Introduction
I remember the first time I stumbled upon transfer lists while working on a project. I was perplexed by the concept, but as I delved deeper, I realized the tremendous benefits of using transfer lists in web development.
With the release of Material UI v5, the developers have made it even easier to incorporate transfer lists into web applications. Transfer lists are a simple yet sophisticated way to organize and manage data, providing both easy transfer between lists and customizable design options.
In this article, I will introduce you to the world of transfer lists in Material UI v5, guiding you through setting up transfer list components, adding data, customizing the list design, and much more. We will explore various examples of using transfer lists with code snippets to help you get started.
So buckle up, and let’s dive straight into the world of transfer lists in Material UI V5.
Benefits of Using Transfer Lists in Material UI V5
Before we delve into how to use transfer lists in Material UI v5, let’s talk about the benefits of using this feature in web applications.
- Easy Data Transfer between Lists
Transfer lists enable easy transfer of data between lists, helping web developers improve user experience. For instance, a user may be required to move items from one list to another list in a shopping cart application. Transfer lists streamline the data transfer experience, making it a seamless and smooth process for the users.
- Better Organization and Management of Data
Transfer lists are an excellent tool for organizing and managing data. They allow web developers to separate data into different lists based on specific characteristics. Users can then move items within these lists without losing track of the data or its purpose.
- Customizable Design Options
Transfer lists’ flexibility and customization options make them great for web developers who want to create custom lists that fit their application’s look and feel. This feature allows developers to set up transfer lists that match their applications’ design and use cases.
- Improved User Experience
For users, transfer lists improve the user experience by simplifying data transfer, making it easy for them to manage multiple lists of data. As users interact with the lists, they can easily customize, sort, and filter the data.
Now that we’ve established the general benefits of using transfer lists let’s dive into precisely how we can use them in our projects.
How to Use Transfer Lists in Material UI V5
To use transfer lists in Material UI V5, you only need a basic understanding of React. So, if you are new to React, I would recommend familiarizing yourself with React basics before jumping into transfer lists.
Here is what you need to do to set up transfer lists in Material UI v5:
- Installing Material UI v5
The first step is to include Material UI v5 in your project. You can install Material UI v5 from npm:
npm install @mui/material @emotion/react @emotion/styled
- Setting up Transfer List Components
Next, you need to create TransferList components and provide them with the right props. TransferList
is a Material UI component that renders two vertical lists and provides a way to transfer items between them.
Here is an example of how you can set up transfer lists in Material UI v5:
import { useState } from "react";
import { Box, List, ListItem, ListItemText } from "@mui/material";
import { TransferList } from "./TransferList";
function App() {
const [left, setLeft] = useState([
{ id: 1, name: "Item 1" },
{ id: 2, name: "Item 2" },
{ id: 3, name: "Item 3" },
]);
const [right, setRight] = useState([
{ id: 4, name: "Item 4" },
{ id: 5, name: "Item 5" },
]);
const handleOnChange = (items) => {
setLeft(items.left);
setRight(items.right);
};
return (
<Box sx={{ display: "flex", justifyContent: "center" }}>
<TransferList
left={
<List dense>
{left.map((item) => (
<ListItem key={item.id}>
<ListItemText primary={item.name} />
</ListItem>
))}
</List>
}
right={
<List dense>
{right.map((item) => (
<ListItem key={item.id}>
<ListItemText primary={item.name} />
</ListItem>
))}
</List>
}
onChange={handleOnChange}
/>
</Box>
);
}
In the code snippet above, we import TransferList, List, ListItem, and ListItemText from Material UI v5. We then create two arrays, left
and right
, representing our two transfer lists.
Our App
component renders one Box
component that centers the TransferList
component on the screen. The left and right lists are passed as props to the TransferList
component.
Note that we also provide the onChange
prop and pass a handler function that updates the left and right arrays to match the current state of the TransferList
component.
When you run this code, you will see two transfer lists with a button that enables movement of data between them.
- Adding Data to the Transfer Lists
The transfer lists we created above have hardcoded data. However, you can add data dynamically to the transfer lists to enable more dynamic usage.
