Introduction:
If you’re familiar with Material UI, then you already know it is a popular React UI framework. It makes web development easier and faster for developers; this is the reason sites such as Harvard Business Review, Lyft, and Netflix use the framework for their web apps. Material UI v5.0 has recently been released, and one of the core features that received a major update is the Button component. In this article, I will be discussing the updates made to the Button component in Material UI v5 and how to use it, including styling, accessibility, and real-world examples.
Button Components in Material UI V5:
In version 5.0, the Button component now contains four types: Contained Button, Outlined Button, Text Button, and Icon Button.
Contained Button: This button has a solid background color and usually represents the primary action of the page.
Outlined Button: This button has an outline but no background color. It’s suitable for secondary actions such as cancel, back, or reset buttons.
Text Button: This button has no background and only consists of text. It’s generally used for lower priority or tertiary actions.
Icon Button: This button consists only of an icon, and just like the Text button is used for tertiary actions.
Each button type can also have various properties such as, color, size, disabled, fullWidth, startIcon, endIcon, onClick among others.
Let’s create a sample Button component using the Contained Button type.
import React from 'react';
import Button from '@material-ui/core/Button';
export default function SampleButton() {
return (
<Button variant="contained" color="primary" onClick={() => console.log('button press')}>
Press Me
</Button>
);
}
Styling Buttons in Material UI V5:
One of the great features of Material UI is its easy-to-use styling system that supports CSS-in-JS styling. Since vanilla CSS can end up becoming a jumbled mess, we would want to avoid that. In Material UI, you can use the makeStyles
function to write your CSS-in-JS styles. With this function, you define your CSS rules, and the style is automatically applied to the component.
Here is an example of how the makeStyles
function can be used to style a button:
import React from 'react';
import Button from '@material-ui/core/Button';
import { makeStyles } from '@material-ui/core/styles';
const useStyles = makeStyles({
root: {
backgroundColor: 'purple',
'&:hover': {
backgroundColor: 'blue',
},
},
});
export default function StyledButton() {
const classes = useStyles();
return (
<Button className={classes.root} onClick={() => console.log('button press')}>
Press Me
</Button>
);
}
Accessibility Features of Buttons in Material UI V5:
It’s important to make sure your application is accessible to everyone, including those with disabilities. Material UI has accessibility features already built-in, making it easier to create accessible button components. Here are some of the properties to make your buttons accessible in Material UI:
- aria-label and aria-labelledby: This is used to provide an accessible label to the button for users who may not understand the text on the button.
- tabIndex: This assigns the tab order of the button.
- Focusable: This determines whether a button can be focused or not.
Here is an example of how to incorporate these features in a button component:
import React from 'react';
import Button from '@material-ui/core/Button';
import { makeStyles } from '@material-ui/core/styles';
const useStyles = makeStyles({
root: {
backgroundColor: 'purple',
'&:hover': {
backgroundColor: 'blue',
},
},
});
export default function AccessibleButton() {
const classes = useStyles();
return (
<Button
className={classes.root}
variant="contained"
color="primary"
focusable
tabIndex={0}
aria-label="Press me"
onClick={() => console.log('button press')}
>
Press Me
</Button>
);
}
Real World Examples:
To better understand how to use buttons in Material UI v5, let’s look at some real-world examples and how they can be implemented.
