Hey there! Today I’m going to talk about Child Processes in Node.js. As you may know, Node.js is a popular open-source, cross-platform, JavaScript runtime environment. It offers a lot of features out of the box, one of them being the ability to work with Child Processes.
Child Processes allow you to run multiple processes simultaneously, and each of them can run separately from the main process. They offer a way to create new processes that are connected to the main Node.js process but operate as if they were separate programs.
Let’s dive into the basics of creating Child Processes.
Creating Child Processes
There are multiple ways to create Child Processes in Node.js, one of them being by using the Child Process class. This class provides the spawn, fork and exec functions for creating child processes.
Spawn is used to spawn a new process and execute a command in it. For example, we can use the spawn function to execute the “ls” command and list all the files inside a directory like this:
const { spawn } = require('child_process');
const child = spawn('ls', ['-la']);
The first parameter of spawn is the command that should be executed and the second is optional and it’s an array of arguments that need to be passed to the command. In this example, we are passing the “-la” argument to the “ls” command, which lists all the files and directories in a long listing format.
Fork, on the other hand, is used to spawn a new Node.js process and execute a module in it. It’s similar to spawn but the important difference is that it will automatically create a communication channel between the parent and child processes. For example:
const { fork } = require('child_process');
const child = fork('/path/to/module.js');
The fork function receives the path to the module that needs to be executed as an argument. It’s important to note that the module needs to be a valid Node.js application with a main function that can be called.
Exec function, just like spawn, is used to spawn a new process. The difference is that it will execute a command and buffer all the output. For example:
const { exec } = require('child_process');
exec('ls -la', (error, stdout, stderr) => {
if (error) {
console.error(`Error: ${error}`);
return;
}
console.log(`stdout: ${stdout}`);
console.log(`stderr: ${stderr}`);
});
In this example, we are executing the same “ls” command as in the previous example. The exec function receives a command as a parameter and a callback function that will be called when the execution finishes.
Now that we know the different methods for creating Child Processes, let’s take a look at how we can communicate with them.
Communication between Parent and Child Processes
When using Child Processes, it’s important to be able to communicate between the parent and child processes. There are three ways to do this in Node.js: stdio (Standard Input/Output), event listening and messages.
Stdio is a way of communicating through the standard input, output, and error streams between the parent and child processes. Here’s how it works:
const { spawn } = require('child_process');
const child = spawn('pwd', [], {
stdio: 'inherit'
});
In this example, we are running the “pwd” command to get the current working directory. By setting the stdio option to ‘inherit’, we are telling the child process to use the same standard input, output, and error streams as the parent process.
Event Listening is another way of communicating between parent and child processes. Here’s an example:
const { fork } = require('child_process');
const child = fork('/path/to/module.js');
child.on('message', (message) => {
console.log(`Parent process received message: ${message}`);
});
child.send('Hello from parent process');
In this example, we are using the fork function to start a new Node.js process and execute a module. The “message” event is triggered when a child process sends a message to the parent process. In this case, we are sending the “Hello from parent process” message.
Messages are yet another way of communicating between parent and child processes. Messages can be of any type such as objects, strings or buffers. The parent process can send a message to a child process like this:
const { fork } = require('child_process');
const child = fork('/path/to/module.js');
child.on('message', (message) => {
console.log(`Child process received message: ${message}`);
});
child.send('Hello from child process');
In this example, we are using the fork function to start a new Node.js process and execute a module. The “message” event is triggered when a parent process sends a message to the child process. In this case, we are sending the “Hello from child process” message.
Now that we know how to communicate between parent and child processes, let’s take a look at some use cases for Child Processes.
Examples of Use Cases for Child Processes
Concurrent Execution of Tasks:
One of the main reasons to use Child Processes is to allow multiple tasks to be executed concurrently. For example:
const { fork } = require('child_process');
const child1 = fork('/path/to/module1.js');
const child2 = fork('/path/to/module2.js');
In this example, we are using the fork function to start two new Node.js processes and execute two different modules concurrently.
Resource-Intensive Tasks:
Another reason to use Child Processes is to run long-running, CPU-intensive, or memory-intensive tasks in a process that is separate from the main process. For example:
const { fork } = require('child_process');
const child = fork('/path/to/module.js');
In this example, we are using the fork function to start a new Node.js process and execute a module that performs a resource-intensive task such as image processing or video transcoding.