Here’s an example of how to add data dynamically to transfer lists:
import { useState } from "react";
import { Box, List, ListItem, ListItemText } from "@mui/material";
import { TransferList } from "./TransferList";
function App() {
const [left, setLeft] = useState([]);
const [right, setRight] = useState([]);
const initialValues = [
{ id: 1, name: "Item 1" },
{ id: 2, name: "Item 2" },
{ id: 3, name: "Item 3" },
{ id: 4, name: "Item 4" },
{ id: 5, name: "Item 5" },
];
useEffect(() => {
setLeft(initialValues);
}, []);
const handleOnChange = (items) => {
setLeft(items.left);
setRight(items.right);
};
return (
<Box sx={{ display: "flex", justifyContent: "center" }}>
<TransferList
left={
<List dense>
{left.map((item) => (
<ListItem key={item.id}>
<ListItemText primary={item.name}</ListItem>
))}
</List>
}
right={
<List dense>
{right.map((item) => (
<ListItem key={item.id}>
<ListItemText primary={item.name} />
</ListItem>
))}
</List>
}
onChange={handleOnChange}
/>
</Box>
);
}
In this example, we use the useEffect
hook to set left
to the initial state with our initialValues
array. We then handle onChange
just as in the first example to update the left and right arrays.
Now, the transfer lists have dynamically added data.
- Customizing Transfer List Design
Material UI v5 provides several options to customize the transfer list design and behavior.
Here are some things you can customize:
- List item text
- List item background-color
- List item color
- List item active background-color
- List item active color
- List item hover background-color
- List item hover color
Below is an example of how to customize the transfer list design:
import { useState } from "react";
import { Box, List, ListItem, ListItemText } from "@mui/material";
import { TransferList } from "./TransferList";
function App() {
const [left, setLeft] = useState([
{ id: 1, name: "Item 1" },
{ id: 2, name: "Item 2" },
{ id: 3, name: "Item 3" },
]);
const [right, setRight] = useState([
{ id: 4, name: "Item 4" },
{ id: 5, name: "Item 5" },
]);
const handleOnChange = (items) => {
setLeft(items.left);
setRight(items.right);
};
const listItemStyles = {
"&:hover": {
backgroundColor: "#e1bee7",
color: "#6a1b9a",
},
"&$selected": {
backgroundColor: "#f8bbd0",
color: "#880e4f",
},
"&$selected:hover": {
backgroundColor: "#f48fb1",
},
selected: {},
};
return (
<Box sx={{ display: "flex", justifyContent: "center" }}>
<TransferList
left={
<List dense>
{left.map((item) => (
<ListItem
key={item.id}
classes={listItemStyles}
button
disableRipple
>
<ListItemText primary={item.name} />
</ListItem>
))}
</List>
}
right={
<List dense>
{right.map((item) => (
<ListItem
key={item.id}
classes={listItemStyles}
button
disableRipple
>
<ListItemText primary={item.name} />
</ListItem>
))}
</List>
}
onChange={handleOnChange}
/>
</Box>
);
}
In this example, we customize the list item hover
and selected styles. We set the backgroundColor
to a pinkish hue and the color to a purple tone during hover
and selected states.
There are extensive options available to customize Material UI Transfer List through the classes
prop with existing Material UI styles or with additional CSS styles.
Examples of Transfer Lists in Material UI V5
Now that we know how to set up transfer lists, customize their design and add data, let’s take a look at some example scenarios where transfer lists can be helpful in web development.
- Basic Transfer List Example
A basic transfer list example is a simple and straightforward way to use transfer lists. In this example, users can move items between two transfer lists.
import { useState } from "react";
import { Box, List, ListItem, ListItemText } from "@mui/material";
import { TransferList } from "./TransferList";
function App() {
const [left, setLeft] = useState([
{ id: 1, name: "Item 1" },
{ id: 2, name: "Item 2" },
{ id: 3, name: "Item 3" },
]);
const [right, setRight] = useState([
{ id: 4, name: "Item 4" },
{ id: 5, name: "Item 5" },
]);
const handleOnChange = (items) => {
setLeft(items.left);
setRight(items.right);
};
return (
<Box sx={{ display: "flex", justifyContent: "center" }}>
<TransferList
left={
<List dense>
{left.map((item) => (
<ListItem key={item.id}>
<ListItemText primary={item.name} />
</ListItem>
))}
</List>
}
right={
<List dense>
{right.map((item) => (
<ListItem key={item.id}>
<ListItemText primary={item.name} />
</ListItem>
))}
</List>
}
onChange={handleOnChange}
/>
</Box>
);
}
- Customized Transfer List Example
The customized transfer list example allows you to customize the design of your transfer lists, as you saw in the previous example. Here, we have set custom background colors and text colors for our transfer lists, which will make them more visually appealing and easier to use.
