Introduction
As a developer, you may have come across the term HTTP quite a few times. It stands for Hypertext Transfer Protocol and is the backbone of how the internet works. It is the protocol you use when you visit a website, send emails, and watch videos online. In the world of programming, HTTP is essential for communication between a client and a server.
In this article, I will explore how to work with HTTP in Node.js. Node.js is an open-source, cross-platform, JavaScript runtime environment used to execute JavaScript code on the server side. With Node.js, it is easier to build scalable and high-performance web applications using JavaScript, making it a popular choice among developers.
Before diving into the main topic, let’s start with the basics.
HTTP basics
HTTP is a request-response-based protocol, where a client makes a request to a server, and the server responds with the requested information. The most common request methods are GET, POST, PUT, and DELETE.
GET
The GET method is used to retrieve data from a server. For example, when you visit a webpage, your browser sends a GET request to the server, asking for the webpage’s HTML code.
POST
The POST method is used to submit data to a server. For example, when you fill out a login form and hit the submit button, your browser sends a POST request to the server with the form data.
PUT
The PUT method is used to update data on a server. For example, when you edit your profile information on a website, your browser sends a PUT request to the server, updating your information.
DELETE
The DELETE method is used to delete data from the server. For example, when you want to delete a file from a server, your browser sends a DELETE request to the server.
HTTP Status Codes
HTTP status codes are three-digit numbers returned by a server in response to a client’s request. They indicate the status of the request’s completion, whether it was successful or not. Some popular status codes are:
200: OK
201: Created
400: Bad Request
403: Forbidden
404: Not Found
500: Internal Server Error
HTTP Headers
HTTP headers are additional information sent from the client to the server or vice versa. Headers contain vital information, such as content type, encoding, and authentication.
Node.js
Node.js is a runtime environment used to execute JavaScript code on the server-side. Created by Ryan Dahl in 2009. Node.js uses the V8 engine developed by Google for its execution process. Node.js has a vast array of libraries and modules that make it effortless and straightforward to create server-side applications using JavaScript.
Installing Node.js
To get started with Node.js, you need to download and install the latest version. You can download it from the official Node.js website. Once installed, you can run the Node.js command-line interface (CLI) to execute JavaScript code on your machine.
Creating a Simple HTTP Server
To create a simple HTTP server in Node.js, you need to use the built-in ‘http’ module. Here’s an example of how to create an HTTP server using the ‘http’ module:
const http = require('http');
http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/html' });
res.end('Hello World!');
}).listen(3000);
In this code, we import the ‘http’ module and use the ‘createServer’ method to create a new HTTP server. Inside the ‘createServer’ method, we define a callback function with two parameters, ‘req’ and ‘res’.
The ‘req’ parameter represents the request sent from the client to the server. It contains information about the request, such as the request method, request URL, and request headers.
The ‘res’ parameter represents the response sent from the server to the client. It contains information about the response, such as the response headers and the response data.
In this code, we use the ‘writeHead’ method to set the response status code and content type. We then use the ‘end’ method to send the response data to the client. The server is started on port 3000 using the ‘listen’ method.
To run this code, save it in a file, say “server.js,” and execute it using the Node.js CLI with the command node server.js
. You can then test the server by navigating to “http://localhost:3000” in your browser.
HTTP requests and responses
To handle HTTP requests and responses in Node.js, you need to use the ‘req’ and ‘res’ objects. Here’s an example of how to handle HTTP requests and responses:
const http = require('http');
http.createServer((req, res) => {
console.log(`Received request from ${req.url}`);
res.writeHead(200, { 'Content-Type': 'text/html' });
res.write('<html><body><h1>Hello World!</h1></body></html>');
res.end();
}).listen(3000);
console.log('Server started...');
In this code, we use the ‘createServer’ method to create a new HTTP server. Inside the ‘createServer’ method, we define a callback function with two parameters, ‘req’ and ‘res’.
The ‘req’ parameter represents the request sent from the client to the server. We use the ‘url’ property to log the requested URL to the console.
The ‘res’ parameter represents the response sent from the server to the client. We use the ‘writeHead’ method to set the response status code and content type. We then use the ‘write’ method to send the response data to the client, and finally, the ‘end’ method to end the response.
