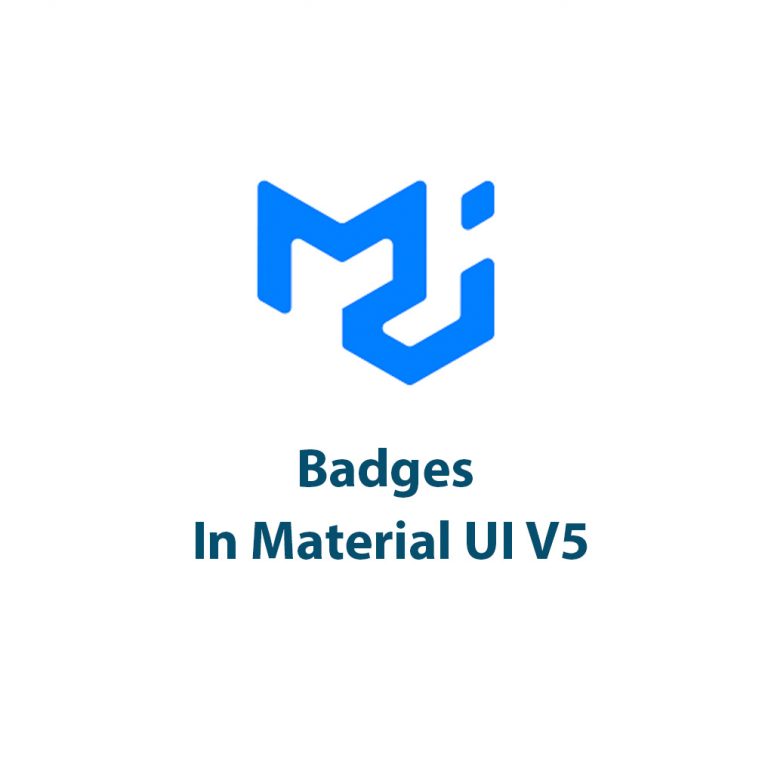
Badges In Material UI V5
As a web developer, I’ve always been fascinated by the small design elements that can make a big impact on the overall user experience of a website. One such element is the badge, often used to highlight new or important information to users. In the latest release of Material UI, version 5, badges have seen […]
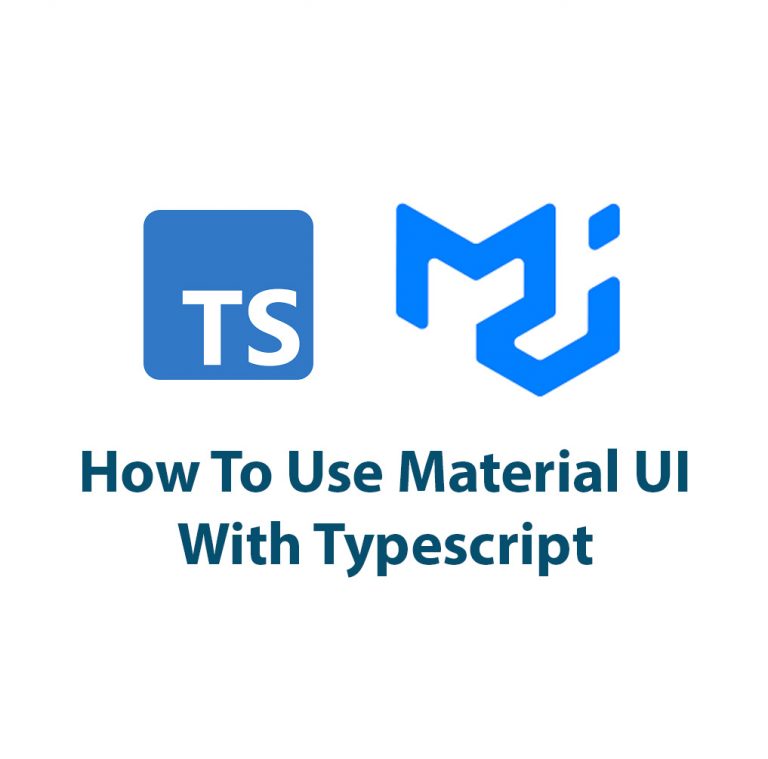
Use Material UI V5 With Typescript
Introduction Hello everyone! In this article, we are going to talk about Material UI V5 with Typescript. If you are a React developer, you must have heard of Material UI. It is one of the most popular React UI component libraries out there. On the other hand, Typescript is a superset of JavaScript that provides […]