Introduction
As a web developer, I’m always on the lookout for efficient ways to create stunning user interfaces. That’s why I’m excited to explore Material UI V5, a library of React components that make it easy to build beautiful web apps. And in this article, I’m going to focus on one essential element of any web app: the login form.
Login forms are the first point of interaction your users have with your website, and they’re critical in terms of usability and security. Material UI V5 makes it easy to build fantastic login forms that stand out and are easy to use. Let’s dive in!
Setting up Material UI V5
Let’s get started by installing the Material UI V5 package. We can do this by running the following command in our terminal.
npm install @mui/material@next @emotion/react @emotion/styled
This command installs the latest version of Material UI V5 along with Emotion for styling. We also need to import the Material UI components we’ll be using in our login form, so let’s do that in our React component file.
import {Box, Button, TextField, Typography} from '@mui/material';
Great! Now we’re ready to start building our login form.
Basic structure of a login form with Material UI V5
We’re going to build a basic login form using Material UI V5. In this form, we’ll have two input fields: one for the user’s email and another for the password. We’ll also add a submit button that will trigger a function to validate the form.
Here’s the code for the basic structure of the login form using Material UI V5:
const LoginForm = () => {
return (
<Box
sx={{
display: 'flex',
flexDirection: 'column',
alignItems: 'center',
mt: 8,
}}
>
<Typography component="h1" variant="h5">
Sign in
</Typography>
<Box component="form" noValidate sx={{ mt: 1 }}>
<TextField
margin="normal"
required
fullWidth
id="email"
label="Email Address"
name="email"
autoComplete="email"
autoFocus
/>
<TextField
margin="normal"
required
fullWidth
name="password"
label="Password"
type="password"
id="password"
autoComplete="current-password"
/>
<Button
type="submit"
fullWidth
variant="contained"
sx={{ mt: 3, mb: 2 }}
>
Sign In
</Button>
</Box>
</Box>
);
};
As you can see, we have used several Material UI components, including Typography, Box, TextField, and Button. We’ve also added some custom styling using the sx prop. The sx prop is a shorthand for applying style properties to the component.
Customization of login form with Material UI V5
Now that we have the basic structure of our login form, let’s customize it a bit. Material UI V5 comes with theming options, which make it easy to apply a consistent color scheme and typography to our app.
Here’s an example of how you can create a custom theme and apply it to the login form.
import {createTheme, ThemeProvider} from '@mui/material/styles';
const theme = createTheme({
palette: {
primary: {
main: '#2196f3',
},
secondary: {
main: '#f50057',
},
},
typography: {
fontFamily: ['Open Sans', 'sans-serif'].join(','),
h5: {
fontWeight: 600,
fontSize: '1.5rem',
},
},
});
const LoginForm = () => {
return (
<ThemeProvider theme={theme}>
{/* form code */}
</ThemeProvider>
);
};
In this example, we’ve created a custom theme with a primary color of #2196f3 and a secondary color of #f50057. We’ve also set the typography to use the Open Sans font and made the h5 component bold and increased the font size.
Adding advanced styling techniques to login form with Material UI V5
Now that we’ve applied a custom theme to our login form, let’s take it a step further by adding some more advanced styling techniques.
We’re going to add a background image to our login form and center the form itself. We’ll also add some custom styles to the text input fields to style them when they’re focused. Here’s the code for the updated login form:
import {makeStyles} from '@mui/styles';
const useStyles = makeStyles((theme) => ({
root: {
height: '100vh',
backgroundImage: `url(${process.env.PUBLIC_URL + '/img/login-background.jpg'})`,
backgroundSize: 'cover',
backgroundPosition: 'center',
},
paper: {
display: 'flex',
flexDirection: 'column',
alignItems: 'center',
height: '100vh',
justifyContent: 'center',
backgroundColor: 'rgba(255, 255, 255, 0.9)',
},
form: {
width: '100%', // Fix IE 11 issue.