Handling errors and exceptions
In any application, it is essential to handle errors and exceptions. In Node.js, you can use the ‘try-catch’ block to handle errors and exceptions. Here’s an example of how to handle errors and exceptions:
const http = require('http');
http.createServer((req, res) => {
try {
// Code that may throw an error
} catch (err) {
console.error(`Error occurred: ${err}`);
res.writeHead(500, { 'Content-Type': 'text/html' });
res.write('<html><body><h1>Server Error</h1></body></html>');
res.end();
}
}).listen(3000);
console.log('Server started...');
In this code, we use the ‘try-catch’ block to catch any errors that may occur within the callback function. Inside the catch block, we use the ‘console.error’ method to log the error to the console, and we set the response status code to 500 to indicate a server error. We then send an error message to the client using the ‘write’ method and end the response using the ‘end’ method.
HTTP request methods
In Node.js, you can handle HTTP request methods using the ‘req.method’ property. Here’s an example of how to handle different HTTP request methods:
const http = require('http');
http.createServer((req, res) => {
if (req.method === 'GET') {
// Handle GET requests
} else if (req.method === 'POST') {
// Handle POST requests
} else if (req.method === 'PUT') {
// Handle PUT requests
} else if (req.method === 'DELETE') {
// Handle DELETE requests
} else {
res.writeHead(405, { 'Content-Type': 'text/html' });
res.write('<html><body><h1>Method Not Allowed</h1></body></html>');
res.end();
}
}).listen(3000);
console.log('Server started...');
In this code, we use conditional statements to handle different HTTP request methods. If the request method is not one of the four methods, we set the response status code to 405 to indicate a method not allowed and send an error message to the client.
HTTP headers
In Node.js, you can set HTTP headers using the ‘res.setHeader’ method. Here’s an example of how to set headers in Node.js:
const http = require('http');
http.createServer((req, res) => {
res.setHeader('Content-Type', 'text/html');
res.write('<html><body><h1>Hello World!</h1></body></html>');
res.end();
}).listen(3000);
console.log('Server started...');
In this code, we use the ‘setHeader’ method to set the ‘Content-Type’ header to ‘text/html.’ We then use the ‘write’ method to send the response data to the client and end the response using the ‘end’ method.
Handling cookies in Node.js
Cookies are small pieces of information sent from the server to the client and stored on the client’s machine. In Node.js, you can handle cookies using the ‘cookie-parser’ middleware. Here’s an example of how to use the ‘cookie-parser’ middleware in Node.js:
const http = require('http');
const cookieParser = require('cookie-parser');
http.createServer((req, res) => {
// Parse cookies
const cookies = cookieParser.JSONCookies(req.cookies);
// Read a cookie
const name = cookies.name;
// Set a cookie
res.cookie('name', 'John Doe', { maxAge: 900000 });
res.writeHead(200, { 'Content-Type': 'text/html' });
res.write('<html><body><h1>Hello World!</h1></body></html>');
res.end();
}).listen(3000);
console.log('Server started...');
In this code, we first import the ‘cookie-parser’ middleware. We then use the ‘JSONCookies’ method to parse the cookies sent from the client to the server. We can then read and set cookies using the ‘cookies’ object and the ‘cookie’ method, respectively.
Conclusion
In conclusion, working with HTTP in Node.js is essential for building scalable and high-performance web applications using JavaScript. In this article, we covered the basics of HTTP, explored how to create a simple HTTP server, handle HTTP requests and responses, handle errors and exceptions, handle HTTP request methods, set HTTP headers, and handle cookies in Node.js. With this knowledge, you can now build robust and efficient server-side applications that communicate with clients using the HTTP protocol.