Separation of Concerns:
Sometimes it’s useful to separate functionality into different processes. For example, if you have a server application that needs to perform multiple tasks such as file upload, handling WebSocket connections, and database queries, you could separate each task into a separate Child Process.
const { fork } = require('child_process');
const child = fork('/path/to/module.js');
```
In this example, we are using the fork function to start a new Node.js process and execute a module that performs a resource-intensive task such as image processing or video transcoding.
Separation of Concerns:
Sometimes it's useful to separate functionality into different processes. For example, if you have a server application that needs to perform multiple tasks such as file upload, handling WebSocket connections, and database queries, you could separate each task into a separate Child Process.
```JavaScript
const { fork } = require('child_process');
const child1 = fork('/path/to/file-upload-module.js');
const child2 = fork('/path/to/websocket-connection-module.js');
const child3 = fork('/path/to/database-query-module.js');
In this example, we are using the fork function to start three new
Node.js processes and execute three different modules that handle file uploads, WebSocket connections, and database queries respectively.
Now that we have some practical examples of how Child Processes can be used, let’s take a look at some best practices when working with them.
Best Practices when Working with Child Processes
Avoiding Deadlocks and Starvation:
Deadlocks and Starvation can occur when Child Processes are waiting for input from the parent process and the parent process is waiting for the Child Processes to complete. This can create a situation where neither the parent nor the child processes can proceed.
To avoid these issues, it’s important to ensure that messages are being passed between the parent and child processes in a timely manner. This can be done by setting up timeouts or by using an event-driven architecture.
Proper Error handling:
It’s important to handle errors properly when working with Child Processes. This means that in the event of an error, the parent process should handle it gracefully and provide feedback to the user.
Reducing Overhead:
Child Processes can be resource-intensive, so it’s important to use them wisely. One way to reduce overhead is to reuse Child Processes where possible instead of creating new ones for each task.
Now that we know how to use Child Processes and best practices for working with them, let’s take a look at some code snippets to put all this information into practice.
// Creating a child process using spawn function
const { spawn } = require('child_process');
const child = spawn('ls', ['-la']);
child.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
child.stderr.on('data', (data) => {
console.error(`stderr: ${data}`);
});
child.on('error', (error) => {
console.error(`error: ${error.message}`);
});
child.on('close', (code) => {
console.log(`child process exited with code ${code}`);
});
In this example, we are creating a Child Process using the spawn function. We are running the “ls” command to list all the files and directories in a long listing format. We are listening to the stdout and stderr events to log the output in the console. We are also handling errors and the close event.
// Creating a child process using fork function
const { fork } = require('child_process');
const child = fork('/path/to/module.js');
child.on('message', (message) => {
console.log(`parent process received message: ${message}`);
});
child.send('Hello from parent process');
In this example, we are creating a Child Process using the fork function. We are executing a module and sending a message to the Child Process. We are also listening to the message event to log the response in the console.
Conclusion
In conclusion, Child Processes are a powerful feature of Node.js that allow you to run multiple processes simultaneously and communicate between them through stdio, event listening, and messages.
By using Child Processes, you can perform long-running, resource-intensive tasks in a separate process, allow multiple tasks to be executed concurrently, and separate functionality into different processes, among other use cases.
When working with Child Processes, it’s important to avoid deadlocks and starvation, handle errors properly, and reduce overhead.
I hope this article has provided you with a solid introduction to Child Processes in Node.js and has inspired you to explore this powerful feature further.