marginTop: theme.spacing(1),
},
submit: {
margin: theme.spacing(3, 0, 2),
color: theme.palette.secondary.contrastText,
backgroundColor: theme.palette.secondary.main,
'&:hover': {
backgroundColor: theme.palette.secondary.dark,
},
},
input: {
'&:focus': {
borderColor: theme.palette.primary.main,
borderWidth: 2,
},
},
}));
const LoginForm = () => {
const classes = useStyles();
return (
<Box className={classes.root}>
<Box className={classes.paper}>
<Typography component="h1" variant="h5">
Sign in
</Typography>
<Box component="form" className={classes.form} noValidate>
<TextField
margin="normal"
required
fullWidth
id="email"
label="Email Address"
name="email"
autoComplete="email"
autoFocus
className={classes.input}
/>
<TextField
margin="normal"
required
fullWidth
name="password"
label="Password"
type="password"
id="password"
autoComplete="current-password"
className={classes.input}
/>
<Button
type="submit"
fullWidth
variant="contained"
className={classes.submit}
>
Sign In
</Button>
</Box>
</Box>
</Box>
);
};
We’ve used the makeStyles hook to create custom styles for our login form. The root class sets the height and the background image of the login form and centers it horizontally and vertically. The paper class is used to center the form elements vertically within the login form. The form class sets the width and margin of the form element, and the input class adds a custom focus style to the input fields.
