Debugger In Node JS: Getting a Deeper Understanding
One thing we might all have in common as developers in the always changing tech industry is the ongoing need to come up with new and better approaches to debug our code. Since troubleshooting is a crucial step in the development process, we must be well-equipped with the appropriate equipment to complete the task more quickly and effectively.
Since I’ve been working with Node.js for a while, the debugger has been one of the things that has made debugging a lot simpler for me. In order to help you get started using the debugger in Node.js, I want to walk you through my experience with it, its fundamental and advanced approaches, common problems, and some real-world examples.
Setting Up The Environment
Before delving into the intricacies of debugging with Node.js, you need to set up your environment. The first step is to install Node.js. You can download the latest version of Node.js from the official website. Once done, you can check that Node and NPM (Node Package Manager) have been installed successfully using the following commands:
node -v
npm -v
If the Node and NPM versions are displayed in the terminal, they have been installed successfully. Node.js comes with a built-in debugging tool, but for more advanced features, we need an additional tool. Here are some of them:
- Visual Studio Code Debugger
- Chrome Developer Tools
- Node Inspector
For this article, we will be using the Visual Studio Code debugger. To install it, download Visual Studio Code from its official website. Once installed, launch Visual Studio Code, open a Node.js project, and navigate to the Debug tab in Visual Studio Code. From there, select the “Configure or fix ‘launch.json'” option. The “launch.json” file has a JSON configuration that contains the settings you need to run your applications.
Understanding The Debugger
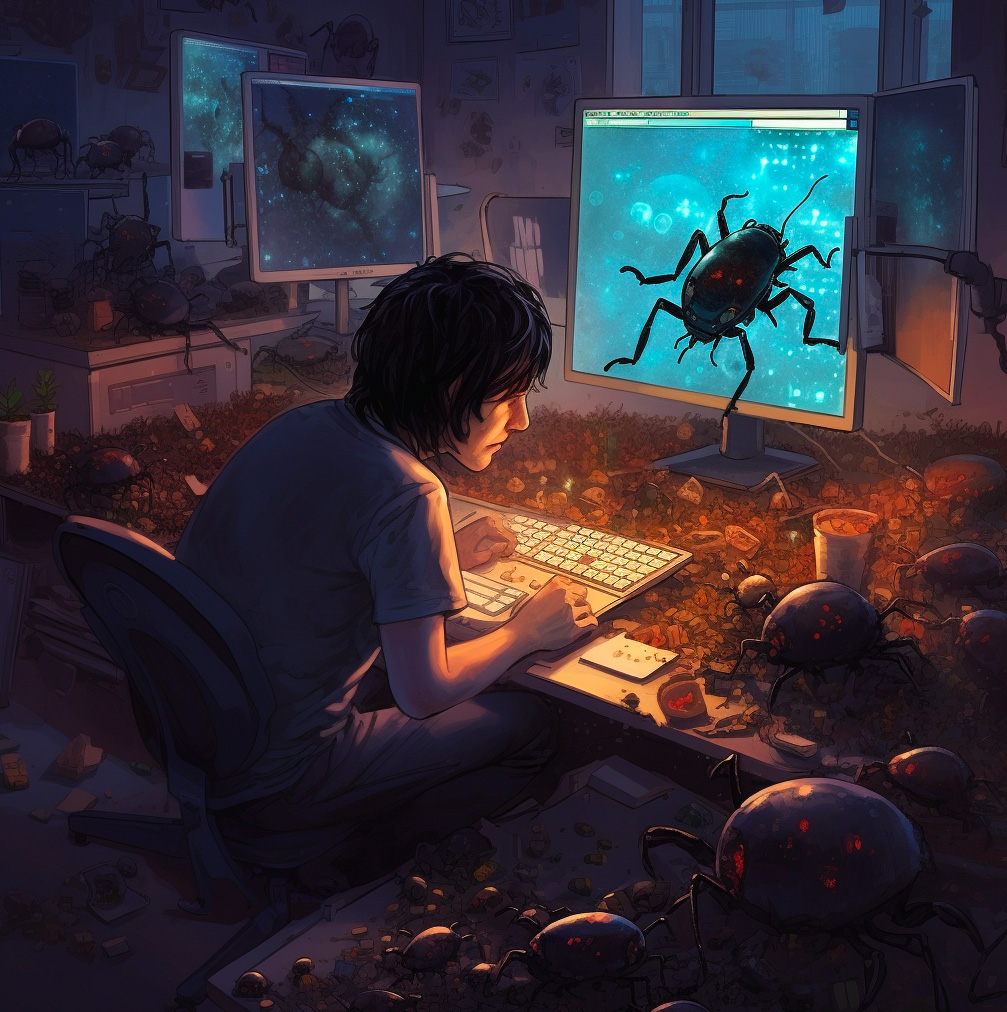
A debugger, in its most basic form, is a tool that enables programmers to place breakpoints in their code. The debugger pauses execution when the code reaches a breakpoint, allowing the developer to examine and modify the code. This makes it simpler to comprehend how the code is acting and to correct mistakes.