import { useState } from "react";
import { Box, List, ListItem, ListItemText } from "@mui/material";
import { TransferList } from "./TransferList";
function App() {
const [left, setLeft] = useState([
{ id: 1, name: "Item 1" },
{ id: 2, name: "Item 2" },
{ id: 3, name: "Item 3" },
]);
const [right, setRight] = useState([
{ id: 4, name: "Item 4" },
{ id: 5, name: "Item 5" },
]);
const handleOnChange = (items) => {
setLeft(items.left);
setRight(items.right);
};
const listItemStyles = {
"&:hover": {
backgroundColor: "#4caf50",
color: "#ffffff",
},
"&$selected": {
backgroundColor: "#3f51b5",
color: "#ffffff",
},
"&$selected:hover": {
backgroundColor: "#5c6bc0",
},
selected: {},
};
return (
<Box sx={{ display: "flex", justifyContent: "center" }}>
<TransferList
left={
<List dense>
{left.map((item) => (
<ListItem
key={item.id}
classes={listItemStyles}
button
disableRipple
>
<ListItemText primary={item.name} />
</ListItem>
))}
</List>
}
right={
<List dense>
{right.map((item) => (
<ListItem
key={item.id}
classes={listItemStyles}
button
disableRipple
>
<ListItemText primary={item.name} />
</ListItem>
))}
</List>
}
onChange={handleOnChange}
/>
</Box>
);
}
- Transfer List with API Data Example
The transfer list with API data example is useful when you have large amounts of data that need to be displayed in transfer lists. With the useEffect
hook, we can retrieve data from an API and display that data in our transfer lists.
import { useState, useEffect } from "react";
import { Box, List, ListItem, ListItemText } from "@mui/material";
import { TransferList } from "./TransferList";
function App() {
const [left, setLeft] = useState([]);
const [right, setRight] = useState([]);
const [loading, setLoading] = useState(false);
useEffect(() => {
setLoading(true);
fetch("https://jsonplaceholder.typicode.com/users")
.then((res) => res.json())
.then((data) => {
setLeft(data.map((item) => ({ id: item.id, name: item.name })));
setLoading(false);
})
.catch((err) => console.log(err));
}, []);
const handleOnChange = (items) => {
setLeft(items.left);
setRight(items.right);
};
return (
<Box sx={{ display: "flex", justifyContent: "center" }}>
{loading ? (
<p>Loading...</p>
) : (
<TransferList
left={
<List dense>
{left.map((item) => (
<ListItem key={item.id}>
<ListItemText primary={item.name} />
</ListItem>
))}
</List>
}
right={
<List dense>
{right.map((item) => (
<ListItem key={item.id}>
<ListItemText primary={item.name} />
</ListItem>
))}
</List>
}
onChange={handleOnChange}
/>
)}
</Box>
);
}
Conclusion
In conclusion, Transfer List in Material UI v5 is an amazing and flexible tool to work with lists. With just a few lines of code, it allows you to transfer data across multiple lists. You can also easily customize the design and behavior of your transfer lists. By understanding how to set up transfer lists, add data, and customize the design, you can create powerful and elegant UIs that make using your app a joy.
