Domain Package In Node JS
Have you ever been annoyed by dealing with mistakes in a Node.js application? It might be difficult for Node.js developers to handle problems when they happen. However, handling errors becomes much simpler with the Node.js Domain package. In this article, I’ll give a general overview of the Node.js Domain package, go through its key components, and explain how to use it efficiently.
So let’s get going.
What is the Domain Package in Node.js?
A built-in module in Node.js called the Domain package is intended to deal with mistakes that happen in Node applications. To put it simply, the Domain class offers a method for handling failures that arise during numerous operations in your Node.js application.
You must efficiently manage the mistakes that arise within your program as a developer. The Domain package wraps events and the related event handlers, making faults easier to control.
The Importance of Managing Errors
In any Node.js application, managing errors is essential. Your entire application could be stopped by one mistake during an operation, rendering it unavailable to users. Therefore, managing errors is crucial to ensuring that your program continues to run and function as intended.
Multiple failures can be handled with ease using the Domain Package. Depending on your desire, the mistakes can be caught, logged, forced, or exited from. When an error happens, the program keeps running while handling it to save the system from going offline.
Understanding the Domain Package
Let’s look more closely at how to use the Node.js Domain Package now that you know what it is and how important it is.
The EventEmitter class, a subclass of the Domain class in Node.js, offers a simple method for managing errors in Node applications. Having the ability to associate many callback procedures with a single error event is one of the key advantages of utilizing the Domain class.
Additionally, the Domain class offers a property that enables you to include new members in the Domain. Members that are added to the domain will be contextually bound, which means that when they are called, the domain itself will execute them.
The code below demonstrates how to generate a fresh instance of the Domain class in Node.js:
const domain = require('domain');
const myDomain = domain.create();
After creating a Domain instance, you can add error handlers to the domain by using the myDomain.on('error', handleError)
method.
The handleError
callback function will be called when an error occurs in the Domain instance.
Handling Errors with the Domain Package
Error handling using the Domain package is a simple procedure. To run code inside a domain, first create an instance of the domain, then add event handlers, and then link the domain to the code you want to run inside the domain.
Context-bound callback functions are first added when using the Domain package by executing domain.bind(callback). The bound callback function will then automatically correlate any events it triggers with the Domain instance.
For instance, you can use the following code to link an HTTP request and response to a Domain instance:
const domain = require('domain');
const server = require('http').createServer();
const myDomain = domain.create();
server.on('request', myDomain.bind((req, res) => {
// Handle the request
}));
myDomain.on('error', (err) => {
console.log(`Caught error: ${err}`);
});
In the code above, the server.on
method is used to associate the myDomain.bind
function with the request/response event. Any errors that occur during the execution of the request/response logic will be caught by the error handler in the Domain instance.
Best Practices for Using the Domain Package
Like any method used in Node.js, there are best practices that you should follow when using the Domain package in your applications.
First, it is recommended to use the Domain class for managing errors within individual Node.js processes. If you are dealing with multi-process Node.js applications, using the Domain class is not recommended since it is process-bound.
Secondly, only lexical scope and synchronous calls are supported by the Domain class. Event-driven or asynchronous calls such as fs.readFile
, setTimeout
, and mongodb.connect
are not supported by the Domain class.
Lastly, it is advisable to use a combination of the Domain package and other error handling methods such as try-catch blocks and logging to ensure that the errors in your Node.js application are appropriately managed.
Conclusion
In conclusion, managing errors in Node.js applications can be a daunting task, but with the Domain package, it becomes much simpler. In this article, we have discussed what the Domain package is in Node.js, its importance, and how to use it effectively.
By using the best practices outlined in this article, you can ensure your Node.js application is running smoothly, and errors are handled effectively, preventing your application from crashing and becoming unavailable to users.