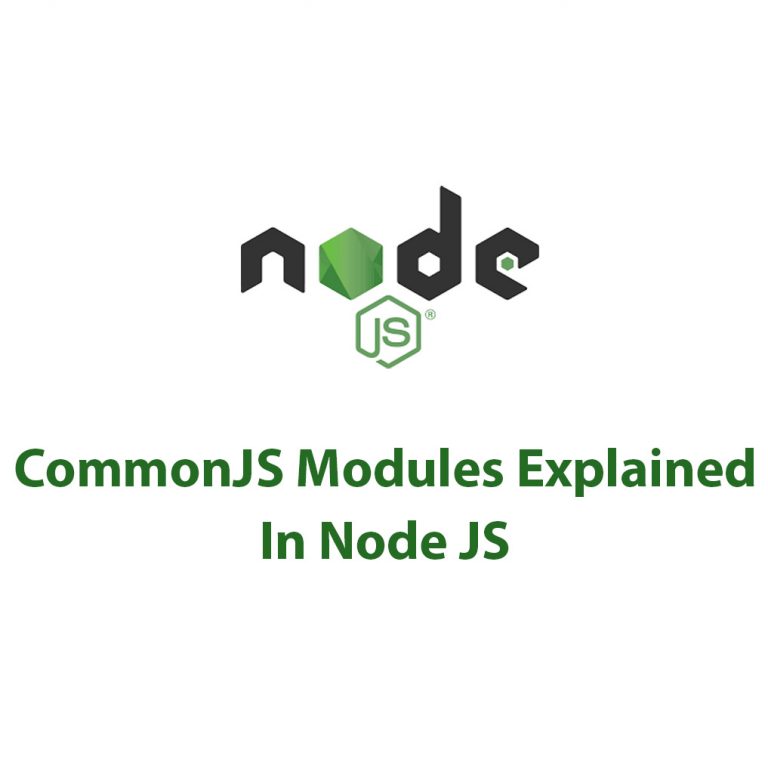
CommonJS Modules In Node JS
Introduction As a developer, I’ve always been interested in the ways that different technologies and tools can work together to create truly powerful and flexible applications. And one of the cornerstones of modern development is the use of modular code – breaking up large, complex programs into smaller, more manageable pieces. One technology that has […]
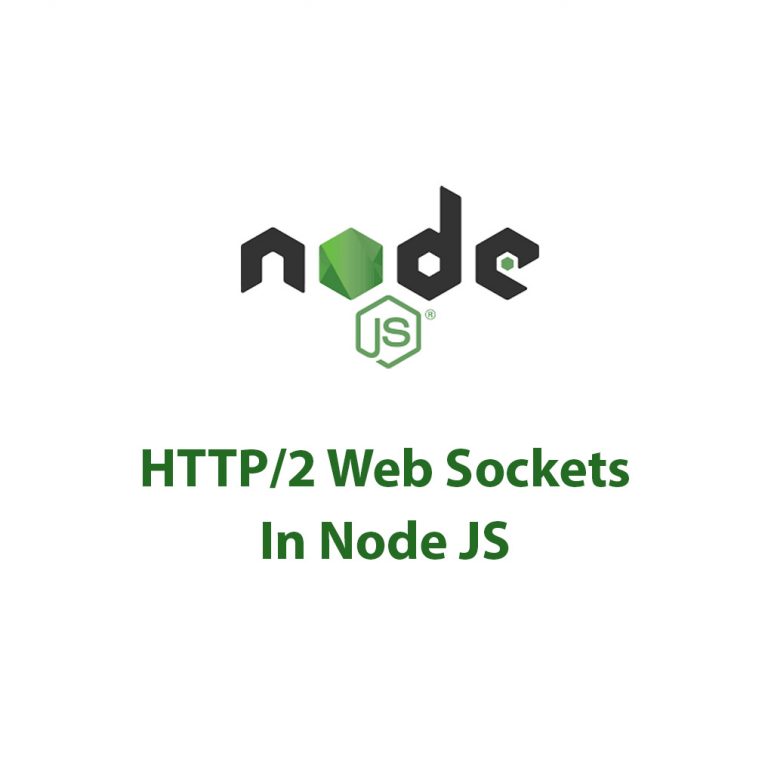
Working With HTTP/2 (Web Sockets) In Node JS
Introduction As a web developer, I’m always on the lookout for improvements in the technology that drives our web applications. Lately, HTTP/2 and WebSockets are getting a lot of attention for their potential to enhance web browsing experiences and make web applications even faster and more dynamic. Both of these specifications are a departure from […]