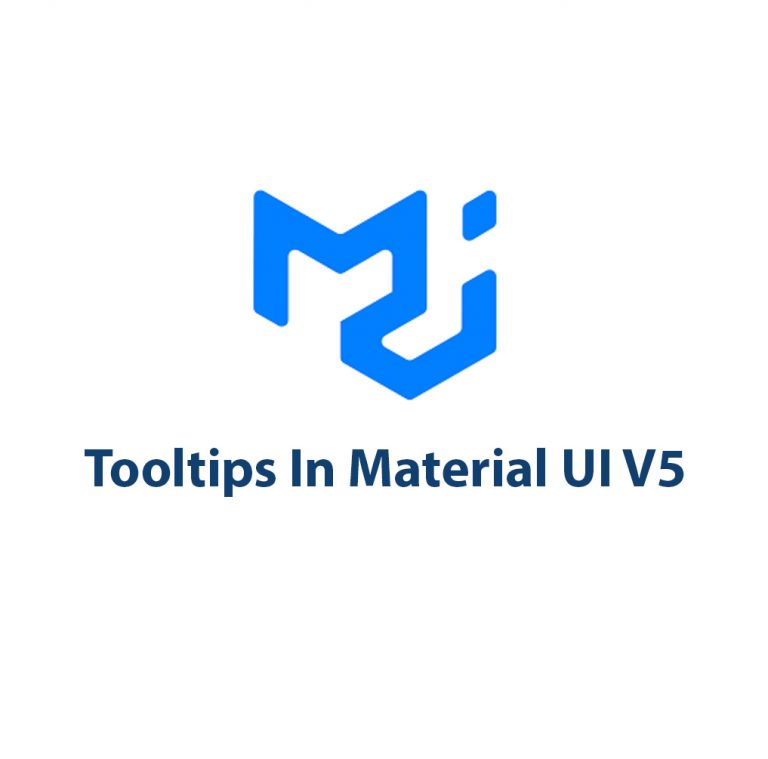
Tooltips in Material UI V5: A Guide To Dynamic UI Elements
Introduction As a web developer, I know that one of the most important things to consider when building a website or web application is how the user interface (UI) will interact with the user. A good UI design should be both intuitive and dynamic – allowing the user to perform actions with ease. And one […]
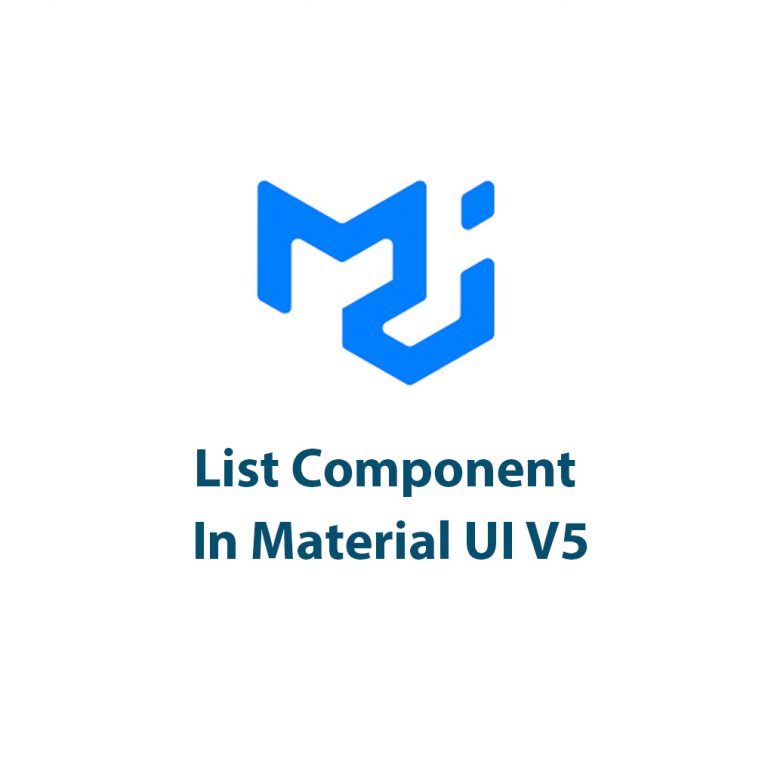
Lists In Material UI V5
Introduction As a UX designer and front-end developer, I’m always on the lookout for tools and libraries that make my job easier. When I first discovered Material UI, I was impressed by how it simplified UI development and improved the consistency of my designs. In this article, I want to focus specifically on lists in […]
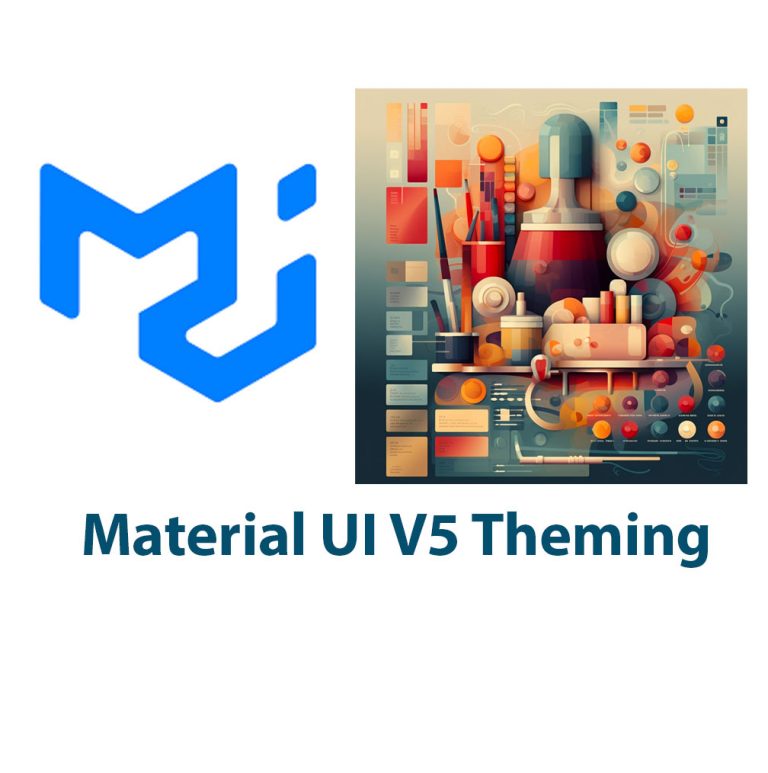
