As a developer, I’ll be the first to admit that sometimes I find myself perplexed by certain UI design choices. As someone who spends a lot of time within the realm of code, I focus more on functionality and less on design. But the more I learn about UI design, the more I realize the importance of creating an interface that is user-friendly and visually appealing. This is where UI frameworks like Material UI V5 come into play.
In this article, I want to explore one specific component of Material UI V5: the toggle button. Toggle buttons are an essential component that allows users to switch between two states. They are commonly used in many applications, from settings menus to filtering mechanisms. As someone who has used toggle buttons before, I was perplexed by how to create them in Material UI V5, so naturally, I dove in headfirst to figure it out.
Before we get started, there are a few prerequisites that you should keep in mind. Firstly, it’s important to have a basic understanding of React, as Material UI V5 is built on top of it. If you’re unfamiliar with React, I highly recommend starting with the official React documentation to get up to speed. Secondly, you’ll need to have Material UI V5 installed and set up in your project. You can do this by following the official installation guide on Material UI’s website.
Now that we have the prerequisites out of the way, let’s dive into creating our first toggle button!
Creating a Basic Toggle Button
Material UI V5 has done an excellent job of creating reusable components that make it easy to create UI elements quickly. As such, it should come as no surprise that they’ve included a toggle button component that we can use right off the bat. Let’s go ahead and create a basic toggle button using the ToggleButton component provided by Material UI V5.
import React, {useState} from 'react';
import {ToggleButton, ToggleButtonGroup} from '@mui/material';
function App() {
const [selectedButton, setSelectedButton] = useState(false);
const handleToggleButtonChange = (event, newSelectedButton) => {
setSelectedButton(newSelectedButton);
};
return (
<div>
<ToggleButtonGroup>
<ToggleButton
value="check"
selected={selectedButton}
onChange={handleToggleButtonChange}
>
Toggle Me
</ToggleButton>
</ToggleButtonGroup>
</div>
);
}
export default App;
In this code snippet, we’ve imported the ToggleButton and ToggleButtonGroup components from Material UI V5. The ToggleButtonGroup component is used to group together multiple toggle buttons, but in this case, we only have one, so we’ve wrapped it inside a div for simplicity.
Next, we’ve defined a state hook using the useState function provided by React. The state hook has two pieces of state: the currently selected button (initialized to false), and a function that will allow us to update the selected button.
Our handleToggleButtonChange function takes an event and a newSelectedButton. The event parameter is used to prevent the default behavior, and the newSelectedButton parameter will tell us which button was clicked. We set the new value of the selectedButton state using the setSelectedButton function.
Finally, we’ve rendered the ToggleButton component and passed in some important props. The value prop is used to identify the button. In this case, we’ve set the value to “check”, but you can change it to whatever value you’d like. The selected prop tells us whether the button is currently selected (initialized to false), and the onChange prop is used to handle changes to the selected button.
And just like that, we’ve created our first toggle button!
Working with Icons in Toggle Buttons
Okay, so we’ve created a basic toggle button, but what if we want to add an icon to it? Fortunately, Material UI V5 has a lot of built-in icons that we can use in our toggle buttons. Let’s go ahead and add an icon to our existing toggle button.
import React, {useState} from 'react';
import {ToggleButton, ToggleButtonGroup} from '@mui/material';
import {Check, Clear} from '@mui/icons-material';
function App() {
const [selectedButton, setSelectedButton] = useState(false);
const handleToggleButtonChange = (event, newSelectedButton) => {
setSelectedButton(newSelectedButton);
};
return (
<div>
<ToggleButtonGroup>
<ToggleButton
value="check"
selected={selectedButton}
onChange={handleToggleButtonChange}
startIcon={<Check />}
endIcon={<Clear />}
>
Toggle Me
</ToggleButton>
</ToggleButtonGroup>
</div>
);
}
export default App;
In this code snippet, we’ve added two icons to our toggle button using the startIcon and endIcon props. The startIcon prop is used to add an icon before the button’s text, and the endIcon prop is used to add an icon after the button’s text.