A debugger operates by starting the application in a particular mode that gives you control over how it is executed. You can observe how the application behaves and determine whether it is functioning as planned by halting the application at specific locations in the code and stepping through it line by line. Real-time object and variable inspection is another useful tool for troubleshooting.
Basic Debugging Techniques
We’ll examine some fundamental debugging approaches to get you started now that we know what a debugger is and how it functions.
Setting Breakpoints: For debugging, setting breakpoints is crucial. When you wish to investigate a variable or run your code line by line, you may use this feature to halt execution of your code. Simply click on the left side of the line of code you wish to pause in order to establish a breakpoint. The presence of a breakpoint at that line will be indicated by the appearance of a red dot.
Variable Inspection: Variable inspection is also essential for debugging. Most debuggers provide a watch window that you can use to add variables to for inspection, or you can just hover your cursor over the names of variables to do so. A useful tool that shows the current value of a variable is the watch window.
Stepping Through Code: Instead of running your code all at once, stepping through code involves reading it line by line. The Step Over, Step Into, and Step Out functions allow you to step through your code. Step Over enables you to run one line of code before running another. You can enter a function or method call by using the Step Into command. Last but not least, Step Out enables you to exit a function or method call and return to the prior line.
Advanced Debugging Techniques
Breaking on a line of code only when a specific condition is satisfied is possible with conditional breakpoints, a useful tool. A conditional breakpoint, for instance, can only occur when a variable has a specific value. Right-click the breakpoint and choose Edit Breakpoint to accomplish this. Add the desired condition in the window that displays, then click OK.
Watching Expressions: The watch window does more than just show a variable’s current value. Additionally, you can use it to observe expressions, which are calculations with variables. This is a great tool for monitoring certain calculations to make sure they are performing as intended if your code is very complex.
Remote debugging enables you to troubleshoot your code on a machine or server that is not part of your development environment. When working in groups or addressing problems that are unique to deployment scenarios, this is tremendously helpful.
Debugging Pitfalls
Debugging can be a tricky process. Even the most experienced developers can make mistakes. Here are some common errors in debugging:
- Overcomplicating the code: Debugging can be time-consuming, and sometimes developers tend to overcomplicate the code. Simplify the code as much as possible to make debugging easier.
- Not understanding the error message: Error messages can be cryptic, and sometimes it’s tempting to ignore them. However, they often provide essential information, so it’s crucial to understand and address them.
- Not using breakpoints: Breakpoints allow you to pause the code execution at specific points, making debugging more manageable. Using them is crucial when debugging.
Real-World Examples
Now that we’ve covered the basics and advanced techniques of debugging, let’s look at some real-world examples.
Debugging A Simple Node.js Application
The following is a simple Node.js application that logs a message to the console:
console.log('Hello, world!');
To debug this application, you would do the following:
- Create a file named “app.js” containing the code above.
- Open the folder in Visual Studio Code.
- Navigate to the Debug tab and click “Create a launch.json file.”
- Select “Node.js” as your environment.
- Enter the path to your “app.js” file in the “program” field of the launch.json configuration file.
- Set a breakpoint by clicking to the left of the “console.log” line.
- Click “Start Debugging.”
- Your code will run to the point of the breakpoint, and the debugger will pause it there.
Debugging A More Complex Node.js Application
In a more complex Node.js application, the problem may not be immediately apparent, so you need to use a combination of breakpoints, expression watching, and conditional breakpoints. Suppose you have a simple image search application that calls an API to retrieve images and displays them. If the images are not loading, you need to debug your code to find out why.
To debug this application, you could do the following:
- Find the point in your code where the images should be loaded and set a breakpoint there.
- Start the debugger and step through the code using the Step Over function until you find the line of code that isn’t working as expected.
- Add the variable responsible for loading the images to the watch window. As you step through the code, you can watch this variable to see what value it has at each stage of the debugging process.
- Add a conditional breakpoint to the line of code where the images are loaded. This breakpoint will only pause the debugger when the variable responsible for loading the images is undefined or has a wrong value.
With these debugging techniques, you can find the issue and fix it.
