As a web developer, I’ve always been fascinated by the small design elements that can make a big impact on the overall user experience of a website. One such element is the badge, often used to highlight new or important information to users. In the latest release of Material UI, version 5, badges have seen some significant changes and new features that have left me both perplexed and bursting with excitement. In this article, I’ll dive deep into the world of badges in Material UI V5, exploring the new features, benefits, and best practices for implementing badges effectively in your web projects.
What are Badges and Why Do We Use Them?
Badges are small design elements that can be used to alert users to new or important information. Typically a circle or square with a number or symbol inside, badges are used to indicate the status of an element, such as the number of unread messages in a user’s inbox or the availability of a new feature within an app. Badges are often placed on top of an icon or text as a subtle, but attention-grabbing design element that can improve the overall user experience.
Overview of Badges in Material UI V4
Before we dive into Material UI V5, it’s important to look at how badges were implemented in the previous version, V4. In V4, badges could be created using the Badge
component, which accepted several props to customize the look and behavior of the badge.
import Badge from '@mui/material/Badge';
<Badge badgeContent={4} color="primary">
<MailIcon />
</Badge>
This code would create a badge with a number “4” inside and a primary color. While the V4 implementation worked well enough, it had some limitations and drawbacks. For example, it did not allow for customizable shapes or sizes, making it difficult to fit badges into unique design situations. The V4 Badge
component was also criticized for its limited accessibility for visually impaired users.
What’s New in Material UI V5
Material UI V5 brings some significant updates and changes to badges that address the limitations of the previous version while adding new features and benefits. One major change is the introduction of the Badge
class, which uses the new styled
API to allow for greater customization options. The Badge
class also enables the use of different shapes, such as circles, dots, and squares, and can take multiple child elements.
Implementing Badges in Material UI V5
Now that we know the differences between V4 and V5 badges, let’s dive into the implementation process for V5. The code below shows how to use the new Badge
component in V5.
import { Badge } from '@mui/material';
<Badge badgeContent={4} color="primary">
<MailIcon />
</Badge>
The new Badge
component accepts several props to customize the badge’s look and behavior.
<Badge
badgeContent={4} // The content to be displayed inside the badge
color="primary" // The color of the badge
overlap="circle" // The overlap behavior of the badge
anchorOrigin={{vertical:'top', horizontal:'right'}} // The badge's anchor point
>
<MailIcon />
</Badge>
In Material UI V5, the Badge
component can take children as well as multiple child elements.
<Badge
badgeContent={4}
color="primary"
overlap="circle"
anchorOrigin={{vertical:'top', horizontal:'right'}}
>
<MailIcon />
<span>New</span>
</Badge>
To create a dot badge, simply add the variant="dot"
prop to the Badge
component.
<Badge variant="dot" color="primary">
<MailIcon />
</Badge>
Moreover, the badge’s content can be customized with additional text or icons using the _badgeContent
class.
<Badge
badgeContent={
<>
<NotificationsIcon />
<span className='badgeContent'>2</span>
</>
}
color="primary"
>
<MailIcon />
</Badge>
Badge Accessibility in Material UI V5
One of the criticisms of the V4 implementation of badges was its limited accessibility features. In V5, the Material UI team addressed this issue by ensuring that badges are fully accessible to visually impaired users. This involves using the aria-describedby
attribute to provide a short description of the badge content to screen readers.
<Badge
badgeContent={4}
aria-describedby='mail-badge'
>
<MailIcon />
</Badge>
Additionally, the Badge
component can be customized for accessibility using the badgeProps
prop. This allows developers to add custom accessibility properties to the badge.
<Badge
badgeContent={4}
color="primary"
badgeProps={{ 'aria-label': 'Mail' }}
>
<MailIcon />
</Badge>
Best Practices for Using Badges in Material UI V5
While badges can be an effective design element, it’s important to use them appropriately and not overuse them. Here are some best practices for using badges in Material UI V5:
- Use badges to highlight important elements, but don’t overuse them.
- Keep badge content short and concise to ensure maximum readability.
- Consider accessibility and use the appropriate attributes to ensure that the badge is fully accessible to visually impaired users.
- Customize the badge to fit your design needs, but ensure that it does not detract from the overall user experience.
Conclusion
Material UI V5 brings some exciting new changes to the world of badges. The new Badge
component offers more flexibility and customization options than the previous V4 implementation. With these new changes and accessibility features, badges in Material UI V5 are a powerful tool for improving the user experience. It’s important to use them appropriately and adhere to best practices to ensure that badges enhance the overall design of your web projects. With this newfound knowledge, I’m excited to explore more design possibilities using badges in Material UI V5.