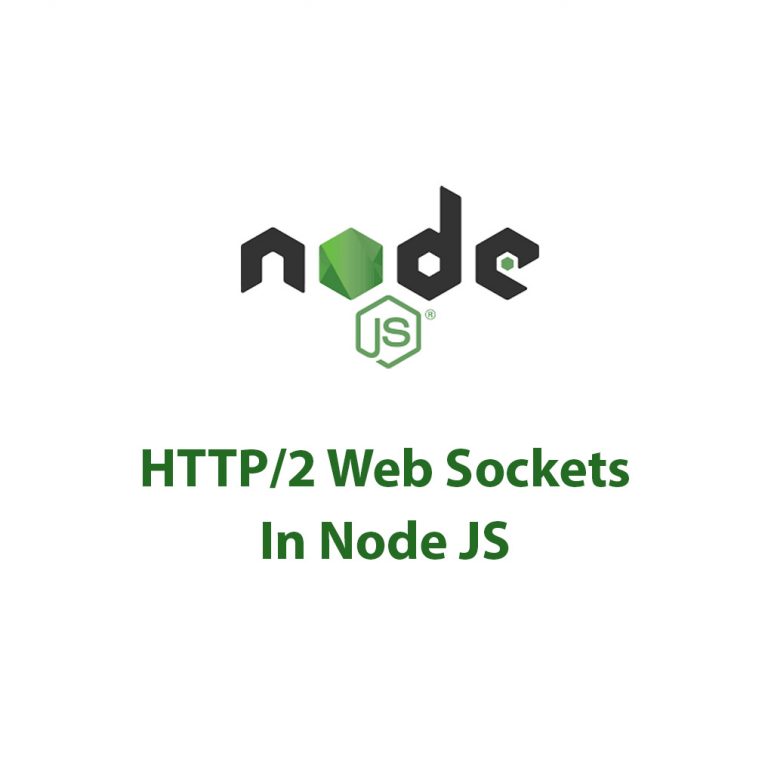
Working With HTTP/2 (Web Sockets) In Node JS
Introduction As a web developer, I’m always on the lookout for improvements in the technology that drives our web applications. Lately, HTTP/2 and WebSockets are getting a lot of attention for their potential to enhance web browsing experiences and make web applications even faster and more dynamic. Both of these specifications are a departure from […]
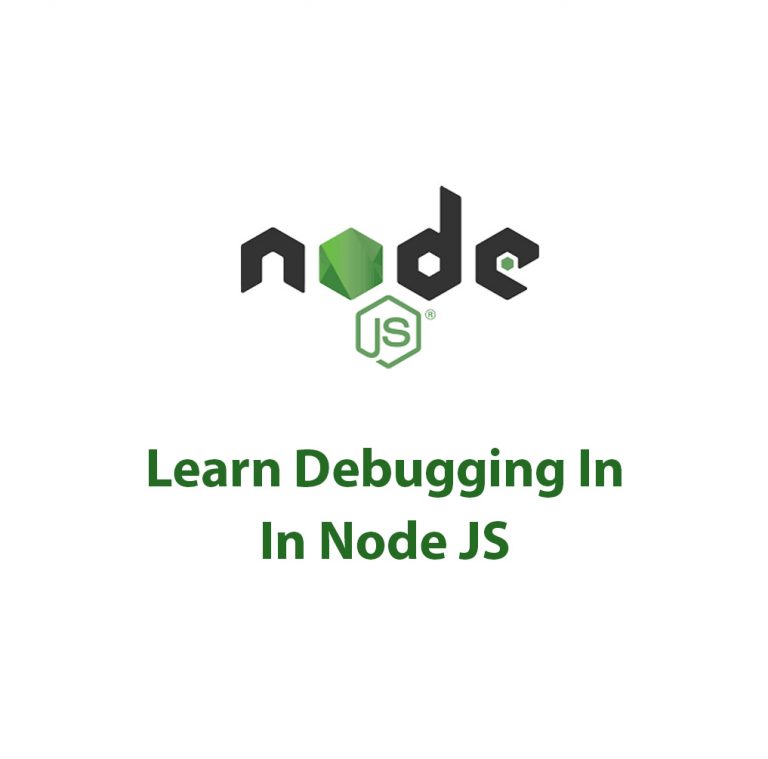
Debugger In Node JS
Debugger In Node JS: Getting a Deeper Understanding One thing we might all have in common as developers in the always changing tech industry is the ongoing need to come up with new and better approaches to debug our code. Since troubleshooting is a crucial step in the development process, we must be well-equipped with […]