Conclusion
In conclusion, debugging is a crucial part of the development process, and as Node.js developers, we need to be well-equipped with debugging tools to make our job easier. In this article, we’ve explored the basics of debugging, advanced debugging techniques, debugging pitfalls, and some real-world examples of debugging in action. With this knowledge, you can take your debugging skills to the next level and become a more efficient and effective Node.js developer.
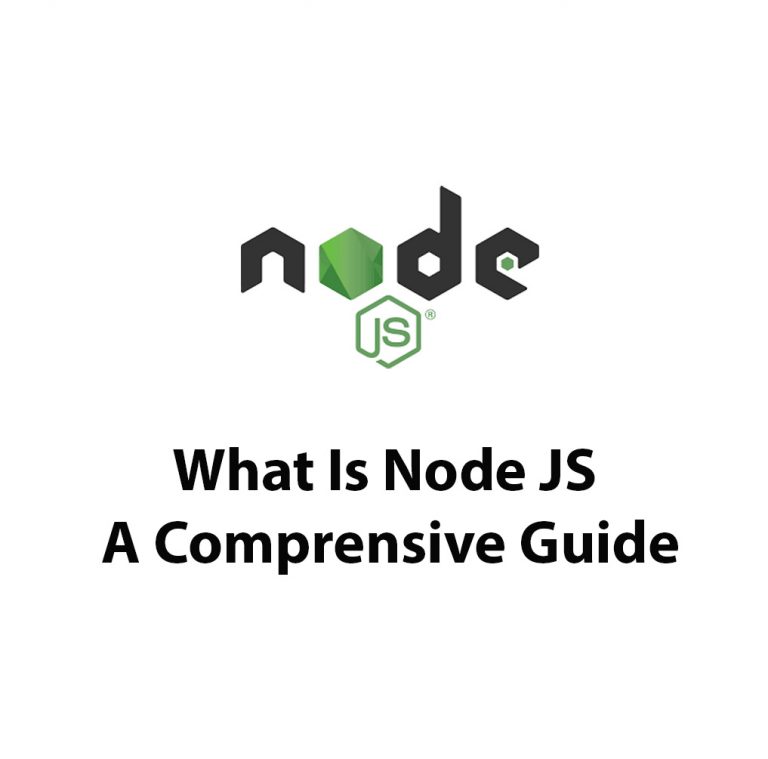
What Is Node JS: A Comprehensive Guide
Introduction As a full-stack developer, I have been working with various technologies over the past few years. But one technology that has caught my attention recently is NodeJS. With its event-driven and non-blocking I/O model, NodeJS has become an excellent choice for building real-time and highly-scalable applications. So, what is NodeJS, and how does it […]
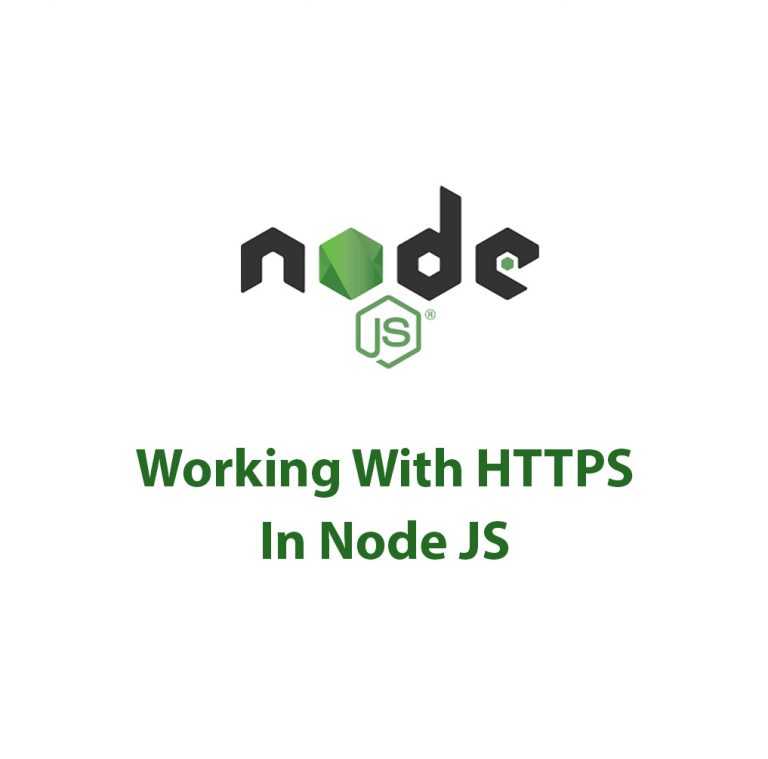
Working With HTTPS In Node JS