We’ve imported the Check and Clear icons from Material UI’s icons-material package. We’ve then passed them in as properties to the appropriate props: startIcon and endIcon.
And just like that, we’ve added icons to our toggle button!
Handling State in Toggle Buttons
Now that we’ve created a basic toggle button and added some icons to it, let’s talk about handling state. Our existing toggle button only has two states: on and off. But what if we want to change it so that the button can toggle between multiple states?
Let’s go ahead and update our code to accommodate this.
import React, {useState} from 'react';
import {ToggleButton, ToggleButtonGroup} from '@mui/material';
import {Check, Clear} from '@mui/icons-material';
function App() {
const [selectedButton, setSelectedButton] = useState(null);
const handleToggleButtonChange = (event, newSelectedButton) => {
setSelectedButton(newSelectedButton);
};
return (
<div>
<ToggleButtonGroup
value={selectedButton}
onChange={handleToggleButtonChange}
>
<ToggleButton value="check">
<Check />
</ToggleButton>
<ToggleButton value="cross">
<Clear />
</ToggleButton>
</ToggleButtonGroup>
</div>
);
}
export default App;
In this code snippet, we’ve made some changes to our handleToggleButtonChange function. Instead of setting the selected button to either true or false, we’re now setting it to null. This allows us to have multiple states that can be toggled on and off.
We’ve also updated our ToggleButtonGroup component to
take in the selectedButton state as its value prop, and the handleToggleButtonChange function as its onChange prop. This ensures that the selected value is always up-to-date.
Finally, we’ve added two ToggleButton components to our ToggleButtonGroup, each with a unique value prop. We’ve also added the Check and Clear icons to their respective buttons.
Now when we run our code, we’ll notice that we can toggle between two states instead of just one.
Advanced Toggle Button Features
Up to this point, we’ve covered how to create a basic toggle button, add icons to it, and handle state in different ways. But Material UI V5 also offers some advanced features that we should explore.
Disabled Toggle Buttons
One of those features is the ability to disable a toggle button. Let’s go ahead and add a disabled toggle button to our code.
import React, {useState} from 'react';
import {ToggleButton, ToggleButtonGroup} from '@mui/material';
import {Check, Clear} from '@mui/icons-material';
function App() {
const [selectedButton, setSelectedButton] = useState(null);
const handleToggleButtonChange = (event, newSelectedButton) => {
setSelectedButton(newSelectedButton);
};
return (
<div>
<ToggleButtonGroup
value={selectedButton}
onChange={handleToggleButtonChange}
>
<ToggleButton value="check">
<Check />
</ToggleButton>
<ToggleButton value="cross" disabled>
<Clear />
</ToggleButton>
</ToggleButtonGroup>
</div>
);
}
export default App;
In this code snippet, we’ve added the disabled prop to our second ToggleButton component. This prop disables the toggle button, preventing the user from selecting it. We’ve also added the disabled attribute to its child icon, which will visually indicate that it’s disabled to the user.
Checked Toggle Buttons
Another advanced feature of Material UI V5 toggle buttons is the ability to set the default state of a toggle button. We can do this by setting the checked prop to true. Let’s see how this works.
import React, {useState} from 'react';
import {ToggleButton, ToggleButtonGroup} from '@mui/material';
import {Check, Clear} from '@mui/icons-material';
function App() {
const [selectedButton, setSelectedButton] = useState("check");
const handleToggleButtonChange = (event, newSelectedButton) => {
setSelectedButton(newSelectedButton);
};
return (
<div>
<ToggleButtonGroup
value={selectedButton}
onChange={handleToggleButtonChange}
>
<ToggleButton value="check" checked>
<Check />
</ToggleButton>
<ToggleButton value="cross">
<Clear />
</ToggleButton>
</ToggleButtonGroup>
</div>
);
}
export default App;
In this code snippet, we’ve added the checked prop to our first ToggleButton component. This sets the default state of the button to true, meaning it will be selected by default when the component is rendered.
onChange Toggle Buttons
Finally, Material UI V5’s toggle buttons offer the onChange event, which allows us to take action in response to a change in the toggle button’s selected state. Let’s see how we can use this to our advantage.