Material UI V5 Theming
Introduction: Popular React UI framework Material UI provides a large selection of pre-built components to easily build responsive user interfaces. The library’s design approach, which is based on Google’s Material Design principles, has helped it become more well-liked among developers. The design framework of Material UI is crucial to the creation of user interfaces since […]
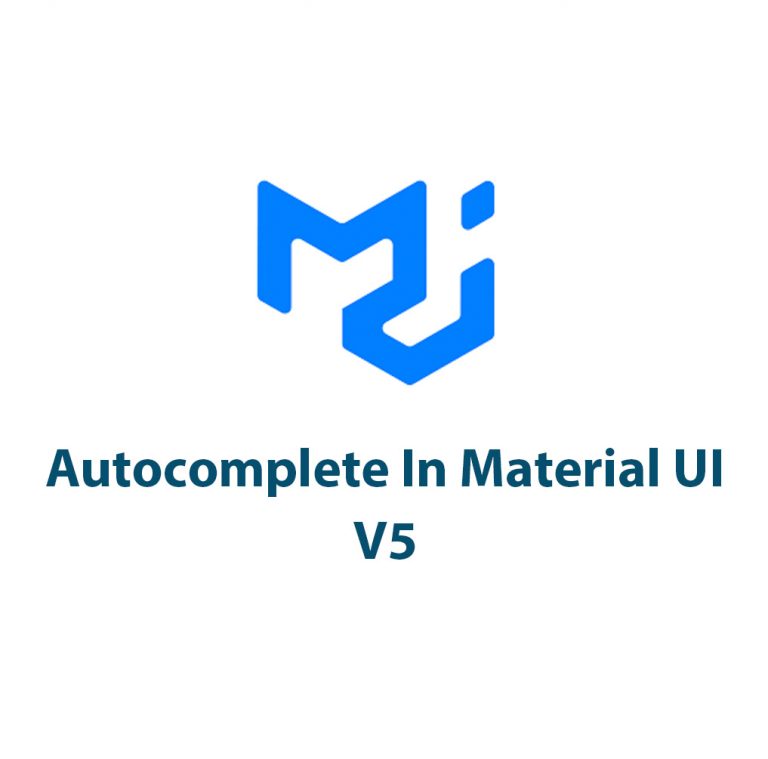
Autocomplete In Material UI V5
Introduction Hello everyone, today I am going to talk about one of the most useful components in Material UI V5 – Autocomplete. Material UI is a popular React UI framework that provides a wide range of pre-designed components to make the development of complex user interfaces simpler. Autocomplete is an intuitive component that provides suggestions […]