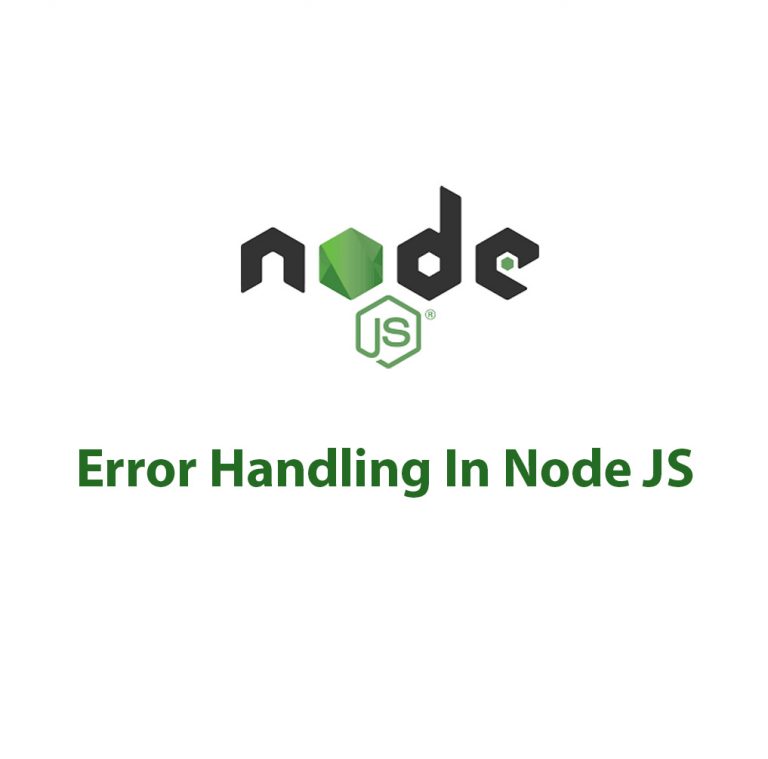
Error Handling in Node JS
A Node JS developer may struggle with error handling on occasion. Although faults are occasionally unavoidable, you can ensure that your application continues to function flawlessly by implementing error handling correctly. Let’s start by discussing the many kinds of problems you could run into when working with Node JS. The Three Types of Errors in […]
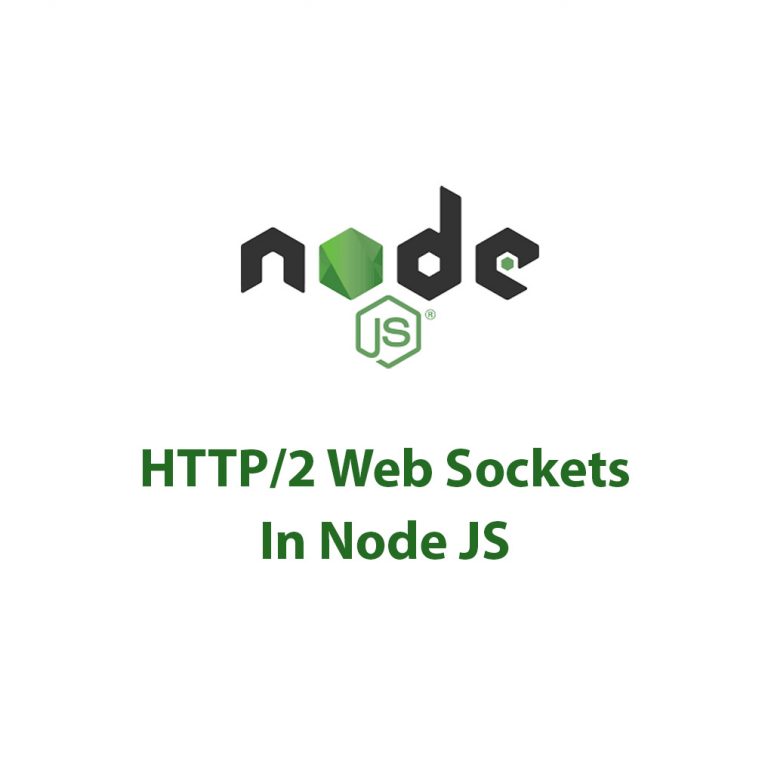
Working With HTTP/2 (Web Sockets) In Node JS
Introduction As a web developer, I’m always on the lookout for improvements in the technology that drives our web applications. Lately, HTTP/2 and WebSockets are getting a lot of attention for their potential to enhance web browsing experiences and make web applications even faster and more dynamic. Both of these specifications are a departure from […]