import React, {useState} from 'react';
import {ToggleButton, ToggleButtonGroup} from '@mui/material';
import {Check, Clear} from '@mui/icons-material';
function App() {
const [selectedButton, setSelectedButton] = useState("check");
const handleToggleButtonChange = (event, newSelectedButton) => {
setSelectedButton(newSelectedButton);
};
const onToggle = () => {
console.log("Toggle button has been toggled!");
}
return (
<div>
<ToggleButtonGroup
value={selectedButton}
onChange={handleToggleButtonChange}
onChangeCapture={onToggle}
>
<ToggleButton value="check" checked>
<Check />
</ToggleButton>
<ToggleButton value="cross">
<Clear />
</ToggleButton>
</ToggleButtonGroup>
</div>
);
}
export default App;
We’ve added a new function, onToggle, to our code. This function logs a message to the console indicating that the toggle button has been toggled.
We’ve also added the onChangeCapture prop to our ToggleButtonGroup component, which is triggered whenever a change occurs in any of the toggle buttons within the group.
Theming Toggle Buttons
Finally, let’s talk about theming our toggle buttons. Material UI V5 has a robust theming system that allows us to customize the look and feel of our app’s components, including toggle buttons.
import React, {useState} from 'react';
import {ThemeProvider, createTheme} from '@mui/material/styles';