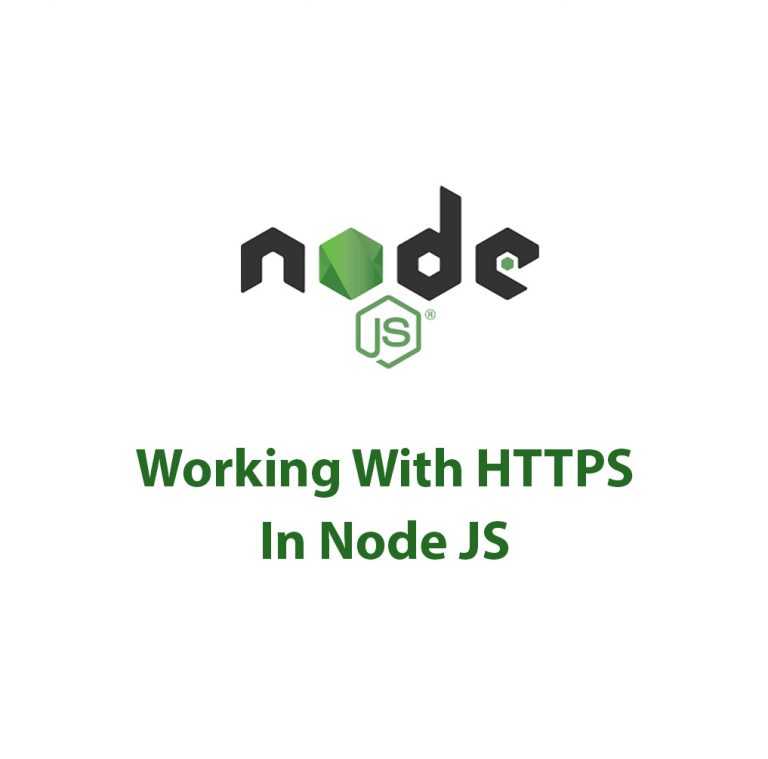
Working With HTTPS In Node JS
As a developer, I’m always on the lookout for security protocols that can help me protect users’ sensitive information. HTTP, while functional, lacks the encryption necessary to truly secure data transmission over the web. This is where HTTPS comes in. But working with HTTPS can be a bit daunting, especially if you’re new to it. […]
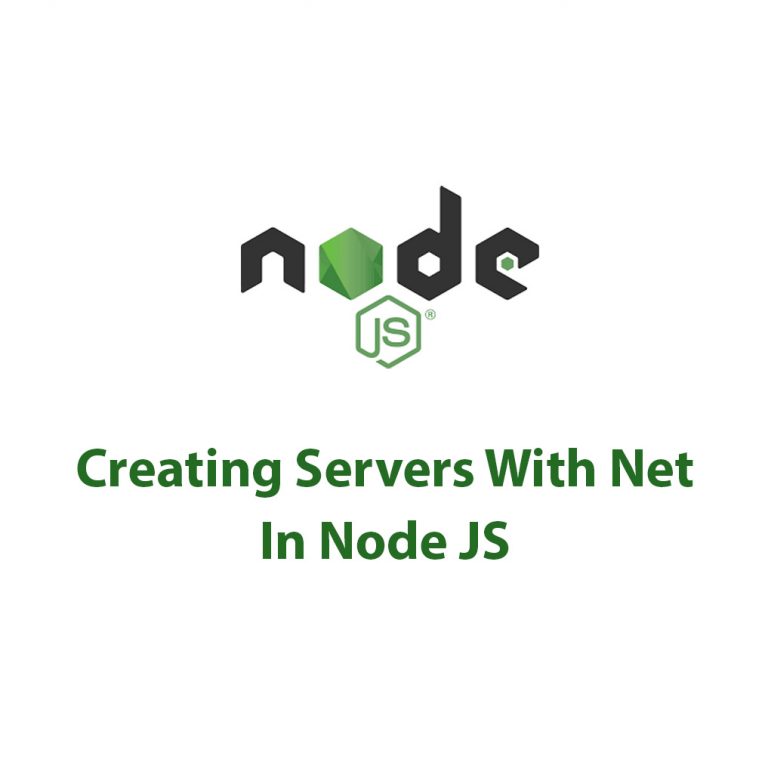
Working With The Net Package In Node JS
As a Node.js developer, I have always been fascinated by the vast array of modules and packages that can be used to simplify the development process. One such package that has long intrigued me is the Net package. In this article, I’ll delve deep into what the Net package is, how to set it up, […]