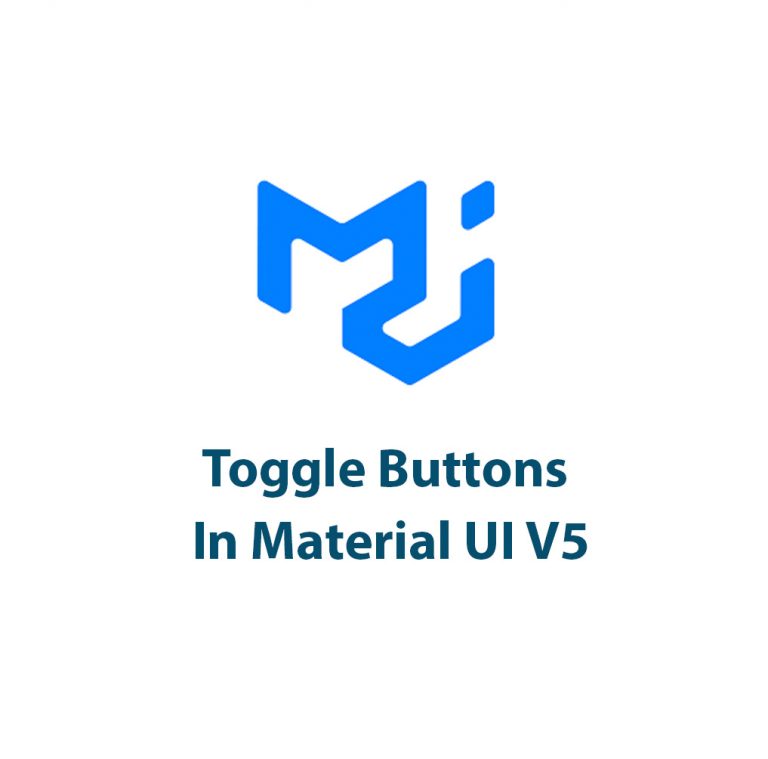
Toggle Buttons In Material UI V5
As a developer, I’ll be the first to admit that sometimes I find myself perplexed by certain UI design choices. As someone who spends a lot of time within the realm of code, I focus more on functionality and less on design. But the more I learn about UI design, the more I realize the […]
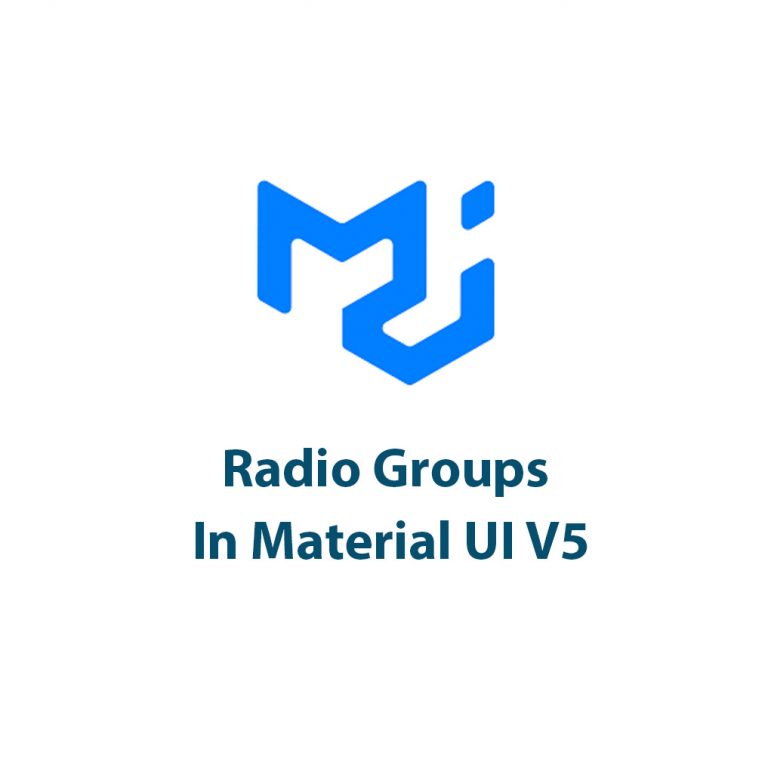
Radio Groups In Material UI V5
Introduction Hello everyone! Material UI has long been one of my favorite UI libraries, and with the recent release of version 5, I thought it would be a great time to explore one of its fundamental components: Radio Groups. In this article, we’ll dive into what Radio Groups are, how they can be used in […]
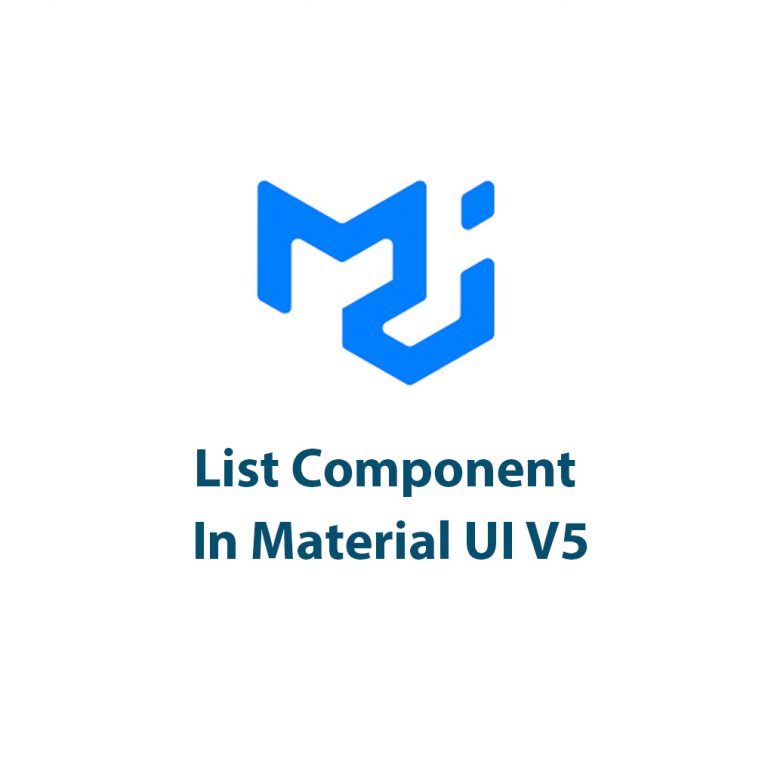