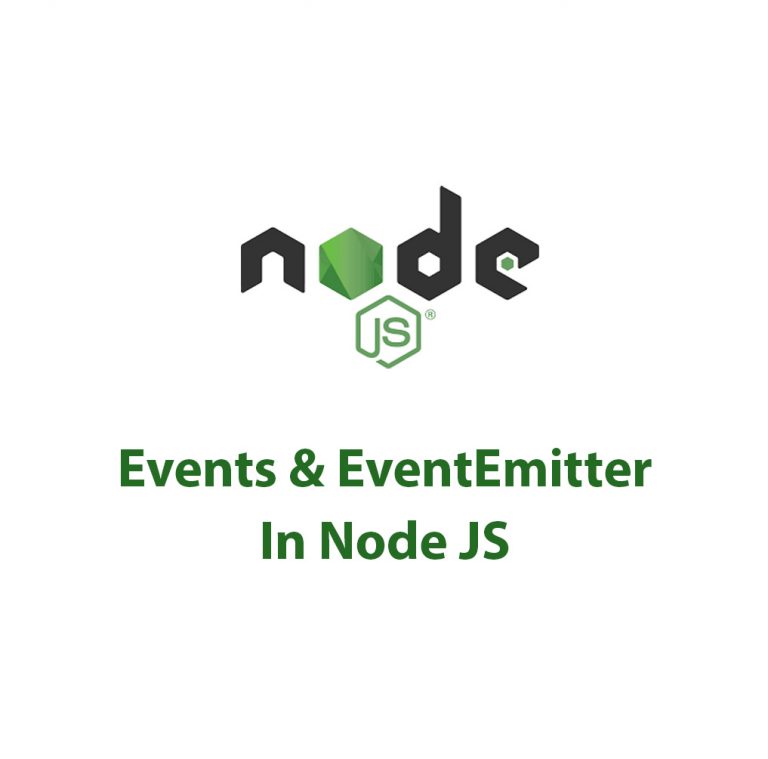
Events In Node JS
Introduction As a Node JS developer, I have often found myself perplexed by events in Node JS. At first, I thought that events were just functions that get triggered when something happens. However, as I delved deeper into this topic, I realized that events are much more powerful and efficient than I had originally thought. […]
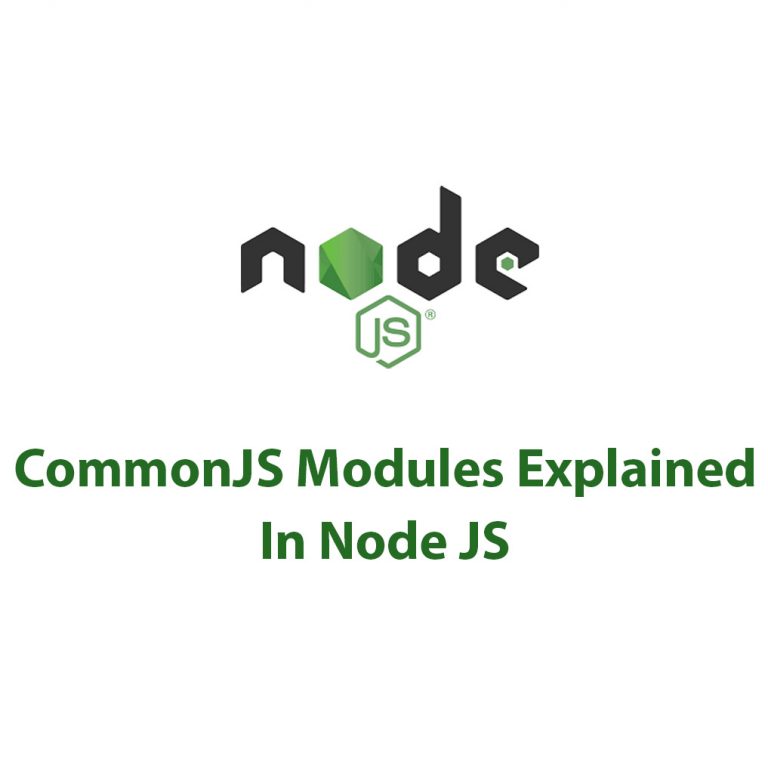
CommonJS Modules In Node JS
Introduction As a developer, I’ve always been interested in the ways that different technologies and tools can work together to create truly powerful and flexible applications. And one of the cornerstones of modern development is the use of modular code – breaking up large, complex programs into smaller, more manageable pieces. One technology that has […]