Example 1: Login Button
import React from 'react';
import Button from '@material-ui/core/Button';
import { makeStyles } from '@material-ui/core/styles';
const useStyles = makeStyles({
root: {
backgroundColor: 'purple',
'&:hover': {
backgroundColor: 'blue',
},
},
});
export default function LoginButton() {
const classes = useStyles();
return (
<Button
className={classes.root}
variant="contained"
color="primary"
focusable
tabIndex={0}
aria-label="Login"
onClick={() => console.log('button press')}
>
Login
</Button>
);
}
Example 2: Cart Button
import React from 'react';
import Button from '@material-ui/core/Button';
import { makeStyles } from '@material-ui/core/styles';
const useStyles = makeStyles({
root: {
backgroundColor: 'green',
'&:hover': {
backgroundColor: 'darkgreen',
},
},
});
export default function CartButton() {
const classes = useStyles();
return (
<Button
className={classes.root}
endIcon={<ShoppingCartIcon />}
variant="contained"
color="default"
tabIndex={0}
aria-label="Add to cart"
onClick={() => console.log('button press')}
>
Add to Cart
</Button>
);
}
Example 3: Sign-Up Button
import React from 'react';
import Button from '@material-ui/core/Button';
import { makeStyles } from '@material-ui/core/styles';
const useStyles = makeStyles({
root: {
backgroundColor: 'orange',
'&:hover': {
backgroundColor: 'red',
},
},
});
export default function SignupButton() {
const classes = useStyles();
return (
<Button
className={classes.root}
variant="contained"
color="secondary"
fullWidth
tabIndex={0}
aria-label="Sign up"
onClick={() => console.log('button press')}