Lists In Material UI V5
Introduction As a UX designer and front-end developer, I’m always on the lookout for tools and libraries that make my job easier. When I first discovered Material UI, I was impressed by how it simplified UI development and improved the consistency of my designs. In this article, I want to focus specifically on lists in […]
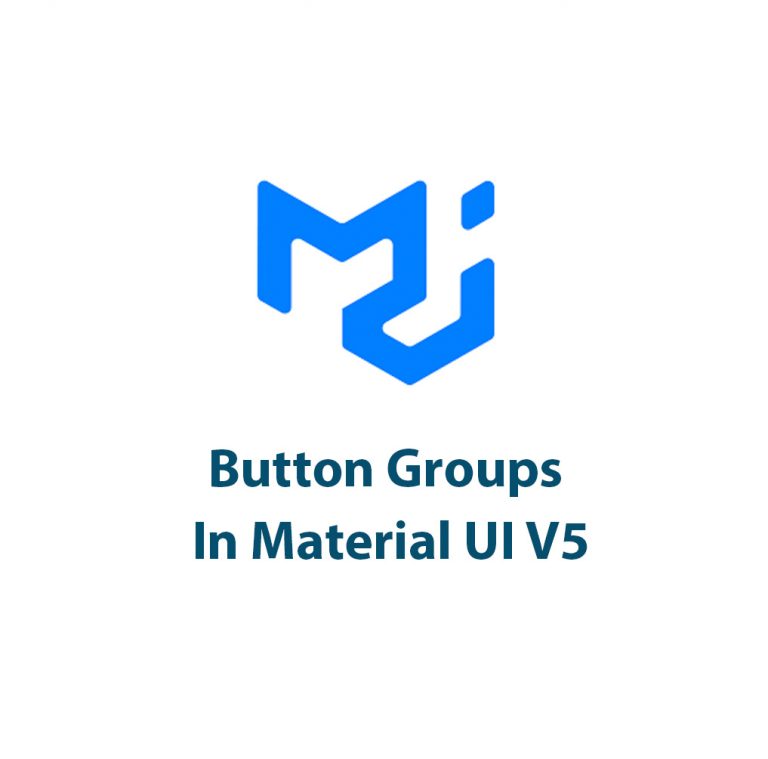
Button Groups In Material UI V5
Hey there! Welcome to my article all about button groups in Material UI V5. In this article, I’ll be giving you an in-depth insight into button groups, how they are used in UI designing, and how you can create them in Material UI V5. Introduction Before we dive into button groups, let’s start with some […]
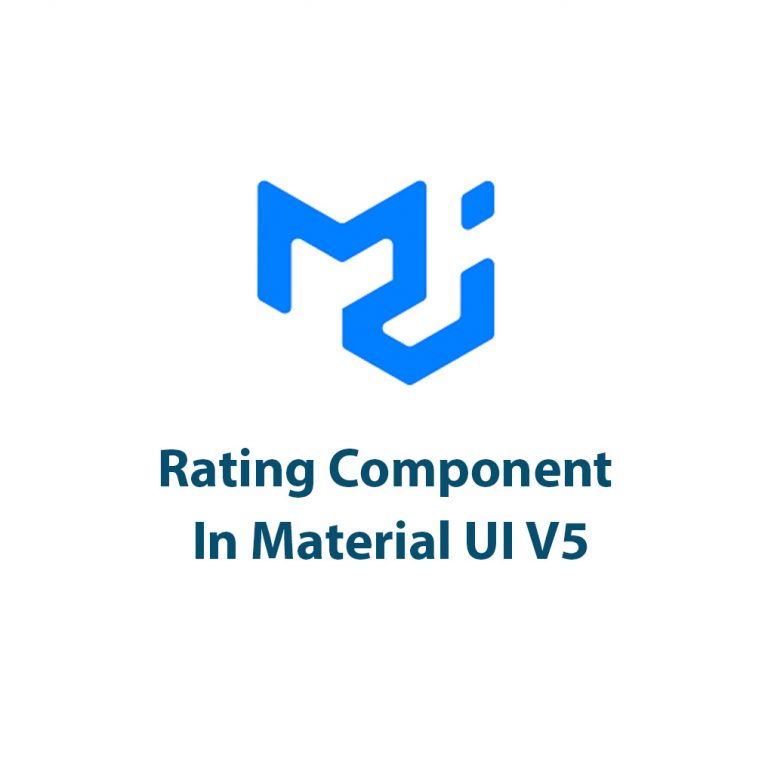