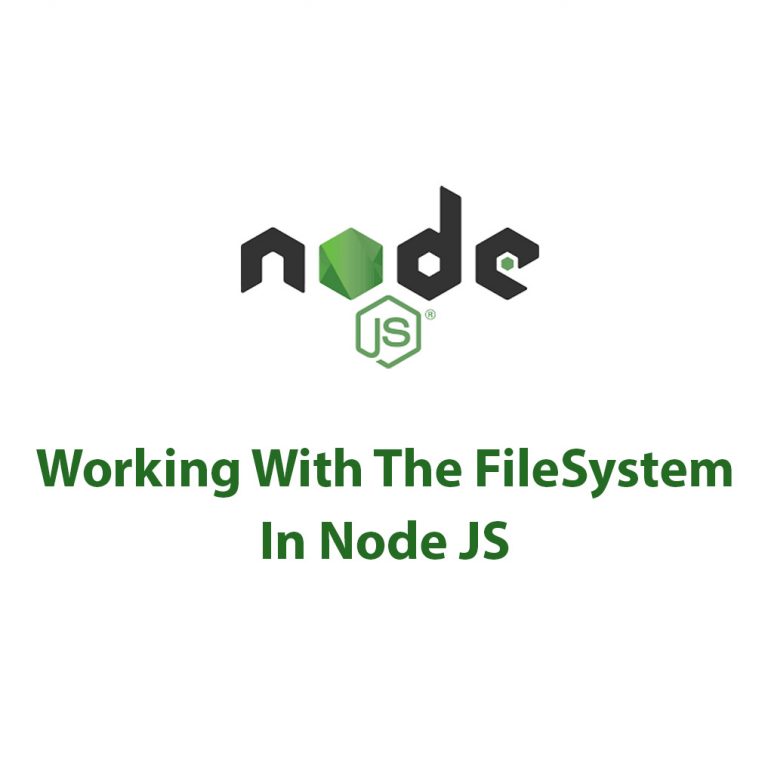
Working With The FileSystem In Node JS
Working With The FileSystem In Node.js Node.js is a powerful platform for building web applications and backend services. One of the most common things that developers need to do is work with files and the file system. This involves creating, modifying, and deleting files, as well as reading from and writing to them. In this […]
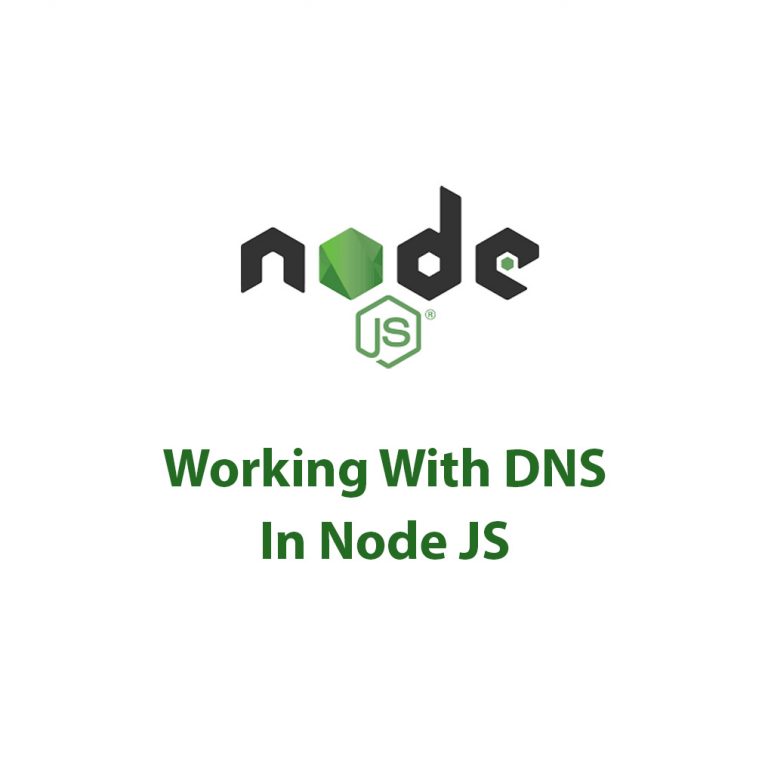
Working With DNS In Node JS
DNS Package in Node JS: A Complete Guide As a web developer, I have always been fascinated by how websites work. I am constantly seeking ways to improve the performance and efficiency of my web applications. One of the critical factors in web development is Domain Name System (DNS). DNS is like a phonebook of […]