Introduction
As a Node JS developer, I have often found myself perplexed by events in Node JS. At first, I thought that events were just functions that get triggered when something happens. However, as I delved deeper into this topic, I realized that events are much more powerful and efficient than I had originally thought.
In this article, I will discuss everything you need to know about events in Node JS. I will give you a comprehensive overview of what events are, how they work in Node JS, and how you can use them to build event-driven programs. Additionally, I will share some of the best practices for working with events in Node JS.
Understanding Node JS Events
When it comes to programming, events are simply notifications that something has happened. In Node JS, events are an integral part of its architecture and play a critical role in building scalable and efficient applications.
The EventEmitter class is at the core of Node JS’s event-driven architecture. This class provides an interface that allows you to listen to and emit events.
Here’s an example:
const events = require('events');
const emitter = new events.EventEmitter();
emitter.on("message", function(data) {
console.log(`The message is: ${data}`);
});
emitter.emit("message", "Hello, World!");
In this code, we first import the events
module and create a new instance of EventEmitter
using the new
keyword. Then we define a listener function that logs the message that has been passed as data to the message
event. Finally, we emit the message
event with the data “Hello, World!”.
Event Loop in Node JS
Another important aspect of Node JS’s event-driven architecture is its event loop. The event loop is a continuously running loop that checks for events and executes queued tasks.
Here’s a simplified explanation of how the event loop works in Node JS:
- Node JS waits for incoming events
- When an event is detected, Node JS adds the event to the event queue
- Node JS runs the event loop continuously, checking the event queue for new events
- Node JS executes the event handlers for the events that are next in the queue
- After the event handler finishes executing, Node JS removes the event from the queue
This process allows Node JS to handle a large number of requests efficiently without blocking the server or causing it to crash.
Building Event-Driven Programs in Node JS
Now that you have a fundamental understanding of what events are in Node JS, let’s dive deeper into how you can use them to build event-driven programs.
How to create Custom Events in Node JS
One of the most powerful features of Node JS is the ability to create custom events. Custom events allow you to define your own events and trigger them when specific actions occur.
To create a custom event in Node JS, you need to use the EventEmitter
class and define your own event name. Here’s an example:
const EventEmitter = require('events');
const myEmitter = new EventEmitter();
myEmitter.on('myEvent', (data) => {
console.log(`Data received: ${data}`);
});
myEmitter.emit('myEvent', 'Hello!');
In this code example, we create a new EventEmitter
instance, define a new event with the string “myEvent”, and then emit the event with the string “Hello!”. Finally, we pass the emitted string as an argument to the event listener function, which prints “Data received: Hello!” to the console.
How to Listen to Events in Node JS
Listening to events is the equivalent of adding an event handler in JavaScript. To listen to an event in Node JS, you need to use the on()
method of the EventEmitter
class.
myEmitter.on('myEvent', (data) => {
console.log(`Data received: ${data}`);
});
Here, we listen for the myEvent
event and pass in a callback function that will be called whenever the event occurs.
How to Emit Events in Node JS
To trigger an event in Node JS, you need to use the emit()
method of the EventEmitter
class.
myEmitter.emit('myEvent', 'Hello!');
This code will trigger the myEvent
event and will pass the string “Hello!” as an argument to any listener functions attached to the event.
Error Handling in Event-Driven Programming
When building event-driven programs, it’s essential to handle errors effectively. One way to handle errors is by using the error
event provided by the EventEmitter
class.
myEmitter.on('error', (err) => {
console.error('An error occurred:', err);
});
This code listens for any errors that may occur and logs them to the console.
Use Cases for Events in Node JS
There are many use cases for events in Node JS. Here are a few examples:
- Real-time applications: Events can be used to trigger updates in real-time applications such as chat applications or online gaming platforms.
- Chat applications: Events can be used to notify users when a new message is received or when a user leaves the chat.
- Server-side programming: Events can be used to handle requests and responses in server-side programming.
Best Practices for Working with Events in Node JS
Now that you know how to create and listen to events, it’s essential to follow some best practices to ensure that your code is as efficient and scalable as possible.
Naming Conventions for Events in Node JS
When defining custom events, it’s essential to follow a consistent naming convention. The event name should be descriptive and should reflect the action that triggered the event.
myEmitter.on('userLoggedIn', (user) => {
console.log(`User logged in: ${user}`);
});
Here, we define an event called userLoggedIn
that will be triggered when a user logs in to our application.