>
Sign up
</Button>
);
}
Conclusion:
In conclusion, the Button component in Material UI receives a major update in version 5. It’s now easier to work with and also includes accessibility features. Material UI is a fantastic UI library that reduces the development time and has inbuilt features that work out of the box
such as responsiveness, customizability and easy-to-use APIs. By using these new Button components and implementing accessibility features, we can ensure that our web applications are more user-friendly and that everyone has an equal opportunity to use them. It’s also essential to incorporate the flexibility and customization of Material UI buttons to fit the unique needs of your application; with this in mind, you can create stunning and user-friendly buttons that will engage your users.
Material UI is a vast library, and the Button component is just one of the many components available. The community is continually contributing to the framework, and this means that there are plenty of resources to use, such as updates, documentations, and articles that will expand your knowledge about the Material UI framework.
Furthermore, the material UI’s support team is also active on Github, and they release regular updates and patches to fix any bugs and problems related to the framework. Hence, Material UI holds a strong future for the web development industry, and incorporating its components, including the Buttons component, can prove to be a game-changer for developers and designers alike.
In conclusion, Material UI V5’s Button component is an essential tool to create elegant and user-friendly button designs for web applications. It includes accessibility features and CSS-in-JS styling, making it easy to customize and fit to the unique needs of your application. The updated version 5 of the component offers designers and developers a better chance to achieve unique designs for their applications quickly. By utilizing the Button component, developers can create buttons that enhance the user experience significantly. And above all, with a willingness to experiment and dive into the bug community, developers can continually explore new and creative ways to enhance their applications and the user experience.
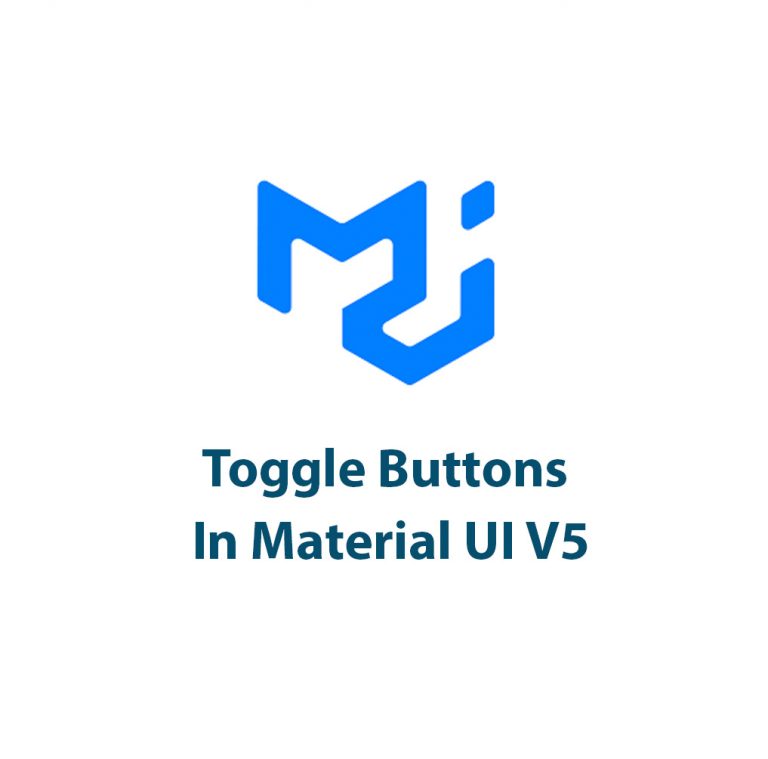
Toggle Buttons In Material UI V5
As a developer, I’ll be the first to admit that sometimes I find myself perplexed by certain UI design choices. As someone who spends a lot of time within the realm of code, I focus more on functionality and less on design. But the more I learn about UI design, the more I realize the […]
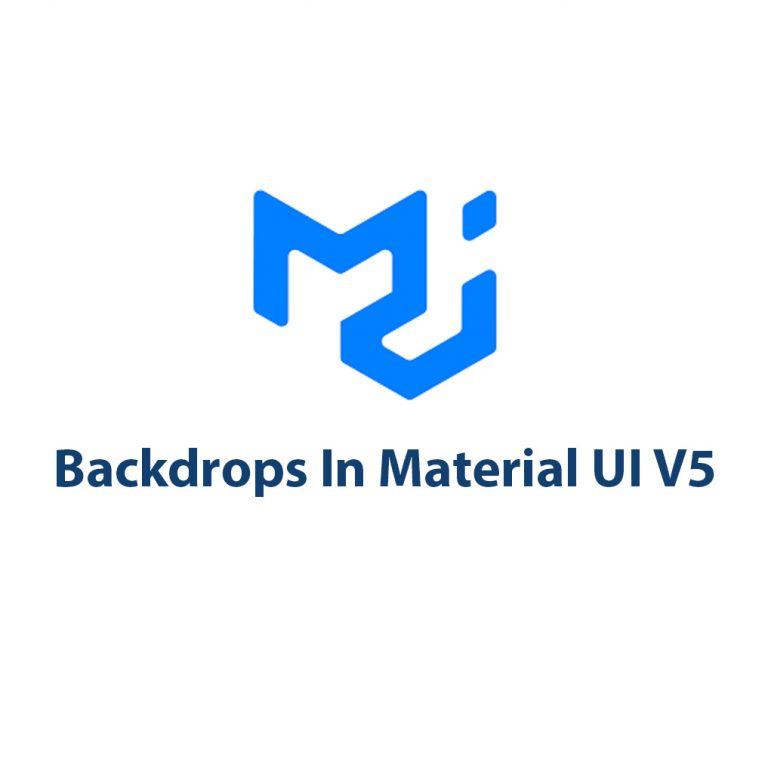
Backdrops In Material UI V5
As a developer, I’m always on the lookout for tools and frameworks that can make my job easier. That’s why I love Material UI. This popular React UI component library provides a wealth of customizable and responsive components that can be easily integrated into any project. One useful component of Material UI is the backdrop […]
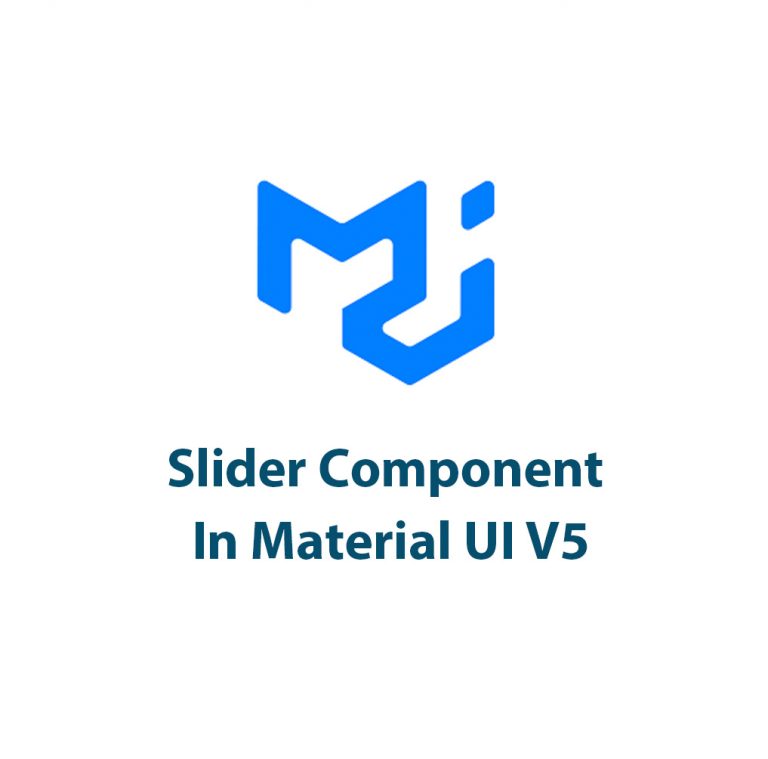
Slider Component In Material UI V5