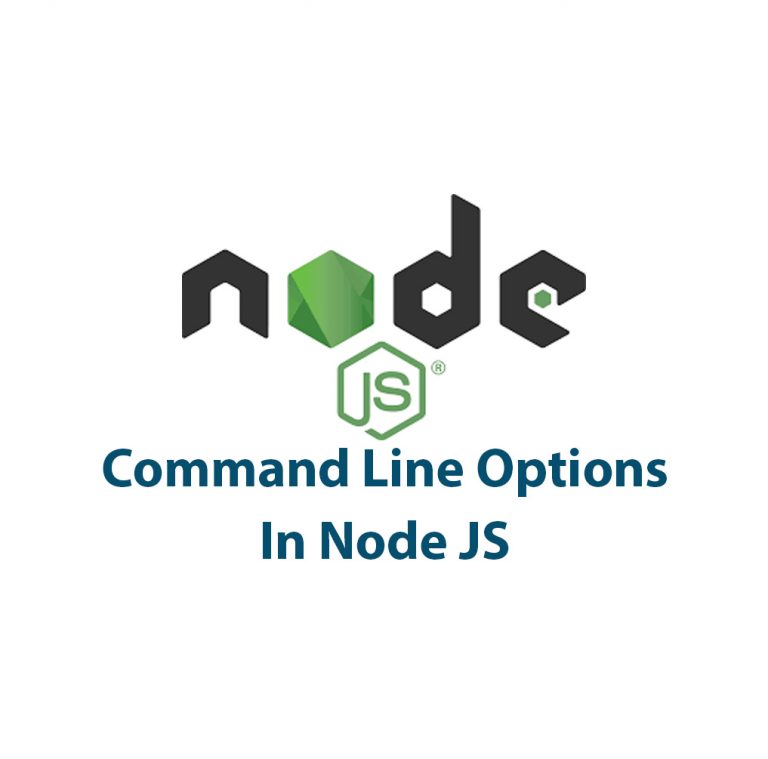
Command Line Options In Node JS
As a developer who loves working with Node JS, I’ve found myself using command line options in many of my projects. Command line options can make our Node JS programs more user-friendly and powerful. In this article, I’ll explain what command line options are, how to parse them in Node JS, how to use them […]
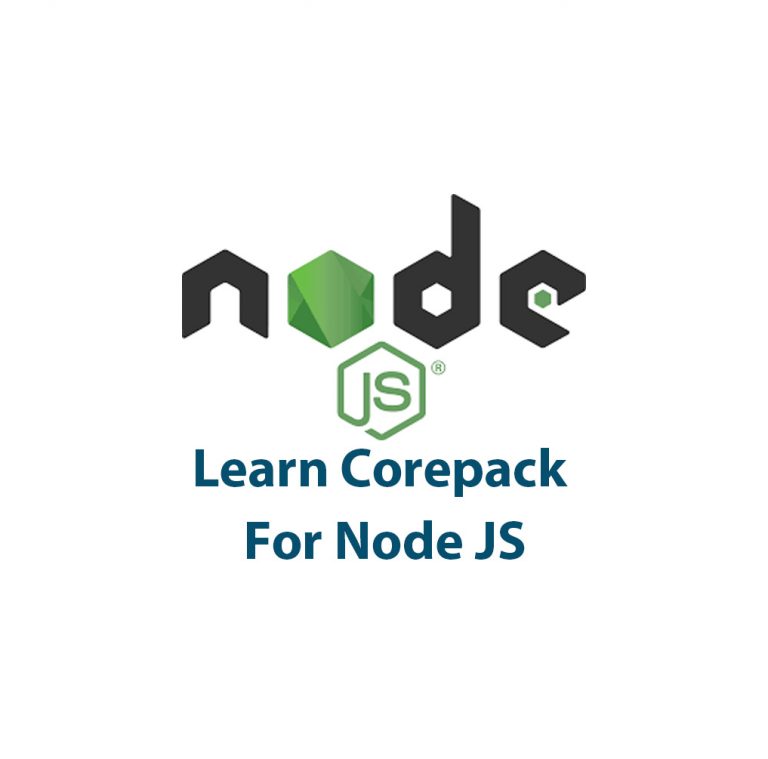
Corepack In Node JS
Have you ever worked on a Node JS project and struggled with managing dependencies? You’re not alone! Managing packages in Node JS can get confusing and messy quickly. That’s where Corepack comes in. In this article, we’ll be diving into Corepack in Node JS and how it can make dependency management a breeze. First of […]
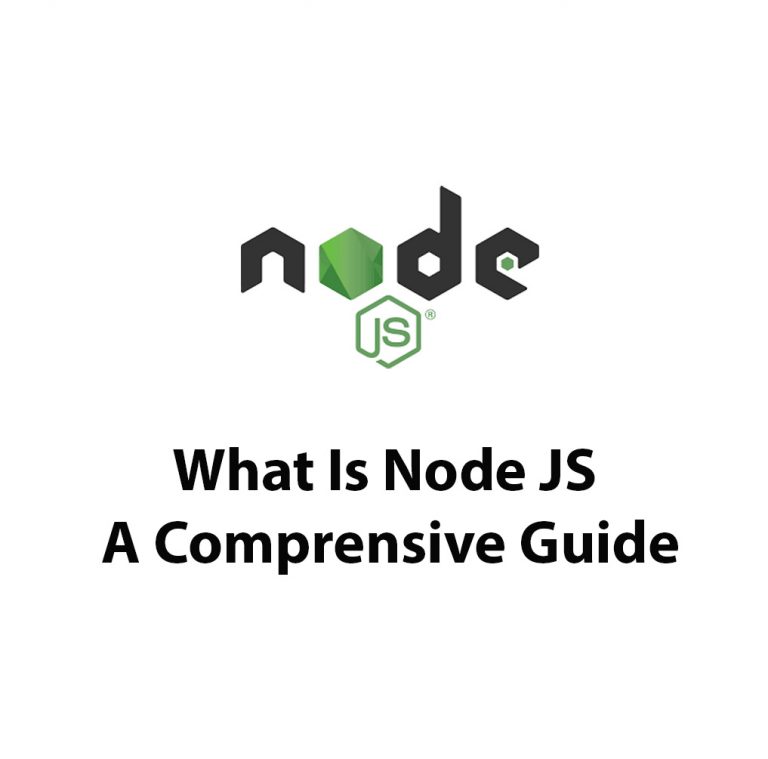