import {ToggleButton, ToggleButtonGroup} from '@mui/material';
import {Check, Clear} from '@mui/icons-material';
const theme = createTheme({
components: {
MuiToggleButton: {
styleOverrides: {
root: {
borderRadius: "20px",
backgroundColor: "lightblue",
},
selected: {
backgroundColor: "blue"
}
},
},
},
});
function App() {
const [selectedButton, setSelectedButton] = useState("check");
const handleToggleButtonChange = (event, newSelectedButton) => {
setSelectedButton(newSelectedButton);
};
return (
<ThemeProvider theme={theme}>
<div>
<ToggleButtonGroup
value={selectedButton}
onChange={handleToggleButtonChange}
>
<ToggleButton value="check" checked>
<Check />
</ToggleButton>
<ToggleButton value="cross">
<Clear />
</ToggleButton>
</ToggleButtonGroup>
</div>
</ThemeProvider>
);
}
export default App;
In this code snippet, we’ve created a custom theme using the createTheme function provided by Material UI V5. This theme object overrides the default styles of the MuiToggleButton component. We’ve set the border radius to 20px, and the background color to light blue.
We’ve also added a selected styleOverride, which is applied to the selected toggle button. This changes the background color to blue when the selected button is toggled on.
We’ve wrapped our ToggleButtonGroup component within a ThemeProvider component, and passed in our custom theme as a prop to the ThemeProvider. This ensures that our toggle buttons are styled according to our custom theme.
And there you have it, a fully-functioning toggle button, styled to our liking using Material UI V5’s theming system.
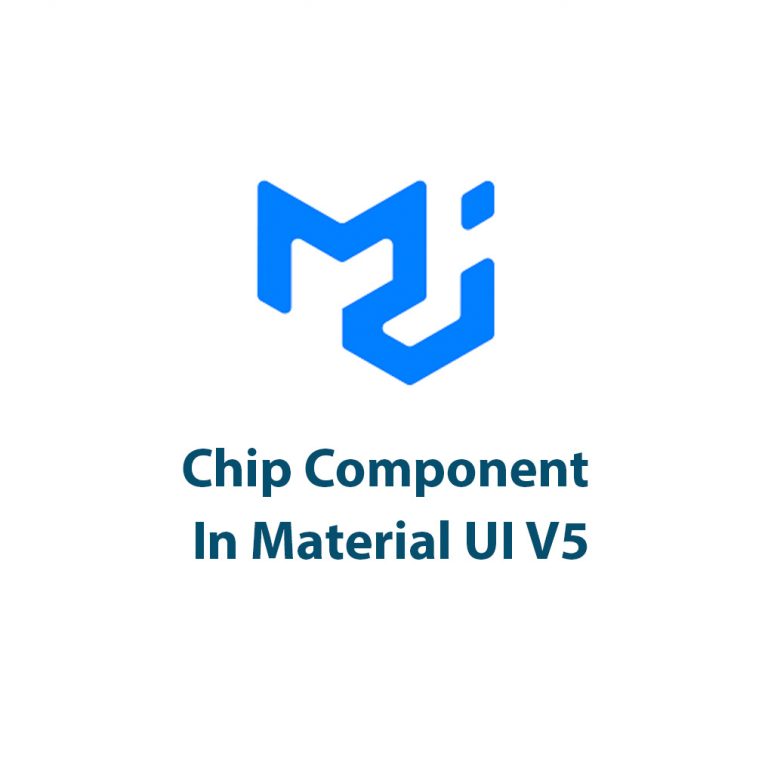
Chip Component In Material UI V5
As a web developer, I’m always on the lookout for new and improved tools and frameworks to make my life easier. And one of the tools that has been a staple in my toolkit is Material UI. If you’re not familiar with Material UI, it is a library that provides pre-built UI components for web […]
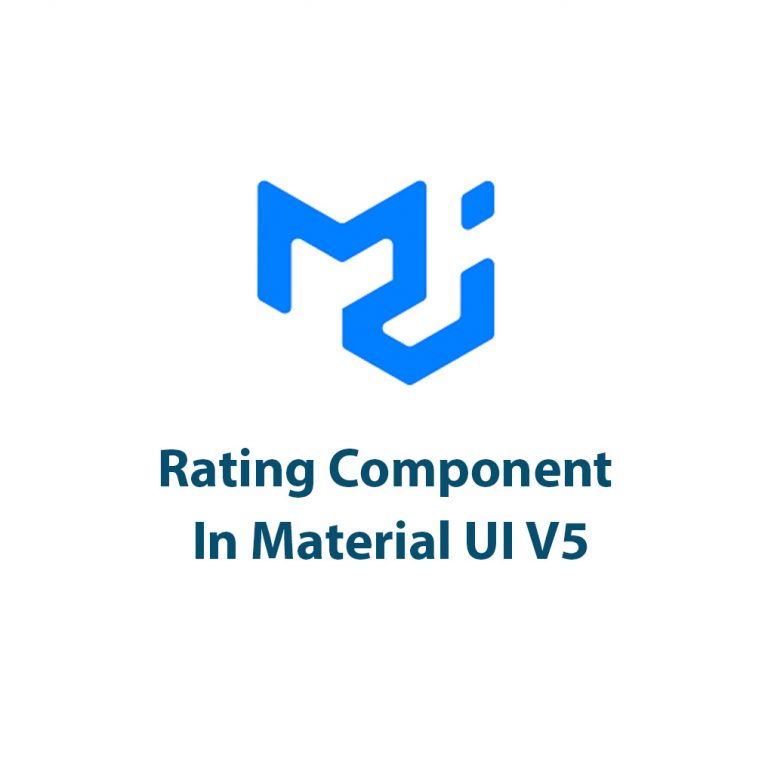
Rating Component In Material UI V5
Hello and welcome to this exciting article about the Rating Component in Material UI V5! As a web developer, I have come to really appreciate the simplicity and flexibility that this UI library provides, especially when it comes to components that add interactivity to user interfaces. In this article, I’m going to walk you through […]
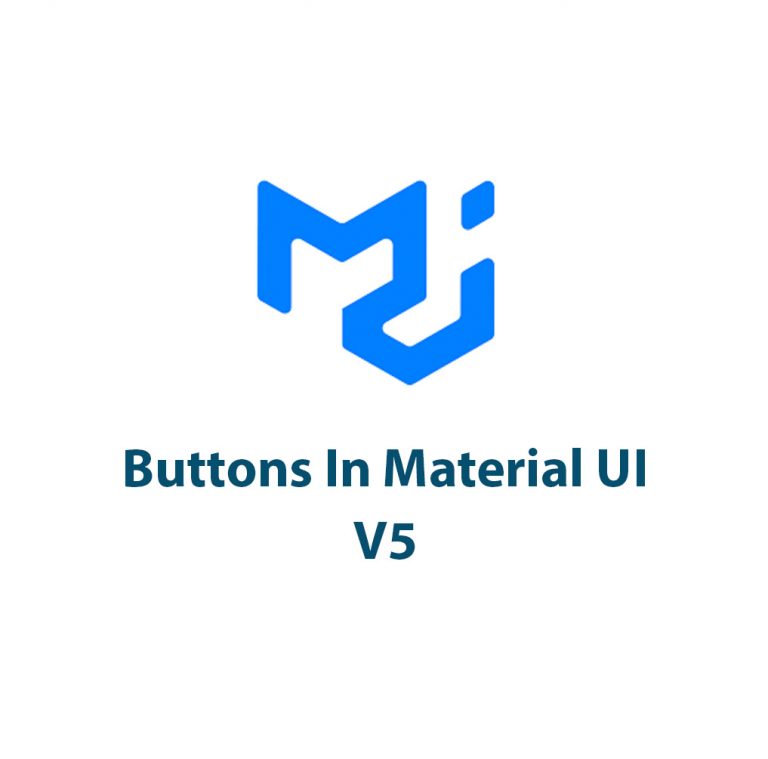
Material UI V5 Buttons
Introduction: If you’re familiar with Material UI, then you already know it is a popular React UI framework. It makes web development easier and faster for developers; this is the reason sites such as Harvard Business Review, Lyft, and Netflix use the framework for their web apps. Material UI v5.0 has recently been released, and […]