How to use Event Emitter Correctly
It’s critical to use the EventEmitter
class correctly to avoid performance issues or memory leaks. For example, if you’re creating multiple instances of an EventEmitter
, you should only emit and listen to events on the same instance.
const myEmitter1 = new EventEmitter();
const myEmitter2 = new EventEmitter();
myEmitter1.on('message', (data) => {
console.log(`Data received on myEmitter1: ${data}`);
});
myEmitter1.emit('message', 'Hello!'); // This will print "Data received on myEmitter1: Hello!"
// This will not print anything since there are no event handlers attached to myEmitter2
myEmitter2.emit('message', 'Hello from myEmitter2!');
In this code example, we create two separate instances of EventEmitter
. We attach a listener to myEmitter1
for the message
event, emit the message
event on myEmitter1
with the string “Hello!”, and print the output to the console. We then emit the message
event on myEmitter2
, but since there are no listener functions attached to myEmitter2
, nothing will be printed to the console.
Avoiding Memory Leaks in Event Listeners
One common issue with event listeners is that they can cause memory leaks if they’re not detached properly. To avoid memory leaks, you should always detach event listeners when you no longer need them.
function listener() {
console.log('Hello!');
}
myEmitter.on('myEvent', listener);
myEmitter.emit('myEvent'); // Prints "Hello!"
myEmitter.removeListener('myEvent', listener); // Detaches the listener function from the event
myEmitter.emit('myEvent'); // Does not print anything since the listener function has been detached
In this code example, we define a listener function called listener
, attach it to the myEvent
event using the on()
method, and emit the myEvent
event. We then detach the listener using the removeListener()
method, which removes the listener function from the myEvent
event. Finally, we emit the myEvent
event again, but since the listener function has been detached, nothing is printed to the console.
Monitoring Event Listeners in Production
It’s essential to monitor your event listeners in production to ensure that they’re not consuming too much memory or causing performance issues. One way to monitor event listeners is by using tools like NodeJS’s built-in process
module or third-party monitoring tools like New Relic or AppDynamics.
Here’s an example of how you can use the process
module to monitor event listeners:
const EventEmitter = require('events');
const myEmitter = new EventEmitter();
const listener1 = () => console.log('Listener 1');
const listener2 = () => console.log('Listener 2');
myEmitter
.on('myEvent', listener1)
.on('myEvent', listener2);
// Get the current memory usage
const initialMemory = process.memoryUsage().heapUsed;
// Emit the event multiple times
for (let i = 0; i < 10000; i++) {
myEmitter.emit('myEvent');
}
// Check the memory usage after emitting the event multiple times
const finalMemory = process.memoryUsage().heapUsed;
console.log(`Memory used with 2 listeners: ${finalMemory - initialMemory} bytes`);
In this code example, we define two listener functions and attach them to the myEvent
event using the on()
method. We then emit the myEvent
event 10,000 times using a for
loop and log the memory usage after emitting the event multiple times.
Conclusion
In conclusion, events are a powerful and efficient way to build event-driven programs in Node JS. The EventEmitter
class provides an interface that allows you to listen to and emit events, and it’s essential to follow best practices to ensure that your code is as efficient and scalable as possible.
By using the tips and best practices outlined in this article, you can take full advantage of Node JS’s event-driven architecture and build robust, scalable applications.