Introduction Hey, everyone! If you’ve been following Material UI, you’re probably aware of how frequently this UI kit is updated. Recently, they released the version V5, which came with lots of new features and updates. One of the components that received great attention is the slider component. In this article, we’ll discuss everything you need […]
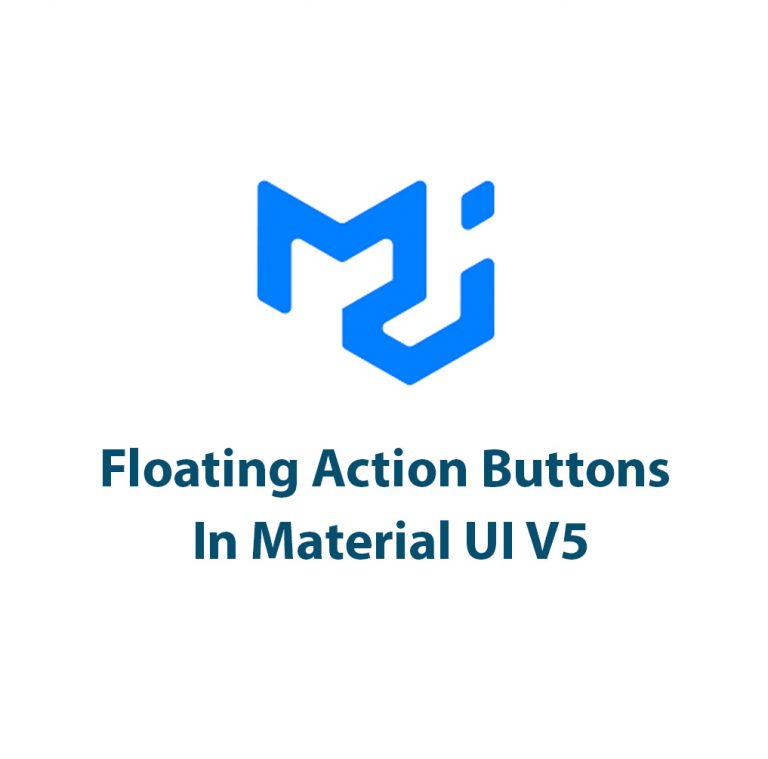
Floating Action Buttons In Material UI V5
Introduction Hey there fellow developers! Have you ever wanted to create a floating action button that seamlessly integrates with your project’s design? Well, look no further than Material UI V5 floating action buttons! Floating action buttons, or FABs, have become a popular design element in web design due to their ease of use and functionality. […]
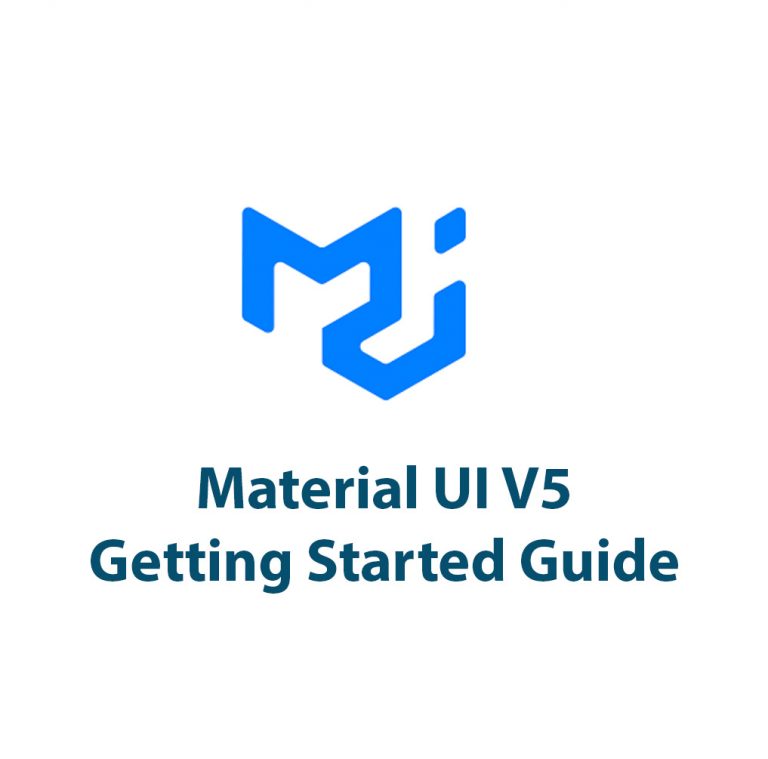
Material UI V5: Getting Started Guide
Introduction Hello, fellow online professionals! Are you prepared to explore the fascinating Material UI V5 world? You’ve come to the correct place if you’re trying to improve the appearance and feel of your online applications. The powerful framework that implements Google’s Material Design principles, Material UI V5, will be demonstrated to you step-by-step in this […]
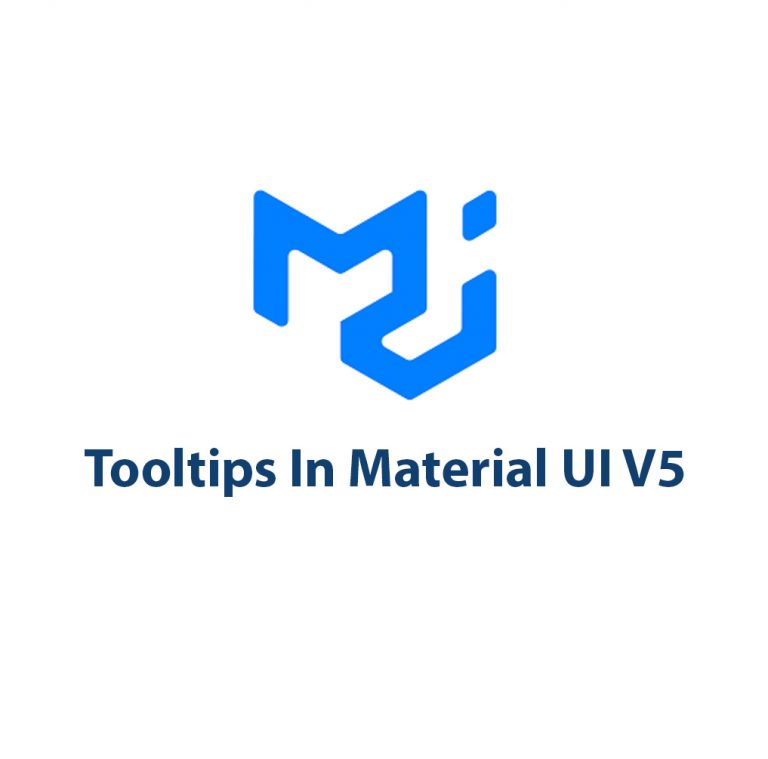
Tooltips in Material UI V5: A Guide To Dynamic UI Elements
Introduction As a web developer, I know that one of the most important things to consider when building a website or web application is how the user interface (UI) will interact with the user. A good UI design should be both intuitive and dynamic – allowing the user to perform actions with ease. And one […]