What Is Node JS: A Comprehensive Guide
Introduction As a full-stack developer, I have been working with various technologies over the past few years. But one technology that has caught my attention recently is NodeJS. With its event-driven and non-blocking I/O model, NodeJS has become an excellent choice for building real-time and highly-scalable applications. So, what is NodeJS, and how does it […]
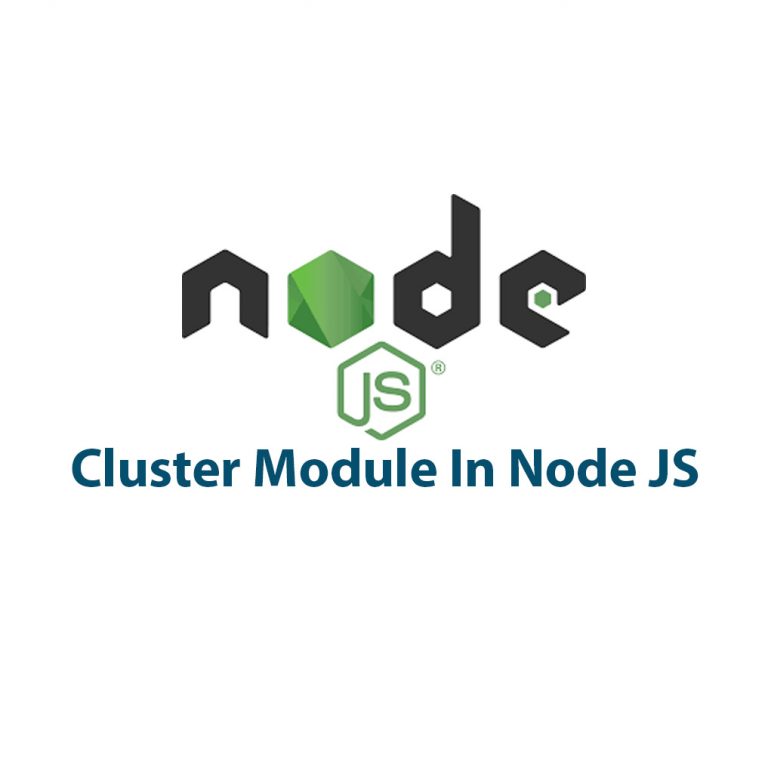
How To Cluster In Node JS
As a developer, I’ve always been interested in exploring different ways to improve the performance of my Node JS applications. One tool that has proven to be immensely beneficial is cluster module. In this article, we’ll dive deep into clustering for Node JS, how it works, how to implement it, and the benefits it provides. […]