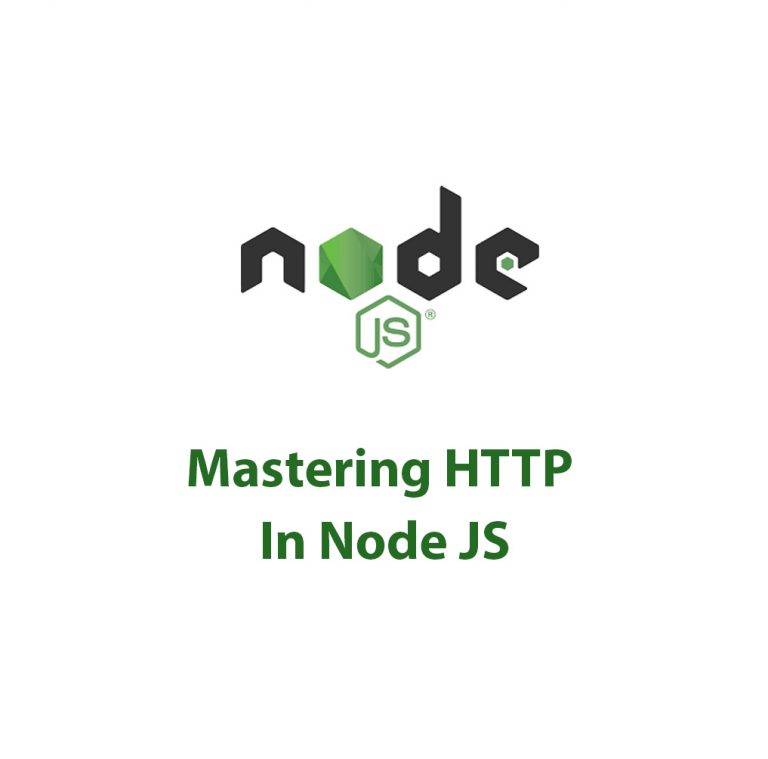
Working With HTTP In Node JS
Introduction As a developer, you may have come across the term HTTP quite a few times. It stands for Hypertext Transfer Protocol and is the backbone of how the internet works. It is the protocol you use when you visit a website, send emails, and watch videos online. In the world of programming, HTTP is […]
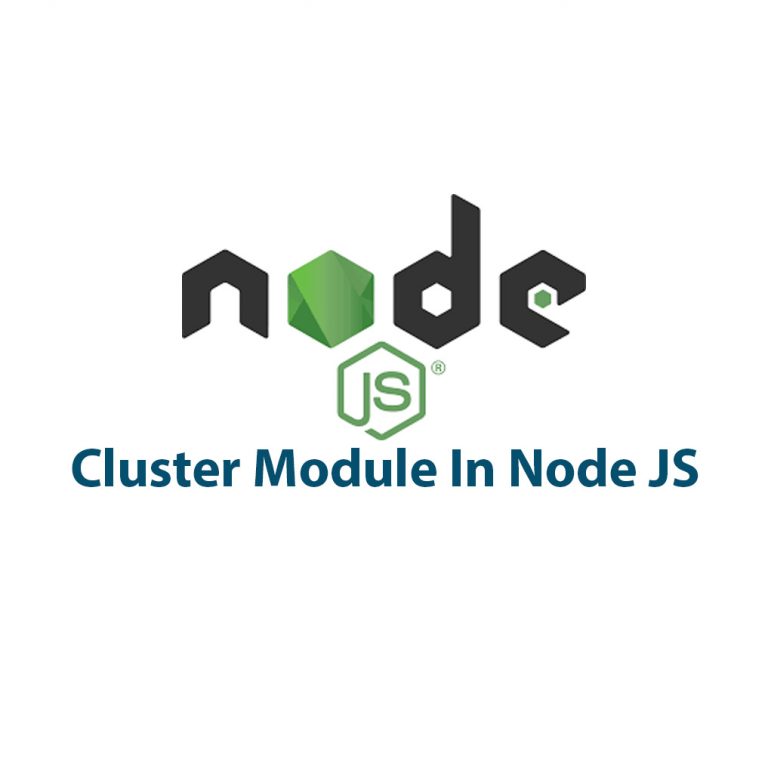
How To Cluster In Node JS
As a developer, I’ve always been interested in exploring different ways to improve the performance of my Node JS applications. One tool that has proven to be immensely beneficial is cluster module. In this article, we’ll dive deep into clustering for Node JS, how it works, how to implement it, and the benefits it provides. […]