Best practices for using Material UI V5 login forms
Now that we’ve built a beautiful login form using Material UI V5, let’s talk about some best practices for using it.
Accessibility considerations
Accessibility should always be a top priority when building web apps. Material UI V5 components come with built-in accessibility features that make it easier to ensure that your app is accessible to all users.
In the login form we built, we used the label prop in the TextField component to provide an accessible label for the input fields. We also set the required prop to true to indicate that the fields are required.
User experience improvements
In addition to accessibility, user experience is also critical in web development. Material UI V5 components come with intuitive user interface features that can help improve the user experience of your app.
For example, in our login form, we set the autoFocus prop to the email input field to automatically focus on the field when the page loads, making it easier for users to start typing right away. We also styled the input fields with an onFocus prop to help users visually distinguish which field they’re currently typing in.
Conclusion
Material UI V5 is a fantastic library for building web apps with beautiful, customizable user interfaces. In this article, we focused on building login forms with Material UI V5, and we saw how easy it is to create stunning login forms using Material UI V5 components.
We also explored some advanced styling techniques and best practices for accessibility and user experience. Hopefully, this article has given you a good starting point for building your own login forms with Material UI V5. Happy coding!
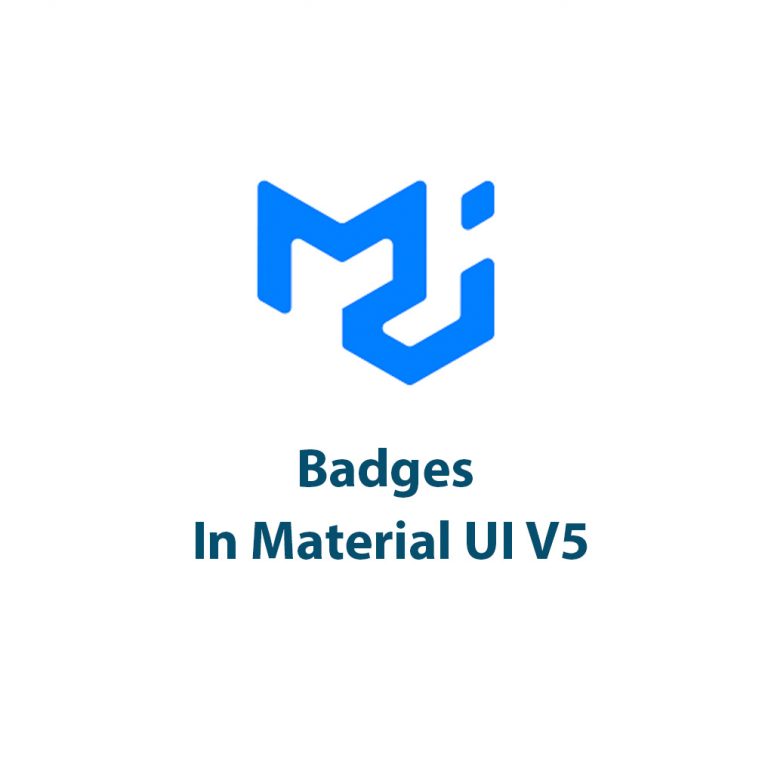
Badges In Material UI V5
As a web developer, I’ve always been fascinated by the small design elements that can make a big impact on the overall user experience of a website. One such element is the badge, often used to highlight new or important information to users. In the latest release of Material UI, version 5, badges have seen […]
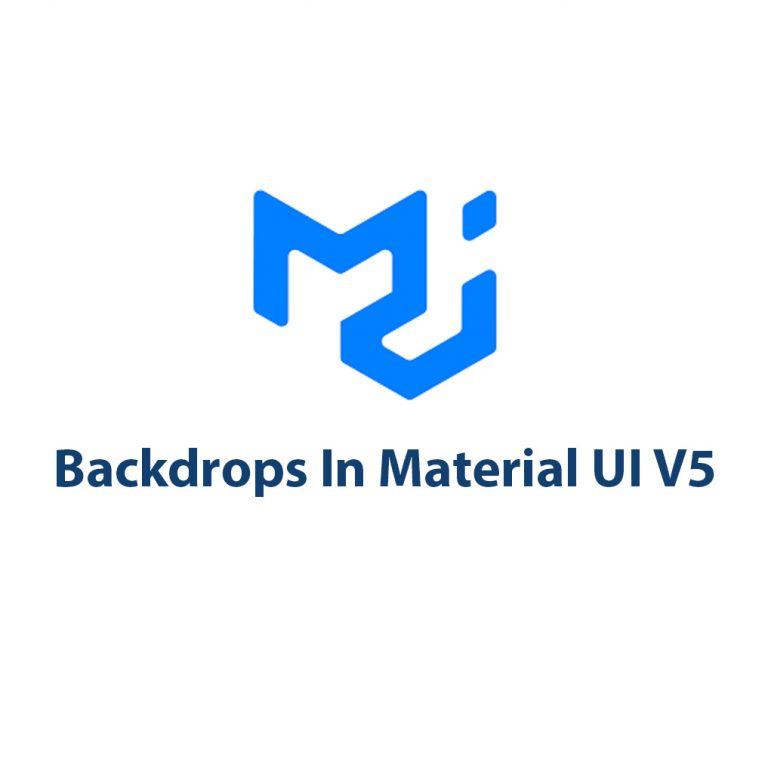
Backdrops In Material UI V5
As a developer, I’m always on the lookout for tools and frameworks that can make my job easier. That’s why I love Material UI. This popular React UI component library provides a wealth of customizable and responsive components that can be easily integrated into any project. One useful component of Material UI is the backdrop […]
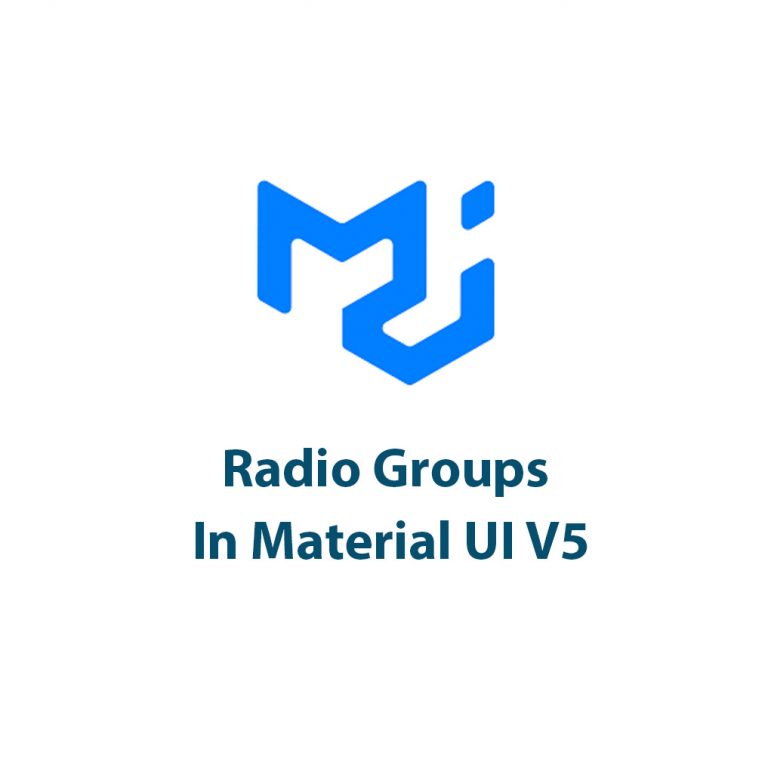
Radio Groups In Material UI V5