As a developer, I’m always on the lookout for security protocols that can help me protect users’ sensitive information. HTTP, while functional, lacks the encryption necessary to truly secure data transmission over the web. This is where HTTPS comes in. But working with HTTPS can be a bit daunting, especially if you’re new to it. […]
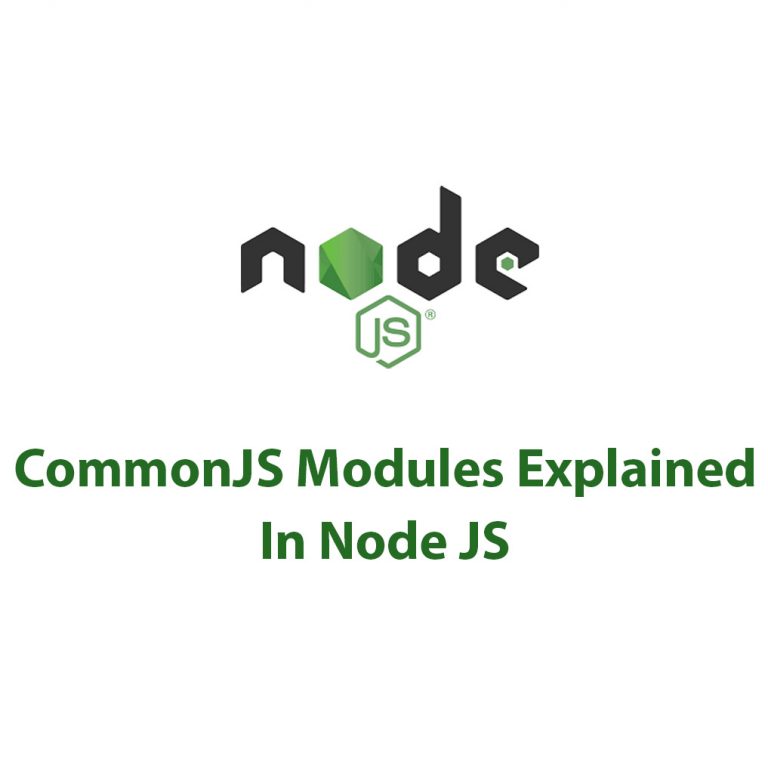
CommonJS Modules In Node JS
Introduction As a developer, I’ve always been interested in the ways that different technologies and tools can work together to create truly powerful and flexible applications. And one of the cornerstones of modern development is the use of modular code – breaking up large, complex programs into smaller, more manageable pieces. One technology that has […]
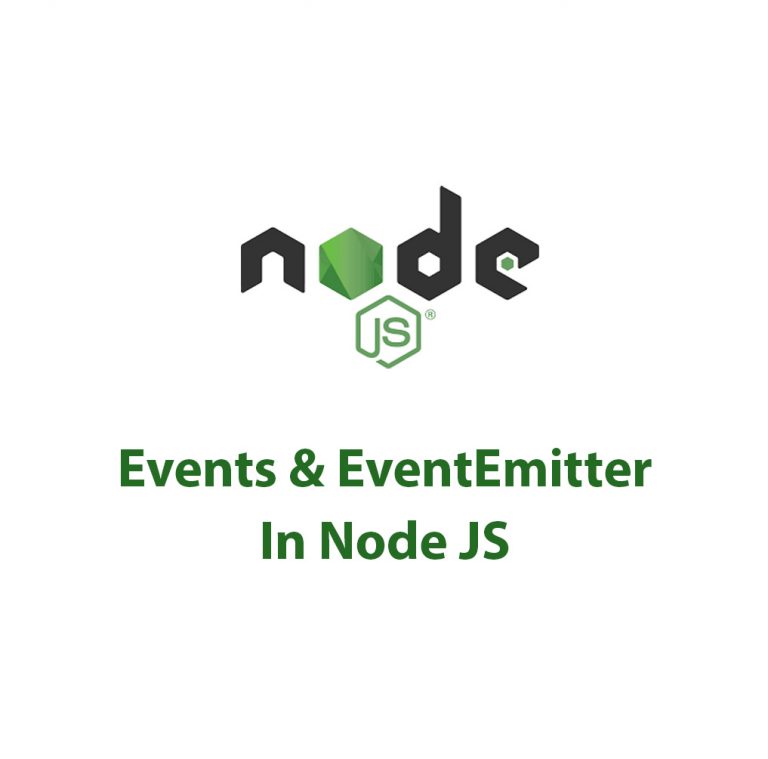
Events In Node JS
Introduction As a Node JS developer, I have often found myself perplexed by events in Node JS. At first, I thought that events were just functions that get