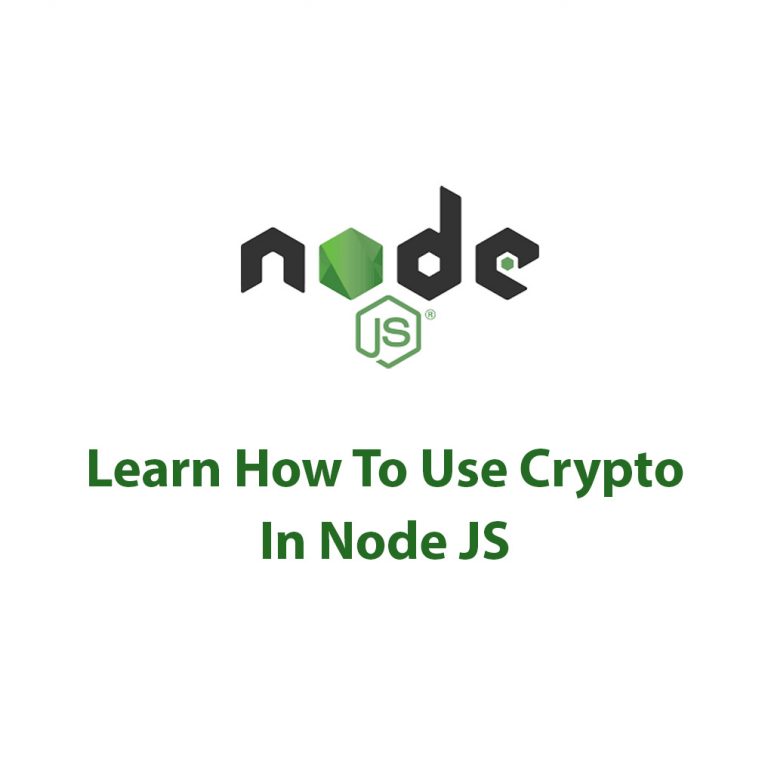
Using Crypto In Node JS
Have you ever wondered how some of the most secure websites and applications keep your data safe from malicious attacks? Well, one of the answers lies in the use of cryptography! Cryptography is the art of writing or solving codes and ciphers, and it has been around for centuries. In the world of computer science, […]
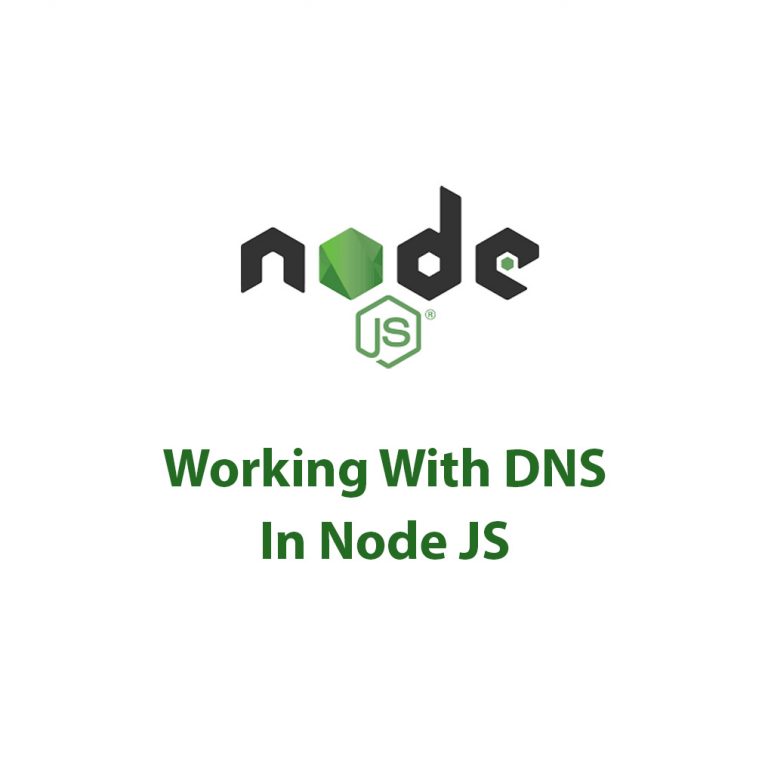
Working With DNS In Node JS
DNS Package in Node JS: A Complete Guide As a web developer, I have always been fascinated by how websites work. I am constantly seeking ways to improve the performance and efficiency of my web applications. One of the critical factors in web development is Domain Name System (DNS). DNS is like a phonebook of […]
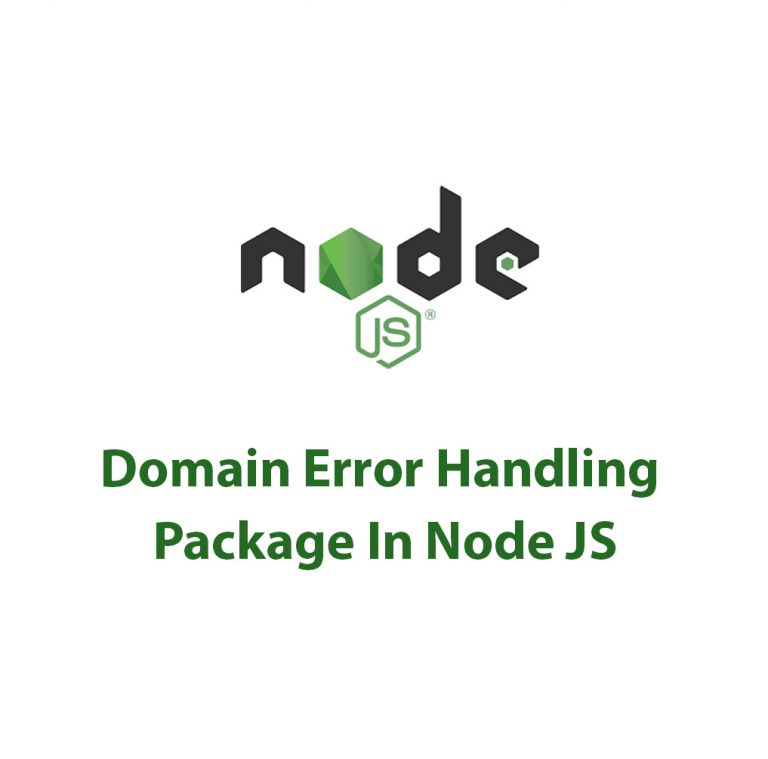