Introduction Hello everyone! Material UI has long been one of my favorite UI libraries, and with the recent release of version 5, I thought it would be a great time to explore one of its fundamental components: Radio Groups. In this article, we’ll dive into what Radio Groups are, how they can be used in […]
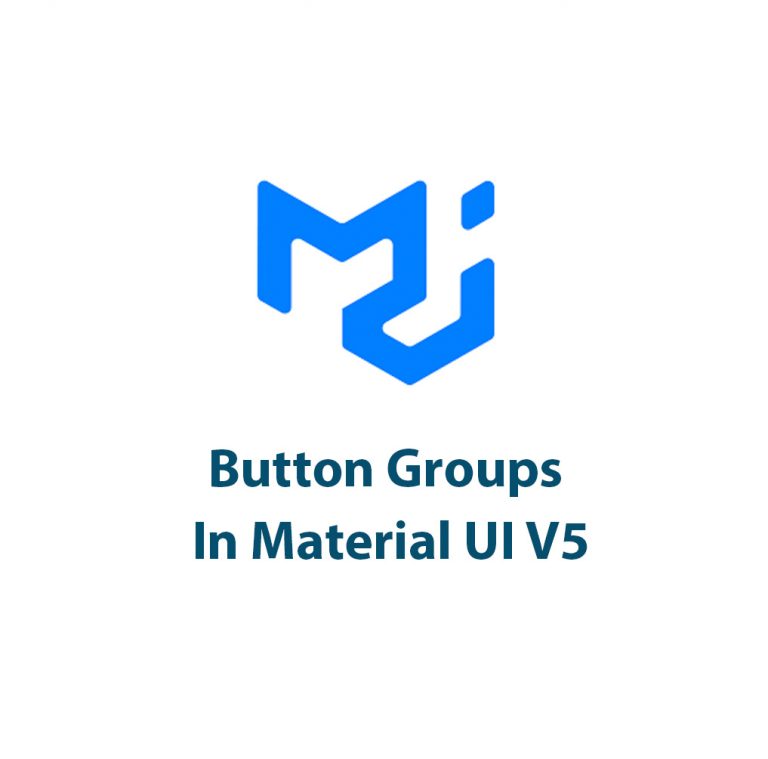
Button Groups In Material UI V5
Hey there! Welcome to my article all about button groups in Material UI V5. In this article, I’ll be giving you an in-depth insight into button groups, how they are used in UI designing, and how you can create them in Material UI V5. Introduction Before we dive into button groups, let’s start with some […]
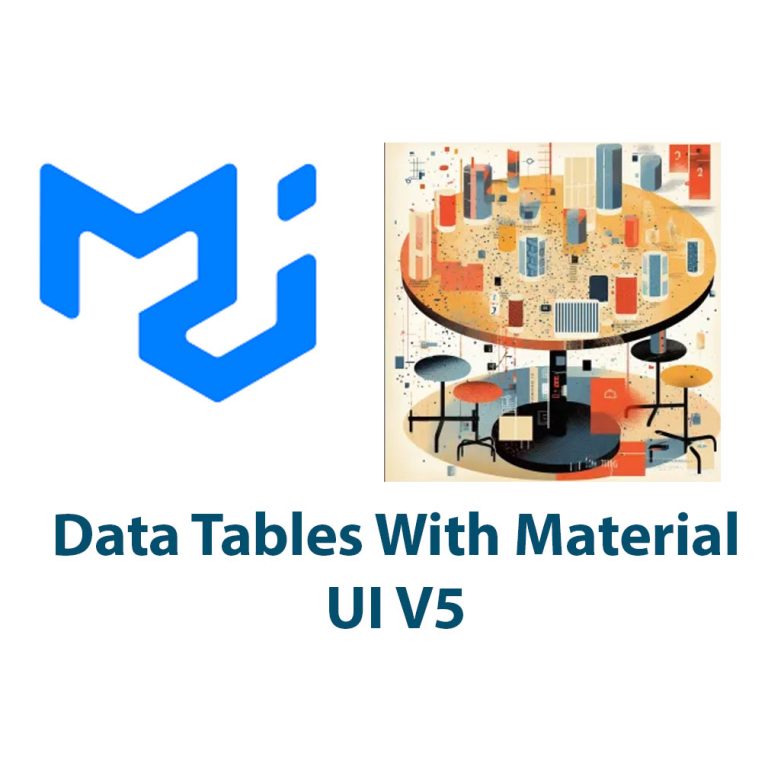
Datatables With Material V5
Introduction Material UI V5 is a popular, open-source library that provides pre-built UI components, themes, and styles for building user interfaces with React. Data tables are a common component used in web development to display data in a tabular format. In this article, I’ll show you how to use Material UI V5 to create stylish […]
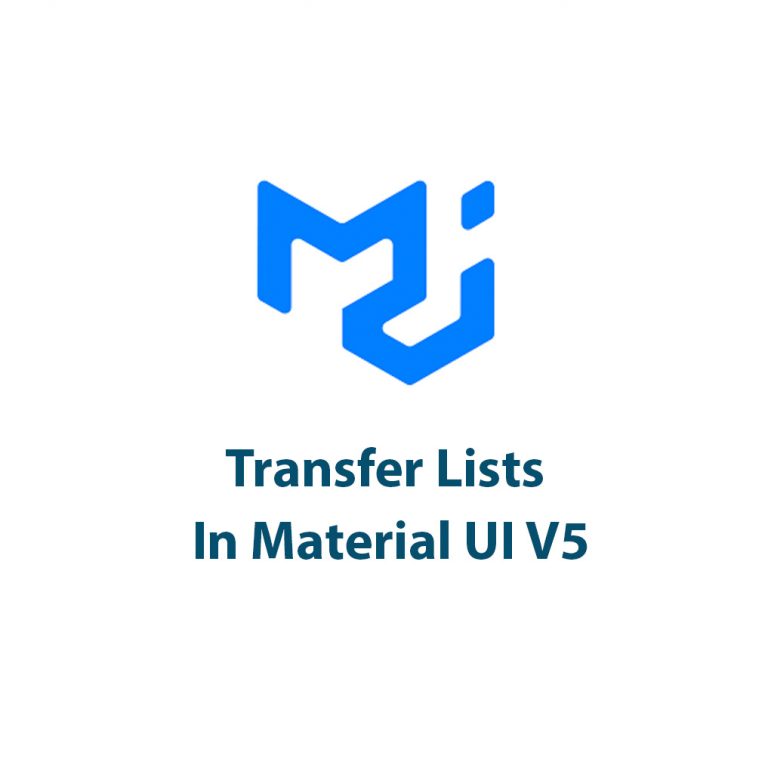
Transfer Lists In Material UI V5
Introduction I remember the first time I stumbled upon transfer lists while working on a project. I was perplexed by the concept, but as I delved deeper, I realized the tremendous benefits of using transfer lists in web development. With the release of Material UI v5, the developers have made it even easier to incorporate […]