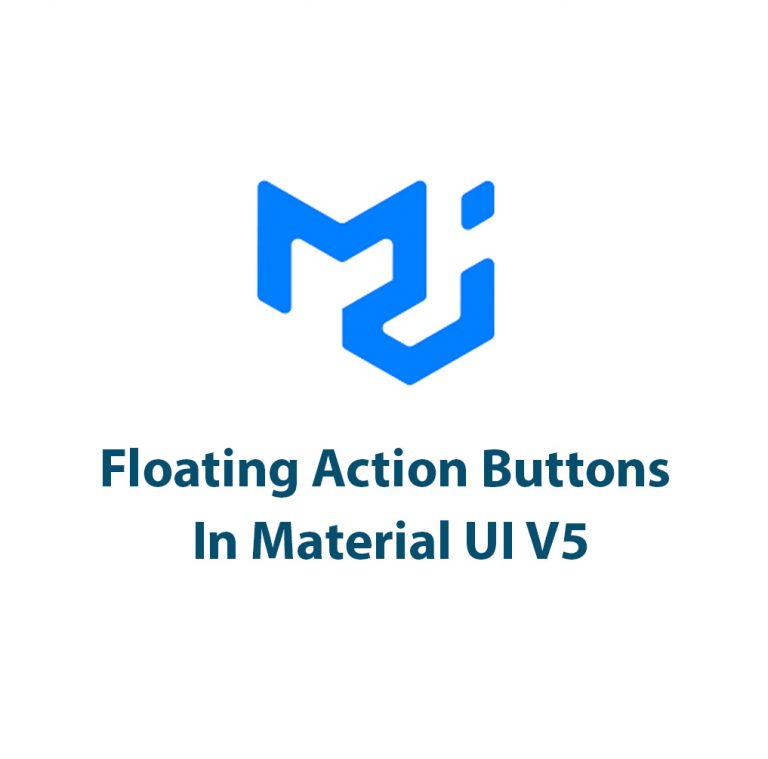
Floating Action Buttons In Material UI V5
Introduction Hey there fellow developers! Have you ever wanted to create a floating action button that seamlessly integrates with your project’s design? Well, look no further than Material UI V5 floating action buttons! Floating action buttons, or FABs, have become a popular design element in web design due to their ease of use and functionality. […]
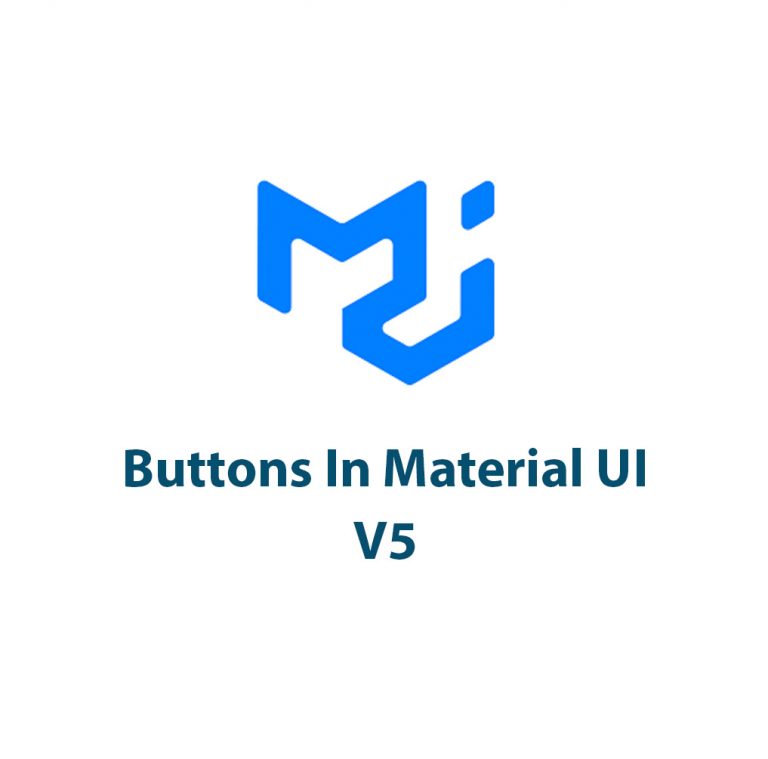
Material UI V5 Buttons
Introduction: If you’re familiar with Material UI, then you already know it is a popular React UI framework. It makes web development easier and faster for developers; this is the reason sites such as Harvard Business Review, Lyft, and Netflix use the framework for their web apps. Material UI v5.0 has recently been released, and […]