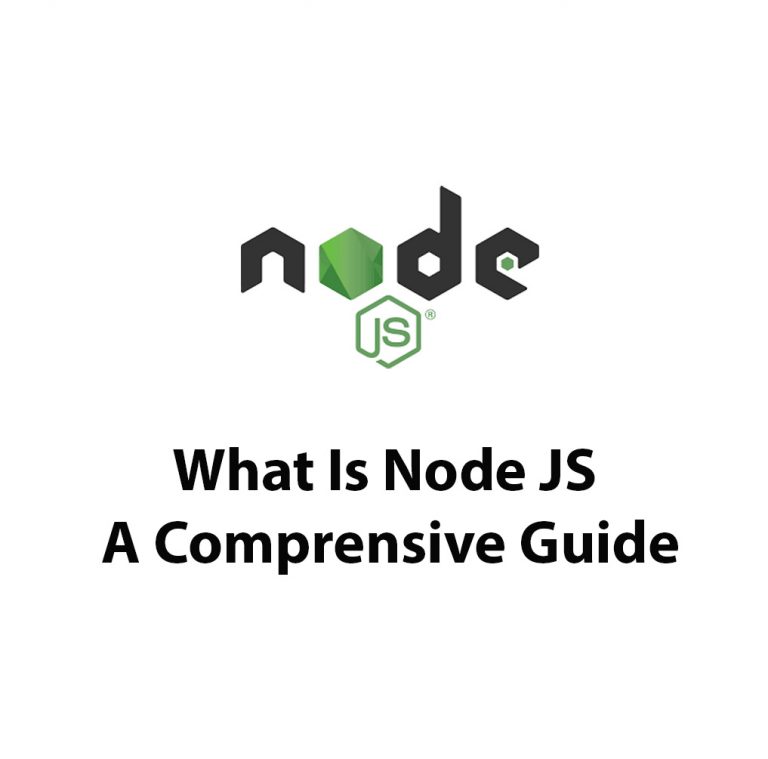
What Is Node JS: A Comprehensive Guide
Introduction As a full-stack developer, I have been working with various technologies over the past few years. But one technology that has caught my attention recently is NodeJS. With its event-driven and non-blocking I/O model, NodeJS has become an excellent choice for building real-time and highly-scalable applications. So, what is NodeJS, and how does it […]
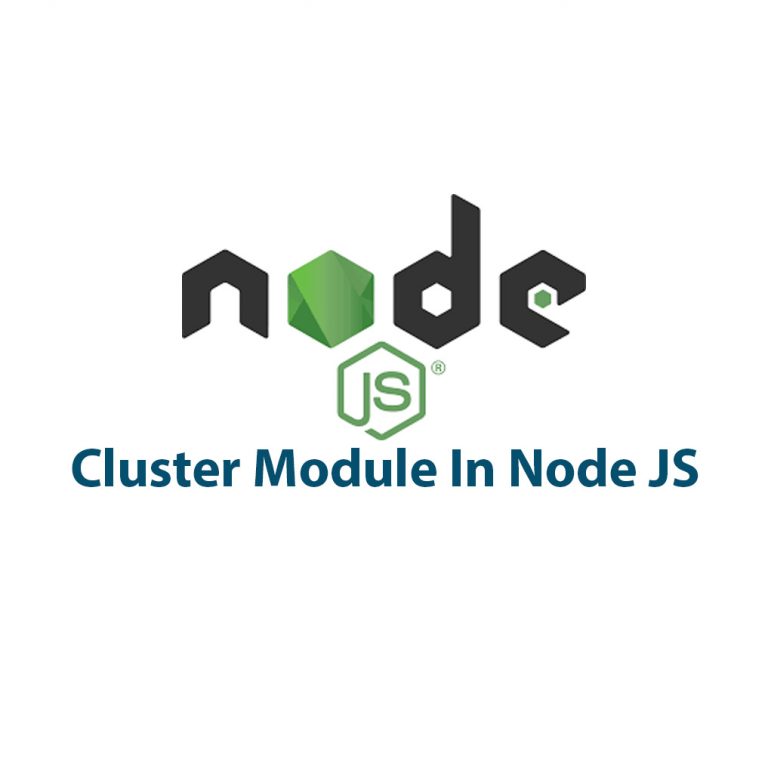
How To Cluster In Node JS
As a developer, I’ve always been interested in exploring different ways to improve the performance of my Node JS applications. One tool that has proven to be immensely beneficial is cluster module. In this article, we’ll dive deep into clustering for Node JS, how it works, how to implement it, and the benefits it provides. […]