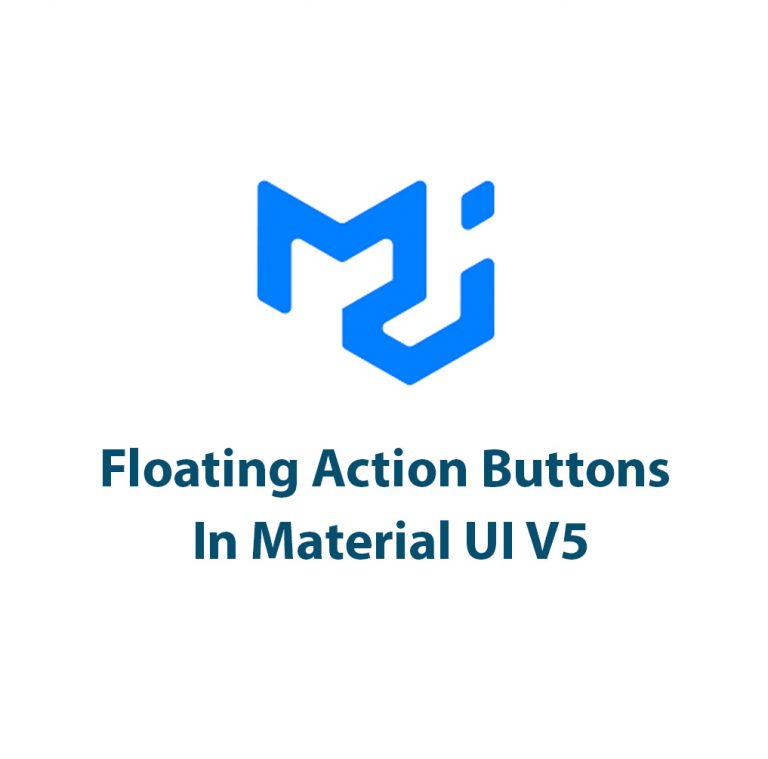
Floating Action Buttons In Material UI V5
Introduction Hey there fellow developers! Have you ever wanted to create a floating action button that seamlessly integrates with your project’s design? Well, look no further than Material UI V5 floating action buttons! Floating action buttons, or FABs, have become a popular design element in web design due to their ease of use and functionality. […]
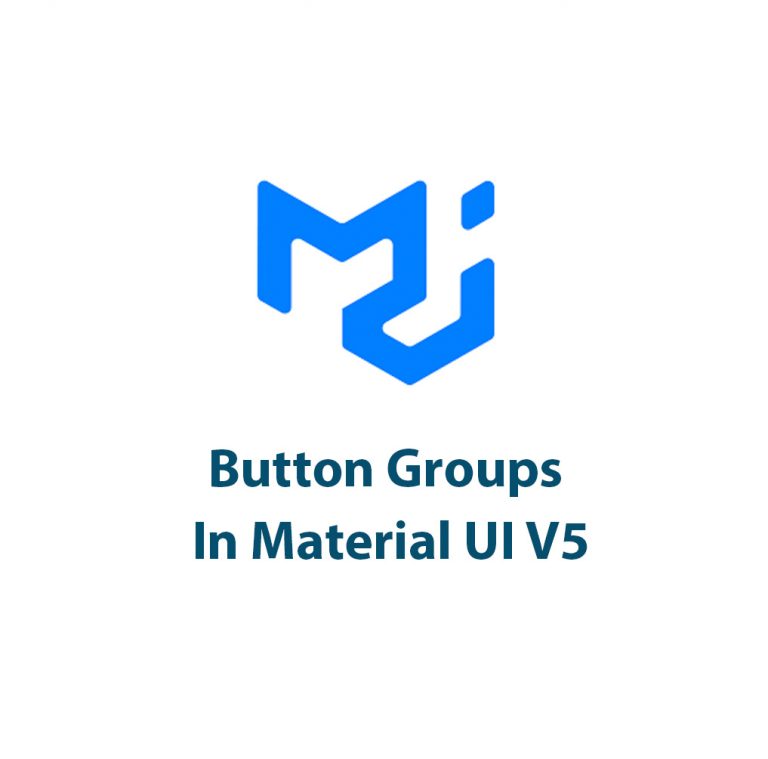
Button Groups In Material UI V5
Hey there! Welcome to my article all about button groups in Material UI V5. In this article, I’ll be giving you an in-depth insight into button groups, how they are used in UI designing, and how you can create them in Material UI V5. Introduction Before we dive into button groups, let’s start with some […]
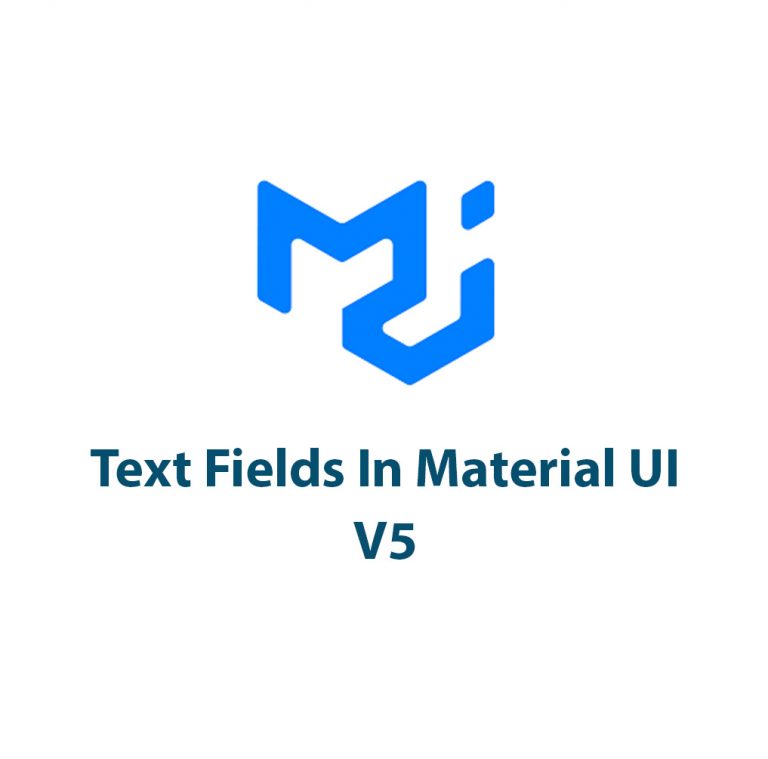
Text Fields In Material UI V5
I. Introduction As the popularity of Material UI as a user interface kit continues to rise, developers have found themselves working with text fields more often. Text fields are one of the most essential elements in any form and Material UI has made using them fun and straightforward.This article seeks to highlight the features, use, […]