Domain Error Handling Package In Node JS
Domain Package In Node JS Have you ever been annoyed by dealing with mistakes in a Node.js application? It might be difficult for Node.js developers to handle problems when they happen. However, handling errors becomes much simpler with the Node.js Domain package. In this article, I’ll give a general overview of the Node.js Domain package, […]
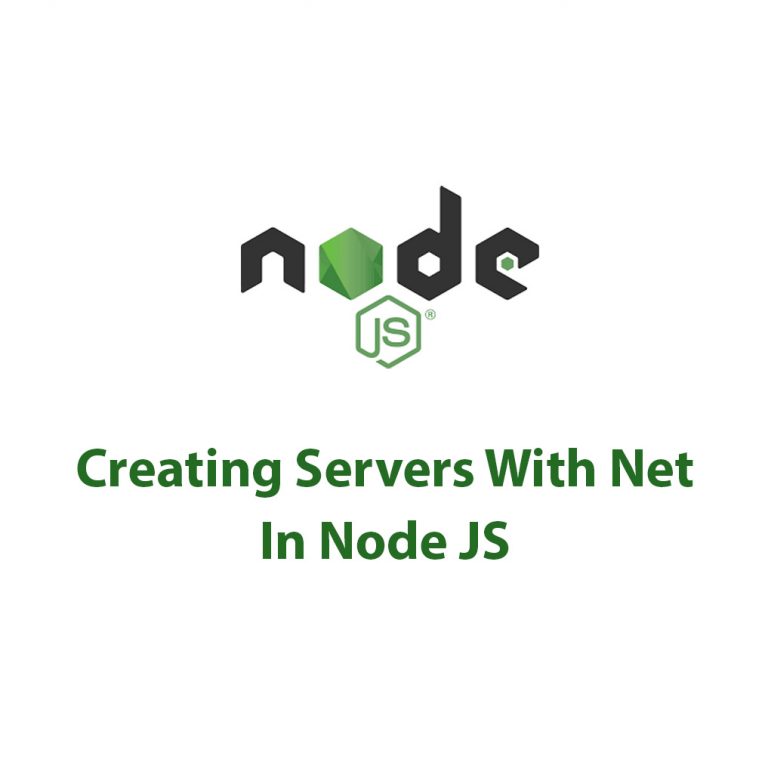
Working With The Net Package In Node JS
As a Node.js developer, I have always been fascinated by the vast array of modules and packages that can be used to simplify the development process. One such package that has long intrigued me is the Net package. In this article, I’ll delve deep into what the Net package is, how to set it up, […]