triggered when something happens. However, as I delved deeper into this topic, I realized that events are much more powerful and efficient than I had originally thought. […]
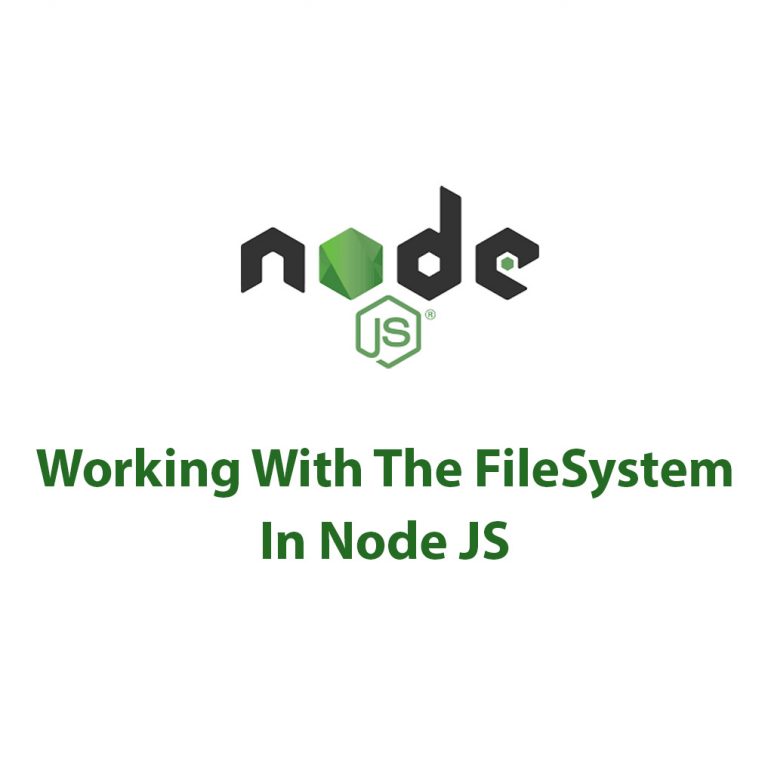
Working With The FileSystem In Node JS
Working With The FileSystem In Node.js Node.js is a powerful platform for building web applications and backend services. One of the most common things that developers need to do is work with files and the file system. This involves creating, modifying, and deleting files, as well as reading from and writing to them. In this […]
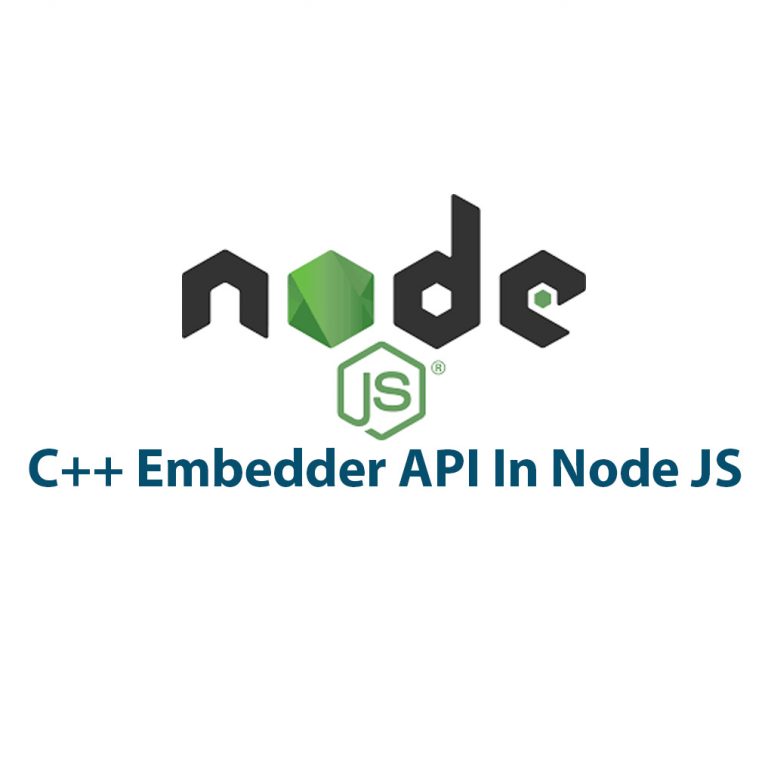
C++ Embedder API With Node JS
Introduction: As a programmer, I have always been fascinated with the power of NodeJS. It is a popular JavaScript runtime that can be used for server-side scripting. The beauty of NodeJS is that it allows for easy handling of I/O operations. However, sometimes the complexities of a project may go beyond just JavaScript coding, and […]