Rating Component In Material UI V5
Hello and welcome to this exciting article about the Rating Component in Material UI V5! As a web developer, I have come to really appreciate the simplicity and flexibility that this UI library provides, especially when it comes to components that add interactivity to user interfaces. In this article, I’m going to walk you through […]
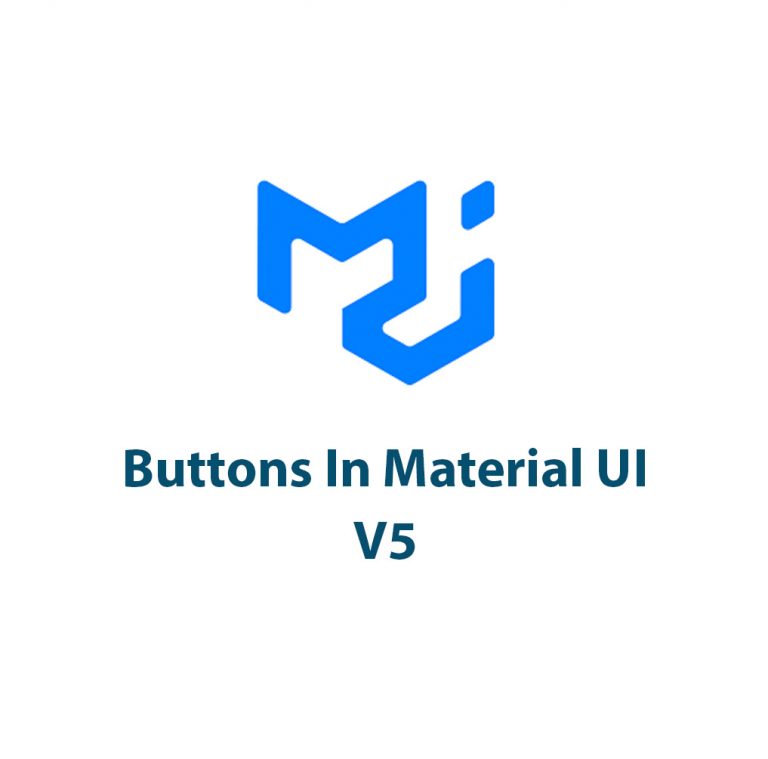
Material UI V5 Buttons
Introduction: If you’re familiar with Material UI, then you already know it is a popular React UI framework. It makes web development easier and faster for developers; this is the reason sites such as Harvard Business Review, Lyft, and Netflix use the framework for their web apps. Material UI v5.0 has recently been released, and […]